How Can You Effectively Test JavaScript Code?
Introduction
In the dynamic world of web development, JavaScript reigns supreme as the backbone of interactive and engaging user experiences. However, with great power comes great responsibility, and ensuring that your JavaScript code runs smoothly and efficiently is paramount. Testing JavaScript is not just a technical necessity; it’s an art that can significantly enhance the quality and reliability of your applications. Whether you’re a seasoned developer or just starting your coding journey, understanding how to effectively test JavaScript will empower you to write cleaner, more robust code and ultimately deliver a better product.
As you dive into the realm of JavaScript testing, you’ll discover a variety of methodologies and tools designed to streamline the process. From unit tests that validate individual functions to integration tests that assess the interaction between different components, each approach serves a unique purpose in the development lifecycle. Moreover, the rise of frameworks and libraries such as Jest, Mocha, and Jasmine has transformed the way developers approach testing, making it easier than ever to implement comprehensive test suites.
In addition to enhancing code quality, testing JavaScript also fosters a culture of collaboration and confidence among development teams. By adopting a test-driven development (TDD) approach, teams can ensure that features are built with testing in mind from the outset, reducing the likelihood of
Unit Testing
Unit testing is a fundamental practice for testing JavaScript code, allowing developers to test individual components or functions for correctness. Frameworks like Jest, Mocha, and Jasmine provide robust environments for writing and executing these tests.
– **Jest**: Often used for React applications, it includes a built-in test runner and assertion library.
– **Mocha**: A flexible framework that can be paired with various assertion libraries like Chai.
– **Jasmine**: Offers a behavior-driven development (BDD) framework for testing JavaScript code.
To begin unit testing, follow these steps:
- Choose a testing framework that suits your project requirements.
- Write test cases for each function or component, ensuring each test covers specific scenarios.
- Run the tests and verify that they pass or fail as expected.
Example of a simple Jest test case:
javascript
function add(a, b) {
return a + b;
}
test(‘adds 1 + 2 to equal 3’, () => {
expect(add(1, 2)).toBe(3);
});
Integration Testing
Integration testing focuses on verifying the interaction between various modules or components of an application. It ensures that the combined parts of the system work together as intended.
When performing integration tests, consider the following:
- Identify the modules that need to interact.
- Write tests that cover the interactions between these modules.
- Use tools like Cypress or TestCafe for end-to-end testing that includes integration tests.
A comparison of popular integration testing tools is as follows:
Tool | Features | Best For |
---|---|---|
Cypress | Real-time reloading, time travel debugging | Web applications |
TestCafe | Supports multiple browsers, no browser plugins required | Cross-browser testing |
Functional Testing
Functional testing evaluates the application against its functional requirements. This type of testing ensures that the software behaves as expected when subjected to specific inputs.
To conduct functional testing, follow these steps:
- Define the functional requirements of your application.
- Create test cases that cover each requirement.
- Use automated testing tools like Selenium or Puppeteer to execute tests.
Key aspects to keep in mind:
- Ensure tests are repeatable and reliable.
- Validate both positive and negative scenarios.
Performance Testing
Performance testing assesses how the application behaves under various load conditions. It determines the application’s responsiveness, stability, and scalability.
Types of performance testing include:
- Load Testing: Measures the application’s performance under expected load.
- Stress Testing: Evaluates how the application behaves under extreme conditions.
- Endurance Testing: Tests the application’s stability over prolonged periods.
Tools such as JMeter and LoadRunner are commonly used for performance testing.
To set up performance testing:
- Define the performance criteria.
- Choose the appropriate tool.
- Simulate user loads and analyze the results.
By following these methodologies, developers can ensure a comprehensive testing strategy for their JavaScript applications, leading to higher quality code and improved user experience.
Unit Testing JavaScript
Unit testing is a crucial practice in JavaScript development that involves testing individual components or functions to ensure they work as intended. This is typically done using testing frameworks that facilitate the creation and execution of test cases.
- Popular Testing Frameworks:
- Jest: Developed by Facebook, it is widely used for its simplicity and powerful features.
- Mocha: A flexible testing framework that allows the use of various assertion libraries.
- Jasmine: An all-in-one testing framework that comes with its own assertion library.
- Basic Steps to Write Unit Tests:
- Set Up the Testing Framework: Install your chosen framework using npm or yarn.
- Create Test Files: Organize your tests in a dedicated directory.
- Write Test Cases: Define what you are testing using `describe` and `it` functions.
- Run Tests: Execute your tests through the command line or a test runner.
Integration Testing
Integration testing evaluates how different components of a JavaScript application work together. This is essential to identify issues that may not be evident in unit tests.
- Tools for Integration Testing:
- Cypress: Focuses on end-to-end testing but is also effective for integration tests.
- TestCafe: A framework for testing web applications that allows cross-browser testing.
- Considerations for Integration Tests:
- Ensure that the tests mimic real user interactions.
- Validate that data flows correctly between components.
- Use mocks and stubs where necessary to isolate components.
End-to-End Testing
End-to-end testing simulates real user scenarios and tests the application from start to finish. This type of testing is crucial for ensuring that the entire application behaves as expected.
- Common Tools for End-to-End Testing:
- Selenium: A widely used tool for automating web browsers.
- Puppeteer: A Node library that provides a high-level API to control headless Chrome.
- Workflow for End-to-End Testing:
- Define User Scenarios: Identify critical user paths that need to be tested.
- Automate Test Scripts: Write scripts that perform actions as a user would.
- Run Tests: Execute tests against a deployed version of the application.
Testing Asynchronous Code
Testing asynchronous code can be tricky due to its non-blocking nature. Proper handling of promises and callbacks is essential.
– **Methods to Test Asynchronous Code**:
- Use `async/await` syntax in your tests for clarity.
- Utilize testing frameworks that support asynchronous testing natively.
– **Example of Testing Asynchronous Functions**:
javascript
test(‘fetches data successfully’, async () => {
const data = await fetchData();
expect(data).toEqual(expectedData);
});
Code Coverage
Code coverage is a measure that describes the degree to which the source code is tested. High code coverage indicates that most of the code is exercised by tests.
- Tools for Measuring Code Coverage:
- Istanbul: A popular code coverage tool that integrates well with various testing frameworks.
- nyc: The command-line interface for Istanbul, providing coverage reports.
- Key Metrics to Consider:
- Statement Coverage: The percentage of executable statements that have been executed.
- Branch Coverage: The percentage of control flow branches that have been executed.
- Function Coverage: The percentage of functions that have been called.
Best Practices for Testing JavaScript
Adopting best practices can significantly enhance the effectiveness of your testing strategy.
- Maintainable Tests:
- Write clear and concise test descriptions.
- Group related tests using `describe` blocks.
- Automate Testing:
- Integrate tests into your CI/CD pipeline to ensure they run on every commit.
- Regularly Review Tests:
- Refactor and update tests as the application evolves to keep them relevant.
- Test Both Happy and Sad Paths:
- Ensure to cover both successful scenarios and edge cases to improve robustness.
Expert Insights on Effective JavaScript Testing
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively test JavaScript, it is crucial to utilize a combination of unit tests and integration tests. Frameworks such as Jest and Mocha provide robust environments for unit testing, while tools like Cypress can facilitate end-to-end testing to ensure that all components work together seamlessly.”
Michael Tran (Lead QA Analyst, CodeGuard Solutions). “Incorporating automated testing into your JavaScript development process is essential. Automated tests not only save time but also enhance code reliability. I recommend using tools like Selenium for browser testing and ensuring that your test cases cover edge scenarios.”
Sarah Patel (JavaScript Framework Specialist, DevMasters). “Testing JavaScript should involve a thorough understanding of the asynchronous nature of the language. Using async/await in your test cases can help manage asynchronous code effectively. Additionally, leveraging testing libraries like Testing Library can improve the quality of your tests by focusing on user interactions.”
Frequently Asked Questions (FAQs)
How can I test JavaScript in a web browser?
You can test JavaScript directly in a web browser using the Developer Tools. Access it by right-clicking on the webpage and selecting “Inspect” or pressing F12. Navigate to the “Console” tab, where you can enter and execute JavaScript code.
What are some popular JavaScript testing frameworks?
Popular JavaScript testing frameworks include Jest, Mocha, Jasmine, and QUnit. These frameworks provide tools for writing unit tests, integration tests, and end-to-end tests, ensuring your code behaves as expected.
How do I write a simple unit test in JavaScript?
To write a simple unit test, you can use a framework like Jest. First, install Jest via npm. Then, create a test file and use the `test` function to define a test case, asserting expected outcomes using `expect`.
What is the difference between unit testing and integration testing in JavaScript?
Unit testing focuses on testing individual components or functions in isolation to ensure they work correctly. Integration testing, on the other hand, evaluates how different components work together, checking for issues that may arise when they interact.
Can I test JavaScript code without a testing framework?
Yes, you can test JavaScript code without a testing framework by using simple `console.log` statements to output values and check for expected results. However, this method is less structured and may not scale well for larger projects.
What tools can I use for automated JavaScript testing?
Tools for automated JavaScript testing include Selenium for browser automation, Cypress for end-to-end testing, and Puppeteer for headless Chrome testing. These tools help automate the testing process, improving efficiency and reliability.
Testing JavaScript is an essential practice for ensuring the reliability and functionality of web applications. Various testing methodologies, such as unit testing, integration testing, and end-to-end testing, play a critical role in identifying bugs and verifying that code behaves as expected. By employing testing frameworks like Jest, Mocha, and Jasmine, developers can create automated tests that streamline the testing process and enhance code quality.
Moreover, understanding the importance of test-driven development (TDD) can significantly improve the development workflow. TDD encourages writing tests before the actual code, which helps clarify requirements and leads to cleaner, more maintainable code. Additionally, utilizing tools like Cypress for end-to-end testing allows developers to simulate user interactions, ensuring that the application functions correctly from the user’s perspective.
adopting a robust testing strategy for JavaScript applications not only mitigates risks associated with software bugs but also fosters a culture of quality and accountability within development teams. By integrating testing into the development lifecycle, teams can deliver more reliable software, ultimately enhancing user satisfaction and trust in their applications.
Author Profile
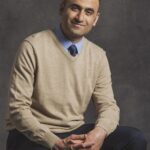
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?