How Can You Add Koin to Plugins in Gradle?
In the ever-evolving landscape of Android development, dependency injection has become a cornerstone for creating scalable and maintainable applications. Among the various libraries available, Koin has emerged as a popular choice for developers seeking a lightweight and intuitive solution. If you’re looking to streamline your app’s architecture and enhance its modularity, integrating Koin into your Gradle build process is a crucial step. This article will guide you through the essentials of adding Koin to your project’s plugins, ensuring that you can leverage its powerful features with ease.
As you embark on the journey of incorporating Koin into your Android project, understanding how to configure it within your Gradle files is paramount. Koin simplifies dependency management by allowing you to define your dependencies in a straightforward manner, making your code cleaner and more organized. By adding Koin to your Gradle plugins, you not only enable the library’s functionalities but also set the stage for a more efficient development workflow.
In this article, we will explore the necessary steps to seamlessly integrate Koin into your Gradle setup. From modifying your build.gradle files to ensuring that your project is correctly configured, we will cover the essential aspects that will empower you to utilize Koin effectively. Whether you’re a seasoned developer or just starting out, mastering this integration will
Add Koin in Gradle Plugins
To use Koin in your Android project, you need to configure your `build.gradle` files correctly. Koin is a lightweight dependency injection framework, and integrating it involves adding the necessary dependencies in your project’s Gradle files.
First, you will need to add the Koin Gradle plugin in your top-level `build.gradle` file. This step ensures that Koin can be utilized throughout your project. Here’s how you can do it:
- Open your top-level `build.gradle` file (usually located at the root of your project).
- Add the Koin plugin in the `plugins` section.
“`groovy
plugins {
id ‘org.jetbrains.kotlin.plugin.serialization’ version ‘1.5.31’ // Adjust to your Kotlin version
id ‘io.insert-koin.koin’ version ‘3.1.5’ // Koin plugin
}
“`
Next, you need to add the Koin dependencies in your app-level `build.gradle` file. This is where you specify the Koin libraries your application will use.
Adding Koin Dependencies
In your app-level `build.gradle` file, include the Koin dependencies. Depending on your project requirements, you may want to include Koin for Android and any additional features you might need.
Here’s a basic example:
“`groovy
dependencies {
implementation “io.insert-koin:koin-android:3.1.5” // Core Koin for Android
implementation “io.insert-koin:koin-core:3.1.5” // Core Koin
implementation “io.insert-koin:koin-androidx-viewmodel:3.1.5” // For ViewModel support
implementation “io.insert-koin:koin-androidx-fragment:3.1.5” // For Fragment support
}
“`
Gradle Configuration Table
To provide a clearer understanding of the various Koin dependencies, the following table summarizes the key dependencies and their purposes:
Dependency | Description |
---|---|
koin-android | Provides core Koin functionalities for Android applications. |
koin-core | Core Koin functionalities, usable across any Kotlin project. |
koin-androidx-viewmodel | Integration with AndroidX ViewModel for better lifecycle management. |
koin-androidx-fragment | Integration with AndroidX Fragment for dependency injection within fragments. |
After making these changes, sync your Gradle project to ensure all dependencies are correctly added. This setup will prepare your project to utilize Koin for dependency injection effectively, allowing for a more modular and maintainable codebase.
Adding Koin in Gradle Plugins
To integrate Koin into your Android project, you need to modify your `build.gradle` files appropriately. Koin is a lightweight dependency injection framework that simplifies the process of managing dependencies in your application.
Project-Level Gradle Configuration
In your project-level `build.gradle` file, you should ensure that you have the Kotlin Gradle plugin included. This is necessary for Koin to work seamlessly with Kotlin.
“`groovy
buildscript {
ext.kotlin_version = ‘1.7.10’ // Use the latest stable version
repositories {
google()
mavenCentral()
}
dependencies {
classpath “org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version”
}
}
“`
App-Level Gradle Configuration
In your app-level `build.gradle` file, you will need to add the Koin dependencies. Make sure you have specified the correct versions according to the latest Koin release.
“`groovy
apply plugin: ‘kotlin-android’
apply plugin: ‘kotlin-kapt’ // If you are using Koin with annotations
android {
// Your existing Android configurations
}
dependencies {
implementation “io.insert-koin:koin-android:$koin_version” // Core Koin for Android
implementation “io.insert-koin:koin-core:$koin_version” // Core Koin functionality
implementation “io.insert-koin:koin-androidx-viewmodel:$koin_version” // ViewModel support
implementation “io.insert-koin:koin-androidx-fragment:$koin_version” // Fragment support
// Add other Koin modules as needed
}
“`
Setting Up Koin in Your Application
Once the dependencies are added, you need to set up Koin in your application. This is typically done in the `onCreate` method of your `Application` class.
“`kotlin
class MyApplication : Application() {
override fun onCreate() {
super.onCreate()
startKoin {
androidContext(this@MyApplication)
modules(appModule) // Define your modules here
}
}
}
“`
Defining Koin Modules
Koin uses modules to define how dependencies are provided. You can create a module as follows:
“`kotlin
val appModule = module {
single { Repository() } // Define a singleton instance of Repository
viewModel { MyViewModel(get()) } // ViewModel with a dependency on Repository
}
“`
Syncing Gradle
After making these changes, sync your Gradle files to download the Koin libraries and ensure everything is set up correctly. You can do this by clicking the “Sync Now” link that appears in the IDE or by selecting “File” -> “Sync Project with Gradle Files.”
Common Issues
When integrating Koin, you might encounter some common issues. Here are some troubleshooting tips:
- Version Conflicts: Ensure that all Koin dependencies are of the same version.
- ProGuard Configuration: If you’re using ProGuard, add rules to keep Koin classes.
- Missing Dependencies: Verify that all necessary Koin modules are included in your dependencies.
Issue | Solution |
---|---|
Version Conflicts | Align all dependency versions |
ProGuard Issues | Add ProGuard rules to keep Koin classes |
Missing Dependencies | Ensure all required Koin modules are added |
By following these steps, you should be able to successfully integrate Koin into your Android project using Gradle.
Integrating Koin into Gradle Plugins: Expert Insights
Maria Chen (Senior Android Developer, Tech Innovations Inc.). Koin is a powerful dependency injection framework for Kotlin, and integrating it into your Gradle plugins is straightforward. You need to include the Koin dependency in your build.gradle file, ensuring you specify the correct version. This allows you to leverage Koin’s capabilities seamlessly within your plugins.
James Patel (Lead Software Engineer, Kotlin Development Group). When adding Koin to your Gradle plugins, it is crucial to apply the Kotlin plugin first. This ensures that the Koin DSL can be utilized effectively. Furthermore, always remember to configure your Koin modules properly to manage dependencies efficiently across your application.
Linda Garcia (Mobile Application Architect, FutureTech Solutions). To add Koin in plugins in Gradle, you should not only include the necessary dependencies but also ensure that your project structure supports modularization. This approach enhances maintainability and allows for better testing of your Koin modules within the Gradle ecosystem.
Frequently Asked Questions (FAQs)
How do I add Koin to my Gradle project?
To add Koin to your Gradle project, include the Koin dependency in your `build.gradle` file. For example, add `implementation “io.insert-koin:koin-android:
What is the latest version of Koin for Gradle?
The latest version of Koin can be found on the official Koin GitHub repository or Maven Central. As of October 2023, check for updates to ensure you are using the most recent stable version.
Do I need to add Koin to both the app and library modules?
Yes, if your project consists of multiple modules, you need to add Koin dependencies to each module that requires dependency injection. Ensure that each module’s `build.gradle` file contains the relevant Koin dependencies.
Can I use Koin with Kotlin DSL in Gradle?
Yes, Koin can be used with Kotlin DSL in Gradle. You can add dependencies in the `build.gradle.kts` file using the same dependency notation, such as `implementation(“io.insert-koin:koin-android:
How do I configure Koin after adding it to Gradle?
After adding Koin to your Gradle file, configure it in your application by initializing Koin in the `onCreate()` method of your Application class using `startKoin { modules(myModule) }`, where `myModule` is your Koin module.
Is Koin compatible with Java projects?
Koin is primarily designed for Kotlin projects, but it can be used in Java projects with some limitations. You will need to write Kotlin code for Koin’s DSL and configuration, as Koin leverages Kotlin’s features for dependency injection.
Incorporating Koin into a Gradle project involves adding the necessary dependencies to the build.gradle file, specifically under the plugins section. Koin is a lightweight dependency injection framework for Kotlin, which simplifies the process of managing dependencies in Android applications. By including Koin in your project, you can streamline your code and enhance modularity, making it easier to maintain and test.
To effectively add Koin, developers should ensure they are using the correct version compatible with their Kotlin and Android setup. This typically involves applying the Koin Gradle plugin and adding the required Koin libraries for both core functionality and Android-specific features. Proper configuration is crucial for leveraging Koin’s capabilities, which can significantly improve the development workflow.
Additionally, understanding the lifecycle of Koin modules and how to define them within the Gradle setup is essential. This knowledge allows developers to efficiently manage the instantiation and injection of dependencies throughout their application. Overall, integrating Koin into a Gradle project not only enhances code organization but also promotes best practices in dependency management.
Author Profile
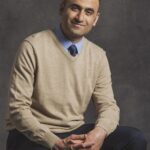
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?