How Can You Use ‘CASE WHEN’ in a SQL WHERE Clause Effectively?
In the world of SQL, crafting efficient queries is both an art and a science. One of the most powerful tools at a developer’s disposal is the ability to use conditional logic directly within the `WHERE` clause. The `CASE WHEN` statement adds a layer of sophistication to your queries, allowing for dynamic filtering based on varying conditions. Whether you’re sifting through vast datasets or fine-tuning reports, mastering this technique can significantly enhance your data manipulation skills and lead to more insightful analyses.
The `CASE WHEN` statement serves as a versatile tool that can transform how you approach data retrieval. By incorporating conditional logic into your `WHERE` clause, you can tailor your queries to return precisely the information you need, based on specific criteria or changing circumstances. This functionality not only streamlines your SQL code but also improves readability and maintainability, making it easier for others (and future you) to understand the logic behind your data selections.
As we delve deeper into the intricacies of using `CASE WHEN` in the `WHERE` clause, you’ll discover how to implement this feature effectively, explore common scenarios where it shines, and learn best practices to avoid potential pitfalls. Whether you’re a seasoned SQL veteran or just starting on your data journey, understanding this powerful construct will elevate your query-writing capabilities and
Using CASE WHEN in the WHERE Clause
The `CASE WHEN` statement is a powerful conditional expression in SQL that allows for more complex logic within queries. While it is commonly used in the `SELECT` statement to manipulate output data, it can also be effectively utilized within the `WHERE` clause to filter records based on dynamic conditions.
When integrating `CASE WHEN` in a `WHERE` clause, it’s essential to understand its structure and functionality. The typical syntax follows this format:
“`sql
SELECT column1, column2
FROM table_name
WHERE condition1 AND
(CASE
WHEN condition2 THEN result1
WHEN condition3 THEN result2
ELSE result3
END) = value;
“`
This enables SQL to evaluate various conditions and return corresponding results, which can then be compared against a specified value.
Examples of CASE WHEN in WHERE Clause
To illustrate, consider a scenario where you want to filter employees based on their performance ratings or department. The SQL query might look like this:
“`sql
SELECT employee_name, department
FROM employees
WHERE
(CASE
WHEN performance_rating >= 90 THEN ‘High’
WHEN performance_rating >= 75 THEN ‘Medium’
ELSE ‘Low’
END) = ‘High’;
“`
In this example, only employees with a high performance rating (90 and above) will be selected.
Important Considerations
- Performance: Using `CASE WHEN` in the `WHERE` clause can impact query performance. It is advisable to ensure that the use of such expressions does not hinder the efficiency of the SQL execution plan.
- Readability: While powerful, overuse or complex `CASE` statements can lead to harder-to-read code. Clear documentation and straightforward logic are recommended.
Practical Applications
Utilizing `CASE WHEN` in the `WHERE` clause can significantly enhance data querying capabilities in various scenarios, such as:
- Dynamic Filtering: Filtering data based on user-defined criteria.
- Conditional Logic: Applying different filtering logic based on the values of one or more columns.
Comparison of Conditional Logic in SQL
The following table summarizes the different ways to implement conditional logic in SQL:
Method | Description | Use Case |
---|---|---|
CASE WHEN | Evaluates a set of conditions and returns a value based on the first true condition. | Dynamic filtering in the WHERE clause. |
IF | Used primarily in procedural SQL to execute conditional logic. | Control flow in stored procedures. |
COALESCE | Returns the first non-null value from a list of arguments. | Handling null values in queries. |
NULLIF | Returns null if two expressions are equal, otherwise returns the first expression. | Preventing division by zero. |
By understanding and applying the `CASE WHEN` statement within the `WHERE` clause, you can create more nuanced and powerful SQL queries that cater to specific business logic and requirements.
Using CASE WHEN in WHERE Clause
The `CASE WHEN` statement is a powerful SQL construct that allows for conditional logic in queries. While it is commonly used in `SELECT` statements for transforming data, it can also be effectively utilized within the `WHERE` clause to filter results based on specific conditions.
Syntax of CASE WHEN in WHERE Clause
The basic syntax for using `CASE WHEN` within a `WHERE` clause is as follows:
“`sql
SELECT column1, column2
FROM table_name
WHERE
condition1
AND (
CASE
WHEN condition2 THEN true_condition
WHEN condition3 THEN _condition
ELSE default_condition
END
);
“`
This structure allows for complex filtering based on various conditions.
Examples of CASE WHEN in WHERE Clause
Consider a scenario where we want to filter employees based on their roles and salary levels. Here are a few practical examples:
– **Example 1: Filtering by Role and Salary**
“`sql
SELECT employee_id, employee_name
FROM employees
WHERE
department_id = 5
AND (
CASE
WHEN role = ‘Manager’ AND salary > 60000 THEN 1
WHEN role = ‘Staff’ AND salary > 40000 THEN 1
ELSE 0
END = 1
);
“`
In this example, employees are selected if they are either Managers earning more than $60,000 or Staff earning more than $40,000.
- Example 2: Multiple Conditions
“`sql
SELECT product_id, product_name
FROM products
WHERE
category_id = 2
AND (
CASE
WHEN stock_quantity < 10 THEN 'Low Stock'
WHEN stock_quantity BETWEEN 10 AND 50 THEN 'Medium Stock'
ELSE 'High Stock'
END = 'Low Stock'
);
```
This query retrieves products from a specific category that have low stock levels.
Performance Considerations
While using `CASE WHEN` in the `WHERE` clause can enhance query flexibility, it is important to consider its impact on performance:
- Execution Plan: The presence of `CASE WHEN` may complicate the execution plan, leading to potential performance degradation.
- Index Utilization: Standard filtering conditions are more likely to leverage indexes effectively compared to conditional logic.
- Complexity: Overly complex conditions can make the query harder to read and maintain.
Alternative Approaches
In some cases, using standard boolean logic might be preferable. Here are alternative approaches:
– **Using OR Logic**: Instead of `CASE WHEN`, use straightforward `AND`/`OR` conditions to achieve similar filtering.
“`sql
SELECT employee_id, employee_name
FROM employees
WHERE
department_id = 5
AND ((role = ‘Manager’ AND salary > 60000) OR (role = ‘Staff’ AND salary > 40000));
“`
– **Common Table Expressions (CTEs)**: For more complex scenarios, CTEs can be used to simplify the main query.
“`sql
WITH FilteredEmployees AS (
SELECT employee_id, employee_name, role, salary
FROM employees
WHERE department_id = 5
)
SELECT *
FROM FilteredEmployees
WHERE
(role = ‘Manager’ AND salary > 60000) OR (role = ‘Staff’ AND salary > 40000);
“`
These alternatives may enhance readability and performance, depending on the context of the query.
Understanding the Use of CASE WHEN in SQL WHERE Clauses
Dr. Emily Carter (Senior Data Analyst, Tech Insights Corp). “Utilizing the CASE WHEN statement within a WHERE clause can significantly enhance query flexibility. It allows for conditional logic that can simplify complex filtering scenarios, making it easier to retrieve data that meets specific criteria.”
Michael Chen (Database Architect, Cloud Solutions Inc). “Incorporating CASE WHEN in a WHERE clause is a powerful technique, especially when dealing with multiple conditions. It enables developers to create dynamic queries that adapt based on varying input parameters, ultimately improving the efficiency of data retrieval.”
Lisa Patel (SQL Consultant, Data Strategies Group). “While using CASE WHEN in a WHERE clause can be advantageous, it is crucial to understand its performance implications. Overusing this approach may lead to slower queries, so it is essential to balance readability with execution speed.”
Frequently Asked Questions (FAQs)
What is the purpose of using CASE WHEN in a WHERE clause?
The CASE WHEN statement in a WHERE clause allows for conditional filtering of records based on specific criteria, enabling more complex queries that adapt based on the values of the data being queried.
Can you provide an example of a CASE WHEN statement in a WHERE clause?
Certainly. An example would be: `SELECT * FROM employees WHERE department_id = CASE WHEN job_title = ‘Manager’ THEN 1 ELSE 2 END;`. This filters employees based on their job title.
Is using CASE WHEN in a WHERE clause efficient?
Using CASE WHEN can introduce complexity and may affect performance, especially on large datasets. It is advisable to evaluate the execution plan and optimize the query as necessary.
Are there any limitations to using CASE WHEN in a WHERE clause?
Yes, CASE WHEN cannot be used to return multiple columns or to perform actions that are not related to filtering. It is strictly for conditional logic within the filtering process.
What are the alternatives to using CASE WHEN in a WHERE clause?
Alternatives include using simple boolean logic with AND/OR operators or employing subqueries to achieve similar filtering without the complexity of CASE WHEN.
Can CASE WHEN be used in combination with other SQL clauses?
Yes, CASE WHEN can be utilized in SELECT, ORDER BY, and GROUP BY clauses, providing flexibility in data manipulation and presentation beyond just the WHERE clause.
The use of the “CASE WHEN” statement in a SQL WHERE clause is a powerful technique that allows for conditional logic within queries. This functionality enables developers to create more dynamic and flexible SQL statements by evaluating conditions and returning specific values based on those evaluations. By incorporating “CASE WHEN” into the WHERE clause, users can filter records based on complex criteria that may not be achievable through standard comparison operators alone.
One of the key advantages of using “CASE WHEN” in the WHERE clause is its ability to simplify complex queries. Instead of writing multiple OR conditions, a single CASE statement can encapsulate various conditions, making the SQL code cleaner and more maintainable. This can lead to improved readability and easier debugging, which is especially beneficial in larger databases or intricate reporting scenarios.
However, it is essential to use this feature judiciously. Overusing “CASE WHEN” can lead to performance issues, especially if the conditions are computationally expensive or if the dataset is large. Therefore, while this technique enhances the flexibility of SQL queries, developers should always consider the potential impact on query performance and optimize accordingly.
In summary, the “CASE WHEN” statement within a WHERE clause is a valuable tool for SQL developers. It allows for more
Author Profile
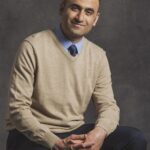
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?