Why Am I Getting ‘An Error is Expected but Got Nil’ in My Code?
In the realm of programming and software development, encountering errors is an inevitable part of the journey. However, what happens when you expect an error to occur, only to be met with silence—specifically, a nil value? This perplexing scenario can leave developers scratching their heads, questioning their logic and the very foundations of their code. The phrase “an error is expected but got nil” encapsulates a common frustration in debugging, where the anticipated chaos of an error message is replaced by an unsettling void. This article delves into the nuances of this phenomenon, exploring its implications, potential causes, and effective strategies for resolution.
Overview
When developers write code, they often build in error handling mechanisms to catch and respond to issues that may arise during execution. However, there are instances when the expected error fails to materialize, leaving a nil response in its place. This situation can arise from various factors, including incorrect assumptions about the data being processed, overlooked edge cases, or even flaws in the error handling logic itself. Understanding why this occurs is crucial for improving code reliability and ensuring that applications behave as intended.
Navigating the landscape of programming errors requires a blend of analytical thinking and practical troubleshooting skills. By examining the scenarios in which an expected error yields a nil
Error Handling in Programming
Error handling is a crucial aspect of programming, as it allows developers to anticipate and manage potential issues that arise during execution. The phrase “an error is expected but got nil” typically indicates a situation where the code is designed to handle specific errors, but instead, it received a `nil` value, which may not have been anticipated. This discrepancy can lead to unexpected behavior in applications.
Key concepts in error handling include:
- Error Types: Understanding the different types of errors that can occur, such as syntax errors, runtime errors, and logical errors.
- Error Propagation: Managing how errors are passed through different layers of an application, ensuring they are logged or handled appropriately.
- Graceful Degradation: Designing systems that can continue to function, albeit at a reduced level, when an error occurs.
Common Causes of Nil Errors
Nil errors can arise from various programming practices or oversights. Some common causes include:
- Uninitialized Variables: Variables that have not been assigned a value may default to `nil`, leading to errors when accessed.
- Incorrect Function Return Values: Functions expected to return an error object may instead return `nil` under certain conditions.
- Data Type Mismatches: Operations on incompatible data types can inadvertently result in a `nil` return.
Strategies for Handling Nil Errors
To effectively manage nil errors, developers can employ several strategies:
- Input Validation: Always check inputs for validity before processing.
- Error Checking: Implement checks after function calls to determine if the expected output is `nil`.
- Logging: Maintain logs to record when and where nil values occur for easier debugging.
Here’s a summary table of potential strategies and their applications:
Strategy | Description | Application |
---|---|---|
Input Validation | Ensuring inputs meet required criteria before processing. | Form submissions, API requests |
Error Checking | Assessing return values from functions to catch errors early. | Database queries, external service calls |
Logging | Recording occurrences of nil values for future debugging. | Application monitoring, error reporting |
Best Practices for Avoiding Nil Errors
Adopting best practices can significantly reduce the occurrence of nil errors:
- Consistent Initialization: Always initialize variables upon declaration.
- Clear Documentation: Document functions to specify expected return values clearly.
- Unit Testing: Implement comprehensive unit tests that include edge cases for nil scenarios.
By integrating these practices into the development process, programmers can minimize the risks associated with unexpected nil values and enhance the reliability of their applications.
Understanding the Error Message
The error message “an error is expected but got nil” typically arises in programming contexts, particularly in languages that utilize error handling. This message indicates that the code execution anticipated an error to occur, but instead, it received a `nil` value, which can signify the absence of a value or a non-existent object.
Key aspects of this error include:
- Context of Occurrence: The error often appears in scenarios involving functions or methods that are expected to return an error object.
- Programming Languages: Commonly encountered in languages such as Go, Swift, and other languages that utilize explicit error handling.
Common Causes
Several factors can lead to this error message:
- Function Expectations: A function is designed to return an error along with a value, but the implementation does not align with this expectation.
- Conditional Logic: Logic paths within the code may inadvertently lead to a state where an error is not thrown, despite the expectation of failure.
- Misconfigured Error Handling: Improperly set up error handling may result in situations where `nil` is returned instead of an appropriate error message.
Debugging Strategies
To address the issue, consider the following debugging strategies:
- Review Function Definitions: Ensure that functions are designed to return errors appropriately when conditions warrant.
- Check Logic Paths: Trace through the code to verify that all possible outcomes are accounted for, especially those that should trigger an error.
- Add Logging: Implement logging statements to capture the flow of execution and identify where the function fails to return an expected error.
- Use Assertions: Incorporate assertions to validate assumptions about error handling throughout the code.
Example Scenario
Here is an illustrative example in Go that demonstrates how this error can arise:
“`go
func performOperation() (result int, err error) {
if someConditionFails {
return 0, errors.New(“operation failed”)
}
return 42, nil // Correctly returns a value with no error
}
func main() {
result, err := performOperation()
if err != nil {
fmt.Println(“Error occurred:”, err)
} else {
fmt.Println(“Result:”, result)
}
}
“`
In the above code, if `someConditionFails` is not properly handled, the function might return a `nil` when an error was expected.
Best Practices for Error Handling
To minimize occurrences of this error, adhere to best practices in error handling:
- Consistent Error Returns: Always return an error alongside results from functions that may encounter issues.
- Comprehensive Testing: Implement unit tests that validate both success and failure cases to ensure errors are handled as expected.
- Clear Documentation: Document function contracts clearly, specifying what callers should expect in terms of return values and errors.
Practice | Description |
---|---|
Consistent Error Returns | Ensure every function returns an error when applicable |
Comprehensive Testing | Test for both successful and erroneous outcomes |
Clear Documentation | Clearly state expected return values and errors |
Understanding the Implications of Nil Errors in Programming
Dr. Emily Carter (Software Reliability Engineer, Tech Innovations Inc.). “Encountering a situation where an error is expected but nil is returned can often indicate a flaw in the error handling mechanism. It is crucial to ensure that all potential failure points are adequately monitored and that the system is designed to return meaningful error messages that can guide developers in troubleshooting.”
James Thornton (Lead Developer, CodeGuard Solutions). “When developers see ‘an error is expected but got nil’, it usually suggests that the logic within the application may not be robust enough to handle edge cases. Implementing comprehensive unit tests can help identify these scenarios before they escalate into production issues.”
Linda Zhao (Senior Software Architect, FutureTech Labs). “This type of error is a classic example of a silent failure in software systems. It is essential to adopt defensive programming practices, ensuring that every function returns a value, even in the event of an error, to avoid confusion and facilitate debugging.”
Frequently Asked Questions (FAQs)
What does the error message “an error is expected but got nil” indicate?
This message typically indicates that a function or operation was expected to return an error object, but instead returned a nil value, suggesting that the error handling may not be functioning as intended.
In which programming languages is this error commonly encountered?
This error is commonly encountered in languages like Go, where explicit error handling is a standard practice, but it can also appear in other languages that utilize similar error handling paradigms.
What are the common causes of receiving a nil value instead of an error?
Common causes include improper error handling logic, overlooking error return values, or the function being designed to return nil under certain conditions that were not accounted for.
How can I troubleshoot this error in my code?
To troubleshoot, review the function calls to ensure that all error returns are properly checked. Implement logging to capture the flow of execution and identify where the nil return occurs.
What steps can I take to ensure that errors are handled correctly in my program?
Ensure that all functions return an error value and that the calling code checks for this value. Use consistent error handling patterns and consider employing unit tests to validate error scenarios.
Is there a way to avoid this error in future developments?
To avoid this error, adopt best practices for error handling, such as using comprehensive error checks, implementing thorough testing, and adhering to coding standards that emphasize explicit error management.
The phrase “an error is expected but got nil” typically arises in programming and software development contexts, particularly in languages that utilize error handling mechanisms. This situation indicates that the code anticipated an error to occur during execution, but instead, it received a ‘nil’ value, which often signifies the absence of an error or an unexpected state. This discrepancy can lead to confusion and bugs, as the logic of the program may not account for the possibility of receiving a nil value when an error was anticipated.
One of the key takeaways from this discussion is the importance of robust error handling in software development. Developers must ensure that their code can gracefully handle various outcomes, including unexpected nil values. This involves implementing thorough checks and balances within the code to account for different scenarios, thereby preventing potential failures and ensuring a smoother user experience.
Additionally, the occurrence of “an error is expected but got nil” serves as a reminder of the need for comprehensive testing and debugging practices. By rigorously testing code under various conditions, developers can identify and rectify areas where assumptions about error handling may not hold true. This proactive approach not only enhances the reliability of the software but also contributes to overall code quality and maintainability.
Author Profile
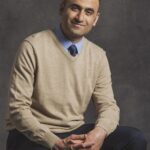
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?