Can You Index a Dictionary in Python? Exploring Key Concepts and Techniques!
In the world of programming, particularly when working with Python, dictionaries stand out as one of the most versatile and powerful data structures. They allow developers to store and manipulate data in a way that is both intuitive and efficient. But as you dive deeper into the capabilities of dictionaries, you might find yourself wondering: can you index a dictionary in Python? This question opens the door to a rich exploration of how dictionaries function, their unique characteristics, and the various methods you can employ to access and manipulate the data they contain.
Dictionaries in Python are designed to hold key-value pairs, making them ideal for situations where you need to associate specific data with unique identifiers. Unlike lists or tuples, which are indexed by their position in the sequence, dictionaries use keys as their indexing mechanism. This fundamental difference not only enhances the flexibility of data retrieval but also introduces a new layer of complexity when it comes to understanding how to effectively navigate and utilize dictionaries in your code.
As we delve into the intricacies of dictionary indexing, we’ll explore the various ways you can access values, modify entries, and even handle scenarios where keys may not exist. Whether you’re a novice programmer seeking to grasp the basics or an experienced coder looking to refine your skills, understanding how to index a dictionary in Python is
Understanding Dictionary Indexing
In Python, dictionaries are inherently unordered collections of items that store key-value pairs. Unlike lists or tuples, dictionaries do not support indexing based on integer positions. Instead, they utilize keys to access associated values. Each key within a dictionary must be unique and immutable, enabling rapid access to values based on their corresponding keys.
To illustrate, here is a basic example of a dictionary:
“`python
my_dict = {
“name”: “Alice”,
“age”: 30,
“city”: “New York”
}
“`
To access values in a dictionary, you can use the key directly. For instance:
“`python
print(my_dict[“name”]) Output: Alice
“`
Attempting to use an integer index, as one would with a list, will result in a `TypeError`. This is because dictionary keys can be of various types, including strings, integers, and tuples, but they cannot be accessed via positional indexing.
Accessing and Modifying Dictionary Values
Accessing and modifying values in a dictionary is straightforward. You can retrieve a value using its key and update it using the assignment operator.
- Accessing a value: Use the key within square brackets.
- Modifying a value: Assign a new value to an existing key.
Example:
“`python
Access
age = my_dict[“age”]
print(age) Output: 30
Modify
my_dict[“age”] = 31
print(my_dict[“age”]) Output: 31
“`
In cases where you attempt to access a key that does not exist, a `KeyError` will be raised. To avoid this, you can use the `get()` method, which returns `None` or a specified default value if the key is missing.
“`python
height = my_dict.get(“height”, “Not specified”)
print(height) Output: Not specified
“`
Iterating Over a Dictionary
Dictionaries support various methods that allow you to iterate over their keys, values, or both. The most commonly used methods are:
- `keys()`: Returns a view of the dictionary’s keys.
- `values()`: Returns a view of the dictionary’s values.
- `items()`: Returns a view of the dictionary’s key-value pairs.
Example of iterating through a dictionary:
“`python
for key, value in my_dict.items():
print(f”{key}: {value}”)
“`
Dictionary Comprehensions
Python also supports dictionary comprehensions, enabling you to create dictionaries in a concise manner. The syntax follows the pattern:
“`python
new_dict = {key: value for key, value in iterable}
“`
For example, creating a dictionary from a list of tuples:
“`python
pairs = [(“name”, “Bob”), (“age”, 25)]
new_dict = {key: value for key, value in pairs}
“`
This results in the following dictionary:
“`python
{“name”: “Bob”, “age”: 25}
“`
Method | Description |
---|---|
keys() | Returns all keys in the dictionary |
values() | Returns all values in the dictionary |
items() | Returns all key-value pairs in the dictionary |
In summary, while dictionaries do not support traditional indexing, they provide efficient ways to access and manipulate data through keys, making them a powerful tool in Python programming.
Understanding Dictionary Indexing in Python
In Python, dictionaries are collections of key-value pairs. Unlike lists or tuples, dictionaries do not support indexing based on the position of elements. Instead, you access values through their corresponding keys. This unique approach provides efficiency and flexibility when handling data.
Accessing Values by Keys
To retrieve a value from a dictionary, you simply use the key within square brackets or the `get()` method. Here’s how both methods work:
“`python
Example dictionary
my_dict = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
Accessing value using square brackets
name = my_dict[‘name’]
Accessing value using get() method
age = my_dict.get(‘age’)
“`
- Square Bracket Method: This method raises a `KeyError` if the key does not exist.
- Get Method: This method returns `None` (or a specified default value) if the key is absent, providing a safer way to access values.
Checking for Key Existence
Before accessing a value, it is prudent to check if a key exists within the dictionary. This can be achieved using the `in` keyword.
“`python
if ‘city’ in my_dict:
print(my_dict[‘city’])
“`
This approach prevents runtime errors and allows for more robust code.
Iterating Over a Dictionary
You can iterate over dictionaries using loops, allowing you to access keys, values, or both. The following examples illustrate various methods of iteration:
“`python
Iterating over keys
for key in my_dict:
print(key)
Iterating over values
for value in my_dict.values():
print(value)
Iterating over key-value pairs
for key, value in my_dict.items():
print(f”{key}: {value}”)
“`
- Keys: Use the dictionary directly in the loop.
- Values: Use the `values()` method to obtain all values.
- Key-Value Pairs: Use the `items()` method for simultaneous access to keys and values.
Modifying a Dictionary
Dictionaries are mutable, meaning you can modify them after creation. You can add, update, or remove entries as needed:
- Adding/Updating a Key-Value Pair:
“`python
my_dict[‘occupation’] = ‘Engineer’ Adds a new key
my_dict[‘age’] = 31 Updates existing key
“`
- Removing a Key-Value Pair:
“`python
del my_dict[‘city’] Removes the key ‘city’
“`
- Using the `pop()` Method:
“`python
age = my_dict.pop(‘age’) Removes ‘age’ and returns its value
“`
Dictionary Comprehensions
Python also supports dictionary comprehensions, which provide a concise way to create dictionaries. They are particularly useful for transforming or filtering data.
“`python
squared_dict = {x: x**2 for x in range(5)}
“`
This example generates a dictionary where each key is a number and each value is its square.
In summary, while dictionaries in Python do not support traditional indexing like lists, they offer versatile methods for accessing, modifying, and iterating through key-value pairs. Understanding these operations is essential for effective data management in Python programming.
Understanding Dictionary Indexing in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, dictionaries are inherently unordered collections of key-value pairs, which means you cannot index them in the same way you would with a list. However, you can access values using their corresponding keys, making dictionaries highly efficient for lookups.”
James Liu (Data Scientist, Analytics Solutions Group). “While you cannot directly index a dictionary like a list, you can retrieve values through their keys. This key-based access is a fundamental feature of dictionaries in Python, allowing for quick data retrieval and manipulation.”
Sarah Thompson (Software Engineer, CodeCraft Labs). “It is crucial to understand that dictionaries do not support indexing by position. Instead, they utilize hash tables for key access, which provides constant time complexity for lookups, making them an essential data structure for many applications in Python.”
Frequently Asked Questions (FAQs)
Can you index a dictionary in Python?
No, dictionaries in Python do not support indexing like lists or tuples. Instead, dictionaries use keys to access their values.
What is the correct way to access a value in a dictionary?
To access a value in a dictionary, use the syntax `dictionary[key]`, where `key` is the specific key associated with the desired value.
Can you iterate over a dictionary in Python?
Yes, you can iterate over a dictionary using loops. Common methods include iterating through keys, values, or key-value pairs using `for key in dictionary`, `for value in dictionary.values()`, or `for key, value in dictionary.items()`.
What happens if you try to access a non-existent key in a dictionary?
If you attempt to access a non-existent key, Python raises a `KeyError`. To avoid this, you can use the `get()` method, which returns `None` or a specified default value if the key is not found.
Are dictionary keys ordered in Python?
Yes, as of Python 3.7, dictionaries maintain the insertion order of keys. This means that when you iterate over a dictionary, the keys will be returned in the order they were added.
Can you use mutable types as dictionary keys?
No, mutable types such as lists or dictionaries cannot be used as keys in a dictionary. Only immutable types like strings, numbers, and tuples can serve as keys.
In Python, dictionaries are versatile data structures that store key-value pairs. Unlike lists or tuples, dictionaries do not support indexing in the traditional sense because they are unordered collections. Instead of accessing elements by their position, you retrieve values using their associated keys. This distinction is crucial for understanding how to effectively work with dictionaries in Python.
To access a value in a dictionary, you simply use the key within square brackets or the `get()` method. This allows for efficient retrieval of data without the need for indexing. Additionally, dictionaries provide methods for iterating over keys, values, or key-value pairs, which further enhances their usability in various programming scenarios.
In summary, while you cannot index a dictionary in the conventional way, the ability to access values through keys offers a powerful alternative. Understanding this concept is essential for leveraging dictionaries effectively in Python programming, enabling developers to manage and manipulate data with ease.
Author Profile
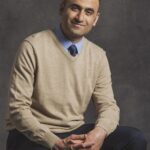
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?