What Does the Hashtag Symbol Mean in TypeScript?
In the ever-evolving world of programming languages, TypeScript has emerged as a powerful tool for developers seeking to enhance their JavaScript applications with static typing and advanced features. Among the various symbols and syntax that developers encounter, the hashtag, or pound sign (), has sparked curiosity and confusion. What does this seemingly simple character signify in the context of TypeScript? As we delve into the intricacies of this language, understanding the role of the hashtag can unlock new possibilities for writing cleaner, more efficient code.
The hashtag in TypeScript is often associated with the concept of private fields in classes, a feature that aligns with the language’s goal of providing better encapsulation and data protection. By using the hashtag, developers can define properties that are not accessible outside of the class, ensuring that sensitive data remains secure and reducing the risk of unintended interactions. This encapsulation is crucial for building robust applications, as it allows for clearer interfaces and better control over how data is manipulated.
As we explore the implications of the hashtag in TypeScript, we will uncover its significance in modern software development. From enhancing code readability to promoting best practices in object-oriented programming, the hashtag plays a pivotal role in shaping how developers approach class design and data management. Join us as we unravel the mysteries of this symbol and
Understanding the Hashtag in TypeScript
In TypeScript, the hashtag symbol (“) is primarily used to denote private fields in classes. This feature was introduced in ECMAScript Private Fields, which enables developers to encapsulate class properties and methods, ensuring they are not accessible from outside the class. This encapsulation is a core principle of object-oriented programming, promoting better data integrity and security.
Private fields are defined by prefixing the field name with a “. For instance:
“`typescript
class Example {
privateField: number;
constructor(value: number) {
this.privateField = value;
}
getPrivateField() {
return this.privateField;
}
}
“`
In the code above, `privateField` is a private property of the `Example` class. It can only be accessed within the class methods, enhancing data protection.
Benefits of Using Hashtags for Private Fields
Utilizing the “ syntax for private fields brings several advantages:
- Encapsulation: It restricts access to class properties, which helps in maintaining a clean interface.
- Reduced Complexity: It simplifies understanding the flow of data within a class since the internal state is hidden from external manipulation.
- Error Prevention: It minimizes the risk of accidental modifications from outside the class, leading to fewer bugs.
Comparison of Private Fields and Other Access Modifiers
TypeScript provides various ways to control access to class members, such as `public`, `private`, and `protected`. The of the hashtag for private fields creates a clear distinction.
Access Modifier | Description | Example |
---|---|---|
public | Accessible from anywhere. | “`public fieldName: type;“` |
private | Accessible only within the class. | “`private fieldName: type;“` |
protected | Accessible within the class and subclasses. | “`protected fieldName: type;“` |
(Private Field) | Strictly accessible only within the class. | “`fieldName: type;“` |
The above table illustrates how the hashtag private field differs from traditional access modifiers in terms of accessibility and usage. The “ syntax provides a stronger encapsulation model, aligning more closely with modern programming practices.
The of the “ symbol for private fields in TypeScript enhances the language’s support for encapsulation and data protection. By using private fields, developers can create more robust and maintainable code, adhering to the principles of object-oriented design.
Understanding the Hashtag in TypeScript
In TypeScript, the hashtag symbol (“) is primarily used for private fields in class definitions. This feature is part of the ECMAScript private class fields proposal, which TypeScript supports to enhance encapsulation.
Private Class Fields
Private class fields allow developers to define properties that cannot be accessed from outside the class. This encapsulation helps maintain a clean and manageable codebase, ensuring that internal states are protected.
- Syntax Example:
“`typescript
class MyClass {
privateField: number;
constructor(value: number) {
this.privateField = value;
}
getPrivateField() {
return this.privateField;
}
}
const instance = new MyClass(10);
console.log(instance.getPrivateField()); // Outputs: 10
// console.log(instance.privateField); // Error: Private field ‘privateField’ must be declared in an enclosing class
“`
In the above example, `privateField` is a private field that cannot be accessed directly outside `MyClass`. Instead, a public method `getPrivateField()` is provided to access its value.
Key Characteristics of Private Fields
- Accessibility: Only accessible within the class where they are defined.
- Visibility: They do not appear in the instances of the class.
- No Inheritance: Private fields are not inherited in derived classes.
Benefits of Using Private Fields
Utilizing private fields in TypeScript offers several advantages:
- Encapsulation: Protects the internal state from outside interference and misuse.
- Clarity: Signals to other developers which properties are meant to be internal and should not be accessed directly.
- Maintainability: Simplifies debugging and refactoring, as internal details can be changed without affecting external code.
Comparison with Other Visibility Modifiers
TypeScript provides other visibility modifiers, such as `public` and `protected`. Here’s a comparison:
Modifier | Accessibility | Example |
---|---|---|
`public` | Accessible from anywhere | `public field: number;` |
`protected` | Accessible in the class and subclasses | `protected field: number;` |
`private` | Accessible only within the class | `privateField: number;` |
Conclusion on the Use of Hashtags
The “ symbol in TypeScript signifies the beginning of private fields, providing a robust mechanism for encapsulation. Understanding how to effectively use private fields enhances the object-oriented capabilities of TypeScript and leads to better-structured code.
Understanding the Role of Hashtags in TypeScript
Dr. Emily Carter (Senior Software Engineer, TypeScript Innovations). Hashtags in TypeScript, often referred to as ‘decorators’, serve as a powerful tool for adding metadata to classes and methods. They enable developers to enhance the functionality of their code by allowing additional behavior to be attached to existing entities without altering their structure.
Michael Thompson (Lead Developer, CodeCraft Solutions). In TypeScript, hashtags are used in the context of decorators, which are a special kind of declaration that can be attached to a class, method, accessor, property, or parameter. They provide a way to modify the behavior of these elements at runtime, facilitating advanced programming patterns such as aspect-oriented programming.
Sarah Kim (Technical Writer, TypeScript Community). The use of hashtags in TypeScript is essential for implementing features like dependency injection and logging. By leveraging decorators, developers can create more modular and maintainable code, making it easier to manage complex applications and improve overall code quality.
Frequently Asked Questions (FAQs)
What does a hashtag mean in TypeScript?
A hashtag in TypeScript typically refers to the “ symbol used to denote private fields in classes. This syntax is part of the class field declarations, allowing encapsulation of class properties.
How do private fields work with hashtags in TypeScript?
Private fields, indicated by a “, restrict access to the field only within the class that defines it. This prevents external code from accessing or modifying the field directly, enhancing data encapsulation.
Can you access private fields outside the class in TypeScript?
No, private fields prefixed with “ cannot be accessed outside their defining class. Attempting to do so will result in a syntax error, ensuring strict encapsulation.
Are hashtags in TypeScript the same as traditional hashtags used in social media?
No, hashtags in TypeScript serve a different purpose. While social media hashtags categorize content, the “ symbol in TypeScript specifically denotes private class members.
What are the benefits of using private fields with hashtags in TypeScript?
Using private fields with hashtags enhances data protection, promotes better software design by enforcing encapsulation, and helps prevent unintended interactions with class internals.
Is the use of hashtags for private fields a standard feature in TypeScript?
Yes, the use of hashtags for private fields is part of the ECMAScript proposal for class fields and is supported in TypeScript, providing a standardized way to define private properties in classes.
In TypeScript, the hashtag symbol () is primarily associated with private class fields. This feature, introduced in ECMAScript proposals and subsequently adopted in TypeScript, allows developers to define class properties that are not accessible outside of the class itself. By using the hashtag, developers can encapsulate data, promoting better data hiding and integrity within their class structures.
The use of private fields enhances the object-oriented programming paradigm by ensuring that certain properties are only modifiable through designated methods within the class. This encapsulation aids in maintaining a clear interface for class users, reducing the likelihood of unintended side effects from external modifications. Consequently, it contributes to more robust and maintainable codebases.
Moreover, the of private fields aligns TypeScript with modern JavaScript practices, making it easier for developers transitioning from JavaScript to TypeScript. It also fosters a clearer understanding of class design and reinforces the principles of encapsulation, which are fundamental to effective object-oriented programming.
In summary, the hashtag in TypeScript signifies private class fields, enhancing encapsulation and data integrity. This feature not only modernizes TypeScript but also supports better software design principles, making it a valuable addition for developers aiming to write cleaner and more maintainable code.
Author Profile
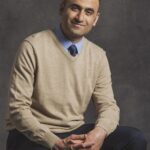
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?