Why Does My Class Path Contain Multiple SLF4J Bindings and How Can I Fix It?
In the world of Java development, logging is a critical aspect that ensures developers can monitor and troubleshoot their applications effectively. One of the most popular logging frameworks used in Java is SLF4J (Simple Logging Facade for Java), which provides a simple interface for various logging implementations. However, as projects grow in complexity and dependencies multiply, developers may encounter a perplexing issue: “class path contains multiple SLF4J bindings.” This warning can be a source of confusion and frustration, leading to unexpected behavior in logging output. In this article, we will unravel the intricacies of this common problem, explore its implications, and provide insights on how to resolve it, ensuring that your logging setup remains clean and efficient.
When you see the message indicating that your class path contains multiple SLF4J bindings, it signifies that more than one logging implementation is present in your project. This situation can arise from various sources, such as transitive dependencies or conflicting library versions. The presence of multiple bindings can lead to unpredictable logging behavior, as SLF4J is designed to work with only one binding at a time. Understanding the root causes of this issue is essential for maintaining a stable and predictable logging environment in your applications.
In the following sections, we will delve deeper into
Understanding SLF4J Bindings
SLF4J (Simple Logging Facade for Java) serves as a façade for various logging frameworks, enabling developers to plug in their preferred logging implementation. However, the presence of multiple SLF4J bindings in the class path can lead to significant issues, including unpredictable logging behavior and runtime exceptions.
When an application is executed, SLF4J attempts to locate the appropriate binding to direct logging calls to the chosen logging framework. If more than one binding is found, SLF4J cannot determine which one to use, resulting in the warning message: “Class path contains multiple SLF4J bindings.” This situation can arise from dependencies, where different libraries include their own SLF4J bindings.
Common Causes of Multiple Bindings
Several scenarios may lead to this issue:
- Transitive Dependencies: A library that your project depends on may itself depend on another library that includes a different SLF4J binding.
- Direct Inclusion: Multiple SLF4J implementations are explicitly included in your project’s build configuration.
- Version Mismatch: Different versions of the same binding may be included due to outdated dependencies.
Identifying and Resolving Multiple Bindings
To resolve the issue of multiple SLF4J bindings, it is critical to identify all bindings present in the class path. Here are steps to help you achieve this:
- Inspect the Dependency Tree: Use build tools like Maven or Gradle to visualize the dependency tree.
- Check Class Path: Review the libraries included in your project for any SLF4J bindings.
Here is a sample command for Maven:
“`bash
mvn dependency:tree
“`
The output will help you identify any conflicting SLF4J bindings.
Resolving Strategies
Once you have identified the conflicting bindings, consider the following strategies:
- Exclude Unwanted Dependencies: Use dependency management features to exclude unnecessary SLF4J bindings.
- Consolidate Versions: Ensure that all dependencies use the same version of SLF4J binding.
- Choose a Single Binding: Decide which SLF4J binding fits your logging needs and retain only that one.
Binding | Purpose | Recommended Action |
---|---|---|
slf4j-log4j12 | SLF4J binding for Log4j | Keep if using Log4j |
slf4j-simple | Simple binding for SLF4J | Keep for lightweight applications |
slf4j-jdk14 | SLF4J binding for Java Util Logging | Keep if using Java Util Logging |
slf4j-nop | No operation binding | Remove unless intentionally suppressing logging |
By following these steps and strategies, you can effectively manage SLF4J bindings in your project, ensuring consistent logging behavior and reducing potential issues arising from multiple bindings.
Understanding SLF4J Bindings
The Simple Logging Facade for Java (SLF4J) is a popular abstraction for various logging frameworks. It allows developers to plug in any logging framework at deployment time. However, when multiple SLF4J bindings are present in the class path, it can lead to conflicts, resulting in unpredictable logging behavior.
Common Causes of Multiple SLF4J Bindings
Several scenarios can lead to multiple SLF4J bindings being included in a project:
- Dependency Conflicts: Different libraries may include their own version of SLF4J bindings.
- Transitive Dependencies: A library that you are using might depend on another library that includes a different SLF4J binding.
- Manual Inclusion: Developers may inadvertently add more than one binding to the project’s configuration.
Identifying SLF4J Binding Conflicts
To identify SLF4J binding conflicts, use the following methods:
- Maven Dependency Tree: Run `mvn dependency:tree` to view all dependencies and their bindings.
- Gradle Dependency Insight: Use `./gradlew dependencies` to check for SLF4J bindings in your Gradle project.
- Class Path Scanning: Utilize tools like `jarscan` to inspect the class path for multiple SLF4J bindings.
Resolving Binding Conflicts
Once you have identified the conflicting bindings, several strategies can help resolve the issues:
- Exclude Transitive Dependencies: Modify your `pom.xml` (for Maven) or `build.gradle` (for Gradle) to exclude unwanted SLF4J bindings.
Example in Maven:
“`xml
“`
- Choose a Single Binding: Opt for one SLF4J binding that meets your project’s needs (e.g., `slf4j-log4j12`, `slf4j-simple`, or `slf4j-logback`).
- Update Dependencies: Ensure that all dependencies are updated to versions that are compatible with your chosen SLF4J binding.
Testing After Resolution
After resolving the bindings, it is crucial to verify that logging functions as expected:
- Run Unit Tests: Execute existing unit tests to ensure logging behaves correctly.
- Check Log Output: Manually check logs for proper output and formatting.
- Use Loggers: Ensure that logger instances are correctly configured and utilized throughout the application.
Best Practices for Managing SLF4J Bindings
To prevent future issues with SLF4J bindings, consider the following best practices:
- Regular Dependency Audits: Periodically review dependencies to catch conflicts early.
- Use Dependency Management Tools: Leverage tools like Maven Enforcer or Gradle’s Dependency Constraints to manage versions.
- Documentation: Maintain clear documentation of chosen logging frameworks and bindings for team members.
Addressing the issue of multiple SLF4J bindings effectively ensures consistent logging behavior in Java applications. By following the outlined strategies and best practices, developers can maintain a clean and efficient logging setup.
Understanding SLF4J Binding Conflicts in Java Applications
Dr. Emily Carter (Senior Software Engineer, Java Development Institute). “When a class path contains multiple SLF4J bindings, it can lead to unpredictable logging behavior. This situation arises because SLF4J is designed to work with a single binding at runtime, and multiple bindings can create conflicts that confuse the logging framework.”
Michael Chen (Lead DevOps Architect, Cloud Solutions Inc.). “Resolving SLF4J binding conflicts is crucial for maintaining clean and efficient logging in applications. Developers should ensure that only one binding is included in the class path to prevent runtime exceptions and ensure that the intended logging implementation is used.”
Sarah Thompson (Java Framework Specialist, Tech Innovations). “It’s essential to regularly audit your dependencies to identify and eliminate multiple SLF4J bindings. Using tools like Maven or Gradle can help manage dependencies effectively, allowing developers to avoid such conflicts and streamline their logging configuration.”
Frequently Asked Questions (FAQs)
What does it mean when the class path contains multiple SLF4J bindings?
The presence of multiple SLF4J bindings indicates that more than one logging implementation is available on the class path. This can lead to unpredictable logging behavior, as SLF4J may not know which binding to use.
How can I resolve the issue of multiple SLF4J bindings?
To resolve this issue, identify and remove the unnecessary SLF4J bindings from your class path. Ensure that only one logging implementation, such as SLF4J with Logback or Log4j, is included in your project dependencies.
What are the potential consequences of having multiple SLF4J bindings?
Having multiple SLF4J bindings can result in runtime exceptions, inconsistent logging output, and increased application size due to redundant libraries. It complicates troubleshooting and may lead to performance issues.
How can I check which SLF4J bindings are present in my project?
You can check the SLF4J bindings by reviewing your project’s dependency tree using build tools like Maven or Gradle. Alternatively, you can inspect the class path at runtime to identify the loaded SLF4J bindings.
Is it safe to ignore warnings about multiple SLF4J bindings?
Ignoring warnings about multiple SLF4J bindings is not advisable. While the application may still function, it can lead to unpredictable behavior and difficult-to-diagnose issues, impacting the reliability of the logging system.
What steps should I take if I encounter SLF4J binding conflicts in a multi-module project?
In a multi-module project, ensure that each module specifies only one SLF4J binding in its dependencies. Use dependency management features of your build tool to enforce consistent versions across modules and avoid conflicts.
The warning message indicating that the class path contains multiple SLF4J bindings is a common issue encountered in Java applications that utilize the SLF4J (Simple Logging Facade for Java) framework. This situation arises when more than one SLF4J binding is present in the classpath, which can lead to unpredictable logging behavior. SLF4J is designed to allow developers to plug in different logging frameworks, but having multiple bindings can cause conflicts, as SLF4J does not know which binding to delegate logging calls to.
To resolve this issue, it is essential to identify and eliminate the redundant SLF4J bindings from the classpath. This can typically be achieved by reviewing the project’s dependencies and ensuring that only one binding, such as SLF4J with Logback or Log4j, is included. Tools like Maven or Gradle can assist in managing dependencies and identifying conflicts. Additionally, it is advisable to check for transitive dependencies that may inadvertently introduce multiple bindings into the project.
In summary, addressing the issue of multiple SLF4J bindings is crucial for maintaining a stable and predictable logging environment in Java applications. Developers should regularly audit their dependencies and be mindful of the logging frameworks they integrate to avoid this common
Author Profile
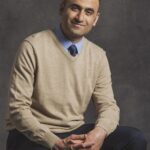
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?