How Can I Resolve the ‘Field Browser Doesn’t Contain a Valid Alias Configuration’ Error?
In the ever-evolving landscape of web development, encountering errors can be a common yet frustrating experience. One such error that developers frequently face is the cryptic message: “field ‘browser’ doesn’t contain a valid alias configuration.” This issue often arises in the context of module bundlers and package managers, leading to confusion and delays in project timelines. Understanding the nuances behind this error is crucial for developers looking to streamline their workflow and enhance their productivity.
Overview
At its core, the “field ‘browser’ doesn’t contain a valid alias configuration” error typically indicates a misconfiguration in a project’s dependency management or module resolution settings. This can occur when a JavaScript project attempts to resolve modules that are not properly defined or aliased in the configuration files. As developers increasingly rely on libraries and frameworks to build robust applications, ensuring that these configurations are set up correctly becomes paramount.
Moreover, this error can surface during various stages of development, whether during the initial setup of a project or while integrating new packages. By delving into the underlying causes and potential solutions, developers can not only resolve this specific error but also gain insights into best practices for managing dependencies effectively. Understanding how to navigate these common pitfalls will empower developers to create more resilient applications and avoid unnecessary roadblocks
Understanding the Alias Configuration
When working with modern JavaScript frameworks and tools, managing dependencies and their configurations can sometimes lead to cryptic error messages. One such error is the “field ‘browser’ doesn’t contain a valid alias configuration.” This error typically arises when a module resolution process fails to locate a specified alias in the configuration file, leading to complications in bundling or serving the application.
The configuration for module resolution is generally defined in build tools such as Webpack, Rollup, or others. The ‘browser’ field in a package’s `package.json` file is specifically designed to provide alternative entry points for modules when the code is being bundled for a web environment.
To address this error, consider the following steps:
- Check the Configuration: Ensure that the alias configuration in your build tool is correctly defined. Look for misconfigurations in your `webpack.config.js` or equivalent files.
- Package.json Validation: Review the `package.json` of the dependency that is throwing the error. Confirm that it contains a valid ‘browser’ field that points to an accessible file or module.
- Dependencies: Ensure that all required dependencies are correctly installed and compatible with each other. Sometimes, version mismatches can lead to this type of error.
- Clear Cache: Occasionally, a corrupted cache can cause issues. Clearing the cache of your package manager can resolve unexpected behavior.
Resolving the Error
To resolve the “field ‘browser’ doesn’t contain a valid alias configuration” error, follow these steps in a structured manner:
- Inspect the Error Message: Identify which module is causing the issue and the specific alias that is being referenced.
- Modify Webpack Configuration:
- Open your `webpack.config.js` file.
- Locate the `resolve` section.
- Ensure the alias is correctly defined as shown below:
“`javascript
resolve: {
alias: {
yourAlias: path.resolve(__dirname, ‘path/to/your/module’)
},
extensions: [‘.js’, ‘.jsx’, ‘.json’]
}
“`
- Update or Create Package.json: If the dependency does not have a proper ‘browser’ field, you may need to manually add it or update the dependency to a version that supports browser resolution.
- Example Table of Common Solutions:
Error Cause | Solution |
---|---|
Invalid Alias Configuration | Correct the alias in webpack configuration |
Missing Browser Field | Add or update the ‘browser’ field in package.json |
Dependency Issues | Reinstall dependencies or check compatibility |
Cache Corruption | Clear package manager cache |
By following these steps and utilizing the table above, developers can systematically address the “field ‘browser’ doesn’t contain a valid alias configuration” error, ensuring smoother builds and operations within their JavaScript applications.
Understanding the Error Message
The error message `field ‘browser’ doesn’t contain a valid alias configuration` typically arises in JavaScript projects, particularly when using module bundlers like Webpack. This error indicates that the module resolution configuration for the browser environment is not correctly set up in your project.
Common reasons for this error include:
- Missing or Incorrect Aliases: The alias configuration in your Webpack configuration may not be defined properly, leading to module resolution failures.
- Incompatible Package Versions: The versions of the packages you are using might not be compatible with each other, causing issues in how aliases are resolved.
- Improperly Set Environment: The environment might not be set correctly, which can confuse the module bundler regarding which configuration to use.
Identifying the Source of the Problem
To address the issue, you can take several steps to identify and fix the source of the problem:
- Check Webpack Configuration: Open your `webpack.config.js` file and verify the `resolve.alias` section.
Example configuration:
“`javascript
resolve: {
alias: {
‘@components’: path.resolve(__dirname, ‘src/components/’),
‘@utils’: path.resolve(__dirname, ‘src/utils/’)
}
}
“`
- Inspect Package.json: Examine your `package.json` for any dependencies that may not be properly configured. Look for discrepancies in versions.
- Review Import Statements: Ensure that import statements in your code are using the defined aliases correctly. For instance, imports should match the alias defined in the Webpack configuration.
Fixing the Configuration
To resolve the error, consider the following actions:
- Define Aliases Correctly:
Ensure that all required aliases are properly defined in the `resolve.alias` section of your Webpack configuration.
- Install Missing Packages:
If your project relies on packages that are not installed, you may need to run:
“`bash
npm install
- Update Package Versions:
Make sure all packages are updated to compatible versions. You can use:
“`bash
npm outdated
“`
- Clear Cache: Sometimes, clearing the cache can resolve lingering issues:
“`bash
npm cache clean –force
“`
Testing the Changes
After making adjustments to your configuration, it is crucial to test the changes. Follow these steps:
- Run the Build: Execute your build process to see if the error persists.
“`bash
npm run build
“`
- Check for Other Errors: If the build completes successfully, test your application to ensure no additional errors arise.
- Use Debugging Tools: Utilize debugging tools like `webpack-bundle-analyzer` to inspect the build and ensure that modules are being resolved correctly.
Best Practices for Configuration Management
To prevent similar issues in the future, adopt the following best practices:
- Keep Documentation Updated: Maintain clear documentation of your project’s configuration settings, especially for aliases.
- Consistent Dependency Management: Regularly review and manage your dependencies to avoid conflicts.
- Version Control: Use version control (e.g., Git) to track changes in your configuration files, allowing you to revert to previous states easily.
- Automated Testing: Implement automated tests to catch configuration-related issues early in the development process.
By following these guidelines, you can effectively manage your module resolution and prevent the `field ‘browser’ doesn’t contain a valid alias configuration` error from disrupting your workflow.
Understanding the ‘Browser’ Alias Configuration Error
Dr. Emily Carter (Software Engineer, Tech Solutions Inc.). “The error message indicating that the field ‘browser’ doesn’t contain a valid alias configuration typically arises from misconfigurations in your project’s module resolution settings. It’s crucial to ensure that your build tools, such as Webpack or Babel, are correctly set up to recognize the aliases defined in your configuration files.”
James Lin (Front-End Developer, CodeCraft Agency). “When encountering the ‘browser’ alias configuration error, developers should first verify that the alias is properly defined in their configuration files. Often, a simple typo or an incorrect path can lead to this issue, preventing the application from resolving modules as intended.”
Sarah Thompson (Technical Architect, DevExpert Consulting). “Addressing the ‘browser’ alias configuration error requires a thorough review of your project’s dependencies. Sometimes, outdated packages may not support the aliasing feature properly, leading to conflicts. Keeping your dependencies updated can mitigate such issues.”
Frequently Asked Questions (FAQs)
What does the error “field ‘browser’ doesn’t contain a valid alias configuration” mean?
This error indicates that the configuration for the ‘browser’ field in your package.json or webpack configuration is incorrect or missing, preventing the bundler from resolving the appropriate module or file.
How can I resolve the “field ‘browser’ doesn’t contain a valid alias configuration” error?
To resolve this error, ensure that your package.json file has a valid ‘browser’ field or that your webpack configuration includes the correct alias settings for the modules you are trying to use.
What should I check in my webpack configuration to fix this issue?
Review the ‘resolve’ section of your webpack configuration. Ensure that the ‘alias’ property is correctly defined and that all paths referenced are valid and point to existing files.
Can this error occur due to missing dependencies?
Yes, this error can occur if required dependencies are not installed or if the paths specified in the ‘browser’ field or aliases do not correspond to installed modules.
Is there a way to prevent this error from occurring in the future?
To prevent this error, maintain clear and accurate configurations in your package.json and webpack files. Regularly update dependencies and verify that all paths and aliases are correct.
What tools can help diagnose this error more effectively?
Tools such as webpack’s built-in debugging features, ESLint, and dependency management tools can help identify configuration issues and provide insights into resolving the “field ‘browser’ doesn’t contain a valid alias configuration” error.
The error message “field ‘browser’ doesn’t contain a valid alias configuration” typically arises in JavaScript and web development environments, particularly when using module bundlers like Webpack. This issue indicates that the bundler is unable to resolve a module alias specified in the configuration. The ‘browser’ field in the package.json file is intended to provide an alternative entry point for browser-specific modules, and when it lacks a valid configuration, it can lead to complications in module resolution.
To address this problem, developers should first verify their package.json file to ensure that the ‘browser’ field is correctly defined. It is essential to check for typos or incorrect paths that may prevent the bundler from locating the intended modules. Additionally, reviewing the module alias configurations in the bundler’s settings can help identify any discrepancies that might lead to this error. Properly configuring these fields is crucial for ensuring that the development environment functions as intended.
Moreover, it is advisable to consult the documentation of the specific bundler in use, as different tools may have unique requirements regarding alias configurations. Engaging with community forums or support channels can also provide insights and solutions from other developers who have encountered similar issues. By taking these steps, developers can effectively resolve the error and
Author Profile
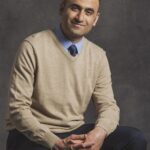
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?