How Can You Remove the Last Character from a String in C#?
In the world of programming, string manipulation is a fundamental skill that every developer should master. Whether you’re cleaning up user input, formatting data for display, or simply refining your code, knowing how to manipulate strings effectively can save you time and enhance your application’s performance. One common task that developers encounter is the need to remove the last character from a string in C. This seemingly simple operation can have a significant impact on your code’s functionality and readability, making it an essential topic for both novice and experienced programmers alike.
Removing the last character from a string in Ccan be achieved through various methods, each with its own advantages and considerations. Understanding the nuances of string manipulation in Cnot only empowers you to execute this task efficiently but also equips you with the knowledge to tackle more complex string operations. As you delve deeper into the topic, you’ll discover different approaches, such as using built-in methods or leveraging the power of string indexing, each offering unique benefits depending on your specific use case.
Moreover, mastering string manipulation opens up a world of possibilities in your programming journey. From data validation to dynamic content generation, the ability to modify strings effectively is a cornerstone of robust application development. So, whether you’re looking to streamline your code or simply curious about best practices, join us as we explore
Using String.Substring Method
One of the most straightforward ways to remove the last character from a string in Cis by utilizing the `Substring` method. This method allows you to create a new string that consists of a specified range of characters from the original string.
To remove the last character, you can specify the starting index as 0 and the length as `string.Length – 1`. Here is an example:
“`csharp
string originalString = “Hello!”;
string modifiedString = originalString.Substring(0, originalString.Length – 1);
“`
This will result in `modifiedString` containing “Hello”.
Using String.Remove Method
Another method to remove the last character is by using the `Remove` method. This method can be particularly intuitive, as it directly specifies the character to be removed by index.
The syntax is as follows:
“`csharp
string originalString = “Hello!”;
string modifiedString = originalString.Remove(originalString.Length – 1);
“`
This will also yield “Hello” as the output.
Using String.TrimEnd Method
If the last character you want to remove is a specific character (for example, an exclamation mark or a space), you can utilize the `TrimEnd` method. This approach is especially useful when dealing with strings that may have trailing characters that need to be handled:
“`csharp
string originalString = “Hello!”;
string modifiedString = originalString.TrimEnd(‘!’);
“`
This will result in `modifiedString` being “Hello”.
Performance Considerations
While all the aforementioned methods are effective, the choice of method may depend on performance needs, especially in scenarios involving large strings or frequent operations. Below is a summary of their performance characteristics:
Method | Complexity | Use Case |
---|---|---|
Substring | O(n) | General purpose |
Remove | O(n) | General purpose |
TrimEnd | O(n) | Specific character removal |
In general, for most applications, any of these methods will perform adequately. However, if you are working in a performance-sensitive context, consider conducting benchmarks to determine the best approach for your specific situation.
Conclusion on Best Practices
When choosing a method to remove the last character from a string in C, consider the following best practices:
- Use `Substring` for a simple and clear-cut removal of the last character.
- Choose `Remove` for clarity when you need to eliminate characters by index.
- Opt for `TrimEnd` when dealing with specific trailing characters.
- Always validate the string length before attempting to remove characters to avoid exceptions.
Using String Manipulation Methods
In C, several methods can be employed to remove the last character from a string. The most common methods include using the `Substring` method and the `Remove` method.
Substring Method
The `Substring` method allows you to extract a portion of a string based on specified starting index and length. To remove the last character, you can specify the starting index as `0` and the length as `string.Length – 1`.
“`csharp
string originalString = “Hello!”;
string modifiedString = originalString.Substring(0, originalString.Length – 1);
// modifiedString is now “Hello”
“`
Remove Method
The `Remove` method can also be utilized to eliminate the last character by specifying the starting index and the number of characters to remove.
“`csharp
string originalString = “Hello!”;
string modifiedString = originalString.Remove(originalString.Length – 1, 1);
// modifiedString is now “Hello”
“`
Using String Interpolation and Length Property
String interpolation is a concise way to handle strings. While it is typically used for building new strings, it can also be combined with the `Length` property to effectively remove the last character.
“`csharp
string originalString = “Hello!”;
string modifiedString = $”{originalString.Substring(0, originalString.Length – 1)}”;
// modifiedString is now “Hello”
“`
Performance Considerations
When dealing with string manipulation in C, it’s essential to understand the performance implications, especially with larger strings.
- Immutability: Strings in Care immutable, meaning any modification creates a new string instance. This can lead to increased memory usage and garbage collection overhead.
- Method Efficiency: Between `Substring` and `Remove`, performance differences are negligible for typical use cases. However, if you’re frequently modifying large strings, consider using `StringBuilder` for better performance.
Method | Description | Performance Impact |
---|---|---|
Substring | Extracts a part of the string | Low for small strings |
Remove | Removes specified characters from a string | Low for small strings |
StringBuilder | Efficient for multiple modifications | Better for large strings |
Example Scenarios
When to use each method can depend on the context of your application:
- Simple Removal: If you simply need to remove one character from a string, `Substring` or `Remove` is sufficient.
- Complex Manipulations: If you are building a string dynamically and making multiple changes, consider using `StringBuilder`.
“`csharp
StringBuilder sb = new StringBuilder(“Hello!”);
sb.Length–; // Removes the last character
string modifiedString = sb.ToString(); // modifiedString is now “Hello”
“`
Utilizing the appropriate method based on the scenario can enhance the readability and performance of your code.
Expert Insights on Removing the Last Character from Strings in C
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “In C, the simplest way to remove the last character from a string is by using the `Substring` method. This method allows developers to specify the starting index and the length of the desired substring, effectively omitting the last character with a straightforward approach.”
Michael Thompson (Lead Developer, Tech Innovations Inc.). “Another efficient method to remove the last character from a string in Cis by leveraging the `Remove` method. By specifying the index of the last character, developers can easily manipulate strings without the need for additional libraries or complex logic.”
Linda Nguyen (CProgramming Instructor, Dev Academy). “It is essential for developers to understand that strings in Care immutable. Therefore, any operation that modifies a string will result in the creation of a new string. Using methods like `Substring` or `Remove` is not only effective but also crucial for efficient memory management in applications.”
Frequently Asked Questions (FAQs)
How can I remove the last character from a string in C?
You can remove the last character from a string in Cusing the `Substring` method. For example, `string result = originalString.Substring(0, originalString.Length – 1);` effectively trims the last character.
What happens if the string is empty when trying to remove the last character?
If the string is empty and you attempt to remove the last character, it will throw an `ArgumentOutOfRangeException`. Always check if the string length is greater than zero before performing the operation.
Is there a built-in method in Cto remove the last character from a string?
Cdoes not have a specific built-in method for removing the last character, but you can achieve this using `Substring` or `Remove` methods, as demonstrated in various examples.
Can I remove multiple characters from the end of a string in C?
Yes, you can remove multiple characters by adjusting the parameters in the `Substring` method. For instance, to remove the last three characters, use `originalString.Substring(0, originalString.Length – 3);`.
What is the performance impact of removing characters from a string in C?
Removing characters from a string using methods like `Substring` or `Remove` creates a new string object, which can have performance implications in scenarios involving large strings or frequent modifications. Consider using `StringBuilder` for more efficient string manipulations.
Are there any alternatives to removing the last character from a string in C?
Alternatives include using `StringBuilder` for mutable strings or leveraging LINQ to create a new string without the last character, such as `new string(originalString.Take(originalString.Length – 1).ToArray());`.
In C, removing the last character from a string is a straightforward task that can be accomplished using various methods. The most common approach is to utilize the `Substring` method, which allows developers to specify the starting index and length of the substring they wish to extract. By taking the substring from the beginning of the string to the second-to-last character, one can effectively remove the last character. Additionally, the `Remove` method can also be employed, which directly modifies the string by specifying the index of the character to be removed and the count of characters to delete.
Another valuable technique involves using the `String.Length` property to determine the length of the string, thus enabling the removal of the last character with precision. It is important to handle potential edge cases, such as empty strings, to avoid exceptions. By incorporating checks to ensure the string has a length greater than zero before attempting to remove a character, developers can write robust and error-free code.
In summary, Cprovides multiple methods to remove the last character from a string, each with its own advantages. Understanding these methods allows developers to choose the most suitable approach based on their specific requirements. By prioritizing code safety and clarity, programmers can enhance the maintainability of their
Author Profile
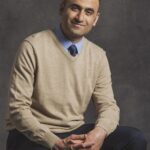
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?