How Can I Add a Class to an Admin Menu Item?
In the world of web development, particularly when working with content management systems like WordPress, customizing the admin interface can significantly enhance user experience and functionality. One such customization involves adding classes to admin menu items, a technique that can help developers and site administrators tailor their dashboards to better meet their needs. Whether you’re looking to improve organization, enhance visibility, or simply add a touch of flair to your admin menu, understanding how to effectively add classes can transform the way you interact with your site’s backend.
When it comes to modifying the admin menu, the ability to add custom classes opens up a world of possibilities. This simple yet powerful technique allows developers to apply specific styles or behaviors to menu items, making them stand out or function differently based on user roles or preferences. By leveraging this capability, you can create a more intuitive and user-friendly admin experience, ensuring that important features are easily accessible and visually distinct.
Moreover, adding classes to admin menu items can serve as a stepping stone to more advanced customizations. It lays the groundwork for implementing JavaScript functionalities or integrating additional CSS styles, ultimately leading to a more dynamic and responsive admin interface. As we delve deeper into this topic, you’ll discover practical methods and best practices for enhancing your WordPress admin menu, empowering you to
Add Class to Admin Menu Item
To customize the appearance and behavior of admin menu items in WordPress, adding a CSS class can be an effective technique. This allows for enhanced styling or functionality through JavaScript. The process involves utilizing WordPress hooks to modify the menu items as needed.
To add a class to a specific admin menu item, you can use the `admin_menu` action hook along with the `add_menu_page` and `admin_menu` functions. Here’s a straightforward approach:
“`php
function my_custom_menu_class($menu) {
foreach ($menu as &$item) {
if ($item[0] == ‘Your Menu Title’) {
$item[1] = ‘your_custom_class’; // Adding your custom class
}
}
return $menu;
}
add_filter(‘admin_menu’, ‘my_custom_menu_class’);
“`
This code snippet effectively loops through the existing admin menu items and checks for the title of the specific menu item you want to modify. When found, it appends your custom class to that menu item.
Using JavaScript for Additional Control
In addition to adding CSS classes via PHP, you can further manipulate admin menu items using JavaScript. This is particularly useful for adding dynamic behavior or styles based on user actions.
Here’s a basic example of how to include JavaScript in your admin panel to add a class:
“`php
function custom_admin_script() {
?>
Considerations for Styling
When adding custom classes to admin menu items, it’s crucial to consider CSS specificity and how it affects the existing styles. Here are some tips:
- Ensure your custom styles are specific enough to override WordPress defaults.
- Use unique class names to avoid conflicts with other plugins or themes.
- Test across different screen sizes to ensure responsiveness.
Example CSS Styles
To illustrate how you might style your custom class, here’s a sample CSS snippet:
“`css
.your_custom_class {
background-color: 0073aa; /* Change background color */
color: white; /* Change text color */
}
.your_dynamic_class {
font-weight: bold; /* Make the text bold */
border-left: 5px solid ff0000; /* Add a left border */
}
“`
This CSS will apply specific styles to your custom class, ensuring that it stands out in the admin menu.
Common Issues and Troubleshooting
While adding classes to admin menu items is generally straightforward, you may encounter a few issues. Here are some common problems and their solutions:
Issue | Solution |
---|---|
Class not applying | Check the specificity of your CSS rules and ensure no conflicting styles exist. |
JavaScript not executing | Ensure jQuery is loaded and there are no JavaScript errors in the console. |
Menu item not found | Verify the menu title and slug are correct in your PHP code. |
By following the outlined methods, you can effectively add classes to admin menu items, enabling customized styling and functionality as needed in your WordPress admin interface.
Add Class to Admin Menu Item
To enhance the appearance or functionality of admin menu items in WordPress, you can add custom CSS classes using hooks. This technique allows for better styling options or JavaScript targeting.
Using the `admin_menu` Hook
The `admin_menu` action hook is commonly used to modify the admin menu. You can utilize this hook to add a class to a specific menu item.
“`php
add_action(‘admin_menu’, ‘customize_admin_menu’);
function customize_admin_menu() {
global $menu;
$menu[5][0] = ‘‘ . $menu[5][0] . ‘‘; // Change index according to the menu item
}
“`
In this example:
- Replace `5` with the appropriate index of the menu item you want to modify.
- The `custom-class` can be any CSS class you wish to apply.
Finding Menu Item Index
To find the correct index of the menu item, you can inspect the admin menu structure. Here’s a simple way to list menu items and their corresponding indices:
“`php
add_action(‘admin_menu’, ‘list_menu_items’);
function list_menu_items() {
global $menu;
foreach ($menu as $index => $item) {
echo ‘
' . $index . ' => ' . $item[0] . '
‘;
}
}
“`
This code will output the indices and titles of all menu items, enabling you to identify the correct index for the desired item.
Styling the Custom Class
Once the class has been added, you can apply CSS rules to style the menu item. Add your styles in the admin area by using the `admin_enqueue_scripts` hook.
“`php
add_action(‘admin_enqueue_scripts’, ‘admin_custom_styles’);
function admin_custom_styles() {
echo ‘
‘;
}
“`
This approach will ensure that your custom styles apply specifically to the targeted menu item.
Benefits of Adding Classes
Adding custom classes to admin menu items can provide several advantages:
- Enhanced Customization: Tailor the look and feel of the admin interface to align with branding.
- JavaScript Targeting: Easily select menu items for dynamic behaviors or interactions.
- Improved Usability: Highlight specific menu items for better visibility or accessibility.
Considerations
When adding classes to menu items, consider the following:
- Performance: Excessive modifications can lead to performance issues, so keep changes minimal.
- Compatibility: Ensure that the added styles do not conflict with existing CSS rules in the admin area.
- Maintainability: Document changes clearly in your codebase to facilitate future updates or troubleshooting.
By implementing these methods, you can effectively customize the WordPress admin menu to better suit your needs or preferences.
Expert Insights on Adding Classes to Admin Menu Items
Jessica Lane (WordPress Developer, CodeCraft Solutions). “Adding a custom class to an admin menu item is an effective way to enhance the user interface. By utilizing the ‘admin_menu’ action hook, developers can easily modify the menu items and apply custom styles, ensuring a more tailored experience for users.”
Michael Chen (Senior Software Engineer, Tech Innovations Inc.). “When you want to add a class to an admin menu item, it is crucial to ensure that the class is properly registered and that it does not conflict with existing styles. This can be accomplished by using the ‘add_menu_page’ function and specifying the class within the menu item parameters.”
Sarah Patel (UI/UX Designer, Creative Solutions Agency). “Customizing admin menu items with additional classes not only improves aesthetics but also enhances functionality. By applying specific classes, developers can leverage CSS to create responsive designs that adapt to different screen sizes, making the admin panel more user-friendly.”
Frequently Asked Questions (FAQs)
How can I add a custom class to an admin menu item in WordPress?
To add a custom class to an admin menu item, you can use the `admin_menu` action hook in your theme’s `functions.php` file. You can modify the menu item by utilizing the `admin_menu` action and applying the `add_menu_page` function with the desired class.
Is it possible to target specific admin menu items for class addition?
Yes, you can target specific admin menu items by checking the menu slug or ID within your custom function. Use conditional statements to apply the class only to the desired menu items.
What is the purpose of adding a class to an admin menu item?
Adding a class to an admin menu item allows for custom styling and functionality enhancements. It enables developers to apply specific CSS styles or JavaScript behaviors to particular menu items.
Can I remove a class from an existing admin menu item?
Yes, you can remove a class from an existing admin menu item by using the `admin_menu` hook. You can dequeue the styles or override the existing classes in your custom function.
Are there any plugins that facilitate adding classes to admin menu items?
Yes, several plugins are available that allow you to customize the admin menu, including adding classes to menu items. These plugins often provide user-friendly interfaces for making such modifications without coding.
What coding skills are required to add a class to an admin menu item?
Basic knowledge of PHP and familiarity with WordPress hooks and functions are required. Understanding how to edit the `functions.php` file and use the WordPress API will help in effectively adding classes to admin menu items.
In summary, adding a class to an admin menu item is a useful technique for developers looking to customize the WordPress admin interface. This process typically involves using WordPress hooks, specifically the ‘admin_menu’ action, to modify the menu items programmatically. By leveraging the ‘add_menu_page’ or ‘add_submenu_page’ functions, developers can assign custom classes to specific menu items, enhancing their visibility and functionality within the admin dashboard.
Moreover, this customization allows for improved user experience by facilitating easier navigation and organization of menu items. By applying specific classes, developers can target these items with CSS or JavaScript, enabling them to create tailored styles or behaviors that align with the overall design of the admin area. This practice not only enhances aesthetics but also contributes to a more efficient workflow for administrators and users alike.
Ultimately, understanding how to effectively add classes to admin menu items empowers developers to create a more intuitive and visually appealing WordPress admin experience. This capability is particularly beneficial for those managing complex sites with numerous admin options, as it allows for a streamlined and organized interface that can adapt to the specific needs of different users.
Author Profile
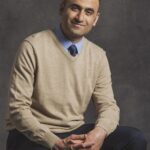
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?