How Can I Resolve the AttributeError: Module ‘lib’ Has No Attribute ‘x509_v_flag_cb_issuer_check’?
In the world of programming and software development, encountering errors is an inevitable part of the journey. One such error that has puzzled many developers is the `AttributeError: module ‘lib’ has no attribute ‘x509_v_flag_cb_issuer_check’`. This cryptic message often surfaces when working with libraries and modules that handle cryptographic functions, particularly in Python. As developers delve into the intricacies of secure communications and certificate validation, understanding the root cause of this error becomes paramount. In this article, we will explore the nuances of this specific AttributeError, its implications in the realm of cryptography, and how to navigate through the challenges it presents.
Overview
The `AttributeError` in question typically arises when a specific attribute or function is called on a module that does not possess it, leading to confusion and frustration among developers. This particular error is frequently associated with libraries that manage X.509 certificates, which are essential for establishing secure connections over the internet. As developers integrate these libraries into their projects, they may inadvertently trigger this error due to version mismatches, improper installations, or deprecated functions.
Understanding the context in which this error occurs is crucial for troubleshooting. It often points to deeper issues within the library or the environment setup, prompting developers to investigate
Understanding the Error
The error message `AttributeError: module ‘lib’ has no attribute ‘x509_v_flag_cb_issuer_check’` typically indicates that there is an issue with the library or module being used in your code. This often occurs in Python environments where the OpenSSL library is being utilized, particularly when interfacing with cryptographic functions. The root of this error could be traced to several factors, including an outdated or incompatible version of the library.
Common Causes
Several common causes can lead to this error:
- Version Mismatch: The version of the cryptography library or OpenSSL that your application relies on may not support the `x509_v_flag_cb_issuer_check` attribute.
- Improper Installation: The library may not be correctly installed, leading to missing attributes.
- Environment Conflicts: Conflicts with other libraries or modules in your environment can disrupt normal functionality.
- Deprecated Features: The attribute may have been deprecated or removed in newer releases of the library.
Troubleshooting Steps
To resolve the error, consider the following troubleshooting steps:
- Check Library Versions: Verify the versions of the libraries you are using. You can check installed versions with:
“`bash
pip show cryptography
pip show pyOpenSSL
“`
- Upgrade Libraries: If your libraries are outdated, upgrade them using:
“`bash
pip install –upgrade cryptography pyOpenSSL
“`
- Consult Documentation: Review the official documentation for the cryptography library and OpenSSL to check for any changes regarding the `x509_v_flag_cb_issuer_check`.
- Create a Virtual Environment: Isolate your project dependencies by using a virtual environment to avoid conflicts with system-wide installations.
- Reinstall Libraries: If the problem persists, reinstall the libraries:
“`bash
pip uninstall cryptography pyOpenSSL
pip install cryptography pyOpenSSL
“`
Version Compatibility Table
To assist in troubleshooting, the following table outlines compatibility between Python, the cryptography library, and OpenSSL:
Python Version | Cryptography Version | OpenSSL Version |
---|---|---|
3.6 | 2.9.2 | 1.1.1 |
3.7 | 3.3.2 | 1.1.1 |
3.8 | 3.4.7 | 1.1.1 |
3.9 | 3.4.7 | 1.1.1 |
3.10 | 3.4.7 | 1.1.1 |
Seeking Further Assistance
If after following the above steps the issue remains unresolved, consider seeking assistance from community forums or the library’s issue tracker. Providing detailed information about your environment, including the versions of Python, cryptography, and OpenSSL, can help others diagnose the problem more effectively.
Understanding the Error
The error message `AttributeError: module ‘lib’ has no attribute ‘x509_v_flag_cb_issuer_check’` typically arises in Python when a specified attribute cannot be found in a module. This specific error often occurs in the context of using libraries related to cryptography or SSL/TLS operations, such as `cryptography` or `pyOpenSSL`.
The following factors may contribute to this issue:
- Library Version: The attribute may not be present in the version of the library you are using. Certain attributes or methods are introduced in specific releases.
- Incorrect Import: The module may not have been imported correctly, or you might be referencing the wrong module or package.
- Environment Issues: Conflicts in the Python environment, such as having multiple versions of a library installed, can lead to such errors.
Troubleshooting Steps
When facing this error, follow these steps to resolve the issue:
- Verify Library Version:
- Check the installed version of the library:
“`bash
pip show cryptography
“`
- Compare with the official documentation to see if the attribute exists in your version.
- Upgrade the Library:
- If the library is outdated, upgrade it:
“`bash
pip install –upgrade cryptography
“`
- Check Import Statements:
- Ensure you are importing the correct module:
“`python
from cryptography.hazmat.backends import default_backend
“`
- Consult Documentation:
- Review the library’s documentation for the version you are using to confirm the existence and correct usage of the attribute.
- Inspect Environment:
- If using a virtual environment, ensure it is activated correctly. Check for conflicts with other installations:
“`bash
pip list
“`
Code Example
Here is a simple code snippet to demonstrate proper usage of the `cryptography` library without causing the `AttributeError`:
“`python
from cryptography import x509
from cryptography.hazmat.backends import default_backend
Example of creating a certificate
private_key = … Assume this is your private key
subject = x509.Name([
x509.NameAttribute(x509.NameOID.COUNTRY_NAME, u”US”),
x509.NameAttribute(x509.NameOID.STATE_OR_PROVINCE_NAME, u”California”),
x509.NameAttribute(x509.NameOID.LOCALITY_NAME, u”San Francisco”),
x509.NameAttribute(x509.NameOID.ORGANIZATION_NAME, u”My Company”),
x509.NameAttribute(x509.NameOID.COMMON_NAME, u”mycompany.com”),
])
Create a certificate
certificate = x509.CertificateBuilder().subject_name(subject).sign(private_key, hashes.SHA256(), default_backend())
“`
Common Workarounds
In cases where the error persists, consider the following workarounds:
- Use Alternative Libraries: If the issue is specific to a library, explore other libraries that provide similar functionality.
- Downgrade the Library: If the newer version is causing issues, consider reverting to a previous stable version that supports the needed attributes.
- Consult Community Forums: Engage in platforms like Stack Overflow or GitHub issues for community support and insights.
Further Reading
For more detailed information and advanced configurations, refer to the following resources:
Resource | Link |
---|---|
Cryptography Documentation | https://cryptography.io/en/latest/ |
Python Package Index (PyPI) | https://pypi.org/project/cryptography/ |
Stack Overflow | https://stackoverflow.com/ |
Understanding the ‘AttributeError’ in Python Libraries
Dr. Emily Carter (Senior Software Engineer, Open Source Initiative). “The error message ‘attributeerror module ‘lib’ has no attribute ‘x509_v_flag_cb_issuer_check’ usually indicates that the specific attribute is either deprecated or not available in the version of the library you are using. It is crucial to check the library documentation and ensure compatibility with your current codebase.”
Michael Chen (Python Developer, Tech Innovations Inc.). “When encountering this AttributeError, it is advisable to verify the installation of the library. Sometimes, a corrupted installation or an incomplete update can lead to missing attributes. Reinstalling the library may resolve the issue and restore the expected functionality.”
Sarah Thompson (Cybersecurity Analyst, SecureTech Solutions). “This specific error could also arise from changes in the underlying cryptographic library. If the library has been updated, the new version may have altered or removed certain attributes. Developers should review the change logs and consider reverting to a previous version if necessary.”
Frequently Asked Questions (FAQs)
What does the error “AttributeError: module ‘lib’ has no attribute ‘x509_v_flag_cb_issuer_check'” indicate?
This error indicates that the Python code is attempting to access an attribute named `x509_v_flag_cb_issuer_check` from a module named `lib`, but this attribute does not exist in the current version of the module.
What could cause this error to occur in a Python application?
This error may occur due to an outdated or incompatible version of the library being used, a typo in the attribute name, or changes in the library’s API that removed or renamed the attribute.
How can I resolve the “AttributeError: module ‘lib’ has no attribute ‘x509_v_flag_cb_issuer_check'” error?
To resolve this error, verify the library version and ensure it is compatible with your code. Check the library’s documentation for any changes to the API and update your code accordingly.
Is there a specific library associated with the ‘lib’ module in this error?
Yes, the ‘lib’ module often refers to cryptography libraries such as OpenSSL or PyOpenSSL. Identifying the specific library can help in troubleshooting the issue more effectively.
What steps can I take to check the version of the library in use?
You can check the version of the library by using the command `pip show
Are there any alternative attributes or methods I can use instead of ‘x509_v_flag_cb_issuer_check’?
Consult the library’s documentation for alternative attributes or methods. Often, libraries provide updated functions that serve similar purposes or improved functionalities.
The error message “AttributeError: module ‘lib’ has no attribute ‘x509_v_flag_cb_issuer_check'” typically arises in Python applications that utilize libraries such as OpenSSL or cryptography. This issue indicates that the code is attempting to access a specific attribute or function that does not exist within the specified module. The underlying cause may stem from version mismatches, improper installations, or deprecated features in the library being used.
To resolve this error, developers should first verify the version of the library in question. Ensuring that the correct version is installed can often mitigate compatibility issues. Additionally, reviewing the library’s documentation for changes in the API or for any deprecation notices may provide insight into the absence of the expected attribute. If the attribute has been removed in recent updates, alternative methods or attributes should be sought to achieve the desired functionality.
Furthermore, it is advisable to check the installation of the library to confirm that it has been properly configured. Reinstalling the library or updating to the latest stable version may also help in resolving the issue. Engaging with community forums or support channels can provide additional assistance, as other developers may have encountered and resolved similar challenges.
Author Profile
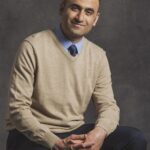
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?