How Can I Call a PowerShell Script from Another PowerShell Script?
In the realm of Windows automation and system administration, PowerShell has emerged as a powerful tool for managing tasks and orchestrating complex workflows. As scripts grow in complexity and size, the need to modularize code becomes increasingly apparent. One effective way to achieve this is by calling a PowerShell script from another PowerShell script. This technique not only enhances code reusability but also fosters better organization and maintenance, allowing administrators and developers to streamline their processes with ease. In this article, we will explore the various methods and best practices for invoking scripts within PowerShell, empowering you to take your automation skills to the next level.
When you call a PowerShell script from another script, you open the door to a world of possibilities. This approach allows you to break down larger tasks into smaller, manageable components, making your scripts easier to read and maintain. By creating a library of reusable scripts, you can save time and reduce redundancy, ensuring that your automation efforts are both efficient and effective. Additionally, understanding how to properly pass parameters between scripts can significantly enhance their functionality and adaptability.
As we delve deeper into this topic, we will discuss the different methods available for invoking scripts, including the use of the `&` call operator, the `Start-Process` cmdlet
Calling a PowerShell Script
To call a PowerShell script from another PowerShell script, you can use several methods, depending on your requirements for parameters, execution context, and output handling. Below are the most common approaches:
- Using the `&` call operator: This is the simplest method to run another script. You can specify the script path directly.
“`powershell
& “C:\Path\To\YourScript.ps1”
“`
- Using `Invoke-Expression`: This method allows you to execute a string as a command. However, it is generally less preferred due to potential security risks with code injection.
“`powershell
Invoke-Expression “C:\Path\To\YourScript.ps1”
“`
- Using `Start-Process`: This method is useful when you want to run the script in a separate process. It also allows you to pass parameters easily.
“`powershell
Start-Process powershell -ArgumentList “-File C:\Path\To\YourScript.ps1”
“`
Passing Parameters
When calling a script, you may need to pass parameters to it. Here’s how you can do this effectively:
- Define parameters in the called script using the `param` block.
“`powershell
YourScript.ps1
param (
[string]$Param1,
[int]$Param2
)
“`
- Pass the parameters when calling the script:
“`powershell
& “C:\Path\To\YourScript.ps1” -Param1 “Value1” -Param2 2
“`
Alternatively, if using `Start-Process`, you can provide parameters in the `-ArgumentList`.
“`powershell
Start-Process powershell -ArgumentList “-File C:\Path\To\YourScript.ps1 -Param1 ‘Value1’ -Param2 2”
“`
Handling Output and Return Values
When a script executes, it may produce output or return values, which can be captured for further processing.
- Capturing output: You can capture the output of a script by assigning it to a variable.
“`powershell
$output = & “C:\Path\To\YourScript.ps1”
“`
- Returning values: Use the `return` keyword in the called script to return a value.
“`powershell
YourScript.ps1
return “Result”
“`
You can then capture this return value in the calling script:
“`powershell
$result = & “C:\Path\To\YourScript.ps1”
“`
Executing Scripts in Different Contexts
You may need to execute scripts in different contexts, such as with different user credentials or in different sessions. Here are a couple of methods:
- Using `Invoke-Command`: This allows execution on a remote machine or in a different session.
“`powershell
Invoke-Command -ScriptBlock { & “C:\Path\To\YourScript.ps1” } -ComputerName “RemoteMachine”
“`
- Using `RunAs` for different user credentials: This method is useful for scripts requiring elevated privileges.
“`powershell
Start-Process powershell -Credential (Get-Credential) -ArgumentList “-File C:\Path\To\YourScript.ps1”
“`
Method | Use Case | Example |
---|---|---|
& Call Operator | Simple execution | & “C:\Path\To\YourScript.ps1” |
Invoke-Expression | Execute string as command | Invoke-Expression “C:\Path\To\YourScript.ps1” |
Start-Process | Execute in a new process | Start-Process powershell -ArgumentList “-File C:\Path\To\YourScript.ps1” |
Calling a PowerShell Script from Another PowerShell Script
To call a PowerShell script from another PowerShell script, you can utilize various methods based on your requirements. Below are the most common approaches:
Using the `&` Operator
The `&` (call) operator allows you to execute a script or command. This is particularly useful when you need to invoke another script within your current script.
“`powershell
& “C:\Path\To\YourScript.ps1”
“`
- The path to the script can be either absolute or relative.
- Ensure the script has the necessary permissions to execute.
Using `Invoke-Expression`
`Invoke-Expression` can also be employed to run a script. This method is generally less preferred due to potential security risks associated with evaluating strings as commands.
“`powershell
Invoke-Expression “C:\Path\To\YourScript.ps1”
“`
- This method is useful when you need to dynamically construct the command string.
Using `Start-Process`
If you want to run a script in a separate process, `Start-Process` is an appropriate choice. This is useful when you want to run the script asynchronously.
“`powershell
Start-Process “powershell.exe” -ArgumentList “-File C:\Path\To\YourScript.ps1”
“`
- This method allows you to pass additional arguments if required.
Passing Arguments to the Called Script
When calling a PowerShell script, you may want to pass parameters. Here’s how you can do it using the `&` operator:
“`powershell
& “C:\Path\To\YourScript.ps1” -Param1 “Value1” -Param2 “Value2”
“`
- Ensure the called script is designed to accept parameters using the `param` block.
Handling Output and Errors
When calling another script, you may want to capture output or handle errors effectively:
“`powershell
try {
$result = & “C:\Path\To\YourScript.ps1” -Param1 “Value1”
Write-Output $result
} catch {
Write-Error “An error occurred: $_”
}
“`
- Wrapping the call in a `try-catch` block helps in managing exceptions.
Examples of Script Calls
Here are a few examples illustrating different methods of invoking scripts:
Method | Example Code | Description |
---|---|---|
Call Operator | `& “C:\Scripts\MyScript.ps1″` | Directly calls the script. |
Invoke-Expression | `Invoke-Expression “C:\Scripts\MyScript.ps1″` | Evaluates and executes the script. |
Start-Process | `Start-Process “powershell.exe” -ArgumentList “-File C:\Scripts\MyScript.ps1″` | Runs in a new PowerShell process. |
With Arguments | `& “C:\Scripts\MyScript.ps1” -Param1 “Hello”` | Calls with parameters. |
By leveraging these methods, you can effectively manage the execution of PowerShell scripts, ensuring modular and maintainable code.
Expert Insights on Calling PowerShell Scripts
Dr. Emily Carter (Senior Systems Administrator, Tech Innovations Inc.). “To call a PowerShell script from another PowerShell script, you can use the `&` (call operator) or `Start-Process` cmdlet. The call operator is particularly useful for executing scripts in the current scope, while `Start-Process` allows for running scripts in a new process, which can be beneficial for performance and isolation.”
Michael Chen (PowerShell Automation Specialist, Automation Gurus). “When invoking a PowerShell script from another, it is crucial to manage the execution policy and ensure that the script paths are correctly defined. Utilizing relative paths can simplify the process, especially when scripts are located in the same directory.”
Lisa Thompson (DevOps Engineer, Cloud Solutions Corp.). “For effective script management, consider using parameters when calling a PowerShell script from another script. This allows for greater flexibility and reusability, enabling you to pass data between scripts seamlessly.”
Frequently Asked Questions (FAQs)
How can I call a PowerShell script from another PowerShell script?
You can call a PowerShell script from another script by using the `&` (call operator) followed by the path to the script. For example: `& “C:\Path\To\YourScript.ps1″`.
What happens if the called script has parameters?
If the called script requires parameters, you can pass them directly after the script path. For instance: `& “C:\Path\To\YourScript.ps1” -Param1 Value1 -Param2 Value2`.
Can I call a script located in a different directory?
Yes, you can call a script from a different directory by providing the full path to the script. Ensure that the path is correctly specified to avoid errors.
Is it possible to call a script and capture its output?
Yes, you can capture the output of a called script by assigning it to a variable. For example: `$output = & “C:\Path\To\YourScript.ps1″`.
What should I do if the called script is not executing?
If the script is not executing, check for execution policy restrictions. You may need to set the execution policy using `Set-ExecutionPolicy` or run the script with the `-ExecutionPolicy Bypass` flag.
Can I call multiple scripts in one script?
Yes, you can call multiple scripts within a single script by using the call operator for each script. For example:
“`powershell
& “C:\Path\To\FirstScript.ps1”
& “C:\Path\To\SecondScript.ps1”
“`
In summary, calling a PowerShell script from another PowerShell script is a straightforward process that enhances modularity and reusability in scripting. By using the `&` call operator or the `Invoke-Expression` cmdlet, users can execute external scripts seamlessly. This practice not only promotes cleaner code but also allows for the efficient management of complex tasks by breaking them down into manageable components.
Moreover, it is essential to consider the execution policy settings of PowerShell, as they can affect the ability to run scripts. Users should ensure that the appropriate execution policy is set, which can be done using the `Set-ExecutionPolicy` cmdlet. Additionally, passing parameters between scripts can be achieved by including them in the call, which further enhances the flexibility of script interactions.
Ultimately, leveraging the ability to call one PowerShell script from another can significantly streamline automation processes. It encourages best practices in script organization and can lead to improved productivity and maintainability in PowerShell scripting projects. By understanding and implementing these techniques, users can maximize the potential of their PowerShell scripts.
Author Profile
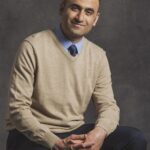
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?