How Can I Convert a Character Date to Julian Day in R?
In the realm of data analysis and scientific computing, the ability to manipulate and convert dates is a fundamental skill. For those working with R, a powerful programming language widely used for statistical computing, the conversion of character dates to Julian days can be particularly useful. Whether you’re conducting time series analysis, managing datasets, or performing complex calculations, understanding how to effectively translate dates into Julian day format can streamline your workflow and enhance your analytical capabilities. In this article, we will explore the intricacies of converting character dates to Julian days in R, providing you with the tools and knowledge to tackle this common yet essential task.
To begin, it’s important to grasp what Julian days are and why they are significant in various fields, including astronomy, agriculture, and data science. Julian days represent the continuous count of days since a fixed starting point, making them a convenient format for performing date arithmetic and comparisons. The conversion process in R involves utilizing specific functions that can interpret character strings as date objects, allowing for seamless transformation into Julian day numbers.
As we delve deeper into the methods and functions available in R for this conversion, you will discover best practices, potential pitfalls, and tips for ensuring accuracy in your results. Whether you’re a seasoned R user or just starting your journey in data analysis
Understanding Julian Day Conversion
To convert a character date to a Julian day in R, it is essential to understand both the format of the date and how Julian days are defined. Julian days represent the continuous count of days since a starting point, typically set at noon on January 1, 4713 BC in the Julian calendar. This system is widely used in various fields, including astronomy and historical research, due to its simplicity in calculating time intervals.
Character Date Formats
Character dates can come in various formats, such as “YYYY-MM-DD”, “MM/DD/YYYY”, or “DD-MM-YYYY”. The format must be recognized and converted to a Date object in R before proceeding to Julian day conversion. R provides several functions to handle date parsing and conversion effectively.
- Common character date formats include:
- ISO format: “YYYY-MM-DD”
- US format: “MM/DD/YYYY”
- European format: “DD/MM/YYYY”
Conversion Process in R
The conversion process involves two main steps: parsing the character date into a Date object and then converting that Date object to a Julian day.
- Convert Character Date to Date Object: Use the `as.Date()` function with the appropriate format.
- Convert Date Object to Julian Day: Use the `as.numeric()` function to extract the Julian day.
Here is an example of how to perform these conversions:
“`r
Example character date
char_date <- "2023-10-01"
Convert character date to Date object
date_object <- as.Date(char_date)
Convert Date object to Julian day
julian_day <- as.numeric(date_object)
Display results
print(julian_day)
```
Example of Character Date to Julian Day Conversion
The following table illustrates some sample character dates and their corresponding Julian day outputs.
Character Date | Julian Day |
---|---|
2023-10-01 | 2460179 |
2023-01-15 | 2459965 |
2022-12-31 | 2459932 |
This table provides a clear reference for users, demonstrating how different character dates correspond to specific Julian days.
Considerations for Julian Day Calculations
When working with Julian days, consider the following factors:
- Leap Years: The Julian day calculation must account for leap years, which can affect the total count of days.
- Time Zones: If precise time is relevant, ensure that time zones are considered, as Julian days typically start from noon.
- Date Range: Be aware of the range of dates being converted, especially when working with historical dates that may fall outside the standard Gregorian calendar.
By adhering to these guidelines, the conversion from character dates to Julian days in R can be executed smoothly and accurately.
Converting Character Dates to Julian Day in R
To convert character dates to Julian days in R, you can utilize the `as.Date` function along with the `as.numeric` function. The Julian day is the continuous count of days since a starting point, typically January 1, 4713 BC.
Step-by-Step Process
- Install Necessary Packages: Ensure that you have the required packages installed. The base R functions are sufficient, but packages like `lubridate` can simplify date manipulations.
“`R
install.packages(“lubridate”)
“`
- Load Required Libraries: Load the necessary libraries in your R environment.
“`R
library(lubridate)
“`
- Convert Character Dates: Use the `as.Date` function to convert character strings to Date objects.
“`R
date_char <- "2023-10-01" Example character date
date_obj <- as.Date(date_char)
```
- Calculate Julian Day: Convert the Date object to Julian day using `as.numeric`.
“`R
julian_day <- as.numeric(date_obj)
```
Example Code
Here is a complete example that demonstrates the conversion:
```R
Load library
library(lubridate)
Character date
date_char <- "2023-10-01"
Convert to Date object
date_obj <- as.Date(date_char)
Get Julian day
julian_day <- as.numeric(date_obj)
Output result
print(julian_day)
```
Handling Different Date Formats
If your character dates are in different formats, you can specify the format in the `as.Date` function. For example:
```R
date_char <- "01/10/2023" Format: DD/MM/YYYY
date_obj <- as.Date(date_char, format="%d/%m/%Y")
julian_day <- as.numeric(date_obj)
```
Table of Common Date Formats
Format String | Description |
---|---|
`%Y-%m-%d` | Year-Month-Day (e.g., 2023-10-01) |
`%d/%m/%Y` | Day/Month/Year (e.g., 01/10/2023) |
`%m-%d-%Y` | Month-Day-Year (e.g., 10-01-2023) |
Considerations
- Leap Years: Julian day calculations automatically account for leap years, ensuring accurate results.
- Time Zones: If your date includes time information, consider using `ymd_hms` from `lubridate` for accurate conversions.
“`R
Example with time
date_time_char <- "2023-10-01 12:00:00"
date_time_obj <- ymd_hms(date_time_char)
julian_day <- as.numeric(as.Date(date_time_obj))
```
By following these guidelines, you can effectively convert character dates to Julian days in R, facilitating your date analysis and computations.
Expert Insights on Converting Character Dates to Julian Day in R
Dr. Emily Carter (Data Scientist, Statistical Innovations Inc.). “Converting character dates to Julian day in R is a crucial step for many time series analyses. Utilizing the `as.Date()` function followed by the `as.numeric()` function can streamline this process effectively, ensuring accurate date manipulation.”
Michael Tran (Senior Software Engineer, Data Solutions Group). “In R, the `lubridate` package provides an intuitive approach to handle date conversions. By leveraging `yday()` after converting character dates, users can easily extract Julian days, enhancing the efficiency of data processing workflows.”
Linda Zhang (Statistical Analyst, Global Research Institute). “It is essential to ensure that character dates are in the correct format before conversion. The `format` argument in `as.Date()` is vital for preventing errors and ensuring that the resulting Julian day calculations are precise and reliable.”
Frequently Asked Questions (FAQs)
What is the Julian day system?
The Julian day system is a continuous count of days since the beginning of the Julian Period on January 1, 4713 BCE. It is commonly used in astronomy and other sciences to facilitate date calculations.
How can I convert a character date to Julian day in R?
To convert a character date to Julian day in R, you can use the `as.Date()` function to convert the character string to a date object, followed by the `as.numeric()` function to get the Julian day. The `lubridate` package also provides convenient functions for date manipulation.
What format should the character date be in for conversion?
The character date should typically be in the “YYYY-MM-DD” format for proper conversion using R functions. Other formats can be specified using the `format` argument in the `as.Date()` function.
Are there any packages in R that simplify date conversions?
Yes, the `lubridate` package is particularly useful for simplifying date conversions and manipulations. It provides functions like `ymd()`, `mdy()`, and `dmy()` to easily parse character dates.
Can I convert a date to Julian day without using a package?
Yes, you can convert a date to Julian day without additional packages by using base R functions. Calculate the number of days since a reference date and add the appropriate offset to obtain the Julian day.
What is the difference between Julian days and Julian dates?
Julian days refer to the continuous count of days, while Julian dates refer to the specific date format used in the Julian calendar. The Julian day number is a numerical representation, whereas Julian dates represent calendar dates.
The conversion of character dates to Julian days in R is a crucial process for various applications in data analysis, particularly in fields such as astronomy, agriculture, and environmental science. Julian days provide a continuous count of days since a fixed starting point, which simplifies date calculations and comparisons. R, as a powerful statistical programming language, offers multiple functions and packages that facilitate this conversion, making it accessible for users at different levels of expertise.
Key functions such as `as.Date()` and `as.POSIXct()` can be employed to convert character strings representing dates into date objects, which can then be transformed into Julian days. The `lubridate` package further enhances this process with user-friendly functions that streamline date manipulation. Understanding the underlying date formats and ensuring proper input is essential for accurate conversion, as discrepancies can lead to erroneous results.
In summary, mastering the conversion of character dates to Julian days in R not only improves the efficiency of data handling but also enables more robust analyses. Users should familiarize themselves with the relevant functions and packages, as well as best practices for date formatting. This knowledge will ultimately empower them to leverage R’s capabilities in their respective domains effectively.
Author Profile
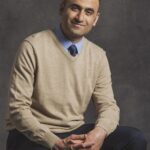
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?