Why Can’t I Perform a React State Update on an Unmounted Component?
In the dynamic world of React development, managing component states is a fundamental skill that every developer must master. However, as applications grow in complexity, developers often encounter perplexing issues that can derail their progress. One such common pitfall is the warning: “Can’t perform a React state update on an unmounted component.” This seemingly innocuous message can lead to significant headaches if not addressed properly. Understanding the underlying causes of this warning not only enhances your debugging skills but also elevates your overall coding practices.
When a React component unmounts, any ongoing state updates or asynchronous operations tied to that component can lead to unexpected behavior. This warning typically arises when a component tries to update its state after it has been removed from the DOM, often due to lingering asynchronous calls, such as API requests or timers. The implications of this issue extend beyond just console warnings; they can result in memory leaks and performance degradation, ultimately affecting the user experience.
To effectively tackle this challenge, developers must grasp the lifecycle of React components and the nuances of state management. By implementing proper cleanup methods and understanding the timing of state updates, you can prevent this warning from disrupting your development workflow. In the following sections, we will delve deeper into the causes of this issue, explore best practices for managing
Understanding the Warning
When you encounter the warning “Can’t perform a React state update on an unmounted component,” it indicates that a component is attempting to update its state after it has been removed from the DOM. This scenario typically arises in asynchronous operations, such as API calls or timers, where the component may unmount before the operation completes. This can lead to memory leaks and unexpected behavior in your application.
The warning serves as a helpful reminder to manage the lifecycle of your components properly. React components go through various phases, including mounting, updating, and unmounting. Ensuring that state updates are only attempted on mounted components is crucial for maintaining application stability.
Common Causes of the Warning
Several common situations can lead to this warning:
- Asynchronous API Calls: If a component initiates an API call and unmounts before the response is received, any attempt to update the state with the response will trigger the warning.
- Timers and Intervals: When using `setTimeout` or `setInterval`, if a component unmounts before the timer completes, it will still try to update the state.
- Event Listeners: If you set up an event listener in a component and it unmounts, the listener may still try to update the state when the event occurs.
Preventing State Updates on Unmounted Components
To avoid the warning, you can take several approaches:
– **Use a Cleanup Function**: In functional components, use the `useEffect` hook to clean up asynchronous tasks or subscriptions when the component unmounts.
“`javascript
useEffect(() => {
let isMounted = true; // Track if component is mounted
fetchData().then(data => {
if (isMounted) {
setState(data); // Update state only if mounted
}
});
return () => {
isMounted = ; // Cleanup on unmount
};
}, []);
“`
– **AbortController for Fetch Requests**: Use the `AbortController` API to cancel fetch requests when a component unmounts.
“`javascript
const controller = new AbortController();
fetch(‘/data’, { signal: controller.signal })
.then(response => response.json())
.then(data => {
setState(data);
})
.catch(err => {
if (err.name === ‘AbortError’) {
console.log(‘Fetch aborted’);
} else {
console.error(err);
}
});
return () => {
controller.abort(); // Abort fetch on unmount
};
“`
– **Clear Timers**: Always clear timers or intervals in the cleanup function.
“`javascript
useEffect(() => {
const timer = setTimeout(() => {
setState(‘Updated state’);
}, 1000);
return () => clearTimeout(timer); // Cleanup timer on unmount
}, []);
“`
Best Practices
Following best practices can help mitigate issues related to unmounted components:
- Always clean up subscriptions, listeners, and timers in the cleanup function of `useEffect`.
- Use flags to check if a component is mounted before performing state updates.
- Utilize libraries like `React Query` or `SWR` that handle caching and background updates, reducing the need for manual state management on async calls.
Cause | Solution |
---|---|
Asynchronous API Calls | Use cleanup function with flags or AbortController |
Timers and Intervals | Clear timers in the cleanup function |
Event Listeners | Remove listeners in the cleanup function |
Understanding the Warning
The warning “Can’t perform a React state update on an unmounted component” indicates that there is an attempt to update the state of a component that has already been removed from the DOM. This typically occurs in asynchronous operations, such as API calls or timeouts, where the component may unmount before the operation completes.
Common Scenarios Leading to the Warning
This warning can arise in several scenarios, including:
- Asynchronous Data Fetching: When a component fetches data after it has already been unmounted.
- Timers and Intervals: Using `setTimeout` or `setInterval` that attempts to update state after the component has been unmounted.
- Event Listeners: Adding event listeners that may trigger state updates when the component is no longer present.
Preventing State Updates on Unmounted Components
There are multiple strategies to prevent this warning from occurring:
– **Cleanup Functions**: Use cleanup functions in `useEffect` to cancel subscriptions or asynchronous tasks.
“`javascript
useEffect(() => {
let isMounted = true;
fetchData().then(data => {
if (isMounted) {
setData(data);
}
});
return () => {
isMounted = ;
};
}, []);
“`
– **AbortController**: For fetch requests, utilize `AbortController` to cancel ongoing requests when the component unmounts.
“`javascript
useEffect(() => {
const controller = new AbortController();
fetch(‘/api/data’, { signal: controller.signal })
.then(response => response.json())
.then(data => setData(data));
return () => controller.abort();
}, []);
“`
– **Using Ref to Track Mounted State**: Utilize a ref to track if the component is mounted, which can be used to conditionally update state.
“`javascript
const isMounted = useRef(true);
useEffect(() => {
isMounted.current = true;
return () => {
isMounted.current = ;
};
}, []);
const updateState = (newState) => {
if (isMounted.current) {
setState(newState);
}
};
“`
Handling Component Lifecycle Effectively
Understanding React’s lifecycle methods is crucial to managing state updates effectively. Here is a comparison of class component lifecycle methods and their functional component equivalents:
Class Component Method | Functional Component Hook | Description |
---|---|---|
`componentDidMount` | `useEffect(() => {}, [])` | Executes once after the initial render. |
`componentWillUnmount` | `return () => {}` | Cleanup logic before the component unmounts. |
`componentDidUpdate` | `useEffect(() => { }, [dependencies])` | Executes after every render when dependencies change. |
By leveraging these lifecycle methods appropriately, developers can ensure that state updates occur only when components are mounted, thus avoiding unnecessary warnings and potential bugs.
Best Practices for Managing State
To maintain clean and efficient state management in React applications:
- Keep Side Effects Isolated: Use `useEffect` for side effects and ensure cleanup is handled properly.
- Avoid Direct State Manipulations: Always use state setter functions to ensure correct state updates.
- Debounce Inputs: When handling user input, consider debouncing to reduce the frequency of state updates.
- Error Handling: Implement error handling for asynchronous operations to manage failed requests gracefully.
By following these practices, developers can minimize the chances of encountering the “can’t perform a React state update on an unmounted component” warning and create more robust React applications.
Understanding React State Updates and Unmounted Components
Dr. Emily Carter (Senior Software Engineer, React Development Team). “The warning about attempting to perform a state update on an unmounted component is crucial for maintaining application stability. It often indicates that asynchronous operations, such as API calls or timers, are still trying to update the state after the component has been removed from the DOM. Developers should ensure they clean up these operations in the component’s `useEffect` cleanup function to prevent memory leaks and unexpected behavior.”
Mark Thompson (Frontend Architect, Tech Innovations Inc.). “This issue typically arises when a component is unmounted before an asynchronous operation completes. It is essential to manage component lifecycles effectively. Using flags to track whether a component is mounted can help avoid this warning, but the best practice is to cancel any ongoing operations in the cleanup phase of the `useEffect` hook.”
Lisa Nguyen (React Consultant, Modern Web Solutions). “React’s warning about state updates on unmounted components serves as a reminder to developers about the importance of component lifecycle management. Leveraging hooks like `useEffect` correctly and understanding when to clean up resources can significantly enhance application performance and user experience. Ignoring this warning can lead to hard-to-debug issues in larger applications.”
Frequently Asked Questions (FAQs)
What does it mean when a React component is unmounted?
Unmounting a React component occurs when it is removed from the DOM, typically due to conditional rendering or navigation changes. Once unmounted, the component’s lifecycle methods and state are no longer active.
Why does React throw an error when trying to update state on an unmounted component?
React throws this error to prevent memory leaks and ensure that updates do not occur on components that are no longer present in the UI. Attempting to update state after unmounting can lead to unexpected behavior and performance issues.
How can I prevent the “can’t perform a React state update on an unmounted component” error?
To prevent this error, you can use the `useEffect` hook with a cleanup function that cancels any ongoing asynchronous operations or subscriptions when the component unmounts. This ensures that no state updates are attempted after the component is removed.
What are common scenarios that lead to this error in React?
Common scenarios include asynchronous data fetching, timers, or subscriptions that continue to run after the component has unmounted. If these operations try to update the state after the component is gone, the error will occur.
Can I safely ignore this error in my React application?
Ignoring this error is not advisable as it can lead to performance issues and memory leaks in your application. It is essential to address the underlying causes to maintain optimal application performance and stability.
What tools or methods can help identify unmounted component issues in React?
You can use React’s built-in error boundaries, console warnings, and profiling tools to identify unmounted component issues. Additionally, libraries like React DevTools can help track component lifecycles and state changes effectively.
The warning “can’t perform a React state update on an unmounted component” typically arises when an attempt is made to update the state of a component that has already been removed from the DOM. This situation often occurs in asynchronous operations, such as API calls or timers, where the component may unmount before the operation completes. React’s lifecycle methods and hooks are designed to manage component states efficiently, but developers must ensure that state updates are only attempted on mounted components to avoid memory leaks and unexpected behavior.
To prevent this warning, developers can implement cleanup functions within the `useEffect` hook or component lifecycle methods like `componentWillUnmount`. By using these cleanup mechanisms, one can cancel any ongoing asynchronous tasks or subscriptions when the component unmounts. This practice not only enhances performance but also ensures that the application remains stable and free from errors related to stale state updates.
Another key takeaway is the importance of managing component state with care, especially in scenarios involving asynchronous operations. Leveraging flags or state variables to track the mounted status of a component can provide additional safety. By checking whether a component is still mounted before attempting to update its state, developers can avoid unnecessary warnings and maintain a clean and efficient codebase.
Author Profile
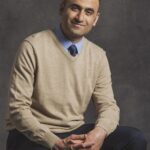
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?