How Can I Use VBA to Unfilter Data Only If It’s Currently Filtered?
In the world of Excel, data management is both an art and a science, and mastering the intricacies of VBA (Visual Basic for Applications) can elevate your spreadsheet skills to new heights. One common scenario that many users encounter is the need to manipulate filtered data effectively. Whether you’re analyzing sales figures, tracking inventory, or compiling reports, knowing how to check if a dataset is filtered and subsequently unfilter it can streamline your workflow and enhance your productivity. In this article, we will delve into the nuances of using VBA to determine if a worksheet is filtered and how to efficiently unfilter it when necessary.
As you navigate through the complexities of Excel, understanding the functionality of filters is crucial. Filters allow you to display only the data that meets certain criteria, making it easier to analyze specific subsets of information. However, there are times when you may need to revert to the original dataset, and this is where VBA comes into play. By leveraging VBA’s capabilities, you can automate the process of checking for filters and unfiltering data, saving you time and reducing the potential for errors in manual operations.
Moreover, mastering this VBA technique not only enhances your data manipulation skills but also empowers you to create more dynamic and responsive Excel applications. Whether you’re a beginner looking to expand
Understanding VBA Filtering
VBA (Visual Basic for Applications) allows users to automate tasks in Excel, including filtering data in a spreadsheet. Filtering enables users to display only the rows that meet specific criteria while hiding others. However, there may be situations where you want to check if a filter is applied and, if so, remove it.
Checking for Active Filters
To determine if a filter is currently applied to a worksheet, you can use the `AutoFilterMode` property of the worksheet object. This property returns `True` if filters are applied and “ otherwise. The syntax is as follows:
“`vba
If ActiveSheet.AutoFilterMode Then
‘ Code to execute if the filter is active
End If
“`
This simple check allows you to incorporate conditions into your VBA scripts effectively.
Unfiltering Data
If you find that a filter is active and you wish to remove it, you can use the `ShowAllData` method. This method will display all the rows in the range that was previously filtered. Here is how you can implement this in your VBA code:
“`vba
If ActiveSheet.AutoFilterMode Then
ActiveSheet.ShowAllData
End If
“`
This code snippet ensures that before attempting to show all data, it first checks if any filters are applied, preventing runtime errors.
Example of VBA Code to Unfilter
The following example illustrates how to check for an active filter and remove it. This code can be placed in any VBA module:
“`vba
Sub UnfilterIfActive()
If ActiveSheet.AutoFilterMode Then
ActiveSheet.ShowAllData
Else
MsgBox “No filters are currently applied.”
End If
End Sub
“`
This subroutine will notify the user if no filters are applied, enhancing user experience by providing feedback.
Considerations When Unfiltering
When unfiltering data, it is important to be aware of the following:
- Data Integrity: Ensure that unfiltering does not disrupt any ongoing data analysis.
- User Awareness: Consider informing users that data has been unfiltered, especially in shared environments.
- Performance: Unfiltering large datasets may take time, so be cautious when running this on extensive data ranges.
Property | Description |
---|---|
AutoFilterMode | Returns True if any filters are applied to the worksheet. |
ShowAllData | Removes all filters and shows all data in the range. |
By understanding these concepts and implementing the provided code snippets, you can efficiently manage filters in your Excel workbooks using VBA.
Using VBA to Check if Filtered and Unfilter
When working with Excel, it is often necessary to determine if a worksheet is currently filtered. If it is, you might want to unfilter the data programmatically. Below is a guide on how to achieve this using VBA.
Checking if a Worksheet is Filtered
To check if a worksheet is filtered, you can utilize the `AutoFilterMode` property of the worksheet object. This property returns `True` if any filters are applied and “ otherwise.
“`vba
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets(“Sheet1”)
If ws.AutoFilterMode Then
‘ The worksheet is filtered
Else
‘ The worksheet is not filtered
End If
“`
Unfiltering a Worksheet
To remove filters from a worksheet, the `ShowAllData` method can be employed, but it should be used only when a filter is applied. If there are no filters, attempting to execute this method will result in an error.
“`vba
If ws.AutoFilterMode Then
On Error Resume Next
ws.ShowAllData
On Error GoTo 0
End If
“`
Combining Both Checks in a Single Procedure
You can combine the checks for whether the worksheet is filtered and the action to unfilter it into a single subroutine. This streamlines your code and enhances readability.
“`vba
Sub UnfilterIfNeeded()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets(“Sheet1”)
If ws.AutoFilterMode Then
On Error Resume Next
ws.ShowAllData
On Error GoTo 0
End If
End Sub
“`
Additional Considerations
When implementing the unfilter functionality, consider these best practices:
- Error Handling: Always include error handling to manage cases where the `ShowAllData` method cannot be executed.
- User Notifications: If desired, inform users via a message box whether the data was filtered or not.
“`vba
If ws.AutoFilterMode Then
ws.ShowAllData
MsgBox “Filters have been removed.”
Else
MsgBox “No filters were applied.”
End If
“`
- Scope of Action: Ensure that the code targets the correct worksheet. You might want to adapt it to work with multiple sheets or specific ranges.
Example of Full Code Implementation
Here’s a complete example that checks if a specific worksheet is filtered and unfilters it if necessary, while providing user feedback.
“`vba
Sub CheckAndUnfilter()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets(“Sheet1”)
If ws.AutoFilterMode Then
On Error Resume Next
ws.ShowAllData
On Error GoTo 0
MsgBox “Filters have been removed.”
Else
MsgBox “No filters were applied.”
End If
End Sub
“`
This implementation ensures efficient checking and managing of filters using VBA, providing a smooth user experience.
Expert Insights on VBA Filtering Techniques
Jessica Lin (Senior VBA Developer, Excel Innovations Inc.). “In VBA, managing filters effectively is crucial for data analysis. Using an ‘If’ statement to check if a filter is applied can streamline your workflow, allowing for automated unfiltering when necessary. This approach enhances usability and ensures that users can access the complete dataset without manual intervention.”
Michael Chen (Data Analyst, Business Intelligence Solutions). “When implementing a VBA script that checks for filters, it is essential to ensure that the logic is robust. An ‘If’ condition can be utilized to determine if any filters are active, and subsequently trigger an unfilter command. This not only saves time but also reduces the risk of errors that may arise from manual filtering.”
Sarah Thompson (Excel Automation Specialist, TechSavvy Corp.). “Utilizing VBA to manage filters can significantly enhance data handling efficiency. By incorporating an ‘If filtered’ check before executing an unfilter command, developers can create more intuitive applications. This method allows users to maintain focus on their analysis without the distraction of unnecessary data visibility issues.”
Frequently Asked Questions (FAQs)
How can I check if a filter is applied in VBA?
You can check if a filter is applied by examining the `AutoFilterMode` property of the worksheet. If it returns `True`, a filter is currently applied.
What VBA code can I use to unfilter a range?
To unfilter a range, you can use the following code:
“`vba
If ActiveSheet.AutoFilterMode Then
ActiveSheet.AutoFilterMode =
End If
“`
Can I conditionally unfilter based on specific criteria in VBA?
Yes, you can write a conditional statement to check for specific criteria before unfiltering. For example, check if a certain cell value meets your criteria before executing the unfilter command.
What happens if I try to unfilter a range that is not filtered?
If you attempt to unfilter a range that is not filtered, VBA will not throw an error, and the command will simply have no effect.
Is it possible to reapply the same filter after unfiltering in VBA?
Yes, you can store the filter criteria before unfiltering and then reapply it afterward using the `AutoFilter` method with the stored criteria.
Can I automate the process of checking and unfiltering in a single VBA procedure?
Yes, you can create a single procedure that checks if the filter is applied and unfilters it if necessary, streamlining your code for efficiency.
In summary, utilizing VBA (Visual Basic for Applications) to manage filtered data in Excel can significantly enhance data manipulation efficiency. The ability to check if a worksheet is currently filtered and subsequently unfilter it using VBA code provides users with a powerful tool for automating repetitive tasks. This functionality is particularly useful for users who frequently analyze large datasets, as it streamlines the process of viewing all data without manually adjusting filter settings.
Key takeaways from the discussion include the importance of understanding the Excel object model when working with VBA. Users should familiarize themselves with the properties and methods associated with the AutoFilter object. Additionally, implementing error handling within the VBA code ensures that the program runs smoothly, even if the expected conditions are not met, such as when no filters are applied initially.
Moreover, leveraging VBA to automate the unfiltering process can lead to improved productivity, allowing users to focus on analysis rather than manual data management. By incorporating conditional statements, users can create dynamic scripts that adapt to the current state of the worksheet, providing a seamless experience when toggling between filtered and unfiltered views.
Author Profile
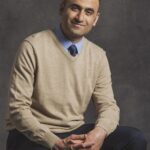
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?