How Can You Clear the Python Screen Effectively?
In the world of programming, a clean workspace is crucial for maintaining focus and efficiency. Just as a cluttered desk can hinder productivity, a messy console can make it challenging to debug or visualize your code’s output. For Python developers, knowing how to clear the screen can be a simple yet powerful tool in your coding arsenal. Whether you’re running scripts in a terminal or developing interactive applications, mastering this skill will enhance your programming experience and keep your workspace organized.
Clearing the screen in Python is not just about aesthetics; it’s about creating an environment conducive to problem-solving and creativity. When you execute multiple lines of code, the output can quickly become overwhelming, making it difficult to track the results of your latest changes. Fortunately, Python offers several methods to clear the console, each suitable for different environments, such as command line interfaces or integrated development environments (IDEs).
In this article, we will explore the various techniques available for clearing the Python screen, providing you with the knowledge to implement them effectively. From built-in functions to platform-specific commands, you’ll discover how to keep your console tidy and your focus sharp, enabling you to tackle your coding challenges with renewed clarity.
Using ANSI Escape Sequences
One of the simplest methods to clear the Python console screen is by using ANSI escape sequences. This method is effective in terminals that support these sequences, such as most Unix-based systems and modern terminal emulators. The escape sequence to clear the screen is `\033[H\033[J`, which moves the cursor to the home position and clears the screen.
Here is a straightforward implementation in Python:
“`python
import os
def clear_screen():
os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)
Call the function
clear_screen()
“`
This function checks the operating system and executes the appropriate command for clearing the screen.
Utilizing the `os` Module
The `os` module provides a platform-independent way to clear the console. As shown in the example above, the function `os.system()` can be used to send commands to the operating system. This is particularly useful because it allows for compatibility with both Windows (`cls`) and Unix-like systems (`clear`).
Employing the `subprocess` Module
Another approach to clear the screen is by using the `subprocess` module, which provides more powerful facilities for spawning new processes and retrieving their results. This method is generally preferred for more complex applications where you may need to capture output or handle errors.
Here is how you can implement it:
“`python
import subprocess
def clear_screen():
subprocess.run(‘cls’ if os.name == ‘nt’ else ‘clear’, shell=True)
Call the function
clear_screen()
“`
This method is robust and allows for better error handling, making it suitable for larger applications.
Creating a Clear Function for Interactive Sessions
In interactive Python sessions or Jupyter notebooks, you might want to clear the output without affecting the entire terminal. Here’s how you can achieve that in a Jupyter notebook:
“`python
from IPython.display import clear_output
def clear_notebook_output():
clear_output(wait=True)
Call the function
clear_notebook_output()
“`
Using `clear_output(wait=True)` allows for a smooth user experience by preventing flicker when clearing the output.
Comparison of Methods
The following table summarizes the different methods to clear the screen in Python, including their compatibility and use cases:
Method | Compatibility | Use Case |
---|---|---|
ANSI Escape Sequences | Unix-based systems | Simple scripts |
os Module | Cross-platform | General purpose |
subprocess Module | Cross-platform | Complex applications |
IPython.display | Jupyter notebooks | Interactive sessions |
By understanding these methods, you can choose the most suitable approach for your specific Python project and environment.
Methods to Clear the Python Screen
There are several approaches to clear the console screen in Python, depending on the operating system you are using. Below are the most common methods.
Using the OS Module
The `os` module provides a simple way to clear the screen across different operating systems.
“`python
import os
def clear_screen():
os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)
“`
- On Windows, the command `cls` is used.
- On Unix-based systems (Linux, macOS), the command `clear` is used.
This method ensures compatibility across platforms when clearing the console.
Using ANSI Escape Sequences
For terminals that support ANSI escape sequences, you can use the following code:
“`python
print(“\033[H\033[J”)
“`
- `\033[H` moves the cursor to the top-left corner of the screen.
- `\033[J` clears the screen from the cursor down.
This method may not work in all environments but is effective in many terminal emulators.
Creating a Function to Clear the Screen
You can encapsulate the clearing logic in a function, making it reusable throughout your code. Here’s an example function:
“`python
def clear():
import os
os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)
“`
This function can be called whenever you want to clear the screen, improving code readability and maintenance.
Considerations When Clearing the Screen
When implementing a screen clear function, consider the following:
- User Experience: Frequent clearing may disrupt user interaction. Use it judiciously.
- Debugging: Clearing the screen during debugging can make it harder to track previous outputs. Use conditionally or during final runs.
- Environment Compatibility: Ensure that the method chosen works well across different IDEs or terminal environments.
Practical Example
Here is a practical example that combines user input and screen clearing:
“`python
import os
def clear_screen():
os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)
while True:
clear_screen()
user_input = input(“Enter something (or ‘exit’ to quit): “)
if user_input.lower() == ‘exit’:
break
“`
This code continuously clears the screen after each input until the user decides to exit. It demonstrates how to maintain a clean interface for user interaction.
Using Third-Party Libraries
There are third-party libraries that also facilitate screen clearing. One such library is `colorama`, which can help manage terminal colors and styles while providing screen clearing capabilities.
“`python
from colorama import init, AnsiToWin32
init() Initializes Colorama
def clear():
AnsiToWin32().write(“\033[H\033[J”)
“`
This method enhances the screen clearing process with additional functionality for color and formatting, making it suitable for more complex applications.
Utilizing the above methods allows developers to effectively manage the console output in Python applications, enhancing user experience and clarity.
Expert Insights on Clearing the Python Screen
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “Clearing the screen in Python can be accomplished using the `os` module. By calling `os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)`, developers can effectively manage their console output, providing a cleaner interface for user interactions.”
Michael Thompson (Python Instructor, Tech Academy). “For beginners, it is crucial to understand that clearing the screen is not a built-in Python function. Utilizing the `os` module is a straightforward approach, but one should also consider using libraries like `curses` for more advanced terminal control.”
Sarah Lee (Lead Developer, OpenSource Innovations). “In a development environment, frequent screen clearing can disrupt the flow of information. I recommend implementing a function that clears the screen only when necessary, ensuring that users retain important context while navigating through outputs.”
Frequently Asked Questions (FAQs)
How can I clear the Python console screen in a terminal?
To clear the console screen in a terminal, you can use the command `os.system(‘cls’)` on Windows or `os.system(‘clear’)` on Unix/Linux systems. Ensure to import the `os` module before executing the command.
Is there a built-in function in Python to clear the screen?
Python does not have a built-in function specifically for clearing the screen. However, you can create a custom function that utilizes the `os` module to achieve this functionality.
Can I clear the screen in a Jupyter Notebook?
In a Jupyter Notebook, you can clear the output of a cell by using the command `from IPython.display import clear_output; clear_output()`. This will remove the cell output but not the entire notebook interface.
What happens if I run `os.system(‘cls’)` on a non-Windows system?
If you run `os.system(‘cls’)` on a non-Windows system, it will result in an error since the `cls` command is specific to Windows. Use `os.system(‘clear’)` for Unix/Linux systems instead.
Are there any third-party libraries that can help clear the screen?
Yes, libraries such as `colorama` can enhance terminal output and provide methods to clear the screen. However, for simple screen clearing, the `os` module is typically sufficient.
Does clearing the screen affect the program’s performance?
Clearing the screen does not significantly impact the overall performance of a Python program. It is primarily a cosmetic operation that refreshes the display for better readability.
In Python, clearing the screen can be achieved through various methods depending on the operating system being used. For Windows users, the command `os.system(‘cls’)` is typically employed, while Unix-based systems, including Linux and macOS, utilize `os.system(‘clear’)`. These commands invoke the system’s terminal commands to clear the display, providing a clean slate for the user.
Additionally, there are alternative approaches that can be implemented, such as using libraries like `curses` for more advanced terminal control. However, for most basic applications, the `os` module suffices. It is important to note that these methods may not work in all environments, such as certain integrated development environments (IDEs) or text editors, where the terminal commands may not be recognized.
In summary, clearing the Python screen is a straightforward task that can be accomplished through simple commands tailored to the operating system. Understanding these methods enhances the user experience by maintaining a tidy workspace, especially during the development and debugging phases of programming.
Author Profile
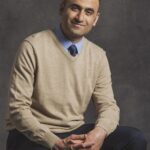
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?