Are There Virtual Functions in Python? Exploring Object-Oriented Concepts
In the world of object-oriented programming, the concept of virtual functions plays a pivotal role in enabling polymorphism, allowing developers to create flexible and dynamic code. While many programming languages, such as C++ and Java, have explicit support for virtual functions, Python takes a different approach. This raises an intriguing question: Are there virtual functions in Python? As we delve into the nuances of Python’s object-oriented capabilities, we will uncover how this versatile language handles method overriding and dynamic dispatch, offering a unique perspective on the traditional concept of virtual functions.
Python, known for its simplicity and readability, embraces a more fluid interpretation of object-oriented principles. Unlike statically typed languages that require explicit declarations for virtual functions, Python’s dynamic nature allows methods to be overridden effortlessly in subclasses. This means that any method defined in a base class can be replaced by a subclass, enabling a form of polymorphism that is inherently built into the language. The absence of a formal “virtual” keyword does not diminish Python’s ability to facilitate dynamic behavior; instead, it showcases the language’s design philosophy that prioritizes flexibility and ease of use.
As we explore the intricacies of Python’s method resolution order and the mechanisms that underpin its object-oriented features, we will reveal how developers can achieve the same outcomes as traditional
Understanding Virtual Functions in Python
In Python, the concept of virtual functions is not explicitly defined as it is in languages like C++ or Java. However, Python achieves similar functionality through its dynamic typing and method resolution order. This allows for methods to be overridden in subclasses, enabling polymorphism—a key feature associated with virtual functions.
Method Overriding
Method overriding occurs when a subclass provides a specific implementation of a method that is already defined in its parent class. This allows the subclass to tailor the method’s behavior to its own requirements. In Python, any method can be overridden, which closely aligns with the behavior of virtual functions.
- Parent Class Example:
python
class Animal:
def sound(self):
return “Some sound”
- Child Class Example:
python
class Dog(Animal):
def sound(self):
return “Bark”
In this example, the `Dog` class overrides the `sound` method of the `Animal` class. When the `sound` method is called on an instance of `Dog`, it returns “Bark” instead of “Some sound”.
Polymorphism in Python
Polymorphism allows for methods to be called on objects of different classes, provided they implement the same method name. This is a cornerstone of object-oriented programming and is facilitated in Python through duck typing.
- Example of Polymorphism:
python
def make_sound(animal):
print(animal.sound())
dog = Dog()
make_sound(dog) # Output: Bark
cat = Animal()
print(make_sound(cat)) # Output: Some sound
In this case, the `make_sound` function can accept any object that has a `sound` method, showcasing polymorphism.
Abstract Base Classes
To formalize the concept of virtual functions, Python provides the `abc` module, which allows developers to define Abstract Base Classes (ABCs). ABCs can define methods that must be created within any subclass, effectively acting as a contract.
- Defining an Abstract Base Class:
python
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def sound(self):
pass
Here, the `Animal` class defines an abstract method `sound`, which must be implemented by any subclass:
- Implementation of ABC:
python
class Dog(Animal):
def sound(self):
return “Bark”
class Cat(Animal):
def sound(self):
return “Meow”
Both `Dog` and `Cat` must implement the `sound` method, ensuring they conform to the interface defined by the `Animal` class.
Class Name | Sound |
---|---|
Dog | Bark |
Cat | Meow |
Virtual Functions
While Python does not have virtual functions in the traditional sense, its method overriding and polymorphism capabilities, along with the use of abstract base classes, provide similar functionality. Developers can leverage these features to create flexible and maintainable code that adheres to the principles of object-oriented programming.
Understanding Virtual Functions in Python
In Python, the concept of virtual functions is approached differently compared to languages like C++ or Java. While Python does not have a formal declaration for virtual functions, it inherently supports polymorphism through its class and method structures.
What Are Virtual Functions?
Virtual functions are typically defined in a base class and can be overridden in derived classes. The core characteristic of virtual functions is that they allow for dynamic dispatch, meaning the method that gets executed is determined at runtime based on the object’s class, rather than the reference type.
### Key Characteristics
- Dynamic Binding: The method to call is determined at runtime.
- Overriding: Subclasses can provide specific implementations of methods defined in the base class.
- Polymorphism: Objects of different classes can be treated as objects of a common superclass.
Implementing Virtual Functions in Python
In Python, all instance methods are virtual by default. This means that you can override them in subclasses without any special syntax. Here’s how you can implement this concept:
python
class Animal:
def speak(self):
return “Animal speaks”
class Dog(Animal):
def speak(self):
return “Bark”
class Cat(Animal):
def speak(self):
return “Meow”
# Example usage
def animal_sound(animal):
print(animal.speak())
animal_sound(Dog()) # Outputs: Bark
animal_sound(Cat()) # Outputs: Meow
In this example, the `speak` method behaves as a virtual function. The appropriate method is determined at runtime based on the actual object type, not the reference type.
Using Abstract Base Classes
For more structured implementations resembling formal virtual functions, Python provides the `abc` module, which allows the definition of abstract base classes (ABCs). An abstract method in an ABC must be overridden in any derived class.
### Example of Abstract Base Class
python
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * (self.radius ** 2)
class Square(Shape):
def __init__(self, side):
self.side = side
def area(self):
return self.side ** 2
# Example usage
shapes = [Circle(5), Square(4)]
for shape in shapes:
print(shape.area())
### Benefits of Using Abstract Base Classes
- Enforcement: Ensures that derived classes implement specific methods.
- Clarity: Provides a clear interface for subclasses.
- Polymorphism: Facilitates the use of different subclasses interchangeably.
Virtual Functions in Python
Although Python does not have virtual functions in the traditional sense, it supports polymorphism and method overriding through its class system. By leveraging abstract base classes, developers can create a more structured approach to defining and enforcing method implementations across subclasses.
Understanding Virtual Functions in Python: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Python Development Institute). Virtual functions, as commonly understood in languages like C++ or Java, do not exist in Python in the same way. However, Python achieves polymorphism through its dynamic typing and method overriding, allowing for similar behavior without the explicit declaration of virtual functions.
Mark Thompson (Lead Python Developer, Tech Innovations Corp). While Python does not have a formal concept of virtual functions, the use of abstract base classes (ABCs) provides a way to define methods that must be implemented in derived classes. This mimics the behavior of virtual functions found in statically typed languages.
Linda Zhao (Professor of Computer Science, University of Technology). The flexibility of Python’s object-oriented programming allows developers to implement polymorphic behavior through duck typing, which means that the suitability of an object is determined by the presence of certain methods and properties, rather than the object’s type itself. This approach effectively replaces the need for traditional virtual functions.
Frequently Asked Questions (FAQs)
Are there virtual functions in Python?
Python does not have virtual functions in the same way as C++ or Java. However, it supports polymorphism through method overriding in subclasses, which allows similar behavior.
How does method overriding work in Python?
Method overriding occurs when a subclass provides a specific implementation of a method that is already defined in its superclass. This allows the subclass to customize or extend the behavior of the inherited method.
Can you achieve polymorphism in Python?
Yes, polymorphism is achieved in Python through method overriding and duck typing. Objects can be treated as instances of their parent class, allowing for flexible and interchangeable code.
What is the difference between abstract methods and virtual methods in Python?
Abstract methods are defined in abstract base classes and must be implemented in derived classes. Virtual methods, while not explicitly defined in Python, refer to methods that can be overridden in subclasses to provide specific behavior.
Do I need to use an interface to implement virtual-like behavior in Python?
While Python does not require interfaces, you can use abstract base classes from the `abc` module to define methods that must be implemented in subclasses, thus simulating interface behavior.
How can I define an abstract class in Python?
To define an abstract class in Python, import the `ABC` class and the `abstractmethod` decorator from the `abc` module. Use the decorator to mark methods as abstract, enforcing their implementation in subclasses.
In Python, the concept of virtual functions as seen in languages like C++ or Java does not exist in the same form. However, Python achieves similar functionality through its use of polymorphism and dynamic typing, which allows methods to be overridden in subclasses. This flexibility enables developers to define methods in a base class and override them in derived classes, effectively mimicking the behavior of virtual functions.
Moreover, Python’s approach to object-oriented programming emphasizes the use of duck typing, where the suitability of an object is determined by the presence of certain methods and properties rather than the object’s type itself. This means that any object can be treated as a virtual function as long as it implements the required methods, thus promoting a highly flexible and dynamic coding style.
In summary, while Python does not have virtual functions in the traditional sense, it offers powerful features that allow for similar behavior through method overriding and polymorphism. This design choice aligns with Python’s philosophy of simplicity and readability, making it easier for developers to implement and maintain code.
Key takeaways include the understanding that Python’s dynamic nature enables effective polymorphism, allowing for method overriding without the need for explicit virtual function declarations. Additionally, the use of duck typing enhances the language’s
Author Profile
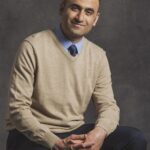
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?