Can We Use an Else Clause with a While Loop in Python?
When diving into the world of Python programming, you quickly discover that loops are fundamental constructs that allow for efficient code execution. Among the various looping mechanisms, the `while` loop stands out for its flexibility and control. However, many programmers, especially those new to Python, often overlook a fascinating feature that can enhance the functionality of their loops: the `else` clause. This intriguing addition to the `while` loop can lead to cleaner, more readable code and can help manage complex conditions with ease. But can we really use `else` with a `while` loop in Python? The answer may surprise you!
In Python, the `else` clause can indeed be used in conjunction with `while` loops, and understanding how it works can unlock new possibilities in your coding toolkit. The `else` block executes after the `while` loop concludes, but only if the loop was not terminated by a `break` statement. This unique behavior allows developers to handle scenarios where a loop completes naturally versus when it is interrupted, providing a nuanced approach to flow control.
As we explore this concept further, we will delve into the mechanics of using `else` with `while` loops, illustrating its practical applications through examples. Whether you are looking to refine your coding skills
Understanding the Else Clause in While Loops
In Python, the `else` clause can indeed be utilized with `while` loops, which may be surprising to those familiar with other programming languages. The `else` block executes after the loop completes normally, meaning it runs when the loop’s condition becomes . However, it does not execute if the loop is terminated prematurely using a `break` statement.
How the Else Clause Works
The general structure of a `while` loop with an `else` clause is as follows:
“`python
while condition:
Loop body
if some_condition:
break
else:
Else block
“`
In this example:
- If the `while` loop completes without hitting a `break`, the code within the `else` block will execute.
- If the `break` statement is encountered, the `else` block is skipped.
Practical Example
Consider the following code snippet that demonstrates the use of an `else` clause with a `while` loop:
“`python
count = 0
while count < 5:
print(count)
count += 1
else:
print("Loop completed without interruption.")
```
Output:
“`
0
1
2
3
4
Loop completed without interruption.
“`
In this case, since the loop completes normally, the message indicating the completion of the loop is printed.
Using Break with Else
To illustrate the behavior when using `break`, consider the following example:
“`python
count = 0
while count < 5:
if count == 3:
break
print(count)
count += 1
else:
print("Loop completed without interruption.")
```
Output:
“`
0
1
2
“`
Here, when `count` equals 3, the loop is exited via the `break` statement, and the `else` block is not executed.
When to Use Else with While Loops
The `else` clause is particularly useful in scenarios where you need to differentiate between a normal loop completion and an early exit due to a break. Here are some use cases:
- Searching for an element in a collection where you want to inform whether the search was successful or not.
- Implementing algorithms where a condition should trigger different flows of execution.
Scenario | Behavior |
---|---|
Normal Completion | Else executes after the loop |
Break Statement Encountered | Else does not execute |
utilizing the `else` clause with `while` loops can enhance code readability and provide clearer control flow in scenarios where the distinction between normal termination and early exit is significant.
Using Else with While Loop in Python
In Python, it is indeed possible to use an `else` statement with a `while` loop. The `else` block will execute after the `while` loop finishes its execution, but it will not run if the loop is terminated by a `break` statement. This feature can be useful for executing code that should run only if the loop completed without interruptions.
How the Else Clause Works
The basic structure of a `while` loop with an `else` clause is as follows:
“`python
while condition:
Loop body
if some_condition:
break Exit the loop
else:
This block executes if the loop is not terminated by a break
“`
Key Points to Remember:
- The `else` block runs when the loop condition becomes .
- If the loop is exited using `break`, the `else` block will be skipped.
- This construct can help clarify the flow of the program by explicitly handling the case where the loop completes normally.
Example of While Loop with Else
Consider the following example that demonstrates the use of an `else` statement with a `while` loop:
“`python
counter = 0
while counter < 5:
print("Counter:", counter)
counter += 1
else:
print("Loop completed without interruption.")
```
Output:
“`
Counter: 0
Counter: 1
Counter: 2
Counter: 3
Counter: 4
Loop completed without interruption.
“`
In this example, the loop increments the `counter` variable until it reaches 5, at which point the `else` block executes.
Example with Break Statement
To illustrate how the `else` clause behaves when a `break` is used, consider the following code:
“`python
counter = 0
while counter < 5:
print("Counter:", counter)
if counter == 3:
break Exit the loop when counter is 3
counter += 1
else:
print("Loop completed without interruption.")
```
Output:
“`
Counter: 0
Counter: 1
Counter: 2
Counter: 3
“`
In this case, since the loop is terminated by the `break` statement when `counter` equals 3, the `else` block does not execute.
Use Cases for Else in While Loops
The `else` clause in a `while` loop is particularly useful in scenarios such as:
- Searching: To indicate that a search was unsuccessful.
- Validation: To confirm that a set of conditions was met without early exits.
- Resource Management: To ensure that resources are released only if the loop completes normally.
Use Case | Description |
---|---|
Searching | Check if an item was found or not |
Validation | Confirm all conditions were satisfied |
Resource Cleanup | Release resources if the loop didn’t break early |
Using the `else` clause effectively can enhance code readability and help maintain a clear control flow in programs.
Understanding the Use of Else with While Loops in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, it is indeed possible to use an else clause with a while loop. This feature allows developers to execute a block of code once the loop condition becomes , which can be particularly useful for handling scenarios where a loop is terminated normally, rather than by a break statement.”
Michael Chen (Software Engineer, CodeCraft Solutions). “The else clause in a while loop can enhance code readability by clearly defining what should happen after the loop completes its iterations. This is especially beneficial in complex algorithms where distinguishing between normal termination and early exits is crucial for maintaining logical flow.”
Sarah Thompson (Python Educator, LearnPython.org). “While many programmers may overlook the else clause when using while loops, it serves as a powerful tool in Python. It allows for cleaner code and can reduce the need for additional flags or checks, making it easier to understand the intended flow of the program.”
Frequently Asked Questions (FAQs)
Can we use else with a while loop in Python?
Yes, Python allows the use of an else clause with a while loop. The else block executes when the while loop terminates normally, meaning it did not encounter a break statement.
What is the purpose of the else clause in a while loop?
The else clause in a while loop is used to define a block of code that will run after the loop completes its iterations, provided that the loop was not exited prematurely with a break statement.
Can you provide an example of a while loop with an else clause?
Certainly. Here’s an example:
“`python
count = 0
while count < 5:
print(count)
count += 1
else:
print("Loop finished without interruption.")
```
In this example, the else block executes after the loop completes.
What happens if a break statement is used in a while loop with an else clause?
If a break statement is encountered within the while loop, the else block will not execute. The else block only runs if the loop concludes naturally without interruption.
Is the else clause mandatory in a while loop?
No, the else clause is optional in a while loop. You can choose to use it based on your specific needs for handling post-loop logic.
Are there any performance implications of using else with a while loop?
There are no significant performance implications of using an else clause with a while loop. The choice to use it should be based on code clarity and logical structure rather than performance concerns.
In Python, it is indeed possible to use an `else` clause with a `while` loop. The `else` block is executed when the loop terminates naturally, meaning it exits without encountering a `break` statement. This feature is somewhat unique to Python and can be useful for indicating that the loop has completed its iterations without interruption.
The primary function of the `else` clause in a `while` loop is to provide a clear, logical separation between the loop’s execution and the code that follows it. This can enhance code readability and maintainability, as it allows developers to handle the completion of the loop distinctly from any early exits that may occur due to `break` statements. This can be particularly beneficial in scenarios where the loop is searching for a specific condition or value.
In summary, the use of an `else` statement with a `while` loop in Python is a valid and useful construct. It allows for clearer control flow and can improve the overall structure of the code. Understanding how to leverage this feature can lead to more efficient and readable programming practices in Python.
Author Profile
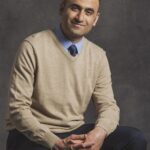
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?