How Can You Check the Number of Arguments in a Bash Script?
In the world of programming, particularly when working with shell scripts, the ability to handle input dynamically is crucial. One of the fundamental aspects of this is checking the number of arguments passed to a script. Whether you’re a seasoned developer or just embarking on your coding journey, understanding how to manage input can significantly enhance the robustness and usability of your scripts. In this article, we will delve into the nuances of argument handling in Bash, exploring how to effectively check the number of arguments and respond accordingly.
When you execute a Bash script, you often want to ensure that the right amount of information is provided by the user. This is where argument checking comes into play. By validating the number of arguments, you can prevent errors, guide users toward correct usage, and create a more user-friendly experience. This practice not only helps in debugging but also ensures that your scripts perform their intended functions without unexpected interruptions.
As we explore this topic, we will cover the various methods available in Bash for checking the number of arguments, along with practical examples that illustrate these techniques in action. Whether you’re looking to enforce mandatory parameters or provide optional ones, mastering this skill will empower you to write cleaner and more efficient scripts. Get ready to enhance your Bash scripting prowess!
Checking Number of Arguments in Bash
In Bash scripting, it is often essential to verify the number of arguments passed to a script or function. This ensures that the script executes correctly and handles any potential errors that arise from insufficient or excessive arguments. The special variable `$` is utilized to determine how many arguments were provided.
To check the number of arguments, you can use a simple conditional statement. Here’s a basic example:
“`bash
!/bin/bash
if [ $-ne 2 ]; then
echo “Usage: $0 arg1 arg2”
exit 1
fi
“`
In this script, the condition checks if the number of arguments (`$`) is not equal to 2. If the condition is true, it outputs a usage message and exits with a status code of 1, indicating an error.
Common Conditional Checks
Here are some common checks that can be performed using the `$` variable:
- Check for No Arguments:
Use `if [ $-eq 0 ];` to determine if no arguments were provided.
- Check for One Argument:
Use `if [ $-eq 1 ];` to ensure exactly one argument is given.
- Check for More Than Expected Arguments:
Use `if [ $-gt N ];` to handle cases where more arguments than expected are passed, where `N` is the maximum number of arguments.
Example Usage
Below is a more comprehensive example that demonstrates various checks based on the number of arguments:
“`bash
!/bin/bash
if [ $-eq 0 ]; then
echo “Error: No arguments provided.”
exit 1
elif [ $-gt 3 ]; then
echo “Error: Too many arguments provided.”
exit 1
else
echo “Number of arguments: $”
Proceed with processing the arguments
fi
“`
This script checks for no arguments and for too many arguments, providing appropriate error messages for each case.
Return Codes and Script Logic
Using return codes effectively allows you to manage script execution flow based on argument validation. Here is a brief overview of return codes:
Return Code | Description |
---|---|
0 | Success |
1 | General error (e.g., incorrect number of arguments) |
2 | Misuse of shell builtins (e.g., syntax error) |
By structuring your Bash scripts with these checks and return codes, you can create robust and user-friendly scripts that handle various input scenarios gracefully.
Checking Number of Arguments in Bash
To determine the number of arguments passed to a Bash script, you can utilize the special variable `$`, which holds the count of positional parameters. This allows you to implement conditional logic based on the number of arguments provided.
Using Conditional Statements
You can use an `if` statement to check the number of arguments and execute code based on that count. Here is a basic example:
“`bash
!/bin/bash
if [ $-eq 0 ]; then
echo “No arguments provided.”
elif [ $-eq 1 ]; then
echo “One argument provided: $1”
else
echo “Number of arguments provided: $”
fi
“`
This script checks:
- If no arguments are given, it outputs a message.
- If one argument is provided, it displays that argument.
- If more than one argument is given, it shows the total count.
Examples of Argument Checks
The following examples demonstrate various ways to check arguments in a Bash script:
- Checking for a specific number of arguments:
“`bash
if [ $-ne 2 ]; then
echo “Usage: $0 arg1 arg2”
exit 1
fi
“`
- Looping through arguments:
“`bash
for arg in “$@”; do
echo “Argument: $arg”
done
“`
- Using a function to validate arguments:
“`bash
check_args() {
if [ $-lt 2 ]; then
echo “At least two arguments are required.”
exit 1
fi
}
check_args “$@”
“`
Best Practices for Argument Handling
When writing scripts that rely on user input via command-line arguments, consider the following best practices:
- Provide usage instructions: Always inform users of the expected input format.
- Validate inputs: Implement checks for both the count and validity of arguments.
- Utilize default values: When appropriate, assign default values to missing arguments.
Table of Commonly Used Special Variables
Variable | Description |
---|---|
`$` | Number of positional parameters |
`$@` | All positional parameters as separate arguments |
`$*` | All positional parameters as a single word |
`$1`, `$2`, … | Individual positional parameters |
By following these guidelines and utilizing the `$` variable effectively, you can manage input arguments in your Bash scripts proficiently.
Expert Insights on Checking Number of Arguments in Bash
Dr. Emily Carter (Senior Software Engineer, Open Source Initiative). “In Bash scripting, checking the number of arguments is crucial for ensuring that scripts run smoothly without unexpected errors. Utilizing the special variable `$` allows developers to easily ascertain the number of arguments passed, enabling them to implement conditional logic based on this count.”
Michael Chen (DevOps Specialist, Tech Innovations Inc.). “A common practice in Bash scripting is to validate the number of arguments at the start of the script. This can prevent runtime errors and improve user experience. By using an if statement to check `$`, scripts can provide informative messages when the required number of arguments is not met.”
Sarah Johnson (Bash Scripting Consultant, CodeMaster Solutions). “Employing argument checks in Bash scripts is not just about error handling; it also enhances the script’s usability. By clearly defining expected arguments and providing feedback through conditional checks, developers can create more robust and user-friendly scripts.”
Frequently Asked Questions (FAQs)
How can I check the number of arguments passed to a bash script?
You can check the number of arguments by using the special variable `$`, which holds the count of positional parameters passed to the script.
What does the special variable `$` represent in a bash script?
The special variable `$` represents the total number of positional parameters or arguments passed to the script when it is executed.
How can I implement a conditional statement based on the number of arguments in bash?
You can use an `if` statement to compare `$` with the expected number of arguments. For example: `if [ $-ne 2 ]; then echo “Two arguments required”; fi`.
Can I check for a specific number of arguments in a bash function?
Yes, you can use `$` within a function to check the number of arguments passed to that function, just as you would in a script.
What happens if I do not pass any arguments to a bash script?
If no arguments are passed, `$` will be equal to zero, and any checks you perform against it will reflect that absence of arguments.
Is it possible to provide default values for arguments in bash?
Yes, you can assign default values by checking `$` and setting variables accordingly, for example: `arg1=${1:-default_value}` to use `default_value` if no argument is provided.
In Bash scripting, checking the number of arguments passed to a script or function is a fundamental practice that ensures the correct execution of code. The special variable `$` is utilized to determine the count of positional parameters, allowing developers to implement conditional logic based on the number of arguments received. This practice not only enhances the robustness of scripts but also improves user experience by providing clear feedback when the expected number of arguments is not met.
Implementing argument checks can prevent errors and unintended behavior in scripts. By using conditional statements, such as `if` statements, developers can easily validate whether the correct number of arguments has been provided. This validation can include displaying usage instructions or error messages, which guide users on how to properly execute the script. Overall, this contributes to more reliable and user-friendly Bash scripts.
In summary, checking the number of arguments in Bash is a crucial aspect of scripting that promotes error handling and user guidance. By leveraging the `$` variable and implementing appropriate conditional logic, developers can create scripts that are not only functional but also resilient to user input errors. This practice is essential for anyone looking to write effective and maintainable Bash scripts.
Author Profile
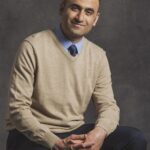
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?