Why Am I Seeing ‘Cannot Use Object of Type stdClass as Array’ Error in PHP?
In the world of PHP programming, encountering errors can be both frustrating and enlightening. One common error that developers face is the infamous “cannot use object of type stdClass as array.” This seemingly cryptic message often surfaces during the transition from object-oriented to array-based data handling, leaving many programmers scratching their heads. Understanding this error is crucial for anyone looking to harness the full power of PHP’s object-oriented features while maintaining flexibility in data manipulation. In this article, we will unravel the intricacies behind this error and provide insights into how to navigate the challenges it presents.
Overview
At its core, the “cannot use object of type stdClass as array” error arises when a developer mistakenly attempts to access properties of an object as if they were elements of an array. This confusion often stems from the dynamic nature of PHP, where both objects and arrays can be used to store and manipulate data. However, the syntax and methods for interacting with these two data types differ significantly, leading to potential pitfalls for the unwary.
As we delve deeper into the topic, we will explore the fundamental differences between objects and arrays in PHP, highlighting how to properly access and manipulate each type. By understanding the underlying principles that govern these data structures, developers can not only resolve this error
Understanding the Error Message
The error message “cannot use object of type stdclass as array” typically arises in PHP when you attempt to access properties of an object using array syntax. In PHP, objects and arrays are two distinct data types, and mixing them can lead to this common error.
When you retrieve data from a database or an API, it is often returned as an object of the `stdClass`. If you try to access its properties using the array syntax (`$object[‘property’]`), PHP will throw this error. Instead, you should use the object property access syntax (`$object->property`).
Common Scenarios Leading to the Error
Several scenarios can lead to encountering this error:
- Database Queries: When fetching results from a database using PDO or MySQLi, the results may be returned as objects. If you use array notation to access the data, this error will occur.
- API Responses: Many APIs return JSON data, which is often converted to an object in PHP. Accessing these properties incorrectly will trigger the error.
- Improper Type Assumptions: Assuming a variable is an array when it is actually an object can lead to this confusion.
Examples of Incorrect and Correct Access
Below is a comparison of incorrect and correct ways to access properties of an object.
Access Method | Example | Result |
---|---|---|
Incorrect (array syntax) | $value = $object[‘property’]; | Error: cannot use object of type stdclass as array |
Correct (object syntax) | $value = $object->property; | Value of property |
Debugging Tips
To effectively debug this issue, consider the following strategies:
- Check Data Types: Use `var_dump()` or `print_r()` to inspect the data type of the variable before accessing it.
- Review Data Sources: Ensure that the data being returned from your database or API is in the expected format.
- Use Type Casting: If you need to convert an object to an array, use the following syntax:
“`php
$array = (array) $object;
“`
This will allow you to access properties using array syntax without encountering the error.
Best Practices
To prevent this error from occurring in your code, adhere to these best practices:
- Always use the appropriate syntax for the data type you are working with.
- Maintain consistency in how you handle data returned from external sources.
- Document your code to clarify whether you expect an object or an array, aiding future maintenance and reducing confusion.
By following these guidelines, you can minimize the chances of encountering the “cannot use object of type stdclass as array” error in your PHP applications.
Understanding the Error
The error message “cannot use object of type stdClass as array” typically arises in PHP when an attempt is made to access an object as if it were an array. This happens when the object is an instance of the `stdClass`, which is a generic empty class in PHP.
Reasons for the Error
– **Incorrect Access Method**: Objects require property access via the arrow operator (`->`), while arrays use square brackets (`[]`).
- Data Type Mismatch: Attempting to treat an object as an array without appropriate conversion.
- Improper Data Handling: Failing to convert data from an external source, such as JSON, into the correct format.
Common Scenarios Leading to the Error
This error often surfaces in various coding scenarios:
– **JSON Decoding**: When JSON data is decoded into an object by default, and the developer attempts to access it as an array.
– **Database Fetching**: Fetching results from a database as an object and trying to use array notation.
Example Cases
Here are some typical examples that illustrate this error:
“`php
// Example 1: JSON Decode
$jsonString = ‘{“name”: “John”, “age”: 30}’;
$data = json_decode($jsonString); // Decodes to stdClass
// Incorrect access
echo $data[‘name’]; // Causes error
// Correct access
echo $data->name; // Correctly accesses the property
“`
“`php
// Example 2: Database Fetching
$result = $db->query(“SELECT * FROM users”);
$user = $result->fetch(PDO::FETCH_OBJ); // Fetches as stdClass
// Incorrect access
echo $user[‘username’]; // Causes error
// Correct access
echo $user->username; // Correctly accesses the property
“`
How to Resolve the Error
To fix the “cannot use object of type stdClass as array” error, you can follow several approaches:
– **Use Object Notation**: Always access properties of an object using the arrow (`->`) operator.
- Convert Object to Array: If array access is necessary, convert the object to an array.
Conversion Example
To convert an object to an array, you can use:
“`php
$arrayData = (array) $data; // Casts the object to an array
echo $arrayData[‘name’]; // Now this works
“`
Using JSON Decode with Associative Arrays
When decoding JSON, you can directly convert it to an associative array:
“`php
$data = json_decode($jsonString, true); // Pass true for associative array
echo $data[‘name’]; // Accessing as an array is now valid
“`
Best Practices
To avoid encountering this error in the future, consider the following best practices:
- Consistent Data Handling: Be aware of the data type you are working with and handle it consistently.
- Use Type Checking: Implement type checking before accessing variables to ensure proper data types.
- Error Handling: Use try-catch blocks to gracefully handle potential errors and exceptions.
Implementing these practices will enhance code reliability and reduce the occurrence of this error in your PHP applications.
Understanding the Error: “Cannot Use Object of Type stdClass as Array”
Dr. Emily Carter (Senior Software Engineer, Code Solutions Inc.). “The error ‘cannot use object of type stdClass as array’ typically arises in PHP when developers attempt to access object properties using array syntax. This common mistake can be avoided by ensuring that the correct object property access method, using the ‘->’ operator, is employed instead.”
Michael Thompson (PHP Framework Specialist, WebDev Insights). “When encountering the ‘cannot use object of type stdClass as array’ error, it’s crucial to understand the underlying data structure. Developers should utilize debugging tools to inspect the variable type and confirm whether they are dealing with an object or an array, thus preventing such type-related errors.”
Lisa Nguyen (Lead Backend Developer, Tech Innovations). “This error serves as a reminder of the importance of type handling in PHP. To resolve it effectively, developers should familiarize themselves with the differences between arrays and objects in PHP, and implement type checks or conversions where necessary to ensure compatibility.”
Frequently Asked Questions (FAQs)
What does the error “cannot use object of type stdClass as array” mean?
This error indicates that you are trying to access properties of an object as if it were an array. In PHP, objects of type `stdClass` should be accessed using the object operator (`->`) instead of array syntax (`[]`).
How can I fix the “cannot use object of type stdClass as array” error?
To resolve this error, ensure that you are using the correct syntax for accessing properties. Replace array access syntax with object access syntax, for example, use `$object->property` instead of `$object[‘property’]`.
What is the difference between an array and an object in PHP?
In PHP, an array is a collection of key-value pairs that can be accessed using either numeric or string keys, while an object is an instance of a class that encapsulates data and behavior. Objects are accessed using the `->` operator, whereas arrays use `[]`.
When might I encounter the “cannot use object of type stdClass as array” error?
You may encounter this error when you retrieve data from a database or an API that returns an object, and then mistakenly attempt to treat that object as an array in your code.
Can I convert a stdClass object to an array in PHP?
Yes, you can convert a `stdClass` object to an array using the `json_decode` function with the second parameter set to `true`, or by casting the object to an array using `(array) $object`.
What should I do if I need to work with both arrays and objects in my PHP code?
If you need to work with both data types, consistently use the appropriate syntax for each. Consider converting objects to arrays when necessary, or vice versa, depending on your specific use case and requirements.
The error message “cannot use object of type stdClass as array” typically arises in PHP when developers attempt to access properties of an object using array syntax. In PHP, stdClass is a generic empty class that is often used to create objects dynamically. When an object of this type is created, its properties must be accessed using the object operator (`->`), rather than the array syntax (`[]`). This fundamental distinction between objects and arrays is crucial for avoiding such errors in code.
To resolve this issue, developers should ensure that they are using the correct syntax when working with stdClass objects. Instead of trying to access the properties as if they were part of an array, the proper method is to utilize the object operator. For example, instead of writing `$object[‘property’]`, one should write `$object->property`. Understanding this difference is essential for effective PHP programming and helps prevent runtime errors that can disrupt application functionality.
Additionally, this error highlights the importance of understanding data types in PHP. Developers should familiarize themselves with the distinctions between arrays and objects, as well as the appropriate methods to manipulate each type. By doing so, they can write more robust and error-free code. Properly handling data types not only aids in debugging but also
Author Profile
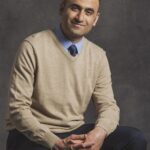
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?