How Can You Resolve Errors in Laravel’s MessageBag or ErrorBag?
In the world of web development, Laravel stands out as a robust framework that simplifies many complex tasks, particularly when it comes to handling user input and validation. However, even the most experienced developers can encounter challenges when managing errors and feedback within their applications. One common point of confusion arises from the use of the MessageBag and ErrorBag in Laravel, which can leave developers scratching their heads when trying to effectively communicate validation errors to users. Understanding these tools is crucial for creating a seamless user experience and ensuring that your application functions as intended.
Laravel’s error handling system is designed to provide developers with a straightforward way to manage validation errors. The MessageBag, a powerful component of the framework, plays a pivotal role in collecting and storing error messages, while the ErrorBag serves as a dedicated repository for these messages during the request lifecycle. As you dive deeper into Laravel’s error handling capabilities, you’ll discover how these two components interact and how they can be leveraged to enhance your application’s responsiveness and user engagement.
Navigating the nuances of Laravel’s error handling might seem daunting at first, but with a clearer understanding of MessageBag and ErrorBag, you can transform error messages from mere notifications into valuable feedback that guides users through their experience. This article will equip you with the insights needed to effectively utilize these tools
Understanding Laravel’s MessageBag
Laravel’s `MessageBag` is a powerful feature that handles error messages and validation feedback. It’s particularly useful in form validation, allowing developers to provide user-friendly error messages that enhance the user experience. The `MessageBag` is utilized internally by the framework to encapsulate validation messages and can be accessed via the `errors` variable in views.
When you perform validation in Laravel, any errors encountered will be stored in a `MessageBag` instance. You can easily retrieve these messages using various methods provided by the `MessageBag` class. Key methods include:
- `has($key)`: Checks if there are any messages associated with the given key.
- `first($key)`: Retrieves the first message associated with the specified key.
- `all()`: Returns all messages as an array.
To illustrate, here’s an example of how to retrieve and display error messages in a Blade template:
“`php
@if ($errors->any())
-
@foreach ($errors->all() as $error)
- {{ $error }}
@endforeach
@endif
“`
This snippet checks if there are any errors present and displays them in an unordered list.
Utilizing the ErrorBag
The `errorBag` is an integral part of Laravel’s validation system. It allows for grouping error messages, which can be particularly useful when dealing with multiple forms on a single page. By default, Laravel uses a single `errorBag`, but you can create custom bags for different forms.
When creating a custom error bag, you can specify it during validation like this:
“`php
$request->validate([
‘name’ => ‘required’,
’email’ => ‘required|email’,
], [], [], ‘customBag’);
“`
To access errors from a custom error bag in a Blade view, you can do the following:
“`php
@if ($errors->customBag->any())
-
@foreach ($errors->customBag->all() as $error)
- {{ $error }}
@endforeach
@endif
“`
This allows you to manage errors more effectively when dealing with multiple forms or complex validation scenarios.
Comparison of MessageBag and ErrorBag
Feature | MessageBag | ErrorBag |
---|---|---|
Default Use | General error handling | Specific to custom validation contexts |
Retrieval Method | `$errors->all()`, `$errors->first()` | `$errors->customBag->all()` |
Customization | No custom bags | Supports multiple custom bags |
Use Case | Simple forms | Complex scenarios with multiple forms |
By understanding the distinctions between `MessageBag` and `ErrorBag`, developers can better manage validation errors in Laravel applications, leading to improved code organization and user experience.
In summary, leveraging Laravel’s `MessageBag` and `ErrorBag` effectively allows for streamlined error handling that can be tailored to the needs of your application.
Error Handling in Laravel with MessageBag and ErrorBag
Laravel provides a robust mechanism for handling validation errors, primarily through the use of the `MessageBag` class. This class is essential for managing and displaying error messages to users effectively.
Understanding MessageBag
The `MessageBag` class is a container for error messages, often utilized when form validation fails. It allows developers to retrieve error messages easily and format them as needed.
**Key Features of MessageBag:**
– **Storage**: It stores multiple error messages associated with different input fields.
– **Retrieval**: You can retrieve messages for specific fields or all messages at once.
– **Manipulation**: It allows for adding, merging, and filtering messages.
**Example Usage:**
“`php
use Illuminate\Support\MessageBag;
// Creating a new MessageBag instance
$errors = new MessageBag();
// Adding errors
$errors->add(’email’, ‘The email field is required.’);
$errors->add(‘password’, ‘The password must be at least 8 characters.’);
// Retrieving all messages
$allErrors = $errors->all();
// Retrieving messages for a specific field
$emailErrors = $errors->get(’email’);
“`
Using ErrorBag in Views
When handling validation errors in views, Laravel provides a convenient way to access the `MessageBag` instance through the `$errors` variable, which is automatically shared with all views when validation fails.
**Displaying Errors in Blade Templates:**
“`blade
@if ($errors->any())
-
@foreach ($errors->all() as $error)
- {{ $error }}
@endforeach
@endif
“`
This code snippet checks if there are any errors and displays them in a list format, enhancing user experience by providing clear feedback.
Common Methods of MessageBag
The `MessageBag` class offers several methods that facilitate error management. Below is a table summarizing some commonly used methods:
Method | Description |
---|---|
`add($key, $message)` | Adds a new error message under the specified key. |
`has($key)` | Checks if there are messages for the specified key. |
`get($key)` | Retrieves messages for a specific key. |
`all()` | Returns all messages in the bag. |
`first($key)` | Retrieves the first message for the specified key. |
`merge($messages)` | Merges a new array of messages with the existing ones. |
Best Practices for Error Handling
To ensure effective error handling in Laravel applications, consider the following best practices:
- Validation Rules: Always define clear validation rules in your controllers to minimize user input errors.
- Custom Messages: Provide custom error messages to make them more user-friendly.
- Flash Messages: Use session flash messages for one-time notifications after form submissions.
- Client-side Validation: Implement client-side validation to enhance user experience and reduce server load.
By adhering to these practices, you can create a more robust and user-friendly validation process in your Laravel applications.
Understanding Laravel’s MessageBag and ErrorBag
Julia Thompson (Senior Laravel Developer, CodeCraft Solutions). “In Laravel, the MessageBag and ErrorBag are essential components for handling validation errors. The MessageBag is primarily used for storing error messages, while the ErrorBag is a more specialized instance that focuses on validation errors. Understanding the distinction between these two can greatly enhance error handling in your applications.”
Michael Chen (Lead Software Engineer, DevOps Innovations). “When you encounter errors in Laravel, utilizing the MessageBag allows for a more streamlined approach to displaying error messages to users. It’s crucial to properly manage these messages to ensure a smooth user experience. Additionally, leveraging the ErrorBag can help in debugging by providing detailed insights into validation failures.”
Sarah Patel (Laravel Consultant, Web Solutions Group). “Many developers confuse the MessageBag with the ErrorBag, but they serve different purposes. The MessageBag is versatile and can be used for various messages, while the ErrorBag is specifically tailored for validation errors. Properly implementing these can lead to clearer, more effective error handling in Laravel applications.”
Frequently Asked Questions (FAQs)
What is the difference between MessageBag and ErrorBag in Laravel?
MessageBag is a general-purpose bag for storing messages, while ErrorBag specifically holds validation error messages. Both are used to manage messages in Laravel, but ErrorBag is tailored for handling errors from form validation.
How can I retrieve error messages from the ErrorBag in Laravel?
You can retrieve error messages by using the `errors()` helper function in your views. For example, `@if ($errors->any())` checks if there are any errors, and `$errors->first(‘field_name’)` retrieves the first error message for a specific field.
Can I customize the error messages in Laravel’s ErrorBag?
Yes, you can customize error messages in Laravel by defining them in the `validation.php` language file or by passing a custom message array when validating data in your controllers.
How do I display error messages in a Blade template?
In a Blade template, you can display error messages by using the `@error` directive. For example, `@error(‘field_name’)
@enderror` will show the error message for the specified field.
What happens if there are no errors in the ErrorBag?
If there are no errors in the ErrorBag, the `any()` method will return , and any associated error display logic in your views will be skipped, ensuring a clean user interface.
Can I store non-error messages in the ErrorBag?
While the ErrorBag is primarily designed for validation errors, you can technically store non-error messages in it. However, it is recommended to use the MessageBag for general messages to maintain clarity in your code.
In Laravel, error handling is a crucial aspect of building robust applications. The framework provides a convenient way to manage validation errors through the use of the MessageBag class, which is often referred to in conjunction with error bags. When a validation error occurs, Laravel automatically populates the error bag, allowing developers to easily retrieve and display error messages to users. Understanding how to effectively utilize these features can significantly enhance user experience by providing clear feedback on form submissions.
One of the key points to consider is the distinction between the MessageBag and the error bag. The MessageBag is a collection of error messages that can be accessed using various methods, such as `errors()->first()` or `errors()->all()`. This allows developers to customize how errors are displayed to users. Furthermore, Laravel’s built-in validation mechanisms seamlessly integrate with the error bag, ensuring that any validation failures are captured and made available for display in views.
Another important takeaway is the flexibility that Laravel offers in managing error messages. Developers can define custom messages for validation rules, which can be set in the form request or directly in the controller. This customization allows for a more user-friendly approach, as developers can tailor messages to be more descriptive and relevant to the context of the form
Author Profile
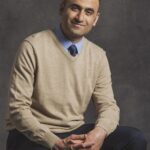
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?