How to Resolve the ‘Cannot Convert java.lang.String to JSON Object’ Error in Kotlin?
In the world of modern software development, the ability to seamlessly manipulate data formats is crucial for building robust applications. Kotlin, a powerful language that runs on the Java Virtual Machine, has gained immense popularity among developers for its concise syntax and interoperability with Java. However, as with any programming language, developers often encounter challenges, particularly when it comes to data conversion. One common issue that many Kotlin developers face is the error message: “cannot convert java.lang.String to JSON object.” This seemingly simple problem can lead to frustration, especially when working with APIs or handling complex data structures. In this article, we will explore the intricacies of this error, its underlying causes, and effective strategies to resolve it, ensuring that your Kotlin applications can handle JSON data with ease.
When working with JSON data in Kotlin, developers frequently rely on libraries such as Gson or Moshi to parse and serialize JSON objects. However, the transition from a raw string representation of JSON to a structured JSON object can sometimes lead to confusion. This is especially true when the data being processed is not formatted correctly or when the expected data types do not align. Understanding the nuances of JSON parsing and the specific error messages that arise can significantly enhance your debugging skills and improve your overall coding experience.
In the following sections,
Understanding the Error
When you encounter the error `cannot convert java.lang.String to JSONObject` in Kotlin, it typically indicates an issue with type conversion when working with JSON data. This error arises when you attempt to treat a plain string as a JSON object without properly parsing it. In Kotlin, as in Java, strings must be parsed before being manipulated as JSON.
Common Causes
Several factors can lead to this error:
- Improper Parsing: Attempting to use a string directly as a JSON object without converting it using a JSON library.
- Incorrect Library Usage: Using a library that does not correctly handle string to JSON conversion.
- Malformed JSON: The string may not be a valid JSON format, leading to parsing failures.
Solutions
To resolve this issue, you should ensure that the string is correctly parsed into a JSON object. Below are the common methods to achieve this:
- Using JSONObject: If you are using the `org.json` library, you can create a `JSONObject` from a string as follows:
“`kotlin
val jsonString = “{\”key\”:\”value\”}” // A valid JSON string
val jsonObject = JSONObject(jsonString)
“`
- Using Gson: With the Gson library, you can convert a string to a JSON object like this:
“`kotlin
val jsonString = “{\”key\”:\”value\”}”
val gson = Gson()
val jsonObject = gson.fromJson(jsonString, JsonObject::class.java)
“`
- Using Kotlin Serialization: If you are using Kotlin’s built-in serialization, make sure to use the appropriate methods:
“`kotlin
import kotlinx.serialization.json.Json
import kotlinx.serialization.json.JsonObject
val jsonString = “{\”key\”:\”value\”}”
val jsonObject = Json.parseToJsonElement(jsonString).jsonObject
“`
Best Practices
To avoid this error in the future, consider the following best practices:
- Always Validate JSON: Before parsing, ensure that the string is a valid JSON format.
- Use Try-Catch Blocks: Implement error handling to manage exceptions that may arise during parsing.
- Utilize Libraries: Leverage libraries such as Gson or Kotlin Serialization that simplify JSON manipulation.
Example Table of JSON Libraries
Library | Usage | Parsing Method |
---|---|---|
org.json | Basic JSON operations | JSONObject(jsonString) |
Gson | Convert JSON to/from Kotlin objects | gson.fromJson(jsonString, Class::class.java) |
Kotlin Serialization | Kotlin-native serialization | Json.parseToJsonElement(jsonString) |
Understanding the Error
The error message `cannot convert java.lang.String to JSONObject` typically arises when attempting to parse a JSON string in Kotlin but encountering issues with the format or content of the string. This can occur for several reasons, including:
- Improper JSON Format: The string does not conform to the JSON format, such as missing brackets or quotes.
- Incorrect Data Type: The variable expected to be a JSON object is actually a plain string.
- Serialization Issues: Problems during the serialization or deserialization process can lead to this error.
Common Causes
Identifying the root cause of this error can often resolve it quickly. Here are the common scenarios:
- Malformed JSON: Ensure that the string you are trying to convert is valid JSON. For example:
- Valid JSON: `{“key”: “value”}`
- Invalid JSON: `{key: value}` or `{“key”: “value”,}` (trailing comma)
- Using `JSONObject` on a Plain String: Ensure that the string you are passing to `JSONObject` is a properly formatted JSON. For instance:
“`kotlin
val jsonString = “{\”key\”:\”value\”}”
val jsonObject = JSONObject(jsonString) // Correct usage
“`
Validating JSON Strings
To ensure that your JSON string is valid before parsing, consider using the following methods:
- Online Validators: Use online tools like JSONLint or similar services to check your JSON structure.
- Kotlin Libraries: Utilize libraries such as `Gson` or `Moshi` that can help with parsing and provide better error messages.
Example of Correct Usage
Here is a simple example demonstrating how to correctly parse a JSON string in Kotlin using `JSONObject`:
“`kotlin
import org.json.JSONObject
fun main() {
val jsonString = “{\”name\”:\”John\”, \”age\”:30}”
try {
val jsonObject = JSONObject(jsonString)
println(“Name: ${jsonObject.getString(“name”)}”)
println(“Age: ${jsonObject.getInt(“age”)}”)
} catch (e: Exception) {
println(“Error parsing JSON: ${e.message}”)
}
}
“`
Handling Exceptions
When working with JSON parsing, it is crucial to handle exceptions gracefully. Use try-catch blocks to manage potential parsing errors:
- JSONException: This exception is thrown when the string cannot be converted to a JSONObject.
- NullPointerException: This may occur if you attempt to access a key that does not exist in the JSON object.
Example:
“`kotlin
try {
val jsonObject = JSONObject(jsonString)
// Access JSON data
} catch (e: JSONException) {
println(“JSON parsing error: ${e.message}”)
} catch (e: NullPointerException) {
println(“Accessed a non-existent key: ${e.message}”)
}
“`
By understanding the common causes and employing best practices in error handling, you can effectively avoid and resolve the `cannot convert java.lang.String to JSONObject` error in Kotlin. Ensure that your JSON strings are valid, utilize appropriate libraries, and implement robust exception handling to enhance your application’s reliability.
Understanding JSON Conversion Issues in Kotlin
Dr. Emily Carter (Software Architect, Tech Innovations Inc.). “The error ‘cannot convert java.lang.string to json object’ often arises when attempting to parse a string that does not conform to JSON format. It is crucial to validate the string before conversion to ensure it is properly structured as JSON.”
Michael Tran (Lead Kotlin Developer, CodeCraft Solutions). “In Kotlin, using libraries like Gson or Moshi can simplify the conversion process. However, developers must ensure that the input string is correctly formatted. Debugging the input string can often reveal hidden issues that lead to this conversion error.”
Sarah Liu (Senior Mobile Developer, AppDynamics). “Handling JSON in Kotlin requires a clear understanding of data types and structures. If you encounter the ‘cannot convert java.lang.string to json object’ error, consider checking the data type being passed and ensure it matches the expected JSON format.”
Frequently Asked Questions (FAQs)
What does the error “cannot convert java.lang.string to json object” mean in Kotlin?
This error indicates that a string is being incorrectly treated as a JSON object. It usually occurs when attempting to parse a string that is not in valid JSON format.
How can I properly convert a string to a JSON object in Kotlin?
To convert a string to a JSON object in Kotlin, ensure the string is formatted correctly as JSON. Use libraries like Gson or Moshi to parse the string. For example, with Gson: `val jsonObject = Gson().fromJson(jsonString, JsonObject::class.java)`.
What are common causes of this error when working with JSON in Kotlin?
Common causes include malformed JSON strings, missing quotes, or incorrect data types. Ensure the JSON string adheres to the proper syntax and structure.
Can I use Kotlin’s built-in libraries to handle JSON conversion?
Kotlin does not have built-in JSON parsing libraries, but you can use third-party libraries like Gson, Moshi, or kotlinx.serialization for effective JSON handling.
How can I debug the “cannot convert java.lang.string to json object” error?
To debug, check the format of the JSON string being passed. Use logging to print the string before parsing, and validate it using online JSON validators to ensure it is correctly structured.
Is there a way to handle JSON exceptions in Kotlin gracefully?
Yes, wrap your JSON parsing code in a try-catch block to handle exceptions. This allows you to manage errors without crashing the application, providing a fallback or error message to the user.
The error message “cannot convert java.lang.String to JSONObject” in Kotlin typically arises when attempting to parse a string that is not in the correct JSON format. This issue often occurs when developers try to create a JSONObject from a plain string that does not adhere to the expected JSON structure, such as missing curly braces or improperly formatted key-value pairs. Understanding the requirements for valid JSON is crucial for avoiding this error and ensuring successful data manipulation.
To resolve this issue, developers should first validate the string to ensure it is properly formatted as JSON. This can involve checking for the presence of necessary characters such as braces, brackets, and quotation marks. Additionally, using libraries like Gson or Moshi can simplify the process of converting strings to JSON objects, as these libraries provide robust methods for serialization and deserialization while handling various edge cases.
Moreover, error handling is essential when working with JSON parsing in Kotlin. Implementing try-catch blocks can help gracefully manage exceptions that may arise during the conversion process. By logging errors and providing user-friendly feedback, developers can enhance the robustness of their applications and improve the overall user experience.
addressing the “cannot convert java.lang.String to JSONObject” error requires a thorough understanding of JSON formatting,
Author Profile
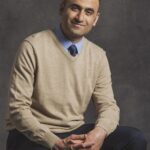
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?