How to Fix the ‘ModuleNotFoundError: No Module Named ‘_cffi_backend’?’ Issue?
In the world of Python programming, encountering errors is an inevitable part of the development process. Among the myriad of error messages that can pop up, the `ModuleNotFoundError: No module named ‘_cffi_backend’` stands out as a common yet perplexing issue for many developers. This error often leaves users scratching their heads, wondering what went wrong and how to resolve it. Whether you’re a seasoned programmer or a newcomer to the Python ecosystem, understanding this error is crucial for maintaining smooth project workflows and ensuring that your applications run seamlessly.
The `_cffi_backend` module is a critical component of the C Foreign Function Interface (CFFI) library, which allows Python code to call C code directly. When this module is missing, it can disrupt the functionality of any application that relies on it, leading to frustrating roadblocks in development. This error typically arises from installation issues, version mismatches, or environment misconfigurations, making it essential for developers to grasp the underlying causes and solutions.
In this article, we will delve into the reasons behind the `ModuleNotFoundError: No module named ‘_cffi_backend’`, exploring its implications on your projects and providing practical guidance to troubleshoot and resolve the issue. By the end, you’ll be equipped with
Understanding the Error
The `ModuleNotFoundError: No module named ‘_cffi_backend’` typically indicates that the CFFI (C Foreign Function Interface) module, which is a dependency for many other Python packages, is not installed or cannot be found by the Python interpreter. CFFI is essential for interfacing Python with C code, enabling the use of C libraries and functions directly in Python scripts.
Common causes of this error include:
- Missing Installation: The CFFI module may not be installed on your system.
- Virtual Environment Issues: If you are using a virtual environment, the module might be installed globally but not within the active virtual environment.
- Corrupted Installation: The CFFI installation may be corrupted or incomplete.
- Python Version Compatibility: Certain versions of Python may not support the installed version of CFFI.
Installing CFFI
To resolve the error, the first step is to ensure that CFFI is installed correctly. You can install it using pip, which is the package manager for Python. The command to do this is straightforward:
“`bash
pip install cffi
“`
If you are using a virtual environment, make sure it is activated before running the installation command. You can check if the installation was successful by running:
“`bash
pip show cffi
“`
This will provide you with information about the CFFI installation, including its version and location.
Troubleshooting Installation Issues
If the installation does not resolve the error, consider the following troubleshooting steps:
- Check Python Version: Ensure that you are using a compatible version of Python for CFFI.
- Reinstall CFFI: Sometimes a simple reinstall can fix issues:
“`bash
pip uninstall cffi
pip install cffi
“`
- Check Environment Variables: Ensure that your environment variables are set correctly, particularly if you have multiple Python installations.
Action | Command | Description |
---|---|---|
Install CFFI | pip install cffi |
Installs CFFI module |
Check Installation | pip show cffi |
Displays information about the installed CFFI |
Uninstall CFFI | pip uninstall cffi |
Removes CFFI module |
Using Virtual Environments
When working on Python projects, using virtual environments is highly recommended. This allows you to manage dependencies separately for each project. To create and activate a virtual environment, follow these steps:
- Create a Virtual Environment:
“`bash
python -m venv myenv
“`
- Activate the Virtual Environment:
- On Windows:
“`bash
myenv\Scripts\activate
“`
- On macOS/Linux:
“`bash
source myenv/bin/activate
“`
After activating the virtual environment, install CFFI as described earlier. This ensures that your project has access to the correct dependencies without conflicts.
Checking for Conflicts
If the error persists, it may be due to conflicts with other installed packages. Use the following command to list installed packages and identify potential conflicts:
“`bash
pip list
“`
Look for any packages that depend on CFFI or may have similar functionalities. It may be necessary to update or reinstall conflicting packages.
Common Causes of the Error
The error `ModuleNotFoundError: No module named ‘_cffi_backend’` typically arises due to several common issues:
- Missing CFFI Installation: The C Foreign Function Interface (CFFI) package is not installed in the Python environment.
- Virtual Environment Issues: If using a virtual environment, CFFI may be installed in the global Python environment but not in the virtual environment.
- Corrupted Installation: The CFFI installation could be corrupted or incomplete, leading to the absence of the `_cffi_backend` module.
- Compatibility Issues: There may be compatibility issues between CFFI and the Python version in use.
How to Resolve the Error
To resolve the `ModuleNotFoundError: No module named ‘_cffi_backend’`, consider the following steps:
- Install CFFI: Ensure that CFFI is installed in your Python environment. You can do this by running:
“`bash
pip install cffi
“`
- Activate Virtual Environment: If you are using a virtual environment, make sure it is activated before installing CFFI:
“`bash
source /path/to/venv/bin/activate On macOS/Linux
.\path\to\venv\Scripts\activate On Windows
“`
- Reinstall CFFI: If CFFI is already installed, it may be beneficial to uninstall and then reinstall it:
“`bash
pip uninstall cffi
pip install cffi
“`
- Check Python Version Compatibility: Ensure that the version of CFFI you are trying to use is compatible with your version of Python.
- Verify Installation: After installation, you can verify that CFFI is correctly installed by running:
“`python
import cffi
“`
Checking Your Environment
It is essential to ensure that your Python environment is set up correctly. Here are steps to check:
- List Installed Packages: Check if CFFI is listed among installed packages:
“`bash
pip list
“`
- Python Path Verification: Ensure that your Python interpreter is pointing to the correct environment:
“`python
import sys
print(sys.executable)
“`
- Inspect Environment Variables: Verify that your environment variables are not interfering with Python’s ability to locate CFFI.
Alternative Solutions
In some cases, alternative solutions may be necessary:
- Use Conda: If you are using Anaconda, consider installing CFFI through Conda, which can resolve dependencies more effectively:
“`bash
conda install cffi
“`
- Check for System Packages: On some systems, CFFI might depend on system-level packages. Ensure that you have the necessary build tools and libraries installed, such as `libffi-dev` for Debian-based systems:
“`bash
sudo apt-get install libffi-dev
“`
- Use a Different Python Distribution: If issues persist, consider using a different Python distribution that may have better support for CFFI.
Additional Resources
For further assistance and information, consult the following resources:
Resource | Link |
---|---|
CFFI Documentation | [CFFI Documentation](https://cffi.readthedocs.io/en/latest/) |
Python Package Index | [PyPI CFFI](https://pypi.org/project/cffi/) |
Stack Overflow Discussions | [Stack Overflow](https://stackoverflow.com/) |
Understanding the ‘ModuleNotFoundError’ in Python Development
Dr. Emily Carter (Senior Software Engineer, Python Development Institute). “The ‘ModuleNotFoundError: No module named ‘_cffi_backend” typically arises when the cffi library is not properly installed or configured in the Python environment. It is crucial to ensure that your environment is set up correctly and that all dependencies are installed, particularly when working with libraries that rely on C extensions.”
Michael Chen (Lead Python Developer, Tech Innovations Corp). “When encountering this error, developers should verify that they are using the correct version of Python and that the cffi module is compatible with their installation. Often, using a virtual environment can help isolate the dependencies and resolve such conflicts effectively.”
Sarah Patel (DevOps Specialist, Cloud Solutions Group). “In many cases, this error can be resolved by reinstalling the cffi library using pip. Running ‘pip install cffi’ in the terminal can ensure that the module is available in the current environment. Additionally, checking for any missing system-level dependencies can also be beneficial.”
Frequently Asked Questions (FAQs)
What does the error “modulenotfounderror: no module named ‘_cffi_backend'” indicate?
This error indicates that Python cannot find the CFFI (C Foreign Function Interface) backend module, which is necessary for certain libraries that rely on C extensions for functionality.
How can I resolve the “modulenotfounderror: no module named ‘_cffi_backend'” error?
To resolve this error, ensure that the CFFI module is installed. You can install it using pip with the command `pip install cffi`. If you are using a virtual environment, make sure it is activated before running the command.
What are the common reasons for encountering this error?
Common reasons include the CFFI module not being installed, issues with the Python environment or virtual environment, or conflicts with other installed packages that may interfere with CFFI.
Does this error occur on all operating systems?
This error can occur on any operating system where Python is installed, including Windows, macOS, and Linux. The underlying cause typically relates to the installation of the CFFI module rather than the operating system itself.
Are there any specific Python versions that are more prone to this error?
While this error can occur across various Python versions, it is more commonly reported in environments where CFFI is not pre-installed or where there are compatibility issues with certain Python packages.
What should I do if reinstalling CFFI does not solve the problem?
If reinstalling CFFI does not resolve the issue, consider checking for conflicting installations, ensuring that your Python environment is correctly set up, or reviewing the installation logs for any errors during the CFFI installation process. Additionally, updating pip and setuptools may help.
The error message “ModuleNotFoundError: No module named ‘_cffi_backend'” typically indicates that the CFFI (C Foreign Function Interface) module is not properly installed or is missing from the Python environment. This issue can arise for several reasons, including an incomplete installation of the CFFI library, a mismatch between the Python version and the installed packages, or the absence of necessary system dependencies required for CFFI to function correctly. Identifying the root cause is essential for resolving the error effectively.
To address this issue, users should first ensure that they have installed the CFFI module correctly. This can be achieved by running the command `pip install cffi` in the terminal or command prompt. Additionally, users should verify that they are using the correct Python environment, especially if they are managing multiple environments with tools like virtualenv or conda. Ensuring that the environment is activated before installation can prevent discrepancies that lead to the error.
Furthermore, users may need to check for any missing system dependencies that CFFI relies on, particularly on Linux systems, where development libraries for C and Python headers might be required. Installing these dependencies can often resolve the issue. In some cases, reinstalling Python or the specific environment
Author Profile
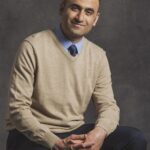
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?