How Can You Effectively Implement A/B Testing in Python?
In the fast-paced world of digital marketing and product development, making data-driven decisions is more critical than ever. Enter A/B testing—a powerful technique that allows businesses to compare two versions of a webpage, app feature, or marketing campaign to determine which one performs better. With the rise of Python as a go-to programming language for data analysis and machine learning, leveraging A/B testing has never been more accessible. This article will guide you through the essentials of A/B testing using Python, empowering you to harness the full potential of your data and optimize your strategies effectively.
A/B testing, also known as split testing, is a method where two variants (A and B) are compared to see which one yields better results based on predefined metrics. By randomly assigning users to either group, businesses can gain insights into user behavior and preferences, leading to more informed decisions. In the realm of Python, a variety of libraries and frameworks are available that simplify the implementation of A/B tests, making it easier for both seasoned developers and newcomers alike to dive into this analytical approach.
As we explore A/B testing in Python, we will touch on the foundational concepts, the statistical significance behind the tests, and the practical tools available to streamline the process. Whether you’re looking to enhance user engagement,
Understanding A/B Testing in Python
A/B testing, also known as split testing, is a method used to compare two versions of a webpage or app against each other to determine which one performs better. In Python, A/B testing can be implemented using various libraries and frameworks that facilitate statistical analysis and data visualization.
To conduct A/B testing in Python, the following steps are typically involved:
- Define the Objective: Clearly outline what you want to measure, such as conversion rates, click-through rates, or user engagement.
- Create Variants: Develop two versions of the same element (A and B) that you want to test.
- Randomly Assign Users: Use a method to randomly assign users to either group A or group B to ensure unbiased results.
- Collect Data: Gather data on user interactions and performance metrics.
- Analyze Results: Use statistical tests to determine if the differences in performance are significant.
Python Libraries for A/B Testing
There are several Python libraries that facilitate A/B testing. Here are some of the most commonly used:
- SciPy: A library for scientific and technical computing that provides functions for statistical tests.
- statsmodels: This library offers a more comprehensive suite for statistical modeling and hypothesis testing.
- PyAB: A lightweight framework specifically designed for A/B testing in Python.
- Matplotlib/Seaborn: Useful for data visualization to present the results of your tests clearly.
Implementing A/B Testing with Python
To illustrate how A/B testing can be implemented in Python, consider the following example using the SciPy library for statistical analysis.
“`python
import numpy as np
from scipy import stats
Sample data: conversions for group A and B
group_a_conversions = np.array([30, 35, 45, 50, 60])
group_b_conversions = np.array([25, 30, 40, 55, 65])
Perform a t-test
t_statistic, p_value = stats.ttest_ind(group_a_conversions, group_b_conversions)
Output the results
print(f”T-statistic: {t_statistic}, P-value: {p_value}”)
“`
In the example above, we create two arrays representing the conversion data for groups A and B. A t-test is then performed to determine if there is a statistically significant difference in conversions between the two groups.
Interpreting A/B Testing Results
The key to interpreting the results of an A/B test lies in understanding the p-value obtained from the statistical tests. Here’s a quick guide:
- P-value < 0.05: Indicates strong evidence against the null hypothesis, suggesting that the variations have a significant impact.
– **P-value >= 0.05**: Indicates weak evidence against the null hypothesis, suggesting that the variations do not have a significant impact.
P-value Range | Interpretation |
---|---|
0.00 – 0.01 | Strong evidence against the null hypothesis |
0.01 – 0.05 | Moderate evidence against the null hypothesis |
0.05 – 0.10 | Weak evidence against the null hypothesis |
0.10+ | Insufficient evidence against the null hypothesis |
By following these guidelines and utilizing Python’s rich ecosystem of libraries, practitioners can effectively conduct A/B tests and draw meaningful conclusions from their data.
A/B Testing in Python
A/B testing is a statistical method used to compare two versions of a variable to determine which one performs better. Implementing A/B testing in Python can be achieved using various libraries and frameworks that streamline the process. Below are key components and methods for conducting A/B testing effectively.
Key Libraries for A/B Testing
Several libraries facilitate A/B testing in Python, providing tools for statistical analysis and data visualization:
- SciPy: For statistical tests (e.g., t-tests, chi-square tests).
- Pandas: For data manipulation and analysis.
- Matplotlib/Seaborn: For visualizing results.
- Statsmodels: For advanced statistical modeling.
Setting Up A/B Testing
To set up an A/B test, follow these structured steps:
- Define the Hypothesis: Clearly state what you are testing (e.g., “Changing the button color will increase the click-through rate”).
- Identify Metrics: Decide which metrics will measure success (e.g., conversion rates, revenue per user).
- Select Sample Size: Determine the number of users needed for valid results, often calculated using power analysis.
- Random Assignment: Randomly assign users to either the control group (A) or the experimental group (B).
Conducting A/B Testing with Python
Here is a basic example of conducting an A/B test using Python:
“`python
import pandas as pd
from scipy import stats
Sample data
data = {
‘group’: [‘A’] * 100 + [‘B’] * 100,
‘conversion’: [0] * 90 + [1] * 10 + [0] * 80 + [1] * 20
}
df = pd.DataFrame(data)
Calculate conversion rates
conversion_rates = df.groupby(‘group’)[‘conversion’].mean()
print(conversion_rates)
Perform a t-test
t_stat, p_value = stats.ttest_ind(
df[df[‘group’] == ‘A’][‘conversion’],
df[df[‘group’] == ‘B’][‘conversion’]
)
print(f”T-statistic: {t_stat}, P-value: {p_value}”)
“`
Interpreting Results
After running the A/B test, it is essential to interpret the results correctly:
- P-Value: Indicates the probability of observing the test results under the null hypothesis. A p-value less than 0.05 typically suggests statistical significance.
- Confidence Intervals: Provide a range of values that likely contain the true effect size.
Metric | Group A | Group B |
---|---|---|
Conversion Rate | 10% | 20% |
P-Value | < 0.05 | |
Confidence Interval | 5% – 15% | 15% – 25% |
Best Practices for A/B Testing
To enhance the reliability of A/B tests, consider the following best practices:
- Test One Variable at a Time: Isolate the effect of a single change.
- Run Tests Long Enough: Ensure that tests run long enough to gather sufficient data, avoiding short-term fluctuations.
- Ensure Randomization: Confirm that user assignment to groups is random to eliminate biases.
- Monitor External Factors: Be aware of other variables that could influence results, such as marketing campaigns or seasonal trends.
By adhering to these principles and utilizing Python’s capabilities, one can conduct effective A/B testing that yields actionable insights.
Expert Insights on A/B Testing in Python
Dr. Emily Carter (Data Scientist, Analytics Innovations). “A/B testing in Python is essential for data-driven decision-making. Utilizing libraries like SciPy and statsmodels allows for robust statistical analysis, ensuring that the results of your tests are both reliable and actionable.”
James Liu (Product Manager, Tech Solutions Inc.). “Implementing A/B testing in Python can significantly enhance user experience by allowing teams to experiment with different features. Frameworks such as Flask or Django can facilitate the deployment of these tests seamlessly within web applications.”
Sarah Thompson (Marketing Analyst, Digital Metrics Group). “The beauty of A/B testing in Python lies in its flexibility. By leveraging libraries like Pandas for data manipulation and Matplotlib for visualization, marketers can easily interpret results and optimize campaigns effectively.”
Frequently Asked Questions (FAQs)
What is A/B testing in Python?
A/B testing in Python refers to the process of comparing two versions of a variable to determine which one performs better. This is commonly used in marketing and product development to optimize user engagement and conversion rates.
How can I perform A/B testing using Python?
To perform A/B testing in Python, you can use libraries such as `scipy` for statistical analysis and `pandas` for data manipulation. You typically start by defining your hypothesis, collecting data, and then using statistical tests to analyze the results.
What libraries are useful for A/B testing in Python?
Key libraries for A/B testing in Python include `scipy` for statistical tests, `statsmodels` for more advanced statistical modeling, and `pandas` for data manipulation. Visualization can be aided by libraries like `matplotlib` and `seaborn`.
How do I interpret the results of an A/B test?
Interpreting A/B test results involves analyzing metrics such as conversion rates and statistical significance. A common approach is to calculate the p-value; if it is below a predetermined threshold (often 0.05), the difference between groups is considered statistically significant.
What are common pitfalls in A/B testing?
Common pitfalls in A/B testing include insufficient sample size, running tests for too short a duration, failing to randomize participants, and not accounting for external variables that may influence results. These can lead to misleading conclusions.
Can A/B testing be automated in Python?
Yes, A/B testing can be automated in Python using scripts that handle data collection, analysis, and reporting. Frameworks like `Airflow` can be employed to schedule and manage A/B test workflows effectively.
A/B testing in Python is a powerful technique used to compare two or more variations of a webpage, product, or feature to determine which one performs better. By utilizing statistical methods, A/B testing allows businesses to make data-driven decisions that can enhance user engagement and improve conversion rates. Python, with its rich ecosystem of libraries such as SciPy, StatsModels, and Pandas, provides robust tools for implementing and analyzing A/B tests efficiently.
One of the key aspects of A/B testing is the importance of proper experimental design. This includes defining clear hypotheses, selecting appropriate metrics for evaluation, and ensuring that the sample size is sufficient to achieve statistically significant results. Python facilitates these processes through its analytical capabilities, allowing users to visualize data and interpret results effectively. Additionally, libraries like Matplotlib and Seaborn can be employed to create informative visualizations that aid in understanding the outcomes of the tests.
Moreover, it is crucial to consider the ethical implications of A/B testing, such as user consent and the potential impact on user experience. By adhering to best practices and ethical standards, organizations can conduct A/B tests that not only yield valuable insights but also maintain trust with their users. Overall, Python serves as an invaluable resource for practitioners looking to implement
Author Profile
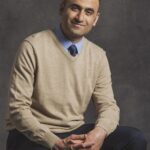
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?