How Do I Resolve the ‘botocore.exceptions.NoCredentialsError: Unable to Locate Credentials’ Issue?
In the realm of cloud computing and data management, Amazon Web Services (AWS) stands out as a powerful platform that enables businesses to harness the full potential of the cloud. However, navigating the intricacies of AWS can sometimes lead to frustrating roadblocks, particularly when it comes to authentication and access management. One common hurdle that developers and system administrators encounter is the notorious `botocore.exceptions.NoCredentialsError: Unable to locate credentials`. This error message can be a source of confusion and delay, but understanding its implications is crucial for anyone working with AWS SDKs.
As you delve deeper into the world of AWS, you may find yourself grappling with the various methods of credential management. The `NoCredentialsError` typically arises when the AWS SDK for Python, known as Boto3, fails to locate the necessary credentials to authenticate API requests. This can stem from a variety of issues, including misconfigured environment variables, missing configuration files, or even the absence of IAM roles. By grasping the underlying causes of this error, you can streamline your development process and ensure seamless interactions with AWS services.
In this article, we will explore the common scenarios that lead to the `NoCredentialsError`, as well as best practices for managing AWS credentials effectively. Whether you’re a seasoned
Understanding NoCredentialsError
The `NoCredentialsError` from the `botocore.exceptions` module indicates that the AWS SDK for Python (Boto3) is unable to find valid AWS credentials. This error can arise from various situations, including misconfigurations in environment variables, missing configuration files, or even issues in the IAM roles assigned to the AWS services being used.
Common scenarios that lead to this error include:
- Missing AWS credentials file: Boto3 expects credentials to be located in the `~/.aws/credentials` file. If this file is absent or improperly formatted, the SDK will not be able to authenticate your requests.
- Environment variables not set: Boto3 can also retrieve credentials from environment variables. If these are not set correctly, the SDK will fail to locate your AWS credentials.
- IAM roles for services: When using AWS services such as EC2 or Lambda, you can assign IAM roles. If the role does not have the necessary permissions, or if it is not correctly configured, you may encounter this error.
Resolving NoCredentialsError
To resolve the `NoCredentialsError`, consider the following steps:
- Check the AWS credentials file:
- Ensure that the `~/.aws/credentials` file exists and is correctly formatted. A typical credentials file looks like this:
“`
[default]
aws_access_key_id = YOUR_ACCESS_KEY
aws_secret_access_key = YOUR_SECRET_KEY
“`
- Set environment variables:
- If you prefer using environment variables, ensure the following are correctly set:
“`
export AWS_ACCESS_KEY_ID=YOUR_ACCESS_KEY
export AWS_SECRET_ACCESS_KEY=YOUR_SECRET_KEY
“`
- Review IAM roles and permissions:
- If your application is running on an AWS service, verify that the assigned IAM role has the necessary permissions to access the services it needs.
- Use the AWS CLI for testing:
- Test your credentials using the AWS CLI. Run the following command to check if the credentials are valid:
“`
aws sts get-caller-identity
“`
If this command returns your account details, then your credentials are correctly set up.
Method | Location | Notes |
---|---|---|
AWS Credentials File | ~/.aws/credentials | Ensure correct format and permissions |
Environment Variables | System Environment | Use export commands in terminal |
IAM Roles | AWS Services | Ensure proper permissions are assigned |
By following these steps and ensuring that your credentials are correctly configured, you can avoid encountering the `NoCredentialsError` when using Boto3 to interact with AWS services.
Understanding NoCredentialsError
The `NoCredentialsError` in the Botocore library occurs when the AWS SDK for Python (Boto3) cannot find valid AWS credentials to authenticate requests. This error indicates that the application is unable to locate the necessary credentials to access AWS services.
Common Causes
Several factors can lead to this error, including:
- Missing Configuration Files: AWS credentials might not be present in the default configuration file, typically located at `~/.aws/credentials`.
- Environment Variables Not Set: The required environment variables (`AWS_ACCESS_KEY_ID` and `AWS_SECRET_ACCESS_KEY`) may not be defined.
- IAM Role Issues: If running on AWS services like EC2 or Lambda, the instance or function might not have an attached IAM role with proper permissions.
- Profile Misconfiguration: Specifying a profile that does not exist in the credentials file can lead to this error.
How to Resolve NoCredentialsError
To fix the `NoCredentialsError`, you can adopt several strategies:
- Configure Credentials File: Ensure that the AWS credentials file is correctly set up. The file should look like this:
“`ini
[default]
aws_access_key_id = YOUR_ACCESS_KEY_ID
aws_secret_access_key = YOUR_SECRET_ACCESS_KEY
“`
- Set Environment Variables: You can manually set the credentials in your environment:
“`bash
export AWS_ACCESS_KEY_ID=YOUR_ACCESS_KEY_ID
export AWS_SECRET_ACCESS_KEY=YOUR_SECRET_ACCESS_KEY
“`
- Use IAM Roles: If deploying on AWS infrastructure, ensure that your instance or Lambda function has an IAM role attached with the necessary permissions.
- Specify the Profile: If using multiple profiles, ensure you specify the profile correctly in your code:
“`python
import boto3
session = boto3.Session(profile_name=’your_profile’)
“`
Verification Steps
After implementing the above fixes, verify if the credentials are correctly configured:
- Test Configuration: Run the following command to check if your credentials are correctly configured:
“`bash
aws sts get-caller-identity
“`
A successful response indicates that your credentials are working.
- Debugging with Boto3: You can enable logging to get more details about the error:
“`python
import logging
logging.basicConfig(level=logging.DEBUG)
“`
- Review IAM Policies: Ensure that the IAM user or role has the necessary permissions to perform the actions you intend.
Best Practices
To avoid encountering `NoCredentialsError` in the future, consider implementing these best practices:
- Use IAM Roles for Applications: Whenever possible, use IAM roles instead of static credentials.
- Secure Your Credentials: Avoid hardcoding credentials in your application. Use environment variables or AWS Secrets Manager.
- Regularly Rotate Credentials: Regularly update and rotate your AWS access keys to enhance security.
- Monitor AWS Logs: Keep an eye on AWS CloudTrail logs to track API calls and identify authentication issues.
Incorporating these strategies will help ensure that your application consistently locates and utilizes the correct AWS credentials, minimizing disruptions caused by the `NoCredentialsError`.
Understanding botocore.exceptions.NoCredentialsError: Insights from Cloud Computing Experts
Dr. Emily Carter (Cloud Solutions Architect, Tech Innovations Inc.). “The `NoCredentialsError` in botocore typically indicates that the AWS SDK is unable to find valid credentials for authentication. This can occur if the AWS credentials are not set in the environment variables, configuration files, or if the IAM roles are not properly assigned.”
Mark Thompson (Senior DevOps Engineer, CloudOps Solutions). “To resolve the `NoCredentialsError`, developers should ensure that the AWS CLI is configured correctly, using the `aws configure` command. It is also essential to verify that the credentials file is located in the correct directory and that the permissions allow access.”
Lisa Nguyen (AWS Certified Solutions Architect, Cloud Consultancy Group). “In scenarios where applications run in AWS environments, such as EC2 instances, it is vital to leverage IAM roles instead of hardcoding credentials. This practice not only mitigates the risk of credential leaks but also simplifies the management of permissions.”
Frequently Asked Questions (FAQs)
What does the error “botocore.exceptions.NoCredentialsError: Unable to locate credentials” mean?
This error indicates that the AWS SDK for Python (Boto3) cannot find the necessary AWS credentials to authenticate requests to AWS services.
How can I resolve the “NoCredentialsError” in my application?
Ensure that your AWS credentials are correctly configured. You can set them in the `~/.aws/credentials` file, use environment variables, or provide them directly in your code.
Where should I store my AWS credentials securely?
Store AWS credentials in the `~/.aws/credentials` file, use AWS Identity and Access Management (IAM) roles for EC2 instances, or utilize AWS Secrets Manager for enhanced security.
Can I use environment variables to provide AWS credentials?
Yes, you can set the following environment variables: `AWS_ACCESS_KEY_ID`, `AWS_SECRET_ACCESS_KEY`, and optionally `AWS_SESSION_TOKEN` for temporary credentials.
What should I do if I have multiple AWS profiles?
Specify the profile you wish to use by setting the `AWS_PROFILE` environment variable or by using the `boto3.Session(profile_name=’your_profile_name’)` method in your code.
Is it possible to run AWS CLI commands without configuring credentials?
No, you must configure AWS credentials to run AWS CLI commands, as they require authentication to access AWS services.
The error message “botocore.exceptions.NoCredentialsError: Unable to locate credentials” is a common issue encountered by users of the AWS SDK for Python, known as Boto3. This error typically arises when the SDK cannot find the necessary AWS credentials to authenticate API requests. The absence of credentials can stem from various factors, including misconfigured environment variables, missing configuration files, or incorrect IAM roles assigned to the executing environment. Understanding the root causes of this error is essential for effective troubleshooting and resolution.
To resolve the NoCredentialsError, users should first ensure that their AWS credentials are correctly set up. This can be achieved by checking the AWS credentials file located at `~/.aws/credentials` or by setting environment variables such as `AWS_ACCESS_KEY_ID` and `AWS_SECRET_ACCESS_KEY`. Additionally, users should verify that the IAM roles associated with their AWS services, such as EC2 instances or Lambda functions, have the appropriate permissions to access the required resources.
It is also beneficial to utilize the AWS CLI to confirm that the credentials are functioning correctly. Running commands such as `aws sts get-caller-identity` can help ascertain whether the SDK is able to authenticate successfully. Furthermore, developers should consider implementing error handling in their applications
Author Profile
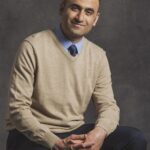
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?