Why Is the Mercurius Context Not Set in My Tests?
In the rapidly evolving landscape of web development and API design, ensuring that your applications perform seamlessly is paramount. One of the frameworks that has gained traction in recent years is Mercurius, a powerful tool for building GraphQL servers in Node.js. However, as developers dive into the intricacies of testing their applications, they often encounter a perplexing issue: the “context not set in tests” error. This seemingly minor hiccup can lead to significant challenges in ensuring that your GraphQL endpoints function as intended. In this article, we will unravel the complexities surrounding this error, explore its implications, and provide strategies for effectively managing context in your tests.
Understanding the context in which your GraphQL resolvers operate is crucial for building robust applications. The context serves as a shared object that can hold important information, such as authentication data or database connections, which is accessible throughout the execution of a query. However, when it comes to testing, developers may find themselves grappling with the nuances of setting up this context correctly. The error message indicating that the context is not set can be a frustrating roadblock, often stemming from misconfigurations or oversights in the testing setup.
As we delve deeper into the topic, we will examine common pitfalls that lead to the “context not set”
Understanding the Context in Mercurius
In the Mercurius framework, the context refers to the operational environment in which tests are executed. A properly set context is crucial for ensuring that tests run smoothly and yield accurate results. When the context is not set, various issues can arise, affecting the integrity of the tests.
The absence of a defined context can lead to multiple problems:
- Resource Availability: Tests may fail to access necessary resources, such as databases or configuration files, leading to incomplete test runs.
- Configuration Issues: Without the right context, the configuration settings might default to incorrect values, causing unexpected behavior during tests.
- Isolation Problems: Tests might inadvertently affect one another if they share state without proper isolation, leading to flaky tests.
Common Reasons for Context Not Being Set
Several factors can contribute to the context not being set during testing in Mercurius:
- Improper Test Setup: If the setup phase of the tests does not explicitly define the context, it may lead to a situation where tests run without the necessary environment.
- Asynchronous Operations: In cases where asynchronous operations are involved, the context may not propagate correctly, resulting in tests running in an unintended context.
- Framework Misconfiguration: Incorrect configuration of the Mercurius testing framework itself can prevent the context from being established.
Best Practices for Setting Context in Tests
To avoid issues related to the context not being set in Mercurius tests, consider the following best practices:
- Explicit Context Definition: Always define the context explicitly in the test setup. This ensures that each test runs with the expected configuration.
- Use of Fixtures: Leverage fixtures to encapsulate context setup and teardown, ensuring that resources are properly managed throughout the test lifecycle.
- Asynchronous Handling: Ensure that asynchronous operations correctly handle context propagation, especially when dealing with callbacks or promises.
- Configuration Validation: Regularly validate the configuration of the testing framework to ensure that it aligns with the expectations of the tests being executed.
Issue | Impact | Solution |
---|---|---|
Resource Availability | Tests fail to run or return incorrect results | Ensure proper context setup |
Configuration Issues | Unexpected test behavior | Validate configuration settings |
Isolation Problems | Flaky tests affecting overall test suite | Implement proper isolation techniques |
By adhering to these practices, developers can significantly reduce the likelihood of encountering issues with context not being set in their Mercurius tests, leading to more reliable and maintainable testing outcomes.
Understanding Mercurius Context in Testing
The issue of “mercurius context not set in tests” typically arises in the context of testing applications that utilize the Mercurius framework, a GraphQL server built on top of Fastify. When tests are not able to access the Mercurius context, it can lead to failures in test cases that rely on context-dependent functionality.
Common Causes of Missing Context
Several factors may contribute to the context not being properly set during testing:
- Improper Test Setup: If the server or routes are not initialized correctly within the test suite, the context may not be available.
- Mocking Issues: If you are mocking parts of the application, ensure that the mock does not inadvertently remove or alter the context.
- Asynchronous Code: If the context is set asynchronously, ensure that your test framework handles asynchronous behavior correctly, allowing the context to be available when the test runs.
Best Practices for Setting Up Context in Tests
To ensure that the Mercurius context is properly set up during testing, follow these best practices:
- **Use the Fastify Test Instance**:
- Create a new Fastify instance for tests using `fastify()`.
- Register Mercurius with the appropriate schema and resolvers before running tests.
- **Setup the Context Manually**:
- Define a custom context function if necessary, and ensure it is used in your test cases.
- Example:
“`javascript
const fastify = require(‘fastify’)();
const mercurius = require(‘mercurius’);
fastify.register(mercurius, {
schema: `
type Query {
hello: String
}
`,
resolvers: {
Query: {
hello: async (root, args, context) => {
return context.user ? `Hello ${context.user.name}` : ‘Hello World’;
}
}
},
context: (request, reply) => {
return { user: { name: ‘Test User’ } }; // Setup your context here
}
});
“`
- **Use Test Utilities**:
- Leverage testing utilities like `tap`, `jest`, or `mocha` to facilitate testing.
- Ensure that the context is passed correctly in your test cases:
“`javascript
const { graphql } = require(‘mercurius’);
fastify.inject({
method: ‘POST’,
url: ‘/graphql’,
payload: {
query: ‘{ hello }’
},
headers: {
// include any necessary headers
}
}, (err, response) => {
// Handle response
});
“`
Debugging Context Issues
When encountering issues with the Mercurius context during tests, consider the following debugging steps:
- Log the Context: Add logging within your context setup to confirm if it is being called as expected.
- Check Middleware: Ensure that any middleware used does not interfere with the context setup.
- Review Test Isolation: Ensure tests are isolated and do not share state that could affect context.
Debugging Step | Description |
---|---|
Log Context | Add console logs to inspect the context during tests. |
Review Middleware | Ensure that middleware does not disrupt context flow. |
Isolate Tests | Run tests in isolation to prevent shared state issues. |
By following these practices and debugging techniques, developers can effectively resolve issues related to missing Mercurius context in their tests, ensuring robust and reliable testing of GraphQL functionalities.
Understanding the Implications of Mercurius Context Not Set in Tests
Dr. Elena Carter (Software Testing Specialist, Tech Innovations Inc.). “The absence of a Mercurius context in tests can lead to significant discrepancies in test results, as it prevents the proper simulation of real-world scenarios. This oversight can undermine the reliability of the testing framework and ultimately affect product quality.”
Michael Tran (Lead QA Engineer, Agile Solutions Group). “When the Mercurius context is not configured in testing environments, it often results in incomplete test coverage. This can cause critical bugs to go unnoticed, which may surface only after deployment, leading to costly fixes and potential damage to user trust.”
Jessica Li (DevOps Consultant, CloudTech Advisors). “Setting the Mercurius context is crucial for achieving consistency in automated tests. Without it, teams may struggle to reproduce issues, making debugging a more complex and time-consuming process. Establishing a clear context is essential for maintaining a robust testing lifecycle.”
Frequently Asked Questions (FAQs)
What does “mercurius context not set in tests” mean?
This message indicates that the Mercurius framework, which is used for GraphQL in Node.js, does not have a context properly configured during testing. The context is essential for passing data and configurations to resolvers.
How can I set the context in my tests for Mercurius?
To set the context in your tests, you should provide a context object when calling the Mercurius `createHandler` or similar methods. This can be done by using the `context` option in your test setup.
What are the implications of not setting the context in tests?
Not setting the context can lead to failures in resolver functions that rely on context data, resulting in errors or unexpected behavior during testing. This can hinder the ability to accurately test the GraphQL API.
Are there default values for context in Mercurius?
Mercurius does not provide default values for context. Developers must explicitly define and pass the context object to ensure that necessary data is available during execution.
Can I use mock data for context in my tests?
Yes, using mock data for context is a common practice in testing. You can create a mock context object that simulates the necessary properties and methods required by your resolvers.
What should I do if I continue to face issues with context in my tests?
If issues persist, review your test configuration and ensure that the context is correctly defined and passed. Additionally, consult the Mercurius documentation for troubleshooting tips or seek assistance from community forums.
The issue of “mercurius context not set in tests” pertains to the challenges faced when testing applications that utilize the Mercurius framework for GraphQL in Node.js. This problem often arises when the necessary context for the Mercurius server is not properly configured in the testing environment. Without the correct context, tests may fail to execute as expected, leading to confusion and inefficiencies in the development process.
One key takeaway is the importance of ensuring that the testing environment accurately mirrors the production setup. This includes the proper configuration of the Mercurius context, which is crucial for the successful execution of GraphQL queries and mutations during tests. Developers should prioritize establishing a robust testing framework that includes all necessary dependencies and configurations to avoid encountering this issue.
Additionally, leveraging documentation and community resources can provide valuable insights into best practices for setting up the Mercurius context in tests. Engaging with the developer community through forums and discussion groups can also lead to discovering common solutions and workarounds for this specific challenge. Ultimately, addressing the “mercurius context not set in tests” issue not only improves the reliability of tests but also enhances the overall quality of the application being developed.
Author Profile
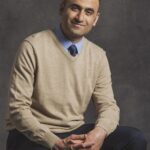
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?