Can I Statically Allocate List Length in Python?
In the dynamic world of programming, Python stands out for its flexibility and ease of use, particularly when it comes to handling data structures like lists. However, as developers dive deeper into Python’s capabilities, they often encounter questions about the nature of these structures. One such question that arises is whether it’s possible to allocate list lengths statically in Python. This inquiry touches on the fundamental differences between Python and statically-typed languages, where developers can define fixed sizes for arrays and lists before runtime.
Understanding the allocation of list lengths in Python is crucial for both novice and experienced programmers. Unlike languages such as C or Java, Python lists are inherently dynamic, allowing for the addition and removal of elements without a predetermined size. This flexibility provides significant advantages in many scenarios, but it also raises questions about performance and memory management. As we explore the nuances of list allocation in Python, we’ll uncover the implications of its dynamic nature and how it compares to static allocation in other programming languages.
Throughout this article, we will delve into various strategies for managing list sizes and explore alternative approaches that can simulate static allocation when necessary. By the end, you’ll have a clearer understanding of how to effectively work with lists in Python, empowering you to make informed decisions in your coding projects. Whether you’re looking to optimize your
Understanding List Allocation in Python
In Python, lists are dynamic data structures, meaning they can grow or shrink in size as needed. Unlike statically typed languages where you can allocate a fixed length for an array, Python does not allow for static allocation of list lengths. Instead, lists are inherently flexible, providing ease of use for developers.
When you create a list in Python, you can initialize it with a certain number of elements, but this number can change as you append or remove items. This dynamic nature allows for efficient memory usage, as Python handles resizing automatically.
Static Allocation Alternative: Using Tuples
Although Python does not support static list allocation, you can achieve similar functionality using tuples. Tuples are immutable sequences that can hold a fixed number of items. Once defined, the size and contents of a tuple cannot be altered.
Consider the following characteristics of tuples:
- Immutability: Once created, you cannot change the contents of a tuple.
- Fixed Size: The number of elements is defined at creation and remains constant.
- Performance: Tuples can be more memory-efficient than lists due to their fixed size.
Here’s an example of tuple creation:
python
fixed_tuple = (1, 2, 3)
Memory Management: Lists vs. Arrays
For scenarios requiring a fixed-size, more performance-oriented approach, you might consider using the `array` module from Python’s standard library. Unlike lists, arrays require you to specify a type code, allowing them to store elements of a single type efficiently.
Feature | List | Array |
---|---|---|
Flexibility | Dynamic | Static |
Element Type | Mixed types | Single type |
Memory Efficiency | Less efficient | More efficient |
Performance | Slower for large data | Faster for large data |
Here’s how to create an array:
python
import array
fixed_array = array.array(‘i’, [1, 2, 3]) # ‘i’ denotes integer type
Dynamic List Management Techniques
If you still prefer to use lists while managing their size dynamically, consider the following techniques:
- List Comprehensions: Create lists based on existing data in a concise manner.
python
squares = [x**2 for x in range(10)]
- Preallocation: If you anticipate needing a certain number of elements, you can initialize a list with placeholder values.
python
preallocated_list = [None] * 10 # Creates a list of 10 None values
- Using `len()`: You can check the length of a list at any time, allowing you to manage its contents based on current requirements.
python
current_length = len(preallocated_list)
In summary, while Python does not support static allocation of list lengths, it provides alternative structures and methods that can effectively meet various programming needs.
Static Allocation of List Length in Python
In Python, lists are dynamic data structures that can change in size during runtime. This flexibility allows for efficient memory usage and adaptation to varying data needs. However, the concept of statically allocating a list length—similar to fixed-size arrays in languages like C or C++—does not directly apply to Python. Here are some considerations regarding list length allocation:
- Dynamic Nature of Lists: Python lists can grow or shrink as elements are added or removed. You can initialize a list with a specific number of elements, but this does not prevent it from being resized later.
- Pre-Allocation: While you cannot statically allocate a list, you can pre-allocate a list with a fixed length using techniques such as:
- Initializing with a placeholder value:
python
fixed_length_list = [0] * 10 # Creates a list with 10 elements, all initialized to 0
- Using a list comprehension:
python
fixed_length_list = [None for _ in range(10)] # Creates a list with 10 elements initialized to None
- Tuple Usage: For scenarios where you want to enforce a fixed size, consider using tuples instead of lists. Tuples are immutable, meaning their size cannot be changed after creation:
python
fixed_tuple = (1, 2, 3) # A tuple with a fixed length of 3
- Array Module: If you require a more static structure with specific data types, the `array` module in Python allows for the creation of arrays that are more memory-efficient than lists:
python
import array
fixed_array = array.array(‘i’, [0] * 10) # Creates an array of integers with a fixed size of 10
Performance Considerations
When managing lists in Python, performance can be impacted by the dynamic resizing that occurs when elements are added or removed. Some points to consider include:
- Amortized Time Complexity: Appending elements to a list is typically O(1) due to amortized time complexity; however, resizing can occasionally lead to O(n) complexity when the underlying array needs to be expanded.
- Memory Overhead: Lists maintain some overhead for dynamic resizing, which can lead to increased memory consumption compared to statically sized data structures.
- Use Cases: Consider the following when deciding whether to use a list or another structure:
- If frequent size changes are required, lists are preferable.
- For fixed-size collections, tuples or arrays may be more efficient.
Data Structure | Mutability | Size Flexibility | Typical Use Cases |
---|---|---|---|
List | Mutable | Dynamic | General-purpose collections |
Tuple | Immutable | Fixed | Fixed-size records |
Array | Mutable | Fixed (with type) | Numerical data storage |
While Python does not support static allocation of list lengths in the traditional sense, various methods exist to create lists that behave in a manner similar to statically allocated arrays. Using tuples or arrays can achieve similar functionality with fixed sizes, depending on the specific requirements of your application.
Understanding Static List Allocation in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Group). “In Python, lists are inherently dynamic, meaning their size can change at runtime. Therefore, you cannot allocate a list’s length statically as you would in languages like C or Java. Instead, Python manages memory allocation automatically, allowing for flexible list sizes.”
Michael Chen (Lead Python Instructor, Code Academy). “While Python does not support static allocation of list lengths, you can initialize a list with a predefined size using list comprehensions or multiplication. However, this does not prevent the list from being resized later; it merely sets an initial capacity.”
Sarah Patel (Data Scientist, Tech Innovations Inc.). “For scenarios requiring fixed-size arrays, consider using the ‘array’ module or NumPy arrays. These alternatives allow for more static-like behavior, providing better performance for numerical computations while maintaining the flexibility of Python.”
Frequently Asked Questions (FAQs)
Can I allocate list length in Python statically?
No, Python does not support static allocation of lists. Lists in Python are dynamic in nature, allowing for flexible resizing as elements are added or removed.
What is the default behavior of list allocation in Python?
When a list is created in Python, it is allocated dynamically. This means that the size of the list can change at runtime based on the number of elements it contains.
How can I create a list with a predefined length in Python?
You can create a list with a predefined length by initializing it with a specific number of elements, such as using list comprehension or the multiplication operator, e.g., `my_list = [0] * 10` creates a list of ten zeros.
Are there performance implications of using dynamic lists in Python?
Yes, dynamic lists can incur performance overhead due to the need for resizing and copying elements when the list grows beyond its current capacity. However, Python’s list implementation is optimized for common use cases.
Can I enforce a maximum length for a list in Python?
While Python does not enforce a maximum length for lists, you can implement custom logic to restrict the number of elements added to a list, such as using a wrapper class or checking the length before appending new items.
What alternatives exist for fixed-size arrays in Python?
For fixed-size arrays, you can use the `array` module or the `numpy` library, which provides array-like structures that allow for more efficient storage and operations on fixed-length collections.
In Python, lists are dynamic in nature, meaning that their length can change at runtime. This flexibility allows for efficient memory usage and ease of use, as developers do not need to define the size of a list beforehand. Consequently, Python does not support static allocation of list lengths, which is a common feature in statically typed languages like C or C++. Instead, Python’s lists can grow or shrink as elements are added or removed, providing a high level of versatility in data handling.
While it is not possible to allocate a list’s length statically in Python, developers can initialize a list with a predefined size filled with default values if necessary. This can be done using list comprehensions or the multiplication operator, which creates a list with a specific number of elements. However, this method does not prevent the list from being modified later, as Python lists remain inherently dynamic.
In summary, the dynamic nature of Python lists is one of the language’s strengths, allowing for flexible data structures that can adapt to varying requirements. Understanding this characteristic is crucial for effective programming in Python, as it influences how data is stored and manipulated. Developers should embrace this dynamic behavior and utilize it to their advantage, rather than attempting to impose static constraints on list
Author Profile
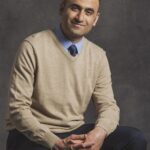
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?