Why Can’t an INSERT EXEC Statement Be Nested? Exploring the Limitations
In the realm of database management, the intricacies of SQL (Structured Query Language) can often lead to perplexing challenges, particularly when it comes to executing complex commands. One such conundrum that developers frequently encounter is the limitation that arises with the phrase: “an insert exec statement cannot be nested.” This seemingly straightforward statement can spark a cascade of questions about its implications, the underlying reasons, and the potential workarounds. As we delve into the nuances of SQL execution, we will unravel the complexities of nested commands and explore how this restriction impacts database operations, performance, and design.
When working with SQL Server, the ability to execute commands efficiently is crucial for maintaining data integrity and optimizing performance. The restriction against nesting `INSERT EXEC` statements can be a source of frustration for developers who seek to streamline their data manipulation processes. Understanding why this limitation exists is essential for anyone looking to enhance their SQL skills and avoid common pitfalls. The prohibition against nesting these commands not only affects how queries are structured but also influences how developers approach data insertion and retrieval strategies.
In this article, we will explore the rationale behind the “insert exec statement cannot be nested” limitation, examining its technical foundations and the implications for database design. We will also discuss alternative approaches and best practices that
Understanding Nested Insert Exec Statements
The error message “an insert exec statement cannot be nested” typically occurs in SQL Server when attempting to execute a nested `INSERT EXEC` statement. This situation arises when a stored procedure that contains an `INSERT EXEC` command is called from another stored procedure that also contains an `INSERT EXEC` command. SQL Server does not allow this kind of nesting, which can lead to confusion and unintended behavior in database operations.
To better understand this limitation, let’s explore how the `INSERT EXEC` statement functions and the implications of nesting.
Mechanics of Insert Exec Statements
The `INSERT EXEC` statement is used to insert the result set of a stored procedure into a table. The syntax typically looks like this:
“`sql
INSERT INTO target_table
EXEC stored_procedure_name @parameter1, @parameter2;
“`
This statement executes the specified stored procedure and inserts its output directly into the `target_table`. However, when trying to nest this command within another `INSERT EXEC`, SQL Server raises an error.
Common Scenarios Leading to the Error
Understanding the scenarios that can lead to this error can help in troubleshooting and avoiding it:
- Calling a Stored Procedure that Executes Another Insert Exec: If `Procedure A` calls `Procedure B`, and both use `INSERT EXEC`, the error will occur.
- Triggers Involving Insert Exec: If a trigger on a table attempts to execute a stored procedure with an `INSERT EXEC`, while the parent context is already executing an `INSERT EXEC`, the error will be raised.
Workarounds for Nested Insert Exec Limitations
There are several strategies to work around the restriction against nested `INSERT EXEC` statements:
- Use Temporary Tables: Store the results of the first `INSERT EXEC` in a temporary table and then use that table for further processing.
“`sql
CREATE TABLE TempTable (Column1 INT, Column2 NVARCHAR(100));
INSERT INTO TempTable
EXEC stored_procedure_name;
INSERT INTO target_table
SELECT * FROM TempTable;
“`
- Refactor Procedures: Modify the design of your stored procedures to avoid nested `INSERT EXEC` calls. This might involve combining procedures or breaking them into simpler components.
- Use Output Parameters: Instead of inserting directly, consider using output parameters to return data from one procedure to another.
Error Handling and Considerations
When dealing with this error, it is essential to implement proper error handling and logging mechanisms. This ensures that when the error occurs, you can trace back to the source and understand the context. Here’s a simple error handling structure:
“`sql
BEGIN TRY
— Your insert exec logic here
END TRY
BEGIN CATCH
SELECT ERROR_MESSAGE() AS ErrorMessage;
END CATCH;
“`
Scenario | Possible Solution |
---|---|
Nesting Insert Exec in Procedures | Use temporary tables to hold results |
Triggers with Insert Exec | Refactor logic to avoid nesting |
Complex Data Manipulation | Break down procedures into simpler parts |
By understanding the limitations and employing effective strategies, developers can navigate around the constraints imposed by SQL Server regarding nested `INSERT EXEC` statements, ensuring robust and error-free database operations.
Understanding Nested INSERT EXEC Statements
The error message “an insert exec statement cannot be nested” typically arises when trying to execute an `INSERT EXEC` statement within another `INSERT EXEC` statement. This situation is crucial to understand, especially for developers and database administrators who work with SQL Server.
How INSERT EXEC Works
The `INSERT EXEC` statement is used to insert the result set of a stored procedure into a table. The basic syntax is as follows:
“`sql
INSERT INTO target_table
EXEC stored_procedure_name;
“`
This command fetches data produced by the stored procedure and inserts it into the specified target table. However, SQL Server restricts nesting of these operations.
Why Nesting is Not Allowed
Nesting `INSERT EXEC` statements is not permitted due to the following reasons:
- Transaction Control: SQL Server manages transactions differently for nested executions, which can lead to complex behaviors and potential data inconsistencies.
- Resource Management: Executing multiple instances of `INSERT EXEC` can lead to increased resource consumption and performance degradation.
- Error Handling: Managing errors and rollbacks becomes complicated with nested operations, increasing the likelihood of unintended consequences.
Common Scenarios Leading to the Error
Developers may encounter this error in various situations, including:
- Attempting to insert results from one stored procedure that calls another stored procedure using `INSERT EXEC`.
- Using triggers that execute stored procedures returning result sets into another `INSERT EXEC`.
- Creating complex views or functions that internally reference other procedures leading to nested execution attempts.
Best Practices to Avoid the Error
To prevent encountering this error, consider the following strategies:
- Refactor Procedures: Instead of nesting `INSERT EXEC`, restructure stored procedures to return data directly without needing another `INSERT EXEC`.
- Use Temporary Tables: Store the results of the first `INSERT EXEC` into a temporary table before running subsequent queries.
Example:
“`sql
CREATE TABLE TempTable (Column1 INT, Column2 VARCHAR(100));
INSERT INTO TempTable
EXEC FirstStoredProcedure;
INSERT INTO SecondTable
SELECT * FROM TempTable;
“`
- Simplify Logic: Analyze the logic flow of your stored procedures to minimize the need for nesting.
Example of a Correct Approach
Here is an example demonstrating how to avoid nesting with temporary tables:
“`sql
CREATE TABLE Results (ID INT, Name VARCHAR(100));
— First EXEC to populate the temporary table
INSERT INTO Results
EXEC GetEmployeeData;
— Second EXEC using the results without nesting
INSERT INTO EmployeesArchive
SELECT * FROM Results;
DROP TABLE Results; — Clean up temporary table
“`
This approach ensures that data is effectively managed without violating SQL Server’s constraints regarding nested `INSERT EXEC` statements. By maintaining clarity and control over the execution flow, developers can enhance performance and reliability.
Understanding the Limitations of Nested Insert Exec Statements
Dr. Emily Carter (Database Systems Analyst, Tech Innovations Inc.). “The restriction on nesting `INSERT EXEC` statements is primarily due to the way SQL Server manages transaction scopes and execution contexts. Allowing such nesting could lead to complex transaction management issues and potential data integrity problems.”
Michael Chen (Senior SQL Developer, Data Solutions Group). “When working with `INSERT EXEC`, it’s crucial to understand that SQL Server does not permit nesting because of its design to maintain performance and avoid deadlocks. This limitation encourages developers to think critically about their data manipulation strategies.”
Linda Patel (Lead Database Architect, Enterprise Data Management). “The inability to nest `INSERT EXEC` statements is a deliberate design choice in SQL Server. It simplifies the execution plan and ensures that the server does not encounter unexpected behavior during complex queries, ultimately leading to a more stable environment.”
Frequently Asked Questions (FAQs)
What does the error “an insert exec statement cannot be nested” mean?
This error occurs when an `INSERT EXEC` statement is attempted within another `INSERT EXEC` statement or within a transaction that is already executing an `INSERT EXEC`. SQL Server does not support nesting these types of commands.
Why can’t I use an `INSERT EXEC` statement inside a stored procedure that already has an `INSERT EXEC`?
SQL Server restricts the nesting of `INSERT EXEC` statements to prevent conflicts and ensure data integrity. When one `INSERT EXEC` is running, the system locks the resources, making it impossible to initiate another.
How can I resolve the “an insert exec statement cannot be nested” error?
To resolve this error, you can refactor your code to avoid nesting `INSERT EXEC` statements. Consider using temporary tables or table variables to store intermediate results before performing the final `INSERT`.
Are there any alternatives to `INSERT EXEC` that allow for similar functionality?
Yes, alternatives include using temporary tables or table variables to capture the results of the executed stored procedure first, then performing an `INSERT` from those tables into the target table.
Can this error occur in transactions?
Yes, this error can occur within transactions if an `INSERT EXEC` is attempted while another `INSERT EXEC` is already in progress. It is advisable to manage transactions carefully to avoid this situation.
What are the best practices to avoid encountering this error?
Best practices include avoiding nested `INSERT EXEC` statements, utilizing temporary tables, and ensuring that stored procedures are designed to handle data without requiring nested execution. Always test procedures in a controlled environment before deployment.
The error message “an insert exec statement cannot be nested” typically arises in SQL Server when a user attempts to execute an `INSERT EXEC` statement within another `INSERT EXEC` statement. This restriction is in place because SQL Server does not allow the execution of stored procedures that return a result set to be nested within another execution context that also expects a result set. Such limitations are crucial for maintaining the integrity of transactions and ensuring that the database engine can manage resources effectively.
One key takeaway from this discussion is the importance of understanding SQL Server’s execution model. When designing database operations, it’s essential to recognize that certain commands, like `INSERT EXEC`, have specific constraints that can affect how queries are structured. This understanding can help database developers avoid common pitfalls and design more efficient and effective queries that adhere to SQL Server’s operational guidelines.
Additionally, developers should consider alternative approaches when they encounter this limitation. For instance, breaking down complex operations into separate steps or using temporary tables to store intermediate results can be effective strategies. By doing so, developers can circumvent the nesting issue while still achieving the desired outcomes in their database interactions.
Author Profile
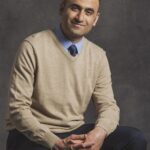
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?