Why Am I Seeing ‘String Was Not Recognized as a Valid DateTime’ Error?
In the world of programming and data manipulation, working with dates and times is a common yet often tricky endeavor. Developers frequently encounter the frustrating error message: “string was not recognized as a valid datetime.” This seemingly innocuous phrase can halt progress and lead to confusion, especially for those new to handling date formats in various programming languages. Understanding the nuances of datetime parsing is essential for anyone looking to build robust applications or analyze data effectively. In this article, we will delve into the intricacies of datetime handling, explore common pitfalls, and provide insights into how to navigate this challenge with confidence.
When dealing with datetime values, the format of the input string is crucial. Different programming languages and frameworks have their own expectations regarding how dates should be represented. A mismatch between the expected format and the actual string can trigger errors that disrupt the flow of your application. This issue often arises in scenarios involving user input, data import from external sources, or even when interfacing with APIs that return date information in various formats.
Moreover, cultural differences in date representation can further complicate matters. For instance, the way dates are formatted can vary significantly from one region to another—some may use the day-month-year format, while others prefer month-day-year. This article will guide you
Understanding the Error
The error message “string was not recognized as a valid datetime” typically occurs in programming environments when a string intended to represent a date and time fails to convert to a valid DateTime object. This can stem from various factors, including incorrect formatting or invalid date values.
Common causes include:
- Format Mismatch: The format of the date string does not match the expected format defined in the system’s locale or program.
- Invalid Date: The string contains a date that does not exist, such as February 30th.
- Culture Settings: Different cultures may interpret date formats differently (e.g., MM/DD/YYYY vs. DD/MM/YYYY).
- Leading or Trailing Spaces: Unintentional spaces can disrupt the parsing process.
Common Scenarios Leading to the Error
Several scenarios can lead to encountering this error message:
- User Input: When user input is not validated properly before attempting conversion.
- Data Import: Importing data from external sources where the date formats vary.
- Database Queries: When dates retrieved from a database do not conform to the expected format.
Handling the Error
To effectively manage and resolve this error, consider the following strategies:
- Input Validation: Always validate user input to ensure it conforms to the expected date format.
- Try-Catch Blocks: Use exception handling to catch and manage errors gracefully during conversion.
- Date Parsing Methods: Utilize robust date parsing methods that can handle multiple formats.
Date Format Handling
When dealing with date conversions, it is critical to ensure that the date string adheres to the expected format. Below is a comparison of common date formats:
Format | Example | Description |
---|---|---|
MM/dd/yyyy | 12/31/2023 | Month/Day/Year (U.S. format) |
dd/MM/yyyy | 31/12/2023 | Day/Month/Year (European format) |
yyyy-MM-dd | 2023-12-31 | ISO 8601 format |
By ensuring that date strings match the expected formats and applying appropriate error handling techniques, the likelihood of encountering the “string was not recognized as a valid datetime” error can be significantly reduced.
Understanding the Error
The error message “string was not recognized as a valid datetime” typically arises when a program attempts to convert a string representation of a date and time into a DateTime object, but the string does not conform to the expected format. This can occur in various programming environments, especially in languages like Cand Java.
Common causes for this error include:
- Incorrect Format: The string does not match the expected date format.
- Culture-Specific Formats: The string uses a format that is not recognized in the current culture settings of the application.
- Invalid Date Values: The string represents a date that does not exist, such as February 30th.
- Leading or Trailing Spaces: Extra whitespace can lead to parsing issues.
Troubleshooting Steps
When encountering this error, follow these troubleshooting steps to resolve the issue:
- Check the Date Format:
- Ensure that the string is in the correct format as expected by your DateTime parsing method.
- Example formats include:
- “MM/dd/yyyy” for US culture
- “dd/MM/yyyy” for many European cultures
- Use TryParse Methods:
- Utilize methods like `DateTime.TryParse` or `DateTime.TryParseExact` to handle parsing safely.
- This approach allows for graceful handling of parsing failures without throwing exceptions.
- Specify the Culture:
- If your application will handle multiple cultures, specify the culture when parsing.
- Example in C:
“`csharp
DateTime dateTime;
bool result = DateTime.TryParse(dateString, new CultureInfo(“en-US”), DateTimeStyles.None, out dateTime);
“`
- Validate Input Strings:
- Implement validation logic to check the string before attempting to parse it.
- Regular expressions can be used to match valid date formats.
Example Scenarios
The following table illustrates common scenarios that can lead to the error along with their respective solutions:
Scenario | Cause | Solution |
---|---|---|
Incorrect date format | “31-12-2023” instead of “12/31/2023” | Adjust the string or specify the expected format |
Culture mismatch | “31/12/2023” in US culture | Use `CultureInfo` to specify the correct culture |
Invalid date | “02/30/2023” | Validate the date before parsing |
Leading/trailing spaces | ” 12/31/2023 “ | Trim whitespace from the string |
Best Practices
To minimize the occurrence of this error in your applications, consider the following best practices:
- Always validate and sanitize input data.
- Use consistent date formats throughout your application.
- Implement comprehensive error handling for date parsing operations.
- Document the expected date formats in your application’s user interface to guide users.
By adhering to these practices, you can enhance the reliability of your date parsing logic and reduce the likelihood of encountering the “string was not recognized as a valid datetime” error.
Understanding the ‘String Was Not Recognized as a Valid Datetime’ Error
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘string was not recognized as a valid datetime’ error typically arises when the input string format does not match the expected date format. It is crucial for developers to ensure that the date strings are properly formatted and validated before parsing to avoid this common pitfall.”
Michael Chen (Data Analyst, Analytics Solutions Group). “In data processing, encountering this error can disrupt workflows significantly. It is essential to implement robust error handling and logging mechanisms to capture the exact input that caused the failure, allowing for easier debugging and resolution of the issue.”
Sarah Thompson (Lead Software Architect, FutureTech Systems). “To mitigate the ‘string was not recognized as a valid datetime’ error, developers should adopt a consistent date format across their applications. Utilizing libraries that handle date parsing can also enhance reliability and reduce the likelihood of format-related errors.”
Frequently Asked Questions (FAQs)
What does the error “string was not recognized as a valid datetime” mean?
This error indicates that a string being parsed into a datetime format does not conform to the expected date and time format, causing the parsing operation to fail.
What are common causes of this error?
Common causes include incorrect date formats (e.g., using “MM/DD/YYYY” instead of “DD/MM/YYYY”), invalid date values (e.g., February 30), and cultural or regional settings that affect date interpretation.
How can I resolve the “string was not recognized as a valid datetime” error?
To resolve this error, ensure that the string being parsed matches the expected datetime format. Use methods such as `DateTime.ParseExact` or `DateTime.TryParseExact` to specify the exact format.
Are there specific formats I should use when parsing dates?
Yes, you should use formats that are consistent with the culture settings of your application. Common formats include “yyyy-MM-dd”, “MM/dd/yyyy”, and “dd-MM-yyyy”, depending on your requirements.
Can this error occur due to culture settings in my application?
Yes, culture settings can affect how dates are interpreted. If your application uses a culture that expects a different date format than what is provided, this error may occur.
What tools or methods can help debug this issue?
You can use debugging tools to inspect the string being parsed and verify its format. Additionally, logging the input string and the expected format can help identify discrepancies leading to the error.
The error message “string was not recognized as a valid datetime” typically arises in programming and data handling contexts when a string representation of a date or time fails to conform to the expected format. This issue is prevalent in various programming languages, including C, Python, and Java, where date and time parsing is a common operation. The underlying cause often stems from discrepancies between the string format provided and the format expected by the parsing function or method being utilized. Understanding the specific format requirements is crucial to resolving this error effectively.
One of the primary insights from the discussion surrounding this error is the importance of consistency in date and time formats. Developers and data analysts must ensure that the input strings align with the expected formats, which can vary based on locale settings, cultural conventions, and the specific programming environment. Utilizing standardized formats, such as ISO 8601 (YYYY-MM-DD), can mitigate the risk of encountering this error. Additionally, implementing robust error handling and validation techniques can help identify and rectify format issues before they lead to runtime errors.
Moreover, leveraging built-in libraries and functions designed for date and time manipulation can significantly reduce the likelihood of format-related errors. Many programming languages offer comprehensive date and time libraries that provide functions for parsing, formatting, and
Author Profile
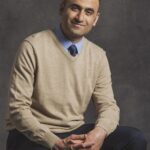
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?