What Does ‘Exception Has Been Thrown by the Target of Invocation’ Mean and How Can You Resolve It?
In the world of software development, encountering errors and exceptions is an inevitable part of the journey. Among the myriad of error messages that developers might face, one that often raises eyebrows is the cryptic phrase: “An exception has been thrown by the target of an invocation.” This message can be both frustrating and perplexing, as it often signifies deeper issues within the code that require careful investigation. Understanding this exception not only aids in troubleshooting but also enhances a developer’s ability to write more robust and error-resistant applications.
When this exception occurs, it typically indicates that a method invoked via reflection has encountered an issue. Reflection, a powerful feature in many programming languages, allows developers to inspect and manipulate object types at runtime. However, the complexity of this feature can lead to unexpected errors, making it crucial for developers to grasp the underlying principles that govern these exceptions. By delving into the causes and implications of this specific error message, developers can gain valuable insights into their code’s behavior and improve their debugging skills.
In the following sections, we will explore the common scenarios that lead to this exception, the potential pitfalls of using reflection, and best practices for handling and preventing such errors. Whether you’re a seasoned developer or just starting out, understanding this exception will empower you to navigate the
Understanding the Exception
The phrase “exception has been thrown by the target of invocation” typically indicates that an error occurred during the execution of a method invoked via reflection in programming languages such as C. Reflection allows for inspection and invocation of types and members at runtime, but it can lead to complications if the called method encounters issues. This error is a wrapper around the original exception thrown by the method being invoked.
Key considerations include:
- Underlying Exception: The actual error that caused this message may be obscured. It is crucial to inspect the inner exception for detailed information regarding the issue.
- Invocation Target: The method that was invoked may not be designed to handle certain inputs or states, leading to exceptions such as `ArgumentNullException`, `InvalidOperationException`, or custom exceptions.
Common Causes
Several scenarios can trigger this exception:
- Null References: Attempting to invoke a method on a null object reference.
- Type Mismatch: The parameters provided to the invoked method do not match the expected types.
- Method Access Issues: The method being invoked may not be accessible due to visibility modifiers, such as private or protected.
- Invalid State: The invoked method may rely on certain conditions being met that are not satisfied at the time of invocation.
Handling the Exception
To effectively handle this exception, consider implementing the following strategies:
- Try-Catch Blocks: Enclose the reflective call within a try-catch block to gracefully handle exceptions.
- Inspect Inner Exception: Always examine the inner exception to pinpoint the root cause of the failure.
- Parameter Validation: Ensure that parameters are validated before invoking methods reflectively.
Example of a try-catch implementation:
“`csharp
try
{
MethodInfo methodInfo = typeof(MyClass).GetMethod(“MyMethod”);
methodInfo.Invoke(instance, parameters);
}
catch (TargetInvocationException ex)
{
// Log the inner exception
Console.WriteLine(ex.InnerException?.Message);
}
“`
Error Handling Best Practices
When dealing with exceptions thrown by invocation targets, adhering to best practices can enhance error management:
- Logging: Implement logging mechanisms to capture exception details for troubleshooting.
- User Feedback: Provide meaningful feedback to users in case of errors, avoiding technical jargon.
- Fallback Procedures: Develop fallback procedures to handle failures gracefully, such as retry mechanisms.
Exception Type | Description |
---|---|
ArgumentNullException | Thrown when a null argument is passed to a method that does not accept it. |
InvalidOperationException | Indicates that a method call is invalid for the object’s current state. |
TargetInvocationException | Occurs when an invoked method throws an exception. Contains the original exception. |
By understanding the nuances of this exception and implementing robust handling strategies, developers can improve the reliability and user experience of their applications.
Understanding the Exception Message
The error message “exception has been thrown by the target of invocation” typically arises in .NET applications, particularly when using reflection. It indicates that an underlying method called via reflection encountered an exception, which is then wrapped in a `TargetInvocationException`.
The key components of this message include:
- Target: This refers to the method that was invoked via reflection.
- Invocation: It indicates the action of calling a method dynamically at runtime.
- Exception: This signifies that an error occurred during the execution of the invoked method.
Common Causes
Several issues can trigger this exception, including:
- Null Reference Exceptions: Attempting to access an object or member that hasn’t been initialized.
- Invalid Cast Exceptions: Trying to cast an object to a type that it doesn’t match.
- Argument Exceptions: Providing parameters that are not valid for the method being called.
- Custom Exceptions: User-defined exceptions thrown within the invoked method.
Debugging Steps
To effectively troubleshoot this exception, consider the following steps:
- Check Inner Exception:
- Always inspect the `InnerException` property of `TargetInvocationException`. This property provides more specific information about the underlying error.
- Review Method Parameters:
- Ensure that the parameters passed to the method are valid and conform to the expected types.
- Use Debugger:
- Employ debugging tools to step through the code and identify where the exception is thrown.
- Logging:
- Implement logging to capture details about method calls and exceptions to aid in identifying recurring issues.
Example Code Snippet
Below is a simple example demonstrating how this exception might occur in a Capplication:
“`csharp
public class Example
{
public void MethodThatThrows()
{
throw new InvalidOperationException(“An error occurred.”);
}
}
// Using Reflection
try
{
var example = new Example();
var method = typeof(Example).GetMethod(“MethodThatThrows”);
method.Invoke(example, null);
}
catch (TargetInvocationException ex)
{
Console.WriteLine(“An error occurred: ” + ex.InnerException.Message);
}
“`
Handling the Exception
Proper handling of the `TargetInvocationException` is crucial for maintaining application stability. Here are strategies for handling such exceptions:
- Try-Catch Blocks: Use to catch exceptions and prevent application crashes.
- Graceful Degradation: Implement fallback mechanisms if a method fails.
- User Notifications: Inform users of errors in a user-friendly manner without exposing technical details.
Best Practices
To minimize the occurrence of this exception, adhere to the following best practices:
- Parameter Validation: Always validate parameters before invoking methods.
- Avoid Reflection: Use reflection judiciously; consider alternatives when possible.
- Error Handling: Implement robust error handling strategies throughout the application.
- Unit Testing: Conduct thorough testing of methods to catch exceptions early in the development cycle.
Understanding the Exception Has Been Thrown by the Target of Invocation
Dr. Emily Carter (Software Architect, Tech Innovations Inc.). “The error message ‘exception has been thrown by the target of invocation’ typically indicates that an underlying method invoked through reflection has encountered an issue. This can stem from various causes, such as null reference exceptions or invalid arguments, and requires careful debugging to trace the root cause.”
James Liu (Lead Developer, CodeCraft Solutions). “In my experience, this exception often arises in scenarios involving dynamic method calls. It is crucial to implement robust error handling and logging mechanisms to capture the specific exception thrown by the target method, which can greatly aid in diagnosing the problem.”
Sarah Thompson (Senior Software Engineer, DevOps Insights). “When dealing with the ‘exception has been thrown by the target of invocation’ error, developers should focus on the inner exception details. This information is vital for understanding the context of the error and can provide insights into whether it is a configuration issue or a coding error.”
Frequently Asked Questions (FAQs)
What does “exception has been thrown by the target of invocation” mean?
This error message indicates that an exception occurred during the execution of a method invoked via reflection. It signifies that the method itself caused an error, which is wrapped in a `TargetInvocationException`.
What are common causes of this exception?
Common causes include unhandled exceptions within the invoked method, invalid arguments passed to the method, or issues related to the state of the object being invoked, such as null references.
How can I troubleshoot this exception?
To troubleshoot, examine the inner exception of the `TargetInvocationException` for more specific details about the root cause. Additionally, review the method being called for potential issues, such as incorrect parameters or logic errors.
Can this exception be avoided in my code?
Yes, you can reduce the likelihood of this exception by implementing proper error handling within the invoked method. Ensure that all potential exceptions are caught and handled appropriately, and validate inputs before processing.
What is the significance of the inner exception in this context?
The inner exception provides critical information about the actual error that occurred within the invoked method, helping to identify the specific issue that led to the `TargetInvocationException`.
Is this exception specific to any programming language?
While the term “exception has been thrown by the target of invocation” is commonly associated with .NET languages, similar concepts exist in other programming environments that use reflection or method invocation, though the exact terminology may vary.
The phrase “exception has been thrown by the target of invocation” typically arises in programming contexts, particularly in languages that utilize reflection or dynamic method invocation, such as C. This error message indicates that an exception occurred during the execution of a method that was invoked indirectly, often through a delegate or reflection. Understanding the underlying causes of this error is crucial for effective debugging and ensuring robust application performance.
Common reasons for this exception include issues such as invalid method parameters, unhandled exceptions within the invoked method, or problems related to the method’s accessibility. Developers must carefully examine the stack trace and inner exceptions to identify the root cause of the problem. By doing so, they can implement appropriate error handling strategies, such as try-catch blocks, to manage exceptions gracefully and enhance the application’s resilience.
In summary, the “exception has been thrown by the target of invocation” message serves as a critical indicator for developers to investigate the execution flow of their applications. By understanding the context in which this error occurs and addressing the underlying issues, developers can improve the reliability and stability of their code. This knowledge not only aids in troubleshooting but also fosters a deeper understanding of exception handling practices in programming.
Author Profile
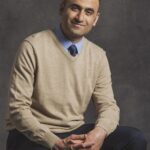
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?