Is There a Version of strcpy That Allocates Memory Automatically?
When it comes to C programming, few functions are as ubiquitous as `strcpy`, the standard library function used to copy strings from one location in memory to another. While `strcpy` is a powerful tool for string manipulation, it comes with its own set of challenges, particularly regarding memory management. Programmers often find themselves wrestling with issues of buffer overflows and memory allocation, leading to potential vulnerabilities in their applications. But what if there were a version of `strcpy` that not only copies strings but also intelligently handles memory allocation? In this article, we will explore innovative alternatives to the classic `strcpy` that prioritize safety and efficiency, offering a fresh perspective on string handling in C.
In the realm of string manipulation, memory allocation is a critical aspect that can significantly impact the performance and reliability of software. Traditional `strcpy` requires the programmer to ensure that the destination buffer is adequately sized to hold the source string, a task that can be error-prone and lead to serious security issues. This is where a memory-allocating version of `strcpy` comes into play, providing a solution that automatically manages memory, thus reducing the risk of overflow and simplifying the developer’s workload.
By leveraging dynamic memory allocation, this enhanced version of `strcpy` not
Understanding Dynamic Memory Allocation for String Copying
In C programming, the traditional `strcpy` function is used to copy strings from one location to another. However, it does not handle memory allocation, which can lead to buffer overflows or behavior if the destination buffer is not adequately sized. To address this limitation, a version of `strcpy` that allocates memory dynamically can be implemented. This approach ensures that the destination buffer is always appropriately sized based on the source string’s length.
Implementation of a Memory Allocating strcpy
The following is an example of a function that copies a string while dynamically allocating memory for the destination:
“`c
include
include
include
char* strcpy_alloc(const char* source) {
if (source == NULL) {
return NULL;
}
size_t length = strlen(source);
char* destination = (char*)malloc((length + 1) * sizeof(char)); // +1 for null terminator
if (destination == NULL) {
return NULL; // Memory allocation failed
}
strcpy(destination, source); // Copy the string
return destination; // Return the newly allocated string
}
“`
In this implementation:
- The `strcpy_alloc` function takes a source string as an argument.
- It calculates the length of the source string and allocates sufficient memory for the destination string, including space for the null terminator.
- It copies the string using `strcpy` and returns the pointer to the newly allocated string.
Benefits of Using a Memory Allocating strcpy
Using a version of `strcpy` that allocates memory offers several advantages:
- Safety: Prevents buffer overflows by ensuring that the destination buffer is appropriately sized.
- Simplicity: Reduces the complexity of managing memory in the calling code.
- Flexibility: Allows the use of strings of varying lengths without prior size determination.
Considerations When Using Dynamic Memory
While this approach provides significant benefits, it also comes with certain responsibilities:
- Memory Management: The caller must free the allocated memory once it is no longer needed to avoid memory leaks.
- Error Handling: It is crucial to check the return value of the `malloc` function to handle potential memory allocation failures gracefully.
Comparison of strcpy vs. strcpy_alloc
To further illustrate the differences, the following table compares the traditional `strcpy` with the dynamic memory-allocating version:
Feature | strcpy | strcpy_alloc |
---|---|---|
Memory Management | Requires predefined buffer | Allocates memory dynamically |
Safety | Risk of buffer overflow | Safer, prevents overflow |
Return Value | Pointer to destination buffer | Pointer to newly allocated string |
Freeing Memory | No action required | Caller must free memory |
This comparison highlights that while `strcpy` is straightforward, `strcpy_alloc` provides a more robust solution for string copying in dynamic contexts.
Memory Allocation in String Copying
When working with C programming, the standard `strcpy` function does not allocate memory for the destination string. A variation of `strcpy` that allocates memory dynamically is essential for situations where the size of the source string is not known beforehand or when managing memory manually is preferable.
Custom Implementation
To create a version of `strcpy` that allocates memory, one can utilize the standard library functions `malloc` and `strlen`. Below is an example implementation:
“`c
include
include
include
char* strcpy_alloc(const char* src) {
if (src == NULL) {
return NULL;
}
size_t length = strlen(src);
char* dest = (char*)malloc(length + 1); // Allocate memory for the string + null terminator
if (dest == NULL) {
return NULL; // Return NULL if memory allocation fails
}
strcpy(dest, src); // Copy the string
return dest; // Return the allocated string
}
“`
Usage Example
Here’s how you can use the `strcpy_alloc` function:
“`c
int main() {
const char* original = “Hello, World!”;
char* copy = strcpy_alloc(original);
if (copy != NULL) {
printf(“Original: %s\n”, original);
printf(“Copy: %s\n”, copy);
free(copy); // Free the allocated memory
} else {
printf(“Memory allocation failed.\n”);
}
return 0;
}
“`
Considerations
When implementing and using a custom version of `strcpy` that allocates memory, several factors must be considered:
- Error Handling: Always check if memory allocation fails and handle it appropriately.
- Memory Management: Ensure that every call to the custom `strcpy` function has a corresponding `free` call to prevent memory leaks.
- Null Input Handling: The function should gracefully handle cases where the input string is `NULL`.
Comparison Table
Feature | Standard `strcpy` | `strcpy_alloc` |
---|---|---|
Memory Allocation | No | Yes |
Input Validation | Minimal | Checks for `NULL` |
Memory Management | User is responsible | Automatically allocates memory |
Return Value | Destination pointer | New allocated string or `NULL` |
Performance Implications
Using a dynamic memory allocation approach like `strcpy_alloc` incurs additional overhead due to:
- Memory Allocation Time: Allocating memory at runtime can be slower than using stack-allocated buffers.
- Fragmentation: Frequent allocations can lead to memory fragmentation, impacting performance.
Choosing between standard `strcpy` and a memory-allocating version depends on the specific use case, particularly regarding memory management requirements and string size uncertainties.
Expert Insights on Memory-Allocating Alternatives to strcpy
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In scenarios where dynamic memory allocation is required, using a version of strcpy that allocates memory can prevent buffer overflows and enhance memory management. Functions like strdup in C serve this purpose effectively by allocating sufficient space for the copied string.”
Michael Thompson (Lead Developer, SecureCode Solutions). “Creating a custom version of strcpy that allocates memory is crucial for applications dealing with user-generated input. This approach not only mitigates risks associated with static buffer sizes but also provides flexibility in handling varying input lengths.”
Sarah Patel (Systems Architect, NextGen Software). “When developing software that requires string manipulation, it is essential to consider alternatives to strcpy that inherently manage memory allocation. Implementing functions like mystrdup can streamline the process and ensure that memory is allocated and freed appropriately, thus enhancing application stability.”
Frequently Asked Questions (FAQs)
What is a version of strcpy that allocates memory?
The version of `strcpy` that allocates memory is typically referred to as `strdup`. This function duplicates a string by allocating sufficient memory for the new string and copying the content from the original string into it.
How does strdup differ from strcpy?
`strdup` differs from `strcpy` in that `strdup` automatically allocates memory for the new string, while `strcpy` requires the destination buffer to be pre-allocated and of sufficient size to hold the copied string.
What are the potential issues with using strdup?
Potential issues with using `strdup` include memory leaks if the allocated memory is not properly freed after use. Additionally, `strdup` may fail if memory allocation is unsuccessful, leading to a null pointer being returned.
Is strdup a standard C function?
`strdup` is not part of the ANSI C standard but is widely available in POSIX-compliant systems and many C standard libraries. It is advisable to check for its availability in your specific environment.
Are there alternatives to strdup for memory allocation and string copying?
Yes, alternatives include using `malloc` to allocate memory followed by `strcpy` to copy the string. Another option is to use C++’s `std::string`, which manages memory automatically.
What should be done after using strdup?
After using `strdup`, the allocated memory should be freed using `free()` to prevent memory leaks. It is essential to ensure that the pointer returned by `strdup` is not used after it has been freed.
The traditional `strcpy` function in C is widely used for copying strings from one location to another. However, it does not allocate memory for the destination string, which can lead to buffer overflows and behavior if the destination buffer is not adequately sized. To address these limitations, a version of `strcpy` that allocates memory dynamically is often required. This alternative approach ensures that the destination string has enough space to accommodate the source string, thus enhancing safety and reliability in string manipulation operations.
One common implementation of this concept is to create a function that not only copies the string but also allocates the necessary memory using functions like `malloc`. This function can return a pointer to the newly allocated memory, which holds the copied string. It is essential for developers to remember to free this memory after use to prevent memory leaks. Such a function can significantly reduce the risk of errors associated with fixed-size buffers and improve the robustness of the code.
In summary, a version of `strcpy` that allocates memory dynamically is a valuable enhancement for string handling in C. By ensuring that there is sufficient space for the copied string, this approach mitigates common pitfalls associated with traditional string copying methods. Developers should adopt this practice to promote safer
Author Profile
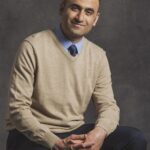
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?