How Can You Use COUNT with CASE in SQL for Conditional Counting?
In the world of SQL, counting records is a fundamental operation that can yield powerful insights into your data. However, when it comes to counting with conditions, the task can become a bit more intricate. Enter the `COUNT` function combined with the `CASE` statement—a dynamic duo that allows you to perform conditional counting with finesse. Whether you’re analyzing sales data, tracking user engagement, or compiling survey results, mastering this technique can elevate your data manipulation skills and provide a clearer picture of your datasets.
The `COUNT` function is straightforward; it simply tallies the number of rows that meet a certain criterion. However, when you introduce the `CASE` statement, you unlock the ability to apply different conditions within your counting logic. This means you can categorize and count data based on specific attributes or statuses, leading to more nuanced analyses. For instance, you might want to count how many sales were above a certain threshold or how many users fall into different age brackets—all within a single query.
By harnessing the power of `COUNT` with `CASE`, you can transform your SQL queries into robust analytical tools. This approach not only enhances your ability to derive insights but also streamlines your reporting processes. As we delve deeper into this topic, you’ll discover practical examples and tips
Understanding COUNT with CASE
Using the `COUNT` function in conjunction with the `CASE` statement in SQL allows for conditional counting of rows based on specified criteria. This technique is particularly useful when you want to categorize or filter data while performing aggregate calculations.
The `CASE` statement acts like an if-then-else logic, enabling you to define specific conditions under which different counts will be computed. This can provide insights into various segments of your dataset without the need for multiple queries.
Syntax Overview
The general syntax for using `COUNT` with `CASE` is as follows:
“`sql
SELECT
COUNT(CASE WHEN condition1 THEN 1 END) AS Count_Condition1,
COUNT(CASE WHEN condition2 THEN 1 END) AS Count_Condition2
FROM
table_name;
“`
This structure allows for the counting of rows that meet the specified conditions, and the results can be aliased for better readability.
Example Scenario
Consider a simple table named `employees` with the following structure:
employee_id | name | department | salary |
---|---|---|---|
1 | Alice | HR | 60000 |
2 | Bob | IT | 70000 |
3 | Charlie | HR | 50000 |
4 | David | IT | 80000 |
5 | Eve | Marketing | 75000 |
To count the number of employees in each department who earn more than $65,000, you can use the following SQL query:
“`sql
SELECT
department,
COUNT(CASE WHEN salary > 65000 THEN 1 END) AS Count_Above_65000
FROM
employees
GROUP BY
department;
“`
This query groups the results by department and counts how many employees in each department have a salary exceeding $65,000.
Interpreting the Results
The output of the above query will yield:
department | Count_Above_65000 |
---|---|
HR | 0 |
IT | 2 |
Marketing | 1 |
This table illustrates that within the HR department, there are no employees earning more than $65,000, while the IT department has two employees meeting this criterion.
Multiple Conditions
It is also possible to count based on multiple conditions by incorporating additional `CASE` statements. For example, if you want to count employees who earn above and below $65,000, the SQL query can be modified as follows:
“`sql
SELECT
department,
COUNT(CASE WHEN salary > 65000 THEN 1 END) AS Count_Above_65000,
COUNT(CASE WHEN salary <= 65000 THEN 1 END) AS Count_Below_65000
FROM
employees
GROUP BY
department;
```
The resulting table would display counts for both categories, offering a more comprehensive view of salary distribution across departments.
Utilizing `COUNT` with `CASE` not only enhances query efficiency but also enriches the analytical capabilities of SQL, allowing for targeted insights into the underlying data.
Using COUNT with CASE in SQL
The `COUNT` function in SQL can be effectively combined with the `CASE` statement to produce conditional counts. This allows you to count rows based on specific criteria, enabling more nuanced data analysis.
Basic Syntax
The basic syntax for using `COUNT` with `CASE` is as follows:
“`sql
SELECT
COUNT(CASE WHEN condition THEN 1 END) AS alias_name
FROM
table_name
WHERE
additional_conditions;
“`
This structure allows you to specify a condition within the `CASE` statement that determines which rows are included in the count.
Examples of COUNT with CASE
Here are some practical examples that demonstrate how to use `COUNT` with `CASE`.
Example 1: Counting Sales by Product Category
Suppose you have a sales table named `sales_data` with a column `category`. You want to count the number of sales for each product category.
“`sql
SELECT
category,
COUNT(CASE WHEN category = ‘Electronics’ THEN 1 END) AS Electronics_Count,
COUNT(CASE WHEN category = ‘Clothing’ THEN 1 END) AS Clothing_Count
FROM
sales_data
GROUP BY
category;
“`
This query counts the number of sales in each category, producing a result set that includes counts for ‘Electronics’ and ‘Clothing’.
Example 2: Conditional Count with Multiple Criteria
To count orders based on their status, consider a table called `orders` with a column `status`. You want to count the number of completed and pending orders.
“`sql
SELECT
COUNT(CASE WHEN status = ‘Completed’ THEN 1 END) AS Completed_Orders,
COUNT(CASE WHEN status = ‘Pending’ THEN 1 END) AS Pending_Orders
FROM
orders;
“`
This query provides a count of completed and pending orders in a single result set.
Handling NULL Values
When using `CASE` within the `COUNT` function, it is important to note that `NULL` values are ignored. If you want to include counts for a specific condition while also considering `NULL` values, you can modify the `CASE` statement accordingly.
“`sql
SELECT
COUNT(CASE WHEN status IS NULL THEN 1 END) AS Null_Status_Count,
COUNT(CASE WHEN status IS NOT NULL THEN 1 END) AS Non_Null_Status_Count
FROM
orders;
“`
This query counts both `NULL` and non-`NULL` statuses in the `orders` table.
Combining with GROUP BY
Using `COUNT` with `CASE` becomes particularly powerful when combined with `GROUP BY`. This allows for segmented counts across multiple categories or groups.
“`sql
SELECT
region,
COUNT(CASE WHEN status = ‘Completed’ THEN 1 END) AS Completed_Count,
COUNT(CASE WHEN status = ‘Pending’ THEN 1 END) AS Pending_Count
FROM
orders
GROUP BY
region;
“`
This statement counts completed and pending orders grouped by region, providing insights into the performance of different regions.
Summary of Benefits
Utilizing `COUNT` with `CASE` offers several advantages:
- Conditional Logic: Enables counting based on specific criteria.
- Flexibility: Can handle multiple conditions within a single query.
- Data Segmentation: Facilitates analysis across different categories or groups.
- Improved Clarity: Makes complex queries easier to understand and maintain.
This approach is a powerful tool for database querying, allowing users to derive meaningful insights from their data efficiently.
Understanding the Use of COUNT with CASE in SQL
Maria Chen (Senior Database Analyst, Data Insights Corp). “Using COUNT with CASE in SQL allows for conditional counting based on specific criteria. This technique is invaluable for generating reports that require segmented data analysis, enabling businesses to derive actionable insights from their datasets.”
James Patel (SQL Developer, Tech Solutions Inc). “Incorporating CASE statements within a COUNT function can greatly enhance the flexibility of your queries. It allows developers to aggregate data based on various conditions, facilitating more nuanced data interpretation and reporting capabilities.”
Linda Gomez (Data Scientist, Analytics Group). “The COUNT function combined with CASE is a powerful tool for data analysis. It provides a means to categorize and quantify data dynamically, which is essential for making informed decisions based on specific conditions within the dataset.”
Frequently Asked Questions (FAQs)
What is the purpose of using COUNT with CASE in SQL?
COUNT with CASE is used to conditionally count rows based on specific criteria. This allows for more granular analysis of data within a single query.
How do you structure a COUNT with CASE statement in SQL?
The structure typically involves using the COUNT function alongside a CASE statement, like this: `COUNT(CASE WHEN condition THEN 1 END)`. This counts only the rows that meet the specified condition.
Can you provide an example of COUNT with CASE in SQL?
Certainly. For instance: `SELECT COUNT(CASE WHEN status = ‘active’ THEN 1 END) AS active_count FROM users;` This counts the number of users with an ‘active’ status.
Is it possible to use multiple COUNT with CASE statements in a single query?
Yes, multiple COUNT with CASE statements can be used in a single query to count different conditions simultaneously, such as:
`SELECT COUNT(CASE WHEN status = ‘active’ THEN 1 END) AS active_count, COUNT(CASE WHEN status = ‘inactive’ THEN 1 END) AS inactive_count FROM users;`
What are common use cases for COUNT with CASE in SQL?
Common use cases include generating reports that require conditional counts, such as counting the number of orders by status, or categorizing survey responses based on specific criteria.
Are there performance considerations when using COUNT with CASE in SQL?
Yes, performance can be affected by the complexity of the CASE logic and the size of the dataset. Optimizing indexes and ensuring efficient query design can help mitigate potential performance issues.
In SQL, counting with case sensitivity can be achieved through various methods, particularly using the `COUNT()` function in conjunction with conditional statements such as `CASE`. This allows for the aggregation of data based on specific criteria, distinguishing between different cases of text values. The use of `CASE` within the `COUNT()` function enables developers to create tailored queries that can differentiate between, for example, uppercase and lowercase values in a dataset.
One of the primary approaches involves utilizing a `CASE` statement inside the `COUNT()` function. By specifying conditions within the `CASE` statement, users can count occurrences of specific values based on their case. For instance, a query can be structured to count how many times ‘Value’ appears versus ‘value’, effectively providing insights into the distribution of data with respect to case sensitivity.
Additionally, understanding the collation settings of the database is crucial, as these settings determine how string comparisons are made, including case sensitivity. By selecting the appropriate collation, users can ensure that their counting operations reflect the intended case sensitivity. This knowledge is particularly valuable when dealing with databases where case distinctions are significant, such as in programming languages or specific business rules.
In summary, counting with case in SQL is
Author Profile
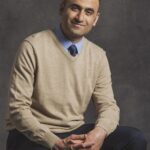
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?