Why Am I Getting ‘AttributeError: Module ‘lib’ Has No Attribute ‘x509_v_flag_cb_issuer_check’?
In the intricate world of programming, errors are an inevitable part of the journey. Among the myriad of issues developers encounter, the `AttributeError` stands out as a particularly perplexing foe. One such error, `attributeerror: module ‘lib’ has no attribute ‘x509_v_flag_cb_issuer_check’`, can leave even seasoned programmers scratching their heads. This specific error often arises in the context of cryptographic libraries, where the delicate interplay of modules and attributes can lead to unexpected roadblocks. Understanding the roots of this error not only aids in troubleshooting but also enriches one’s grasp of the underlying library mechanics.
As we delve into the intricacies of this error, it’s essential to recognize that it often signals a deeper issue related to the library’s version compatibility or improper usage. The `lib` module, commonly associated with cryptographic operations, is a cornerstone in many applications, and any disruption in its functionality can have cascading effects. This article will unravel the layers of this error, exploring its common causes and the best practices for resolution.
Navigating the labyrinth of programming errors can be daunting, but with the right insights, developers can transform these challenges into opportunities for learning and growth. By examining the context in which the `x509_v_flag_cb
Understanding the Error
The error message `attributeerror: module ‘lib’ has no attribute ‘x509_v_flag_cb_issuer_check’` typically indicates a problem with the OpenSSL library or a related Python package, such as cryptography or PyOpenSSL. This situation usually arises when the library versions are incompatible, or the environment is misconfigured.
Key components to consider include:
- Library Version Mismatch: The installed version of the library may not have the expected attributes or functions. This can often occur when a newer or older version is being used that does not support certain features.
- Importing Issues: Sometimes, the way libraries are imported or the order of imports can lead to attribute errors, especially if there are circular dependencies or if a module is partially loaded.
Troubleshooting Steps
To address the error, several steps can be taken:
- Check Installed Library Versions: Verify that you have the correct versions of the libraries required for your application. This can be done using pip:
“`bash
pip show cryptography
pip show pyOpenSSL
“`
- Upgrade/Downgrade Libraries: Based on your project’s requirements, you may need to upgrade or downgrade the libraries. Use the following commands:
“`bash
pip install –upgrade cryptography
pip install –upgrade pyOpenSSL
“`
- Verify Python Environment: Ensure that the Python environment is correctly set up and that there are no conflicts between packages. Utilizing virtual environments can help in managing dependencies effectively.
- Inspect Codebase for Import Errors: Review your code for any import statements that may be causing conflicts or errors. Ensure that the libraries are being imported correctly and in the right order.
- Check Documentation: Refer to the official documentation for the libraries in use to confirm that the functions or attributes you are trying to use are available in the version you have installed.
Example of Resolving the Issue
Suppose you are working on a project that requires specific functionalities from the cryptography library. Here’s how you can check and align your library versions:
“`bash
Check current versions
pip show cryptography
pip show pyOpenSSL
Upgrade to a compatible version
pip install cryptography==3.4.7
pip install pyOpenSSL==20.0.1
“`
Library Compatibility Table
Library | Version | Compatibility Notes |
---|---|---|
cryptography | 3.4.7 | Recommended for many applications; check for breaking changes. |
pyOpenSSL | 20.0.1 | Compatible with cryptography 3.4.x versions. |
OpenSSL | 1.1.1 | Ensure this version supports the required features. |
By following these steps and consulting the compatibility table, you can effectively resolve the `attributeerror` and ensure that your Python environment is set up correctly for your project requirements.
Understanding the Error
The error message `attributeerror: module ‘lib’ has no attribute ‘x509_v_flag_cb_issuer_check’` typically arises in Python applications that utilize libraries such as `cryptography` or `pyOpenSSL`. This error indicates that the code is attempting to access a function or attribute that is either not present in the module or not correctly imported.
Common causes of this error include:
- Version Compatibility Issues: The library in use may be outdated or incompatible with the version of Python being utilized.
- Incorrect Installation: The library may not have been installed properly, leading to missing attributes or functions.
- Changes in Library Structure: New versions of libraries often restructure their modules, which can lead to deprecated or removed attributes.
Troubleshooting Steps
To resolve the `AttributeError`, follow these troubleshooting steps:
- Check Installed Versions:
Use the following command to verify the installed versions of your libraries:
“`bash
pip show cryptography pyOpenSSL
“`
- Upgrade Libraries:
Ensure you have the latest versions by running:
“`bash
pip install –upgrade cryptography pyOpenSSL
“`
- Review Documentation:
Check the official documentation for `cryptography` or `pyOpenSSL` to confirm the availability of the `x509_v_flag_cb_issuer_check` attribute in your version.
- Inspect Code for Typos:
Confirm that there are no typographical errors in the code where the attribute is being accessed.
- Examine Import Statements:
Ensure that you are importing the module correctly. For example:
“`python
from cryptography.hazmat.backends import default_backend
“`
Code Example for Context
Here’s a simplified example demonstrating the correct usage of X.509 flags in a context where the error may arise:
“`python
from cryptography import x509
from cryptography.hazmat.backends import default_backend
Create a certificate and check flags
try:
cert = x509.load_pem_x509_certificate(pem_data, default_backend())
Assuming the correct attribute is x509.VALIDATE_FLAG
flag = x509.VALIDATE_FLAG
except AttributeError as e:
print(f”Error: {e}”)
“`
Ensure that you adapt this example based on the specific needs of your application.
Alternative Solutions
If the error persists after the aforementioned troubleshooting steps, consider the following alternative solutions:
- Reinstall Libraries: Uninstall and reinstall the libraries to ensure a clean installation:
“`bash
pip uninstall cryptography pyOpenSSL
pip install cryptography pyOpenSSL
“`
- Virtual Environment: Utilize a virtual environment to isolate dependencies and mitigate version conflicts:
“`bash
python -m venv myenv
source myenv/bin/activate On Windows use `myenv\Scripts\activate`
pip install cryptography pyOpenSSL
“`
- Seek Community Support: Engage with community forums or platforms like Stack Overflow for additional insights and solutions from other developers experiencing similar issues.
Final Verification
After implementing these steps, rerun your application to verify if the error has been resolved. Monitor for any additional errors that may arise, as they may provide further insight into underlying issues.
Understanding the ‘AttributeError’ in Cryptographic Libraries
Dr. Emily Chen (Senior Software Engineer, OpenSSL Foundation). “The error ‘attributeerror: module ‘lib’ has no attribute ‘x509_v_flag_cb_issuer_check’?’ typically arises when there is a mismatch between the library version and the code attempting to access specific attributes. Ensuring that your environment is using the correct version of the cryptographic library is crucial for resolving this issue.”
Michael Thompson (Lead Developer, SecureTech Solutions). “This error often indicates that the required function or attribute is either deprecated or not included in the current library build. Developers should consult the library’s documentation to confirm the availability of the attribute and consider upgrading or reinstalling the library if necessary.”
Laura Patel (Cybersecurity Analyst, TechGuard Inc.). “In many cases, encountering the ‘attributeerror’ signifies an underlying compatibility issue between different components of a software stack. It is advisable to check for updates in both the library and any dependent modules, as well as to review the installation procedures to ensure all components are correctly configured.”
Frequently Asked Questions (FAQs)
What does the error ‘attributeerror: module ‘lib’ has no attribute ‘x509_v_flag_cb_issuer_check’ mean?
This error indicates that the Python module ‘lib’ does not contain the specified attribute ‘x509_v_flag_cb_issuer_check’. This typically arises from version mismatches or missing components in the library being used, often related to cryptographic libraries like OpenSSL.
What could cause this error in a Python application?
The error may occur due to an outdated or incompatible version of a library, such as cryptography or OpenSSL. It can also result from improper installation or configuration of these libraries within the Python environment.
How can I resolve the ‘attributeerror: module ‘lib’ has no attribute ‘x509_v_flag_cb_issuer_check’ error?
To resolve the error, ensure that you have the latest version of the cryptography library installed. You can upgrade it using pip with the command `pip install –upgrade cryptography`. Additionally, verify that your OpenSSL installation is up to date.
Is this error specific to certain versions of Python?
While the error can occur in any version of Python, it is more commonly seen in environments where the cryptography library is not properly aligned with the version of OpenSSL installed. Compatibility issues can arise particularly in older Python versions.
What should I check if the error persists after updating libraries?
If the error persists, check for conflicting installations of the libraries. Ensure that no other versions of OpenSSL are interfering. Additionally, consider creating a new virtual environment to isolate dependencies and avoid conflicts.
Can this error affect the functionality of my application?
Yes, this error can hinder your application’s ability to perform cryptographic operations, such as SSL/TLS connections. It is crucial to resolve the issue to maintain the security and functionality of your application.
The error message “attributeerror: module ‘lib’ has no attribute ‘x509_v_flag_cb_issuer_check'” typically indicates that there is an issue with the Python environment or the specific library being utilized. This error often arises when the code attempts to access a function or attribute that does not exist in the version of the library currently installed. It is crucial to ensure that the appropriate version of the library is being used, as certain attributes may be introduced or deprecated in different releases.
One of the primary causes of this error is the mismatch between the code and the library’s version. Developers should verify the compatibility of their code with the installed library version. This can be done by checking the library’s documentation or release notes for any changes related to the ‘x509_v_flag_cb_issuer_check’ attribute. Additionally, updating the library to the latest version or reverting to a previous version that is known to work may resolve the issue.
Another important aspect to consider is the potential for conflicts between different libraries or modules within the Python environment. It is advisable to create isolated environments using tools like virtualenv or conda to manage dependencies effectively. This practice can help prevent issues arising from conflicting library versions and ensure that the correct attributes are accessible during
Author Profile
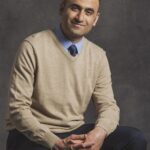
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?