Why Am I Seeing the Error ‘Cannot Read Properties of Undefined (Reading ‘0’)’?
In the world of web development and programming, encountering errors is an inevitable part of the journey. One such error that can leave developers scratching their heads is the infamous “cannot read properties of reading ‘0’.” This cryptic message often appears when working with JavaScript, particularly when dealing with arrays or objects, and can be a source of frustration for both novice and seasoned coders alike. Understanding the nuances of this error is crucial for debugging and enhancing your coding skills, as it not only highlights potential pitfalls in your code but also offers a glimpse into the intricacies of how JavaScript handles data structures.
When you see the “cannot read properties of ” error, it typically indicates that your code is attempting to access a property or element of a variable that hasn’t been properly defined or initialized. This often occurs in scenarios where you expect an array or object to be populated with data, but instead, you encounter an value. The error message’s reference to ‘0’ usually signifies an attempt to access the first element of an array, which can lead to confusion if you’re unaware of the underlying issue.
Understanding the root causes of this error is essential for effective debugging. It can stem from various situations, such as asynchronous data fetching, incorrect variable scoping
Understanding the Error
The error message “cannot read properties of (reading ‘0’)” typically occurs in JavaScript when attempting to access a property or index of an object or array that is not defined. This can happen for various reasons, including:
- Attempting to access an element of an array that hasn’t been initialized.
- Calling a method on an object that hasn’t been instantiated.
- Expecting a function to return an array but encountering an “ instead.
To better understand this error, it is essential to recognize the scenarios where it might arise and how to debug them effectively.
Common Scenarios Leading to the Error
- Accessing an Array Element: If you try to access an index in an array that hasn’t been defined, JavaScript will throw this error.
“`javascript
let arr;
console.log(arr[0]); // Error: cannot read properties of (reading ‘0’)
“`
- Function Returns: If a function is expected to return an array but instead returns “, accessing any index will result in this error.
“`javascript
function getArray() {
// Missing return statement
}
let myArray = getArray();
console.log(myArray[0]); // Error: cannot read properties of (reading ‘0’)
“`
- Object Properties: Attempting to access a property of an object that is not defined can also lead to this issue.
“`javascript
let obj;
console.log(obj.property[0]); // Error: cannot read properties of (reading ‘0’)
“`
Debugging Strategies
To resolve the “cannot read properties of (reading ‘0’)” error, consider the following strategies:
– **Initialize Variables**: Always ensure that variables are initialized before accessing their properties or indices.
– **Check Function Returns**: Verify that functions return the expected data type and handle cases where they may return “.
– **Use Optional Chaining**: Optional chaining (?.) can be used to safely access deeply nested properties without risking an error.
“`javascript
let safeAccess = obj?.property?.[0]; // Returns instead of throwing an error
“`
– **Add Validations**: Implement checks before accessing properties or indices to confirm that the variable is defined.
“`javascript
if (arr && arr.length > 0) {
console.log(arr[0]);
}
“`
Best Practices to Avoid the Error
To prevent encountering this error in the future, consider adopting the following best practices:
- Consistent Initialization: Always initialize arrays and objects when declaring them.
- Clear Function Contracts: Clearly define the expected return types of functions and ensure they adhere to these contracts.
- Error Handling: Use try-catch blocks to handle potential errors gracefully.
- Linting Tools: Utilize linting tools to catch potential issues during development. This can help identify uninitialized variables or incorrect assumptions about data structures.
Scenario | Error Cause | Resolution |
---|---|---|
Accessing an Array | Array not defined | Initialize the array |
Function Returns | Function returns | Ensure function returns an array |
Object Properties | Object is | Initialize the object before access |
Understanding the Error Message
The error message “cannot read properties of (reading ‘0’)” typically occurs in JavaScript when attempting to access an index of an array or a property of an object that is currently . This situation arises due to various coding issues, including:
- Uninitialized Variables: Accessing a variable that has not been assigned a value.
- Incorrect Object References: Trying to access a property of an object that does not exist.
- Array Indexing Errors: Attempting to access an array index that is out of bounds or does not exist.
Common Scenarios and Solutions
Here are some prevalent scenarios where this error might occur, along with potential solutions:
Scenario | Cause | Solution |
---|---|---|
Accessing an uninitialized array | The array has not been defined or initialized. | Ensure the array is initialized before access. |
Accessing a property of an object | The object is or null. | Check if the object is defined before accessing its properties. |
Incorrect data structure assumptions | The expected structure of data does not match reality. | Validate the structure before accessing its properties. |
Debugging Techniques
To efficiently debug this error, consider the following techniques:
– **Console Logging**: Use `console.log()` to output the variable or object you are trying to access before the line that throws the error. This helps in understanding its state.
– **Type Checking**: Utilize `typeof` or `Array.isArray()` to ensure the variable is defined and of the expected type before accessing its properties.
– **Conditional Statements**: Implement checks to verify whether the object or array is defined and has the necessary properties before accessing them. Example:
“`javascript
if (myArray && myArray.length > 0) {
console.log(myArray[0]);
}
“`
Preventive Measures
To mitigate the chances of encountering this error, adopt these preventive measures:
- Initialize Variables: Always initialize variables and data structures before use.
- Use Optional Chaining: Utilize optional chaining (`?.`) to safely access nested properties, which prevents runtime errors if a reference is .
- Implement Default Values: Use default parameters in functions or fallback values when destructuring to ensure that variables are handled gracefully.
“`javascript
const { prop = ‘default’ } = someObject || {};
“`
Understanding the context in which “cannot read properties of (reading ‘0’)” occurs is crucial for effective debugging and error prevention. By implementing the outlined strategies, developers can enhance code robustness and minimize runtime errors associated with variables.
Understanding the ‘Cannot Read Properties of Reading ‘0” Error
Dr. Emily Carter (Senior Software Engineer, Tech Solutions Inc.). “The error ‘cannot read properties of reading ‘0” typically arises when attempting to access an index of an array or a property of an object that has not been properly initialized. It is crucial to ensure that the variable being accessed is defined and has the expected structure before attempting to read from it.”
Michael Chen (Lead JavaScript Developer, CodeCraft Co.). “In JavaScript, this error often indicates a flaw in the logic of the code. Developers should implement thorough checks or use optional chaining to prevent such errors, ensuring that the code gracefully handles cases where data may not be present.”
Sarah Thompson (Technical Writer, Dev Insights). “Understanding the context of the error is essential. It is not merely a coding oversight; it can also reflect issues in data fetching or asynchronous operations. Developers should trace their data flow and validate responses to avoid encountering this error in production.”
Frequently Asked Questions (FAQs)
What does the error “cannot read properties of reading ‘0’” mean?
This error indicates that the code is attempting to access the first element (index 0) of an array or object that is currently . It typically arises when a variable has not been initialized or assigned a value.
What are common causes of this error in JavaScript?
Common causes include attempting to access an array or object before it has been populated, using asynchronous code where the data has not yet been loaded, or mistakenly referencing a variable that has not been declared.
How can I troubleshoot this error?
To troubleshoot, check the variable that is causing the error to ensure it is defined before accessing its properties. Use console logging to inspect the variable’s value at runtime, and consider adding conditional checks to prevent accessing properties of .
What are some ways to prevent this error from occurring?
Prevent this error by initializing variables properly, using default values, and implementing error handling techniques such as try-catch blocks. Additionally, ensure that asynchronous data fetching is complete before accessing the data.
Can this error occur in frameworks or libraries?
Yes, this error can occur in frameworks or libraries, especially when dealing with state management or asynchronous data fetching. It is important to ensure that the data is available before attempting to access it within components.
How can I fix this error in my code?
To fix the error, identify the variable that is and ensure it is assigned a value before accessing its properties. You can also use optional chaining (e.g., `variable?.[0]`) to safely access properties without throwing an error if the variable is .
The error message “cannot read properties of reading ‘0’” typically occurs in JavaScript when attempting to access an element of an array or object that has not been defined. This situation often arises when developers try to access an index of an array or a property of an object that is either not initialized or has been set to . Understanding the context in which this error occurs is crucial for effective debugging and code maintenance.
Common causes of this error include attempting to access an array element before the array has been populated, or referencing an object property that does not exist. This can happen in asynchronous operations where data may not be available at the time of access. Additionally, it can stem from incorrect assumptions about the structure of data received from APIs or other external sources. Ensuring that variables are properly initialized and checking for values before accessing properties can help mitigate this issue.
To effectively handle this error, developers should implement robust error-checking mechanisms, such as using conditional statements to verify that variables are defined before accessing their properties. Utilizing optional chaining (?.) in modern JavaScript can also provide a way to safely navigate through nested objects without encountering this error. Ultimately, adopting best practices in coding and thorough testing can significantly reduce the likelihood of encountering
Author Profile
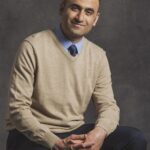
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?