Can You Append Multiple Items at Once in Python? Here’s What You Need to Know!
In the world of Python programming, efficiency and elegance are paramount, especially when it comes to managing collections of data. One common task that developers face is the need to add multiple items to a list or other data structures. While Python offers a variety of methods for appending elements, understanding how to do this effectively can streamline your code and enhance performance. If you’ve ever wondered, “Can you append multiple items at once in Python?” you’re in the right place. This article will delve into the various techniques available for appending multiple items, showcasing their benefits and best practices.
Appending multiple items in Python can significantly reduce the amount of code you write and improve readability. Whether you’re dealing with lists, tuples, or other iterable collections, Python provides several built-in methods that allow you to add multiple elements in a single operation. This not only saves time but also minimizes the potential for errors that can arise from multiple append calls.
As we explore the different approaches to appending multiple items, we’ll highlight the nuances of each method, including performance considerations and scenarios where one technique may be more suitable than another. By the end of this article, you’ll have a comprehensive understanding of how to efficiently manage your data collections in Python, empowering you to write cleaner and more effective code.
Appending Multiple Items to a List
Appending multiple items to a list in Python can be accomplished using several methods. Each method has its advantages depending on the specific use case. Below are some of the most common techniques:
- Using the `extend()` Method: This method allows you to add elements from an iterable (like a list or a tuple) to the end of the list.
“`python
my_list = [1, 2, 3]
my_list.extend([4, 5, 6])
print(my_list) Output: [1, 2, 3, 4, 5, 6]
“`
- Using List Concatenation: You can concatenate two lists using the `+` operator. This creates a new list with the combined elements.
“`python
my_list = [1, 2, 3]
my_list = my_list + [4, 5, 6]
print(my_list) Output: [1, 2, 3, 4, 5, 6]
“`
- Using List Comprehension: This method is particularly useful when you need to apply some transformation to the items being added.
“`python
my_list = [1, 2, 3]
my_list += [x for x in range(4, 7)]
print(my_list) Output: [1, 2, 3, 4, 5, 6]
“`
- Using the `+=` Operator: This operator modifies the list in place, adding elements from the iterable.
“`python
my_list = [1, 2, 3]
my_list += [4, 5, 6]
print(my_list) Output: [1, 2, 3, 4, 5, 6]
“`
Performance Considerations
When appending multiple items, the performance may vary based on the method chosen. The following table outlines the typical performance implications of each method:
Method | Time Complexity | In-place Modification |
---|---|---|
extend() | O(k) where k is the number of elements | Yes |
List Concatenation (+) | O(n + k) where n is the size of the original list | No |
List Comprehension | O(k) | Yes |
+= Operator | O(k) | Yes |
In general, using `extend()` or the `+=` operator is preferred for appending multiple items due to their efficiency and in-place modification capability. However, the choice of method may also depend on the specific requirements of your application, such as the need for readability or the performance characteristics of the operations being performed.
Appending Multiple Items to a List in Python
In Python, there are several efficient methods to append multiple items to a list. The choice of method often depends on the specific requirements of your application, such as whether you are working with another list or other iterable types.
Using the `extend()` Method
The `extend()` method is designed specifically to append elements from an iterable to the end of a list. This method modifies the original list in place.
“`python
my_list = [1, 2, 3]
my_list.extend([4, 5, 6])
print(my_list) Output: [1, 2, 3, 4, 5, 6]
“`
- Pros:
- Efficient for adding elements from other lists or iterables.
- Maintains the order of items.
- Cons:
- Does not return a new list; it modifies the existing one.
Using the `+=` Operator
The `+=` operator can also be utilized to append multiple items from an iterable to a list. It functions similarly to `extend()`.
“`python
my_list = [1, 2, 3]
my_list += [4, 5, 6]
print(my_list) Output: [1, 2, 3, 4, 5, 6]
“`
- Pros:
- Concise syntax.
- In-place modification of the original list.
- Cons:
- Like `extend()`, it does not create a new list.
Using List Concatenation
List concatenation allows you to combine multiple lists into a new list. This approach is useful when you want to maintain the original lists unchanged.
“`python
list1 = [1, 2, 3]
list2 = [4, 5, 6]
combined_list = list1 + list2
print(combined_list) Output: [1, 2, 3, 4, 5, 6]
“`
- Pros:
- Creates a new list, leaving the original lists intact.
- Cons:
- Less memory efficient for large lists as it creates a new list.
Using the `append()` Method in a Loop
If the items to be added are not in a single iterable, you can use a loop with the `append()` method.
“`python
my_list = [1, 2, 3]
for item in [4, 5, 6]:
my_list.append(item)
print(my_list) Output: [1, 2, 3, 4, 5, 6]
“`
- Pros:
- Flexible, as it allows for custom logic during appending.
- Cons:
- Less efficient for large numbers of items due to repeated method calls.
Using List Comprehension
For creating a new list with multiple items, list comprehension provides a concise way to append items.
“`python
my_list = [1, 2, 3]
new_items = [x for x in range(4, 7)]
combined_list = my_list + new_items
print(combined_list) Output: [1, 2, 3, 4, 5, 6]
“`
- Pros:
- Highly readable and allows for inline processing of data.
- Cons:
- May be less clear for complex operations.
Performance Considerations
When deciding which method to use, consider the following factors:
Method | In-Place Modification | Returns New List | Time Complexity |
---|---|---|---|
`extend()` | Yes | No | O(k) |
`+=` | Yes | No | O(k) |
Concatenation | No | Yes | O(n + k) |
Loop with `append()` | Yes | No | O(k) |
List Comprehension | No | Yes | O(n + k) |
Selecting the appropriate method depends on whether you prioritize performance, memory efficiency, or the need for immutability of the original list.
Expert Insights on Appending Multiple Items in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, you can efficiently append multiple items to a list using the `extend()` method. This method allows you to add elements from another iterable, making it a powerful tool for bulk additions.”
Michael Chen (Python Developer and Author, Coding Simplified). “While the `append()` method adds a single item to a list, using `+=` or `extend()` is preferable for appending multiple items at once. This approach not only enhances performance but also improves code readability.”
Sarah Lopez (Data Scientist, Analytics Hub). “For appending multiple items, consider using list comprehensions or the `itertools.chain()` function for more complex scenarios. These methods provide flexibility and can handle various data structures seamlessly.”
Frequently Asked Questions (FAQs)
Can you append multiple items at once in Python?
Yes, you can append multiple items at once in Python using the `extend()` method of a list, which allows you to add elements from an iterable.
What is the difference between `append()` and `extend()` in Python?
The `append()` method adds a single element to the end of a list, while `extend()` adds multiple elements from an iterable, effectively concatenating the iterable to the list.
How do you use the `+=` operator to append multiple items in Python?
You can use the `+=` operator to extend a list by adding elements from another iterable. This operator modifies the original list in place.
Is it possible to append multiple items using a loop in Python?
Yes, you can use a loop to append multiple items individually to a list using the `append()` method, but this is less efficient than using `extend()` or `+=`.
Can you append items from a different data type to a list in Python?
Yes, you can append items from different data types to a list in Python, as lists can contain mixed data types. However, when using `extend()`, ensure the argument is an iterable.
What happens if you try to append a list to another list using `append()`?
If you use `append()` to add a list to another list, the entire list will be added as a single element, resulting in a nested list. Use `extend()` to concatenate the elements instead.
In Python, the ability to append multiple items to a list at once is a common requirement for developers. While the traditional `append()` method allows for adding a single item to a list, Python provides alternative methods such as `extend()` and list concatenation that enable the addition of multiple elements simultaneously. Understanding these methods is essential for efficient list manipulation and can significantly enhance code performance and readability.
The `extend()` method is particularly useful when you need to add elements from an iterable, such as another list or a tuple, to an existing list. This method modifies the original list in place and can handle various data types, making it versatile for different programming scenarios. Alternatively, using the `+=` operator or the `list1 + list2` syntax allows for combining lists without altering the original lists, providing flexibility in managing data structures.
In summary, Python offers several efficient ways to append multiple items to a list, including `extend()`, list concatenation, and the `+=` operator. By leveraging these methods, developers can write cleaner and more efficient code, ultimately improving the functionality and performance of their applications. Mastery of these techniques is crucial for anyone looking to enhance their Python programming skills.
Author Profile
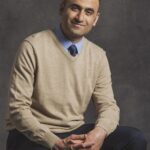
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?