Why Am I Encountering the OutOfMemoryException: What Does the ‘System.OutOfMemoryException Was Thrown’ Error Mean?
In the world of software development, encountering errors is an inevitable part of the journey. Among the myriad of exceptions that can disrupt the flow of a program, the `OutOfMemoryException` stands out as a particularly daunting challenge. This exception, which signifies that the system has run out of memory to allocate for new objects, can halt applications in their tracks, leading to frustration and downtime. Understanding the nuances of this exception is crucial for developers seeking to build robust, efficient applications that can gracefully handle memory constraints.
The `OutOfMemoryException` is not just a technical hiccup; it reflects deeper issues related to memory management, application design, and system resources. When this exception is thrown, it serves as a warning sign that the application may be mismanaging memory or that the system itself is under strain. Developers must be equipped with the knowledge to diagnose the root cause of this exception, as well as strategies to prevent it from occurring in the first place.
In this article, we will explore the intricacies of the `OutOfMemoryException`, examining its causes, implications, and best practices for mitigation. Whether you are a seasoned developer or just starting in the field, understanding this exception will empower you to create more resilient applications and enhance your problem-solving
Understanding OutOfMemoryException
An `OutOfMemoryException` is an exception of type `System.OutOfMemoryException` that occurs when the Common Language Runtime (CLR) cannot allocate enough memory for an operation. This can happen in various scenarios, particularly in applications that handle large data sets or perform extensive memory allocations. It indicates that the process has run out of memory, which can result from several factors, including:
- Large object allocations
- Memory leaks
- Inefficient memory usage patterns
- Fragmentation of the managed heap
The `OutOfMemoryException` can manifest in different environments, such as desktop applications, web applications, and server-side processes. Understanding its causes is crucial for optimizing memory usage and ensuring application stability.
Common Causes of OutOfMemoryException
Several common causes can lead to an `OutOfMemoryException`, including:
- Large Data Structures: Attempting to allocate large arrays or collections may exceed the available memory.
- Memory Leaks: Objects that are no longer needed but are still referenced can lead to increased memory consumption.
- Insufficient System Resources: Running on a system with limited physical memory or virtual memory can trigger this exception.
- Excessive Recursion: Deep recursion can consume stack space, leading to memory exhaustion.
To better understand these causes, consider the following table:
Cause | Description | Solution |
---|---|---|
Large Data Structures | Allocation of large arrays or collections exceeding memory limits. | Optimize data structures or break data into smaller chunks. |
Memory Leaks | Unreleased objects still referenced in memory. | Implement proper memory management and disposal patterns. |
Insufficient Resources | Limited physical or virtual memory on the host system. | Upgrade hardware or optimize applications to use less memory. |
Excessive Recursion | Deep recursion consuming stack space. | Refactor recursive algorithms to iterative solutions where possible. |
Diagnosing OutOfMemoryException
Diagnosing an `OutOfMemoryException` can be challenging. Developers can employ several strategies to identify the root cause:
- Profiling Tools: Use memory profiling tools to analyze memory usage patterns and identify leaks or excessive allocations.
- Performance Counters: Monitor application performance counters related to memory usage, such as the number of Gen 0, Gen 1, and Gen 2 garbage collections.
- Debugging: Implement debugging techniques to track object allocations and their lifetimes, particularly in critical areas of the code.
Utilizing these strategies can help pinpoint memory-related issues and facilitate the implementation of effective solutions.
Preventing OutOfMemoryException
To prevent `OutOfMemoryException`, developers can adopt best practices in memory management, including:
- Use Object Pooling: Reuse objects instead of frequently creating and destroying them.
- Dispose of Resources: Implement the `IDisposable` interface and ensure resources are disposed of properly.
- Limit Object Lifetimes: Minimize the lifespan of objects that consume significant memory.
- Optimize Algorithms: Review algorithms for efficiency, ensuring they do not inadvertently cause high memory usage.
By incorporating these practices into development processes, applications can achieve greater stability and reduce the likelihood of encountering `OutOfMemoryException`.
Understanding OutOfMemoryException
OutOfMemoryException is a runtime exception that occurs in .NET applications when the common language runtime (CLR) cannot allocate enough memory to continue the execution of a program. This situation typically arises due to various factors, including memory leaks, excessively large data structures, or resource-intensive operations.
Common Causes
Several factors can lead to an OutOfMemoryException:
- Memory Leaks: Unreleased resources or objects that remain in memory longer than necessary.
- Large Data Structures: Attempting to load or process large datasets entirely in memory, such as large images, files, or collections.
- Excessive Concurrent Operations: Initiating numerous threads or tasks that consume significant memory.
- Insufficient System Resources: Running applications on machines with limited RAM, especially when other applications are also consuming memory.
Handling OutOfMemoryException
To effectively manage OutOfMemoryException, consider implementing the following strategies:
- Optimize Memory Usage:
- Use data structures that are memory-efficient.
- Release resources as soon as they are no longer needed.
- Implement Paging or Streaming:
- Load only the necessary portions of data into memory.
- Stream data instead of loading it all at once.
- Increase System Resources:
- Upgrade hardware to provide more RAM.
- Optimize the operating system settings to improve memory management.
- Error Handling:
- Use try-catch blocks to gracefully handle exceptions.
- Log detailed error information for debugging purposes.
Best Practices for Prevention
Applying best practices can help prevent OutOfMemoryException from occurring:
Practice | Description |
---|---|
Use Weak References | Allows garbage collection to reclaim memory of non-essential objects. |
Analyze Memory Usage | Utilize profiling tools to monitor and analyze memory consumption. |
Dispose of Resources | Implement IDisposable interface for classes that allocate unmanaged resources. |
Limit Object Lifetimes | Ensure that objects are short-lived and not retained longer than necessary. |
Diagnosing OutOfMemoryException
When encountering an OutOfMemoryException, it is crucial to diagnose the issue accurately:
- Use Profiling Tools: Tools like dotMemory or Visual Studio’s Diagnostic Tools can help identify memory usage patterns and leaks.
- Review Application Logs: Check logs around the time of the exception for any anomalies or patterns leading to the issue.
- Memory Dump Analysis: Capture a memory dump when the exception occurs and analyze it using tools like WinDbg to identify memory consumption and object lifetimes.
While it is essential to understand the implications of OutOfMemoryException, awareness of its causes, handling mechanisms, and preventative measures can significantly reduce the incidence of this exception in .NET applications. Regular monitoring and optimization of memory usage are key practices for maintaining application stability.
Understanding OutOfMemoryException in System Applications
Dr. Emily Carter (Software Architect, Tech Innovations Inc.). “The System.OutOfMemoryException is a critical error that indicates the .NET runtime has exhausted all available memory resources. This can occur due to memory leaks, excessive memory allocation, or inefficient data handling in applications. Developers must implement robust memory management strategies to mitigate this risk.”
James Liu (Senior Developer, Cloud Solutions Group). “When encountering an OutOfMemoryException, it is essential to analyze the application’s memory usage patterns. Profiling tools can help identify memory hogs and optimize resource allocation. Additionally, considering alternative data structures or algorithms can significantly reduce memory consumption.”
Linda Martinez (Technical Consultant, Performance Engineering Experts). “The System.OutOfMemoryException can be particularly challenging in large-scale applications. It is advisable to implement paging and streaming techniques for data processing to avoid loading large datasets into memory all at once. This approach not only enhances performance but also improves application stability.”
Frequently Asked Questions (FAQs)
What is an OutOfMemoryException?
An OutOfMemoryException is an error that occurs when a program attempts to allocate more memory than is available in the system. This typically happens in environments with limited memory resources or when an application has memory leaks.
What causes an OutOfMemoryException in .NET applications?
In .NET applications, an OutOfMemoryException can be triggered by several factors, including excessive memory allocation, large object heap fragmentation, or inefficient memory usage patterns that lead to memory exhaustion.
How can I troubleshoot an OutOfMemoryException?
To troubleshoot an OutOfMemoryException, analyze memory usage patterns using profiling tools, check for memory leaks, optimize data structures, and ensure that large objects are disposed of properly. Additionally, consider increasing the available memory if feasible.
Are there any best practices to prevent OutOfMemoryExceptions?
Best practices to prevent OutOfMemoryExceptions include using memory-efficient data structures, implementing proper disposal patterns, avoiding large arrays or collections, and regularly monitoring memory usage during development and testing.
What should I do if I encounter an OutOfMemoryException in production?
If an OutOfMemoryException occurs in production, log the exception details for analysis, review memory usage patterns, and consider scaling the application or optimizing the code. It may also be necessary to restart the application to free up memory temporarily.
Can OutOfMemoryExceptions be handled in code?
Yes, OutOfMemoryExceptions can be caught using try-catch blocks in code. However, it is essential to handle them gracefully and implement fallback mechanisms, as they indicate a significant issue with memory management that needs to be addressed.
The System.OutOfMemoryException is a critical error encountered in .NET applications when the system runs out of memory resources to allocate for an operation. This exception indicates that the application has attempted to allocate memory for an object or data structure, but the system cannot fulfill the request due to insufficient available memory. It is essential for developers to understand the circumstances that lead to this exception, as it can significantly impact application performance and user experience.
Several factors can contribute to the occurrence of an OutOfMemoryException, including memory leaks, excessive memory consumption due to large data sets, or inefficient memory management practices. Developers should implement best practices such as optimizing data structures, disposing of unused objects, and utilizing memory profiling tools to monitor and manage memory usage effectively. Additionally, understanding the application’s memory requirements and scaling appropriately can help mitigate the risk of encountering this exception.
Key takeaways from the discussion on System.OutOfMemoryException include the importance of proactive memory management and the need for thorough testing under various load conditions. By anticipating potential memory issues and addressing them during the development phase, developers can create more robust applications that are less prone to crashes and performance degradation. Furthermore, adopting a systematic approach to debugging and resolving memory-related issues can lead to more efficient and reliable
Author Profile
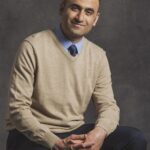
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?