How Can You Get the Current Time of Day in C?
In the world of programming, time is a fundamental concept that often goes overlooked until it becomes essential to a project’s functionality. Whether you’re developing a simple application or a complex system, knowing how to retrieve and manipulate the current time of day in C can significantly enhance your program’s capabilities. From scheduling tasks to timestamping events, understanding how to work with time can make your code more robust and user-friendly. In this article, we will explore the various methods available in C for obtaining the current time, ensuring you have the tools you need to integrate time-based features into your applications.
C provides a rich set of libraries and functions that allow developers to access and manipulate time efficiently. The standard library includes functions that can retrieve the current time, format it, and even perform calculations based on time values. Understanding these functions is crucial for tasks such as logging events, creating timers, or even developing games that rely on real-time mechanics.
As we delve deeper into the topic, we will cover the essential functions and structures that C offers for time management. From the simple `time()` function to more complex structures like `tm` and `struct timeval`, you will learn how to harness the power of time in your C programs. Whether you’re a novice programmer or an experienced developer, mastering these concepts
Using `time.h` for Time Retrieval
The C standard library provides a header file called `time.h`, which offers various functions to manipulate and retrieve time and date information. To get the current time of day, one typically uses the `time()` function alongside `localtime()` or `gmtime()` for local or UTC time, respectively.
The `time()` function retrieves the current calendar time and returns it as a `time_t` object. The `localtime()` function then converts this `time_t` value into a `struct tm`, which holds the broken-down time representation.
Here is an example of how to use these functions to get the current local time:
“`c
include
include
int main() {
time_t now;
struct tm *local;
time(&now); // Get current time
local = localtime(&now); // Convert to local time
printf(“Current local time: %s”, asctime(local));
return 0;
}
“`
This snippet captures the current time and prints it in a human-readable format.
Understanding the `struct tm` Structure
The `struct tm` structure is pivotal for representing time in C. It contains various members that represent different components of time, which can be accessed and modified as needed.
Member | Description |
---|---|
tm_sec | Seconds after the minute (0 – 60) |
tm_min | Minutes after the hour (0 – 59) |
tm_hour | Hour since midnight (0 – 23) |
tm_mday | Day of the month (1 – 31) |
tm_mon | Months since January (0 – 11) |
tm_year | Years since 1900 |
tm_wday | Days since Sunday (0 – 6) |
tm_yday | Days since January 1 (0 – 365) |
tm_isdst | Daylight saving time flag |
By accessing these members, you can retrieve specific time components and manipulate them as necessary.
Formatting Time Output
To format the output of the current time in a more customizable manner, the `strftime()` function can be employed. This function allows you to specify a format string that defines how the date and time should be represented.
Here is an example demonstrating the use of `strftime()`:
“`c
include
include
int main() {
time_t now;
struct tm *local;
char buffer[80];
time(&now);
local = localtime(&now);
strftime(buffer, sizeof(buffer), “Today is %Y-%m-%d, and the time is %H:%M:%S”, local);
printf(“%s\n”, buffer);
return 0;
}
“`
In this code, the output format is specified as “Year-Month-Day, Hour:Minute:Second”, which can be easily modified based on user requirements. The format specifiers used in the string can be adjusted to display the date and time in various formats.
Getting the Current Time of Day in C
To retrieve the current time of day in C, the `
Using time.h Functions
The following steps outline how to fetch and display the current time of day:
- Include the Necessary Header: Ensure that you include the `
` header file in your program.
“`c
include
include
“`
- Get the Current Time: Use the `time()` function to get the current time as a `time_t` object.
“`c
time_t current_time;
current_time = time(NULL); // Get current time
“`
- Convert to Local Time: The `localtime()` function converts the `time_t` object into a `struct tm` format which contains various components of the time.
“`c
struct tm *local_time;
local_time = localtime(¤t_time);
“`
- Format and Print the Time: You can format the time using `strftime()` or simply print the individual components.
“`c
printf(“Current local time: %s”, asctime(local_time));
“`
Example Code Snippet
Here is a complete example that demonstrates how to get and display the current time of day:
“`c
include
include
int main() {
time_t current_time;
struct tm *local_time;
// Get current time
current_time = time(NULL);
// Convert to local time
local_time = localtime(¤t_time);
// Print formatted time
printf(“Current local time: %s”, asctime(local_time));
return 0;
}
“`
Understanding the time_t and struct tm
- time_t: This data type is used to represent time in seconds since the Epoch (00:00:00 UTC on 1 January 1970).
- struct tm: This structure contains the following fields:
- `tm_year`: Year since 1900
- `tm_mon`: Month (0-11)
- `tm_mday`: Day of the month (1-31)
- `tm_hour`: Hour (0-23)
- `tm_min`: Minute (0-59)
- `tm_sec`: Second (0-60, including leap seconds)
Formatting Time Output
To customize the output format, `strftime()` can be used. This function allows you to define a format string.
“`c
char buffer[80];
strftime(buffer, sizeof(buffer), “%Y-%m-%d %H:%M:%S”, local_time);
printf(“Formatted time: %s\n”, buffer);
“`
Format Specifier | Description |
---|---|
`%Y` | Year with century as a decimal |
`%m` | Month as a decimal (01-12) |
`%d` | Day of the month (01-31) |
`%H` | Hour (00-23) |
`%M` | Minute (00-59) |
`%S` | Second (00-60) |
Utilizing these methods provides a robust way to handle time in C, allowing for both raw and formatted outputs as needed.
Expert Insights on Retrieving Time of Day in C Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In C, the standard library provides the `time.h` header, which is crucial for retrieving the current time of day. Utilizing the `time()` function in conjunction with `localtime()` allows developers to convert the time into a human-readable format, making it essential for applications that require accurate time tracking.”
James Liu (Lead Developer, Open Source Projects). “When working with time in C, it is important to consider the implications of time zones and daylight saving time. The `strftime()` function is invaluable for formatting the output, but developers must ensure they handle local time correctly to avoid discrepancies, especially in global applications.”
Sarah Thompson (Systems Analyst, Global Tech Solutions). “For real-time applications, precision is key. Using `gettimeofday()` can provide microsecond precision, which is beneficial for performance monitoring. However, developers should be aware of the limitations of the C standard library when it comes to high-resolution timing and consider platform-specific solutions when necessary.”
Frequently Asked Questions (FAQs)
How can I get the current time of day in C?
You can obtain the current time of day in C using the `time()` function from the `
What libraries do I need to include to work with time in C?
To work with time in C, you need to include the `
Can I get the time in a specific format in C?
Yes, you can format the time using the `strftime()` function. This function allows you to specify the desired format for the output string, such as hours, minutes, and seconds.
What is the difference between `time()` and `gettimeofday()` in C?
`time()` returns the current time as a time_t object, which represents the number of seconds since the Unix epoch. In contrast, `gettimeofday()` provides more precise time information, including microseconds, and can be used for measuring elapsed time.
Is it possible to get the time in UTC using C?
Yes, you can obtain the time in UTC by using the `gmtime()` function instead of `localtime()`. This function converts the time to Coordinated Universal Time (UTC).
How do I handle time zones in C?
In C, you can handle time zones using the `setenv()` function to set the `TZ` environment variable, which specifies the desired time zone. After setting the time zone, you should call `tzset()` to apply the changes.
In C programming, obtaining the current time of day can be accomplished using various functions and structures provided by the standard library. The most commonly used method involves the `time()` function, which retrieves the current time in seconds since the epoch (January 1, 1970). To convert this time into a more human-readable format, developers often utilize the `localtime()` function, which transforms the time into a `struct tm` format, allowing for easy access to individual components such as hours, minutes, and seconds.
Additionally, the `strftime()` function is frequently employed to format the time according to specific requirements. This function provides flexibility in displaying the time in various formats, making it a valuable tool for developers who need to present time data in a user-friendly manner. By combining these functions, programmers can effectively manage and display the time of day in their applications.
Key takeaways from this discussion include the importance of understanding the different time-related functions available in C, as well as the significance of utilizing the `struct tm` for accessing detailed time components. Mastering these functions not only enhances the functionality of C programs but also improves the overall user experience by providing accurate and well-formatted time information.
Author Profile
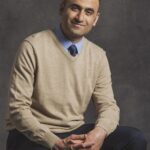
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?