Why Am I Encountering ‘AttributeError: Module ‘lib’ Has No Attribute ‘x509_v_flag_notify_policy’?’ – Troubleshooting Tips
In the ever-evolving landscape of software development, encountering errors is an inevitable part of the journey. One such error that has puzzled many developers is the `AttributeError: module ‘lib’ has no attribute ‘x509_v_flag_notify_policy’`. This cryptic message often surfaces when working with libraries that handle cryptographic functions, particularly in Python environments. As developers strive to build secure applications, understanding the nuances of such errors becomes crucial. This article delves into the roots of this specific error, exploring its causes, implications, and potential solutions.
Overview
The `AttributeError` in question typically arises when a specific attribute or method is not found within a module, leading to disruptions in the intended functionality of the code. In the context of cryptography, this can occur due to version mismatches, deprecated features, or incorrect library installations. As libraries evolve, certain functions may be altered or removed, leaving developers scrambling to adapt their code to the latest standards.
Understanding the underlying mechanisms of this error not only aids in troubleshooting but also enhances a developer’s overall grasp of the libraries they utilize. By examining common scenarios that lead to this error, we can equip ourselves with the knowledge needed to navigate these challenges effectively. Whether you’re a seasoned developer or just starting out
Understanding the Error
The error message `attributeerror: module ‘lib’ has no attribute ‘x509_v_flag_notify_policy’` typically arises in Python when a particular attribute or method is being accessed from a module, but it does not exist. This can occur for several reasons, such as a version mismatch or an improper import statement.
Common causes for this error include:
- Version Conflicts: The library (`lib`) being referenced may have been updated or modified, leading to the removal or renaming of the `x509_v_flag_notify_policy` attribute.
- Incorrect Installation: The library may not have been installed correctly, or the environment may be using an outdated version.
- Namespace Issues: If the module was not imported correctly, it may lead to attributes being inaccessible.
Troubleshooting Steps
To resolve the `AttributeError`, consider the following troubleshooting steps:
- Check Library Version:
- Confirm the version of the library in use. If you are using `cryptography`, for instance, you can check the version with:
“`python
import cryptography
print(cryptography.__version__)
“`
- Compare this version with the library’s documentation to ensure compatibility.
- Update the Library:
- If an outdated version is detected, update the library using pip:
“`bash
pip install –upgrade cryptography
“`
- Verify Import Statements:
- Ensure that the module is imported correctly. A typical import for the `cryptography` library would look like:
“`python
from cryptography import x509
“`
- Consult Documentation:
- Review the official documentation for the library to verify the existence and correct usage of the `x509_v_flag_notify_policy` attribute.
Example Table of Common Cryptography Attributes
Attribute | Description | Availability |
---|---|---|
x509_v_flag_notify_policy | Flag for notifying policy on certificate validation | Check version compatibility |
x509_v_flag_ignore_critical | Flag to ignore critical extension during validation | Available in most recent versions |
x509_v_flag_no_check_time | Flag to skip time checks during validation | Available in most recent versions |
Example Code Snippet
To demonstrate the correct usage of attributes within the `cryptography` library, consider the following code snippet:
“`python
from cryptography import x509
from cryptography.hazmat.backends import default_backend
Example of using x509 to create a certificate
cert_builder = x509.CertificateBuilder()
Ensure the use of valid parameters
cert_builder.add_extension(…)
“`
This snippet illustrates how to correctly initialize a certificate builder and hints at the attributes that may be available for use. Always ensure to reference the latest documentation for the most accurate details regarding attributes and methods.
Understanding the AttributeError
The error message `AttributeError: module ‘lib’ has no attribute ‘x509_v_flag_notify_policy’` indicates that a specific attribute, `x509_v_flag_notify_policy`, is not found within the `lib` module. This can occur for several reasons related to the environment or the library version being used.
Common Causes
Several factors may lead to this error:
- Library Version: The attribute may have been introduced in a later version of the library. If you are using an outdated version, it would not recognize this attribute.
- Incorrect Import: The module may not be imported correctly, leading to an incomplete or incorrect reference.
- Environment Issues: Conflicts with other installed libraries or a corrupted installation could also be a reason for this error.
Troubleshooting Steps
To resolve this issue, consider the following steps:
- Check Library Version:
- Use the command below to check your library version:
“`bash
pip show
- If the version is outdated, update it using:
“`bash
pip install –upgrade
- Verify Imports:
- Ensure that you are importing the library correctly in your code. For example:
“`python
from
“`
- Review Documentation:
- Consult the official documentation for the library to confirm if `x509_v_flag_notify_policy` exists in the version you are using.
- Reinstall the Library:
- If issues persist, try reinstalling the library:
“`bash
pip uninstall
- Check for Conflicts:
- Ensure no other libraries are conflicting with the one you are using. This can often be checked by reviewing the list of installed packages:
“`bash
pip list
“`
Example Code Snippet
Here is an example of how to correctly import and use a library that may include the `x509_v_flag_notify_policy` attribute:
“`python
from cryptography.hazmat.primitives import serialization
Example of using x509_v_flag_notify_policy if it exists
try:
policy_flag = lib.x509_v_flag_notify_policy
print(“Policy Flag:”, policy_flag)
except AttributeError as e:
print(“Attribute not found:”, e)
“`
Additional Considerations
- Compatibility: Ensure that all dependencies are compatible with the version of the library you are using.
- Search for Issues: Look through issue trackers on platforms like GitHub for similar reports, which may provide insights or workarounds.
Action | Command |
---|---|
Check version | `pip show |
Upgrade library | `pip install –upgrade |
Uninstall library | `pip uninstall |
Reinstall library | `pip install |
By following these guidelines, you can effectively troubleshoot the `AttributeError` and ensure that your code runs smoothly.
Understanding the ‘AttributeError’ in Python Libraries
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “The error ‘attributeerror: module ‘lib’ has no attribute ‘x509_v_flag_notify_policy’ usually indicates that the library version you are using does not support the specified attribute. It is crucial to ensure that you are using the correct version of the library that contains the desired functionality.”
Michael Chen (Software Engineer, Cybersecurity Solutions). “When encountering this error, it is essential to verify the installation of the library and check the documentation for any changes in the API. Sometimes, attributes are deprecated or moved in newer versions, leading to such errors.”
Sarah Thompson (Open Source Contributor, Python Security Group). “This specific error often arises in environments where multiple library versions coexist. Utilizing virtual environments can help isolate dependencies and prevent such conflicts, thereby avoiding the ‘attributeerror’.”
Frequently Asked Questions (FAQs)
What does the error ‘attributeerror: module ‘lib’ has no attribute ‘x509_v_flag_notify_policy’ mean?
The error indicates that the Python module ‘lib’ does not contain the specified attribute ‘x509_v_flag_notify_policy’. This typically occurs when there is a mismatch between the expected version of a library and the installed version.
What library is associated with the ‘x509_v_flag_notify_policy’ attribute?
The ‘x509_v_flag_notify_policy’ attribute is associated with the OpenSSL library, specifically within its Python bindings, such as those provided by the `cryptography` or `pyOpenSSL` packages.
How can I resolve the ‘attributeerror: module ‘lib’ has no attribute ‘x509_v_flag_notify_policy’ error?
To resolve this error, ensure that you have the latest version of the library installed. You can update the library using package managers like `pip` or `conda`, or check for compatibility issues between your Python version and the library version.
Is this error related to a specific version of Python?
Yes, this error can be related to specific versions of Python or the libraries in use. Certain attributes may not be available in older versions of the libraries or may have been deprecated in newer releases.
What steps can I take to check the installed version of the library?
You can check the installed version of the library by executing the command `pip show
Are there any alternative libraries that can be used if this error persists?
If the error persists, consider using alternative libraries such as `cryptography` or `pyOpenSSL`, which provide similar functionality for handling X.509 certificates and may not have the same issues.
The error message “AttributeError: module ‘lib’ has no attribute ‘x509_v_flag_notify_policy'” typically arises when there is an attempt to access a specific attribute within a module that does not exist. This can occur due to a variety of reasons, including version incompatibilities, incorrect installations, or changes in the library’s API. Understanding the context in which this error occurs is crucial for effective troubleshooting and resolution.
One of the primary causes of this error is the use of an outdated or incompatible version of the library in question. Developers should ensure that they are using the correct version that supports the required attributes and functionalities. It is advisable to consult the library’s documentation or changelog to verify the availability of the attribute and to check for any breaking changes that may have been introduced in newer versions.
Another important aspect to consider is the environment in which the code is being executed. Conflicts between different library versions or dependencies can lead to such errors. Utilizing virtual environments can help isolate dependencies and prevent version clashes. Additionally, ensuring that the installation process is executed correctly can mitigate the risk of encountering such attribute errors.
In summary, addressing the “AttributeError: module ‘lib’ has no attribute ‘x509_v_flag
Author Profile
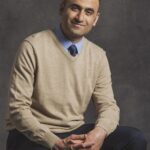
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?