Why Am I Getting an ‘Index Outside Bounds of Array’ Error?
Have you ever encountered the frustrating error message “index outside bounds of array”? If you’ve spent any time programming, chances are you have. This common pitfall can leave even seasoned developers scratching their heads, wondering where they went wrong. As we delve into the world of arrays—one of the fundamental data structures in programming—understanding the nuances of indexing becomes crucial. This article will not only demystify the concept of array bounds but also equip you with strategies to avoid this pesky error in your coding endeavors.
Overview
At its core, the error “index outside bounds of array” occurs when a program attempts to access an element at an index that doesn’t exist within the defined limits of an array. Each array has a specific size, and trying to access an index that is negative or greater than or equal to this size leads to this error. This issue can arise in various programming languages, each with its own way of handling arrays and their indices, making it essential for developers to grasp the underlying principles.
Understanding the implications of array indexing is vital for writing efficient and error-free code. By exploring the reasons behind this error, we can better appreciate how to manage data structures effectively. Whether you’re a novice coder or an experienced programmer, recognizing the common mistakes associated
Understanding Array Indexing
Array indexing is a fundamental concept in programming that allows access to elements stored within an array data structure. Each element in an array is associated with an index that represents its position. Arrays are typically zero-indexed, meaning the first element is accessed with the index 0, the second with index 1, and so forth.
When accessing an array, it is crucial to ensure that the index used is within the valid range. If an index exceeds the bounds of the array, it results in an “index outside bounds of array” error. This error can lead to unexpected behavior in programs, including crashes or incorrect data manipulation.
Common Causes of Indexing Errors
Several common programming mistakes can lead to index out-of-bounds errors:
- Incorrect Loop Conditions: Loops that iterate beyond the length of the array can easily result in accessing invalid indices.
- Dynamic Resizing: Modifying the size of an array (in languages that allow dynamic arrays) without updating all references can lead to invalid accesses.
- Off-by-One Errors: These are frequent mistakes where the programmer accidentally uses an index that is one too high or too low.
Preventing Index Out-of-Bounds Errors
To minimize the risk of encountering index out-of-bounds errors, developers can employ several best practices:
- Always check the length of the array before attempting to access an element.
- Use safe access methods or wrapper functions that handle out-of-bounds checks.
- Implement thorough testing, including edge cases that may expose indexing errors.
The following table summarizes common programming languages and their approaches to array indexing:
Language | Zero-Indexed | Array Bounds Checking |
---|---|---|
Python | Yes | Yes |
Java | Yes | Yes |
C++ | Yes | No ( Behavior) |
JavaScript | Yes | Yes |
Debugging Index Out-of-Bounds Errors
When faced with an index out-of-bounds error, debugging strategies can help identify the source of the issue:
- Print Statements: Use print statements to track the values of indices being accessed.
- Debuggers: Utilize debugging tools to step through the code and monitor the state of the program at runtime.
- Boundary Testing: Test with arrays of various sizes, particularly with empty arrays and arrays with a single element.
By following these guidelines and maintaining awareness of array indexing, developers can significantly reduce the incidence of index out-of-bounds errors in their code.
Understanding Array Indexing
Array indexing is a fundamental concept in programming that allows access to individual elements within an array. Each element in an array is identified by an index, which is typically a non-negative integer.
- Zero-Based Indexing: Most programming languages, including C, C++, Java, and Python, use zero-based indexing. This means:
- The first element is accessed with index `0`.
- The second element is accessed with index `1`, and so on.
- One-Based Indexing: Some languages, like MATLAB and Fortran, use one-based indexing, where:
- The first element is accessed with index `1`.
- The second element is accessed with index `2`, and so forth.
Understanding the indexing system used in a programming language is crucial to avoid errors such as “index outside bounds of array.”
Common Causes of Index Out of Bounds Errors
Index out of bounds errors typically occur due to several common programming mistakes:
- Accessing Beyond the Array Size: Attempting to access an index that is greater than or equal to the array length.
- Negative Index: Using a negative index which is not allowed in most programming languages.
- Dynamic Array Resizing: When resizing an array, if the code tries to access the original indices without adjusting them to the new size.
- Loop Errors: Incorrectly set loop conditions that exceed the array bounds.
Preventing Index Out of Bounds Errors
To prevent these errors, consider implementing the following strategies:
- Bounds Checking: Always verify that an index is within the valid range before accessing an array.
“`python
if 0 <= index < len(array):
element = array[index]
```
- Using Safe Access Methods: Some languages offer built-in methods to safely access array elements, returning a default value if the index is out of bounds.
- Debugging Tools: Leverage debugging tools or features in your IDE that can help track down where index errors occur.
- Unit Testing: Write unit tests that check for edge cases, including accessing the first and last elements, as well as indices that exceed bounds.
Example Scenarios of Index Errors
Scenario | Code Example | Error Type |
---|---|---|
Accessing last element | `array[5]` (for an array of size 5) | Index out of bounds |
Negative index | `array[-1]` | Negative index error |
Incorrect loop condition | `for i in range(6): array[i]` | Index out of bounds for size 5 |
Resizing an array without adjusting indices | `array.append(new_element); array[5]` | Index out of bounds |
Handling Exceptions
In many programming languages, you can handle out-of-bounds access using exceptions. For example:
- Python: You can catch an `IndexError` using a try-except block.
“`python
try:
element = array[index]
except IndexError:
print(“Index is out of bounds.”)
“`
- Java: You can catch an `ArrayIndexOutOfBoundsException`.
“`java
try {
int element = array[index];
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println(“Index is out of bounds.”);
}
“`
Utilizing exception handling can enhance the robustness of your code by gracefully managing errors when they occur, rather than allowing the program to crash.
Understanding Array Indexing Errors in Programming
Dr. Alice Thompson (Senior Software Engineer, CodeSafe Technologies). “Indexing outside the bounds of an array is a common error in programming that can lead to unexpected behavior or application crashes. It is crucial for developers to implement proper boundary checks to prevent such issues.”
Michael Chen (Lead Data Scientist, DataWise Solutions). “When working with arrays, especially in data-intensive applications, understanding the concept of bounds is essential. An ‘index outside bounds’ error can disrupt data processing and lead to significant performance issues.”
Sarah Patel (Computer Science Instructor, Tech University). “Teaching students about array bounds is fundamental in programming courses. It not only helps them avoid runtime errors but also instills a deeper understanding of memory management and data structures.”
Frequently Asked Questions (FAQs)
What does “index outside bounds of array” mean?
This error indicates that a program is attempting to access an array element using an index that is either negative or exceeds the array’s defined size, leading to an invalid memory access.
What causes an “index outside bounds of array” error?
This error typically arises from programming mistakes such as off-by-one errors, incorrect loop conditions, or improper handling of dynamic array sizes, which result in accessing non-existent elements.
How can I fix an “index outside bounds of array” error?
To resolve this error, validate array indices before accessing elements, ensure loop conditions are correctly set, and utilize debugging tools to trace the source of the out-of-bounds access.
Is “index outside bounds of array” specific to any programming language?
No, this error can occur in various programming languages, including C, C++, Java, and Python, as it relates to the fundamental concept of array indexing and memory management.
What are the consequences of ignoring an “index outside bounds of array” error?
Ignoring this error can lead to unpredictable program behavior, including crashes, data corruption, or security vulnerabilities, as it may allow unauthorized access to memory locations.
Can using built-in functions help prevent “index outside bounds of array” errors?
Yes, many programming languages offer built-in functions or methods that automatically handle bounds checking, which can significantly reduce the risk of encountering this error.
The phrase “index outside bounds of array” refers to a common programming error that occurs when a code attempts to access an element of an array using an index that is either negative or exceeds the array’s maximum index. This situation leads to runtime exceptions or errors, which can disrupt the normal flow of a program. Understanding the bounds of an array is crucial for developers, as it ensures that data is accessed safely and correctly, preventing potential crashes or unexpected behavior in applications.
One of the primary causes of this error is a misunderstanding of how arrays are indexed in programming languages. Most languages use zero-based indexing, meaning the first element of an array is accessed with an index of zero, and the last element is accessed with an index equal to the array’s length minus one. Developers must be vigilant in their calculations and logic to avoid referencing indices that fall outside this range.
Key takeaways from the discussion around this topic include the importance of thorough testing and validation of array indices before accessing elements. Implementing checks to ensure that indices are within the valid range can prevent runtime errors. Additionally, leveraging debugging tools and techniques can help identify and resolve these issues during the development process. By adhering to best practices in array management, developers can enhance the reliability and
Author Profile
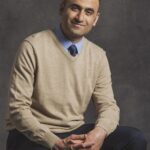
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?