How to Set Default Current Date for Created_at in Laravel Migrations?
In the world of web development, Laravel has emerged as a powerful framework that streamlines the process of building robust applications. One of its standout features is the migration system, which allows developers to define and manage their database schema with ease. Among the various functionalities offered by migrations, setting default values for date fields—particularly the `created_at` timestamp—can significantly enhance data integrity and streamline application logic. But how do you effectively set the default value of `created_at` to the current date in your migrations?
Understanding how to manipulate timestamps in Laravel migrations is crucial for any developer looking to maintain accurate records of when data is created. The `created_at` field serves as a vital marker for tracking the lifecycle of records in your application, and ensuring it captures the correct moment of creation can prevent discrepancies and improve data management. By default, Laravel automatically manages these timestamps, but there are scenarios where you might want to customize this behavior, especially when dealing with legacy databases or specific business requirements.
In this article, we will delve into the nuances of setting default date values in Laravel migrations, focusing on how to configure the `created_at` field to reflect the current date and time. Whether you’re a seasoned Laravel developer or just starting out, understanding these concepts will empower you to create more
Setting Default Values for Timestamps in Laravel Migrations
In Laravel migrations, you can set default values for timestamp columns such as `created_at` and `updated_at`. To set the default value of `created_at` to the current timestamp, you can use the `useCurrent()` method. This method allows the database to automatically set the timestamp when a new record is created.
Here’s an example of how to define a migration with a default current timestamp for the `created_at` column:
“`php
Schema::create(‘example_table’, function (Blueprint $table) {
$table->id();
$table->timestamps(); // This will create both created_at and updated_at
});
“`
If you want to explicitly set the default value for `created_at`, you can do so like this:
“`php
$table->timestamp(‘created_at’)->default(DB::raw(‘CURRENT_TIMESTAMP’));
“`
Using Laravel’s Migration Schema
Laravel provides a fluent interface for defining migrations through the Schema Builder. Here’s how to define a table with default timestamps:
“`php
Schema::create(‘users’, function (Blueprint $table) {
$table->id();
$table->string(‘name’);
$table->timestamp(‘created_at’)->default(DB::raw(‘CURRENT_TIMESTAMP’));
$table->timestamp(‘updated_at’)->default(DB::raw(‘CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP’));
});
“`
In the above example:
- The `created_at` column is set to the current timestamp when a new record is created.
- The `updated_at` column is updated to the current timestamp every time the record is updated.
Considerations for Different Database Systems
When working with different database systems, it’s essential to consider how they handle default timestamps. Below is a comparison of how MySQL and PostgreSQL handle current timestamps:
Database System | Default Value Syntax | Auto Update on Change |
---|---|---|
MySQL | DEFAULT CURRENT_TIMESTAMP | DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP |
PostgreSQL | DEFAULT now() | No automatic update on change; need triggers |
Updating Existing Tables
To modify an existing table to add a default current timestamp for `created_at`, you can use the `table` method in a migration. Here’s an example of how to achieve this:
“`php
Schema::table(‘users’, function (Blueprint $table) {
$table->timestamp(‘created_at’)->default(DB::raw(‘CURRENT_TIMESTAMP’))->change();
});
“`
This command updates the existing `created_at` column to have the default value of the current timestamp.
In summary, setting a default value for `created_at` to the current timestamp in Laravel migrations is straightforward and can be customized based on the database system in use. Always ensure that your migration files accurately reflect the desired behavior for managing timestamps.
Setting Default Values for Timestamps in Laravel Migrations
In Laravel, when creating migrations for database tables, you may want to set default values for timestamp columns such as `created_at`. By default, Laravel automatically manages the timestamps for you, but you can customize the default behavior as needed.
To set the default value of `created_at` to the current timestamp during migration, you can use the `useCurrent()` method. Here is how you can do it:
“`php
Schema::create(‘your_table_name’, function (Blueprint $table) {
$table->id();
$table->timestamps(); // This will create both created_at and updated_at columns
// or to customize the created_at column
$table->timestamp(‘created_at’)->default(DB::raw(‘CURRENT_TIMESTAMP’));
});
“`
Key Points:
– **Automatic Management**: Laravel manages timestamps automatically when you use the `$table->timestamps()` method.
- Custom Default: Use `default(DB::raw(‘CURRENT_TIMESTAMP’))` if you need a specific behavior different from the default Laravel timestamp handling.
Using Carbon for More Flexibility
If you require more complex date manipulations or need to set the default to a specific time other than the current timestamp, you can use the Carbon library, which is integrated into Laravel.
Example:
“`php
$table->timestamp(‘created_at’)->default(Carbon\Carbon::now());
“`
This approach allows you to set the default timestamp to any date you want, although it’s worth noting that this will be evaluated when the migration is run, not at the time of record creation.
Comparison of Methods:
Method | Behavior | Use Case |
---|---|---|
`$table->timestamps()` | Automatically sets `created_at` and `updated_at` to current time on create | Standard use with no customization |
`$table->timestamp()->default(DB::raw(‘CURRENT_TIMESTAMP’))` | Sets `created_at` to current time during migration | Custom behavior needed at migration time |
`$table->timestamp()->default(Carbon\Carbon::now())` | Sets default based on evaluation at migration time | When you need a specific time during migration |
Updating Timestamps
When updating records, Laravel provides functionality to manage the `updated_at` timestamp automatically if you have set the timestamps in your migration. If you need to update `created_at`, you can do so manually:
“`php
$model->created_at = now(); // Set to current time
$model->save();
“`
Important Considerations:
- Consistency: Ensure that the logic for managing timestamps is consistent throughout your application to prevent confusion or data integrity issues.
- Timezone: Be mindful of the timezone settings in your Laravel application and your database to avoid discrepancies.
By understanding these methods and considerations, you can effectively manage timestamp defaults in Laravel migrations to suit your application’s needs.
Best Practices for Setting Default Dates in Laravel Migrations
Jessica Tran (Senior Software Engineer, Tech Innovations Inc.). “When designing a database schema in Laravel, setting the default value of the `created_at` column to the current timestamp is essential for tracking record creation accurately. Using the `timestamps()` method in migrations automatically handles this, ensuring consistency across your application.”
Michael Chen (Database Architect, Cloud Solutions Group). “In Laravel migrations, utilizing the `default` method with `now()` for the `created_at` field is a straightforward approach. This practice not only simplifies the data entry process but also enhances the integrity of time-stamping in your application.”
Laura Patel (Lead Developer, Agile Software Labs). “It’s crucial to remember that while setting a default date for `created_at` in Laravel migrations can streamline operations, developers should also consider the implications for time zones. Using UTC as a standard can prevent discrepancies in multi-regional applications.”
Frequently Asked Questions (FAQs)
How do I set a default value for the created_at column in a Laravel migration?
You can set a default value for the created_at column by using the `default` method in your migration. For example:
“`php
$table->timestamp(‘created_at’)->default(DB::raw(‘CURRENT_TIMESTAMP’));
“`
Can I use the current date and time as the default value for created_at in Laravel?
Yes, you can use `DB::raw(‘CURRENT_TIMESTAMP’)` to set the current date and time as the default value for the created_at column in your migration.
What is the purpose of the created_at column in Laravel migrations?
The created_at column is used to automatically track when a record was created in the database. It is part of Laravel’s Eloquent ORM features for timestamp management.
Is it necessary to manually set the created_at value when using Laravel’s timestamps?
No, if you use Laravel’s `timestamps()` method in your migration, the created_at and updated_at fields will be automatically managed by Eloquent, eliminating the need for manual setting.
Can I change the default value of created_at after the migration has been created?
Yes, you can modify the default value of created_at by creating a new migration that alters the existing column and sets a new default value.
What happens if I don’t set a default value for created_at in my migration?
If you do not set a default value for created_at, it will be null unless you explicitly provide a value when inserting a new record, which may lead to inconsistency in your timestamp tracking.
In Laravel, migrations are a crucial aspect of database management, allowing developers to define and modify database schemas programmatically. One common requirement during migration is setting default values for timestamps, particularly for the `created_at` field. By default, Laravel uses the `CURRENT_TIMESTAMP` for the `created_at` column, ensuring that this field automatically records the time of record creation without requiring manual input.
To implement this functionality in a migration, developers can use the `timestamps()` method, which automatically creates both `created_at` and `updated_at` columns. Additionally, if there is a need to customize the default behavior, developers can specify the default value explicitly using the `default()` method in their migration scripts. This flexibility allows for tailored solutions that meet specific application requirements while adhering to best practices in database design.
In summary, leveraging Laravel’s migration capabilities to set default values for the `created_at` timestamp enhances data integrity and simplifies the development process. This approach not only saves time but also minimizes the potential for errors associated with manual date entry. Understanding and utilizing these features effectively can significantly improve the efficiency of database management in Laravel applications.
Author Profile
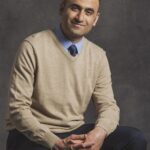
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?