How Can I Retrieve the Job Pod Name Using the Argo RESTful API?
In the rapidly evolving landscape of cloud-native applications, managing workflows efficiently is paramount. Argo, a powerful open-source container-native workflow engine, has emerged as a go-to solution for orchestrating complex jobs in Kubernetes environments. As organizations increasingly adopt microservices architectures, the need for seamless integration and automation becomes critical. One of the essential tasks in this ecosystem is retrieving job pod names through the Argo RESTful API, a process that can significantly enhance monitoring, debugging, and operational efficiency.
Understanding how to leverage the Argo RESTful API to fetch job pod names opens up a realm of possibilities for developers and DevOps teams. This capability not only streamlines the management of Kubernetes jobs but also provides insights into the status and performance of running applications. By utilizing the API, users can automate workflows, retrieve real-time data, and gain a clearer picture of resource utilization, ultimately driving better decision-making and enhancing overall productivity.
As we delve deeper into the intricacies of the Argo RESTful API, we will explore the various endpoints and methods available for retrieving job pod names. Whether you’re a seasoned Kubernetes user or just starting your journey into container orchestration, understanding these concepts will empower you to harness the full potential of Argo, ensuring your workflows are not only efficient but also resilient
Accessing Job Pod Names via the Argo RESTful API
To retrieve the pod names associated with a specific Argo workflow job, you can utilize the Argo RESTful API. This API provides a comprehensive interface for interacting with Argo workflows, allowing you to access various details, including job information.
The process typically involves the following steps:
- Identify the Workflow: You need the name of the workflow for which you want to retrieve the job pod names. This can be obtained through the API by listing all workflows or by querying a specific workflow.
- Retrieve Job Details: Once you have the workflow name, you can fetch the details of the jobs associated with that workflow. Each job will contain information about the pod that is executing it.
- Extract Pod Names: From the job details, extract the pod names to use them for monitoring, debugging, or other purposes.
Here’s a simple outline of how to achieve this:
- Use the endpoint to list workflows:
“`
GET /api/v1/workflows/{namespace}
“`
- For a specific workflow, get job details:
“`
GET /api/v1/workflows/{namespace}/{workflowName}/jobs
“`
- Each job in the response will include the pod name.
Sample API Calls
The following examples illustrate how to use the API to get job pod names.
List Workflows
To list all workflows in a specific namespace:
“`bash
curl -X GET “http://
-H “Authorization: Bearer
“`
Get Workflow Jobs
To retrieve jobs for a specific workflow:
“`bash
curl -X GET “http://
-H “Authorization: Bearer
“`
These commands will return JSON responses containing all relevant information, including job and pod details.
Understanding the JSON Response
The response from the API calls will contain data structured in JSON format. Below is a simplified representation of a typical response when querying workflow jobs:
Field | Description |
---|---|
name | The name of the job |
status | The current status of the job (e.g., Running, Succeeded, Failed) |
podName | The name of the pod executing the job |
namespace | The Kubernetes namespace where the job is running |
From this response, you can extract the `podName` field to get the names of the pods running each job. This information is crucial for troubleshooting and understanding the state of your workflows.
Remember to handle any errors and validate the responses to ensure that you are working with the correct data. By following these steps, you can efficiently retrieve job pod names using the Argo RESTful API.
Using Argo RESTful API to Retrieve Job Pod Names
To obtain the pod names associated with a specific job in Argo Workflows, you can utilize the Argo RESTful API. This process involves sending an HTTP GET request to the appropriate endpoint, which provides detailed information about the job, including the associated pods.
Identifying the API Endpoint
The primary endpoint for querying job details in Argo is structured as follows:
“`
GET /api/v1/workflows/{namespace}/{name}
“`
- {namespace}: The Kubernetes namespace where the workflow is located.
- {name}: The name of the workflow/job.
Request Example
To retrieve the job details, you can use a command-line tool like `curl`:
“`bash
curl -X GET “http://
“`
Replace `
Understanding the Response
The response from the API will be in JSON format and includes various details about the job. Key fields to look for include:
- status: Provides the current status of the workflow (e.g., Running, Succeeded, Failed).
- templateName: The name of the template executed for the job.
- status.nodes: Contains a list of nodes associated with the workflow, where each node corresponds to a pod.
Extracting Pod Names
Within the response JSON, you can find pod names by navigating to the `status.nodes` section. Each node entry will typically include the following relevant fields:
Field | Description |
---|---|
id | Unique identifier for the node. |
name | The name of the pod associated with the node. |
type | Type of the node (e.g., Pod, Steps). |
phase | Current phase of the node (e.g., Running, Succeeded). |
To extract pod names, look for the `name` field within each node:
“`json
{
“status”: {
“nodes”: {
“node-1”: {
“name”: “job-pod-name-1”,
“type”: “Pod”,
“phase”: “Succeeded”
},
“node-2”: {
“name”: “job-pod-name-2”,
“type”: “Pod”,
“phase”: “Running”
}
}
}
}
“`
In this example, you can see two pod names: `job-pod-name-1` and `job-pod-name-2`.
Automating the Process
To automate the retrieval of pod names, you can write a script that performs the API call and parses the JSON response. Here is an example using Python with the `requests` library:
“`python
import requests
def get_job_pod_names(namespace, job_name, argo_server):
url = f”http://{argo_server}/api/v1/workflows/{namespace}/{job_name}”
response = requests.get(url)
if response.status_code == 200:
workflow = response.json()
pod_names = [node[‘name’] for node in workflow[‘status’][‘nodes’].values() if node[‘type’] == ‘Pod’]
return pod_names
else:
raise Exception(“Error fetching workflow details”)
Example usage
pod_names = get_job_pod_names(“default”, “example-job”, “argo-server.example.com”)
print(pod_names)
“`
This script will return a list of pod names associated with the specified job.
Using the Argo RESTful API to get job pod names is straightforward once you understand the endpoint structure and the JSON response format. By automating this process, you can efficiently manage and monitor your workflows in Argo.
Expert Insights on Accessing Job Pod Names via Argo RESTful API
Dr. Emily Chen (Cloud Native Architect, Kubernetes Solutions Inc.). “To effectively retrieve job pod names using the Argo RESTful API, one must utilize the appropriate endpoint that corresponds to the specific workflow. The API provides a straightforward mechanism to query the status of jobs, and by parsing the JSON response, you can extract the pod names associated with each job.”
Mark Thompson (DevOps Engineer, Continuous Delivery Group). “When working with the Argo RESTful API, it is crucial to familiarize yourself with the API’s authentication and authorization mechanisms. Properly configured permissions will ensure that you can access the job details, including pod names, without running into access issues.”
Lisa Patel (Senior Software Developer, CloudOps Technologies). “Utilizing the Argo RESTful API to get job pod names can significantly enhance your CI/CD pipeline’s efficiency. By automating this retrieval process, teams can monitor job statuses in real-time, allowing for quicker troubleshooting and resource management.”
Frequently Asked Questions (FAQs)
How can I retrieve the pod name of a specific Argo job using the RESTful API?
To retrieve the pod name of a specific Argo job, you can use the Argo RESTful API endpoint for jobs. Send a GET request to `/api/v1/workflows/{namespace}/{workflowName}` and parse the response to extract the pod name from the `status` section.
What information is included in the response when querying an Argo job?
The response includes detailed information about the job, such as its status, start time, end time, and the names of the pods associated with the job. You can find the pod names under the `status.nodes` field.
Is authentication required to access the Argo RESTful API for job details?
Yes, authentication is typically required to access the Argo RESTful API. You may need to provide a bearer token or other authentication methods, depending on your cluster’s configuration and security settings.
Can I filter the job results when using the Argo RESTful API?
Yes, you can filter job results by specifying query parameters in your GET request, such as job name, status, or namespace. This allows you to retrieve only the relevant jobs based on your criteria.
What should I do if the pod name is not present in the job details?
If the pod name is not present, it may indicate that the job has not yet started or has failed to create the pod. Check the job’s status and events for any errors that may have occurred during execution.
Are there any limitations on the number of jobs I can query using the Argo RESTful API?
There are no strict limitations on the number of jobs you can query, but performance may vary based on the size of your cluster and the number of jobs. It is advisable to implement pagination or filtering to manage large datasets effectively.
The Argo Workflows RESTful API provides a powerful interface for interacting with workflows and jobs within a Kubernetes environment. One of the common tasks users may need to perform is retrieving the pod name associated with a specific job. This can be crucial for monitoring, debugging, and managing resources effectively. The API offers endpoints that allow users to query job details, including the pod name, which is essential for tracking the execution of tasks and understanding the state of the workflow.
To get the pod name of a job, users typically need to make a GET request to the appropriate endpoint, often structured around the job’s unique identifier. The response from this API call includes detailed information about the job, including the status and the associated pod name. This functionality underscores the importance of having a clear understanding of the API’s structure and the specific parameters required to retrieve the desired information.
In summary, leveraging the Argo RESTful API to obtain job pod names enhances operational efficiency within Kubernetes-managed workflows. By utilizing the API effectively, users can streamline their workflow management processes, improve troubleshooting capabilities, and maintain better oversight of their job executions. Understanding how to interact with the API is a valuable skill for developers and operators working in cloud-native environments.
Author Profile
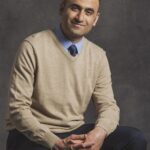
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?