How Can You Pass a Function as an Argument in JavaScript?
In the world of JavaScript, the ability to pass functions as arguments is a powerful feature that opens up a realm of possibilities for developers. This concept, often referred to as “first-class functions,” allows functions to be treated like any other variable, enabling dynamic and flexible programming patterns. Whether you’re designing complex applications or simply looking to streamline your code, understanding how to effectively pass functions as arguments can greatly enhance your coding skills and efficiency.
At its core, passing a function as an argument allows you to create more reusable and modular code. This technique is essential in scenarios such as callbacks, where a function is executed after another function completes its task. By leveraging this capability, developers can write cleaner code that separates logic and enhances readability. Moreover, it empowers you to create higher-order functions—functions that can take other functions as inputs or return them as outputs—further enriching your programming toolkit.
As you delve deeper into this topic, you’ll discover various use cases and practical examples that illustrate how passing functions as arguments can simplify complex operations and improve code maintainability. From event handling in web applications to asynchronous programming, mastering this concept is crucial for any JavaScript developer looking to elevate their coding prowess. Get ready to unlock the full potential of your JavaScript skills!
Understanding Function Arguments
In JavaScript, functions are first-class citizens, meaning they can be treated like any other variable. This allows you to pass functions as arguments to other functions, enabling higher-order programming. When you pass a function as an argument, it can be invoked within the receiving function, allowing for dynamic behavior and code reuse.
Passing a Function as an Argument
To pass a function as an argument, simply define a function and use its name without parentheses. This indicates that you are passing the reference to the function rather than executing it immediately. Here’s a basic example:
javascript
function greet(name) {
return `Hello, ${name}!`;
}
function processGreeting(func, name) {
return func(name);
}
console.log(processGreeting(greet, “Alice”)); // Outputs: Hello, Alice!
In this example, `greet` is passed as an argument to the `processGreeting` function. Inside `processGreeting`, the passed function is called with the provided name.
Using Anonymous Functions
Anonymous functions (also known as function expressions) can also be passed as arguments. This is useful when you need a quick, one-off function without the need to define it separately. Here’s an example:
javascript
function calculate(operation, a, b) {
return operation(a, b);
}
const sum = calculate(function(x, y) {
return x + y;
}, 5, 3);
console.log(sum); // Outputs: 8
In this case, an anonymous function that performs addition is passed to the `calculate` function, demonstrating the flexibility of JavaScript functions.
Using Arrow Functions
Arrow functions provide a more concise syntax for writing functions and can also be passed as arguments. They are particularly useful for inline function definitions. Here’s how you can use them:
javascript
const multiply = (x, y) => x * y;
const result = calculate(multiply, 4, 5);
console.log(result); // Outputs: 20
This example showcases the use of an arrow function to perform multiplication, passed seamlessly as an argument.
Table of Function Types for Arguments
Type | Description | Example |
---|---|---|
Named Function | A function defined with a name and can be reused. | function myFunction() { /* code */ } |
Anonymous Function | A function without a name, often used for short-lived operations. | function() { /* code */ } |
Arrow Function | A concise syntax for writing functions, especially useful for inline use. | (param1, param2) => { /* code */ } |
Practical Use Cases
Passing functions as arguments opens up a variety of practical applications:
- Callbacks: Functions that are executed after a certain task is completed, such as after an API request.
- Event Handlers: Functions that handle events, allowing for dynamic interactions.
- Functional Programming Techniques: Such as map, filter, and reduce, where functions are passed to transform data.
By understanding how to pass functions as arguments, developers can write more flexible and reusable code, taking full advantage of JavaScript’s capabilities.
Understanding Function Arguments in JavaScript
In JavaScript, functions are first-class objects, meaning they can be treated like any other value. This allows functions to be passed as arguments to other functions, enabling powerful patterns such as callbacks and higher-order functions.
Passing Functions as Arguments
To pass a function as an argument, simply define a function and then pass it to another function. Here’s a basic example:
javascript
function greet(name) {
return `Hello, ${name}!`;
}
function processUserInput(callback) {
const name = ‘Alice’;
console.log(callback(name)); // Calls the passed function
}
processUserInput(greet); // Outputs: Hello, Alice!
In this example, the `greet` function is passed to `processUserInput`, which invokes it within its own context.
Using Anonymous Functions
You can also pass anonymous functions (functions without a name) as arguments. This is often done for inline callbacks:
javascript
processUserInput(function(name) {
return `Hi there, ${name}!`;
});
This method enhances readability and reduces the need for separate function declarations when the logic is simple.
Arrow Functions as Arguments
JavaScript ES6 introduced arrow functions, which provide a more concise syntax. They can also be used as arguments:
javascript
processUserInput((name) => `Welcome, ${name}!`);
Arrow functions are particularly useful for preserving the context of `this`, making them ideal for certain use cases, especially in object-oriented programming.
Higher-Order Functions
A higher-order function is a function that takes another function as an argument or returns a function. Here’s an example:
javascript
function multiplier(factor) {
return function(x) {
return x * factor;
};
}
const double = multiplier(2);
console.log(double(5)); // Outputs: 10
In this example, `multiplier` returns a new function that multiplies its argument by a specified factor.
Use Cases
Passing functions as arguments is particularly useful in various scenarios:
- Callbacks: Functions that are executed after a certain event, such as user interaction or data fetching.
- Array Methods: Methods like `map`, `filter`, and `reduce` accept functions as arguments to transform or evaluate array elements.
- Event Handlers: Functions that handle events can be defined and passed directly to event listeners.
Example: Array Methods
Using functions as arguments can be demonstrated with array methods. Here’s how you can use a function with `map`:
javascript
const numbers = [1, 2, 3, 4];
const doubled = numbers.map((number) => number * 2);
console.log(doubled); // Outputs: [2, 4, 6, 8]
In this example, an arrow function is passed to `map`, which applies it to each element in the array.
Best Practices
When passing functions as arguments, consider the following best practices:
- Clear Naming: Use descriptive names for your functions to enhance readability.
- Avoid Side Effects: Functions should ideally not alter external states unless necessary, to maintain predictability.
- Utilize Arrow Functions When Appropriate: They can simplify code, especially in scenarios involving `this`.
By employing these techniques, you can leverage the full power of JavaScript’s first-class functions to create flexible and maintainable code.
Expert Insights on Passing Functions as Arguments in JavaScript
Dr. Emily Carter (Senior JavaScript Developer, Tech Innovations Inc.). “Passing functions as arguments in JavaScript is a powerful feature that enables developers to write more modular and reusable code. This technique, often referred to as higher-order functions, allows for greater flexibility in function execution and enhances the overall design of applications.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “Understanding how to pass functions as arguments is essential for mastering asynchronous programming in JavaScript. It facilitates callbacks and promises, which are crucial for handling operations that may take an indeterminate amount of time, such as API calls or file reading.”
Sarah Thompson (JavaScript Educator, WebDev Academy). “When teaching JavaScript, I emphasize the importance of passing functions as arguments because it introduces students to concepts like closures and functional programming. These concepts not only improve code quality but also prepare them for modern frameworks that heavily utilize these patterns.”
Frequently Asked Questions (FAQs)
What does it mean to pass a function as an argument in JavaScript?
Passing a function as an argument means providing a function to another function, allowing the receiving function to execute the passed function as part of its operation.
How do you define a function that accepts another function as an argument?
You define a function with a parameter that represents the function to be passed. For example:
javascript
function higherOrderFunction(callback) {
callback();
}
Can you pass anonymous functions as arguments in JavaScript?
Yes, anonymous functions can be passed as arguments. For instance:
javascript
higherOrderFunction(function() {
console.log(“Anonymous function executed”);
});
What are some common use cases for passing functions as arguments?
Common use cases include callbacks for asynchronous operations, event handling, and functional programming techniques like map, filter, and reduce.
How can you ensure the correct context when passing a method as an argument?
To ensure the correct context, use the `bind` method to explicitly set the `this` value, or use an arrow function that captures the surrounding context. For example:
javascript
const obj = {
method: function() {
console.log(this);
}
};
higherOrderFunction(obj.method.bind(obj));
What is a callback function in the context of passing functions as arguments?
A callback function is a function passed as an argument to another function, which is then invoked within that function. It allows for asynchronous execution and custom behavior based on the operation’s outcome.
Passing a function as an argument in JavaScript is a fundamental concept that enhances the flexibility and reusability of code. This practice allows developers to create higher-order functions, which can accept other functions as parameters. By doing so, one can implement callbacks, event handlers, and various functional programming techniques, enabling more dynamic and modular code structures.
To effectively pass a function as an argument, one simply needs to reference the function by its name without invoking it. For example, if you have a function named `myFunction`, you can pass it to another function like this: `anotherFunction(myFunction)`. This approach allows the receiving function to invoke the passed function at the appropriate time, thereby facilitating complex behaviors and interactions within the code.
Key takeaways from this discussion include the importance of understanding the context in which functions are executed, as well as the implications of scope and closures when dealing with nested functions. Additionally, utilizing functions as first-class citizens in JavaScript opens up opportunities for more expressive and concise code, ultimately leading to improved maintainability and clarity in programming practices.
Author Profile
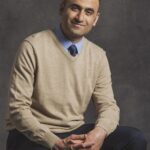
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?