Can a Tuple Serve as a Key in a Python Dictionary?
In the world of Python programming, dictionaries are an essential data structure that allows for efficient data retrieval through key-value pairs. While most developers are familiar with using strings or integers as keys, a lesser-known yet powerful feature is the ability to use tuples as dictionary keys. This capability opens up a realm of possibilities for organizing and accessing complex data structures, making your code not only more efficient but also more intuitive. If you’ve ever wondered about the nuances of using tuples in this context, you’re in for an enlightening exploration.
Tuples, being immutable sequences, bring a unique set of characteristics that make them suitable for use as dictionary keys. Unlike lists, which can change over time and thus cannot be hashed, tuples maintain a fixed state, allowing them to be hashed and used effectively in dictionaries. This property of immutability ensures that once a tuple is created, it can serve as a reliable key, providing stability in data retrieval and manipulation.
Moreover, using tuples as keys can enhance the organization of your data. By combining multiple elements into a single tuple, you can create composite keys that represent complex relationships or attributes. This approach not only simplifies your data structure but also improves the readability of your code. As we delve deeper into this topic, we will explore practical examples and best practices for leveraging
Understanding Tuples as Dictionary Keys
In Python, a dictionary is a mutable, unordered collection of items, where each item is stored as a key-value pair. Keys must be hashable, meaning they must be immutable and have a fixed value throughout their lifetime. Tuples, being immutable sequences, qualify as hashable types, allowing them to be used as keys in dictionaries.
However, there are some important considerations when using tuples as dictionary keys:
- Immutability: The tuple itself must be immutable. This means that the elements within the tuple must also be immutable types. For instance, a tuple containing integers or strings can be used as a key, while a tuple containing a list or a dictionary cannot.
- Hashability: Since dictionaries rely on hash values for their keys, any change to the contents of a tuple (if they were mutable) would affect its hash value, making it unsuitable as a key.
Examples of Tuples as Dictionary Keys
Here are some examples demonstrating the use of tuples as keys in a dictionary:
“`python
Valid tuple as a dictionary key
my_dict = {
(1, 2): “Value A”,
(3, 4): “Value B”
}
Accessing values using tuple keys
print(my_dict[(1, 2)]) Output: Value A
“`
This example shows valid usage, where tuples `(1, 2)` and `(3, 4)` serve as keys, enabling the retrieval of their respective values.
Conversely, consider the following case:
“`python
Invalid tuple as a dictionary key
my_dict_invalid = {
([1, 2], 3): “Value C” This will raise a TypeError
}
“`
In this instance, the list `[1, 2]` is mutable, thus making the tuple `([1, 2], 3)` unhashable and resulting in a `TypeError`.
Key Characteristics of Tuples for Dictionary Use
When considering tuples as dictionary keys, keep in mind the following characteristics:
Characteristic | Description |
---|---|
Immutability | Tuples cannot be changed after creation, ensuring consistent hash values. |
Hashable Elements | All elements within the tuple must be immutable (e.g., integers, strings). |
Usage | Effective for grouping related data as a single key, providing structure. |
Using tuples as keys can provide clarity and efficiency in data organization, especially when dealing with multi-dimensional data or complex structures.
Using Tuples as Dictionary Keys in Python
In Python, tuples can indeed be used as keys in a dictionary. This is primarily because tuples are immutable, which is a necessary property for any data type to serve as a key in a dictionary. The immutability ensures that the key’s hash value remains constant throughout its lifetime, allowing for efficient retrieval.
Characteristics of Tuples as Dictionary Keys
When utilizing tuples as keys in dictionaries, several important characteristics should be noted:
- Immutability: Tuples cannot be altered after creation, making them suitable for use as keys.
- Hashability: As long as all elements within the tuple are also hashable (e.g., integers, strings, or other tuples), the tuple itself can be used as a key.
- Nested Tuples: If a tuple contains mutable elements (like lists), it cannot be used as a dictionary key.
Example of Using Tuples as Keys
Here is a simple example demonstrating how to use tuples as keys in a Python dictionary:
“`python
Creating a dictionary with tuples as keys
coordinate_map = {
(0, 0): “Origin”,
(1, 2): “Point A”,
(3, 4): “Point B”
}
Accessing values using tuple keys
print(coordinate_map[(1, 2)]) Output: Point A
“`
In this example, the tuples representing coordinates serve as keys, and the corresponding values are descriptive strings.
Limitations of Tuples as Dictionary Keys
While using tuples as keys is advantageous, there are limitations to consider:
- Mutable Elements: A tuple containing a list or other mutable types cannot be used as a key.
- Performance: Although dictionary lookups with tuples as keys are efficient, the complexity can increase if the tuple is large or contains complex objects.
Practical Applications
Tuples as dictionary keys can be particularly useful in various scenarios, including:
- Coordinate Systems: Storing points in a 2D or 3D space.
- Multi-dimensional Data: Mapping combinations of multiple features, such as (age, income) for demographic data.
- Graph Representations: Using (node1, node2) pairs to represent edges in a graph.
Conclusion on Usage
tuples are a versatile choice for dictionary keys in Python due to their immutability and hashability. However, care must be taken to ensure that they contain only hashable elements to maintain the integrity of the dictionary. Understanding these principles allows for effective data organization and retrieval in Python applications.
Understanding Tuple Keys in Python Dictionaries
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, tuples can indeed serve as keys in dictionaries. This is primarily due to their immutable nature, which ensures that the hash value remains constant, allowing for reliable key-value associations.”
James Liu (Data Scientist, Analytics Hub). “Utilizing tuples as dictionary keys is particularly beneficial when you need to represent composite keys. This allows for more complex data structures and relationships to be efficiently managed within a single dictionary.”
Dr. Sarah Thompson (Computer Science Professor, University of Technology). “While tuples can be keys in dictionaries, it is crucial to remember that all elements within the tuple must also be hashable. This means that you cannot use tuples containing mutable types, such as lists, as dictionary keys.”
Frequently Asked Questions (FAQs)
Can a tuple be a key in a dictionary in Python?
Yes, a tuple can be used as a key in a dictionary in Python because it is immutable and hashable.
What makes a tuple suitable as a dictionary key?
A tuple is suitable as a dictionary key due to its immutability, which ensures that its hash value remains constant throughout its lifetime.
Are there any restrictions on the contents of a tuple used as a dictionary key?
Yes, all elements within the tuple must also be immutable and hashable. For example, tuples containing lists or other mutable types cannot be used as dictionary keys.
How do you create a dictionary with tuples as keys?
You can create a dictionary with tuples as keys by defining the tuples in parentheses and using them in the key-value pairs, like this: `my_dict = { (1, 2): ‘value1’, (3, 4): ‘value2’ }`.
Can a dictionary with tuple keys be modified?
Yes, while the keys themselves cannot be modified, you can add or remove key-value pairs from the dictionary as needed.
What happens if you use a mutable object as a key in a dictionary?
Using a mutable object as a key will raise a `TypeError` because mutable objects do not have a stable hash value, which is required for dictionary keys.
In Python, tuples can indeed be used as keys in dictionaries. This is primarily due to the fact that tuples are immutable, meaning their contents cannot be altered after creation. This immutability is a crucial requirement for dictionary keys, as keys must remain constant to ensure the integrity of the data structure. Consequently, tuples, when composed of immutable elements, can serve as effective keys, allowing for the association of complex data structures with unique identifiers.
It is important to note that while tuples themselves are immutable, they can contain mutable elements, such as lists. If a tuple includes any mutable objects, it cannot be used as a dictionary key. Therefore, when creating tuples for use as keys, one must ensure that all components are immutable, such as integers, strings, or other tuples. This characteristic allows for the creation of composite keys, which can enhance the flexibility and functionality of dictionaries in Python.
In summary, utilizing tuples as keys in Python dictionaries is not only permissible but also advantageous for certain applications. By leveraging the immutability of tuples, developers can create robust and efficient data structures that facilitate complex data management. Understanding the constraints and proper usage of tuples as dictionary keys is essential for effective programming in Python.
Author Profile
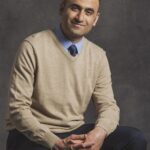
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?