Why Am I Getting ‘Bad Operand Types for Binary Operator ‘?’ Error in My Code?
In the world of programming, few things can be as frustrating as encountering cryptic error messages that halt your progress. One such message that often leaves developers scratching their heads is the infamous “bad operand types for binary operator ‘?'” error. This seemingly innocuous phrase can signal a variety of underlying issues, particularly in languages that leverage conditional operators or ternary expressions. Understanding the nuances of this error not only helps in troubleshooting but also enhances your overall coding prowess.
When you encounter the “bad operand types for binary operator ‘?'” error, it typically indicates a mismatch in data types within a conditional expression. This can occur in several programming languages, where the ternary operator is used to streamline conditional logic. The error serves as a reminder of the importance of type compatibility and the need to ensure that operands are correctly aligned for the operations you intend to perform. As you delve deeper into the intricacies of this issue, you’ll uncover common pitfalls and best practices that can help you write cleaner, more robust code.
Moreover, this error highlights a broader theme in software development: the critical role of type systems in preventing runtime errors and enhancing code reliability. By exploring the causes and solutions to this error, you not only gain insight into your specific coding challenges but also develop a more profound understanding
Understanding the Error Message
The error message “bad operand types for binary operator” typically indicates that an operation is being attempted between two incompatible types. This occurs in programming languages that enforce type checking, such as Java or C. When the compiler encounters an operation involving two operands that cannot be logically combined, it raises this error.
Common scenarios leading to this error include:
- Attempting to perform arithmetic operations on non-numeric types (e.g., adding a string to an integer).
- Using comparison operators on incompatible types (e.g., comparing an object with a primitive type).
- Misusing operators that are not defined for certain data types.
Common Causes of the Error
Understanding the specific causes of this error can help in debugging and resolving issues in code. Here are some common causes:
- Mismatched Data Types: When operands are of different types that do not support the operation.
- Type Casting Issues: Failing to properly cast one of the operands to a compatible type.
- Operator Overloading: In languages that support operator overloading, an operator may not be defined for the given types.
To illustrate, consider the following table that summarizes possible operand types and their compatibility with common operators:
Operator | Operand Type 1 | Operand Type 2 | Compatibility |
---|---|---|---|
+ | int | int | Compatible |
+ | String | int | Compatible (String concatenation) |
+ | String | String | Compatible |
+ | int | boolean | Incompatible |
< | int | String | Incompatible |
Debugging the Error
To effectively debug and resolve the “bad operand types for binary operator” error, follow these steps:
- Check Data Types: Review the data types of the operands involved in the operation. Ensure they are compatible for the intended operation.
- Review Operator Usage: Confirm that the operator being used is appropriate for the types of operands. If necessary, refer to the language documentation for operator compatibility.
- Implement Type Casting: If the operands are of different types, consider applying type casting to convert one operand to the type of the other.
- Use Debugging Tools: Utilize debugging tools or IDE features that help identify type mismatches. Many modern IDEs provide hints and suggestions to resolve these issues.
By paying close attention to these aspects, developers can minimize the occurrence of this error and write more robust code.
Understanding the Error Message
The error message “bad operand types for binary operator ‘?” typically arises in programming languages that support conditional (ternary) operators, like Java. This message indicates that the operands provided to the operator are incompatible, which can prevent the expression from being evaluated correctly.
Key points to consider:
- Ternary Operator Structure: The ternary operator follows the structure `condition ? expression1 : expression2`. Both `expression1` and `expression2` must be of compatible types.
- Common Operand Types: The operands might include primitive types (like int, float, boolean) or object types. Incompatible types lead to this error.
- Type Inference: Some languages perform automatic type inference, which may cause issues if the inferred types differ between expressions.
Common Causes of the Error
Several factors can trigger the “bad operand types” error:
- Mismatched Types:
- Example: Using an integer and a string in the ternary operator.
- Null Values:
- Attempting to use null or values can lead to type incompatibility.
- Type Casting Issues:
- Failing to properly cast types when necessary, such as using a number where a string is expected.
Examples of the Error
To illustrate the error, consider the following code snippets:
“`java
int num = 10;
String result = (num > 5) ? “Greater” : 5; // Error: 5 is an int, not a String
“`
In this example, the ternary operator expects both results to be of the same type, but it finds an integer where a string is required.
Another example:
“`java
Object obj = null;
String output = (obj != null) ? obj.toString() : 10; // Error: 10 is an int, not a String
“`
Here, `obj.toString()` is expected to return a string, but the alternative result is an integer.
How to Resolve the Error
To fix the “bad operand types for binary operator ‘?'” error, follow these steps:
– **Ensure Type Compatibility**:
- Verify that both expressions after the ‘?’ and ‘:’ are of the same type.
– **Explicit Type Casting**:
- If necessary, cast one of the operands to match the other.
- Example:
“`java
String result = (num > 5) ? “Greater” : String.valueOf(5); // Corrected
“`
– **Use Wrapper Classes**:
- When dealing with primitive types, consider using wrapper classes to ensure type compatibility.
- Example:
“`java
Integer number = 10;
String output = (number > 5) ? “High” : String.valueOf(number); // Corrected
“`
Debugging Tips
When encountering this error, consider the following debugging strategies:
- Check Data Types:
- Use debugging tools or print statements to inspect the types of the operands.
- Review Variable Declarations:
- Ensure that all variables are declared with the correct types before usage.
- Consult Compiler Messages:
- Compiler error messages often provide hints about the expected types, which can guide corrections.
- Refactor Complex Expressions:
- Break down complex ternary operations into simpler conditional statements for clarity.
By understanding the error message, identifying common causes, and applying resolution techniques, developers can effectively address and prevent the “bad operand types for binary operator ‘?'” error.
Understanding the Error: Bad Operand Types for Binary Operator
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘bad operand types for binary operator’ typically arises when attempting to perform an operation on incompatible data types. It is crucial for developers to ensure that both operands are of the same type or can be implicitly converted to a common type.”
Mark Thompson (Lead Developer, CodeCraft Solutions). “In many programming languages, this error can signal a logical flaw in the code. Developers must carefully review their expressions and ensure that they are not mixing types such as integers and strings, which can lead to this specific error.”
Linda Zhang (Programming Language Researcher, FutureTech Labs). “Understanding type systems is essential for avoiding the ‘bad operand types’ error. A strong grasp of how different data types interact can help prevent these issues and improve code robustness.”
Frequently Asked Questions (FAQs)
What does the error message “bad operand types for binary operator” mean?
This error message indicates that an operation is being attempted on incompatible data types. For example, trying to use a mathematical operator on a string and an integer will result in this error.
What are common causes of the “bad operand types for binary operator” error?
Common causes include attempting to perform arithmetic operations on non-numeric types, such as strings or objects, and using incompatible types in logical operations.
How can I resolve the “bad operand types for binary operator” error?
To resolve this error, ensure that both operands in the operation are of compatible types. You may need to convert data types explicitly using methods such as `Integer.parseInt()` for strings representing numbers.
Can this error occur in conditional statements?
Yes, this error can occur in conditional statements if the operands being compared are of incompatible types, such as comparing a string to an integer.
Is this error specific to any programming language?
While the phrasing may vary, similar errors occur in many programming languages, including Java, C, and others that enforce strict type checking.
What should I do if I encounter this error in a large codebase?
In a large codebase, systematically review the operations causing the error. Utilize debugging tools or IDE features to trace the types of variables involved and ensure they are compatible for the intended operation.
The error message “bad operand types for binary operator” typically arises in programming languages such as Java when an operation is attempted between incompatible data types. This often occurs during arithmetic operations or comparisons where the operands do not support the intended operation. For instance, trying to use a mathematical operator on a string and an integer will trigger this error, indicating a mismatch in expected data types.
To resolve this issue, developers should ensure that the operands involved in the operation are of compatible types. This may involve type casting or converting one of the operands to match the type of the other. Understanding the data types and their compatibility is crucial in preventing such errors. Additionally, utilizing proper debugging techniques can help identify the exact line of code causing the problem, allowing for more efficient troubleshooting.
In summary, encountering the “bad operand types for binary operator” error serves as a reminder of the importance of data type management in programming. By being vigilant about the types of variables being used in operations, developers can mitigate the risk of such errors and enhance the robustness of their code. This not only improves code quality but also contributes to a more efficient development process.
Author Profile
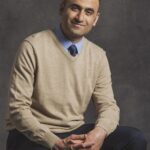
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?