Why Isn’t My Click Event Firing in JavaScript?
In the dynamic world of web development, interactivity is key to creating engaging user experiences. One of the fundamental building blocks of interactivity is the click event in JavaScript, which allows developers to respond to user actions seamlessly. However, there are times when this seemingly straightforward feature can become a source of frustration, as click events refuse to fire as expected. Whether you’re a seasoned developer or a newcomer to the coding scene, understanding the nuances behind click events is crucial for troubleshooting and enhancing your web applications.
When a click event fails to trigger, it can stem from a variety of issues ranging from simple coding oversights to more complex conflicts within the JavaScript environment. Developers often encounter scenarios where event listeners are not properly attached, elements are obscured by CSS layers, or even JavaScript errors are silently preventing execution. Identifying the root cause of these problems requires a systematic approach and a keen eye for detail, as even the smallest oversight can lead to a non-responsive interface.
In this article, we will delve into the common pitfalls that can lead to click events not firing in JavaScript. From examining the structure of your event listeners to exploring the impact of browser compatibility and debugging techniques, we will equip you with the knowledge needed to diagnose and resolve these issues effectively.
Common Causes of Click Event Issues
Click events in JavaScript may not fire due to several common issues. Understanding these can help in debugging and resolving problems effectively.
- Event Delegation: If the click event is attached to a parent element, but the target element is dynamically created or manipulated after the event listener is set, the event will not trigger. Use event delegation by adding the listener to a parent element that exists when the script runs.
- Preventing Default Behavior: If an event handler includes `event.preventDefault()`, it might stop the click event from behaving as expected. Ensure that this method is only called when necessary.
- Incorrect Selector: If the JavaScript code uses a selector that does not match any existing elements, the event listener will not be applied. Double-check that the selector correctly targets the intended elements.
- CSS Pointer Events: If the element has a CSS style of `pointer-events: none;`, it will not respond to click events. Verify the CSS properties to ensure the element is interactive.
- JavaScript Errors: An error in your JavaScript code before the click event listener is registered can prevent it from executing. Use the browser’s console to check for errors.
Troubleshooting Steps
When debugging click event issues, follow these steps:
- Check the Console: Look for any JavaScript errors that may prevent execution.
- Verify Element Existence: Use `console.log()` to confirm that the target element exists before attaching the event listener.
- Inspect Event Listeners: Use browser developer tools to inspect whether the event listeners are attached correctly.
- Testing with Different Selectors: Try different selectors to ensure you are targeting the right element.
Code Examples for Event Handling
Here are examples illustrating common methods for attaching click events effectively.
“`javascript
// Example of direct event listener attachment
document.getElementById(‘myButton’).addEventListener(‘click’, function() {
alert(‘Button clicked!’);
});
// Example of event delegation
document.getElementById(‘parentDiv’).addEventListener(‘click’, function(event) {
if (event.target.matches(‘.childButton’)) {
alert(‘Child button clicked!’);
}
});
“`
Best Practices for Click Events
To ensure that click events work consistently, follow these best practices:
- Always attach event listeners after the DOM has fully loaded.
- Use `event.stopPropagation()` cautiously to avoid blocking parent event listeners unintentionally.
- Keep event handler functions concise and efficient to improve performance.
- Consider using the `once` option for one-time event listeners to automatically remove them after execution.
Common Mistakes Table
Mistake | Consequence | Solution |
---|---|---|
Attaching listener to non-existent element | No event firing | Ensure element exists before attaching |
CSS property `pointer-events: none;` | Element is unresponsive | Remove or modify CSS property |
Using `event.preventDefault()` incorrectly | Default actions suppressed | Use only when necessary |
Common Reasons for Click Event Issues
Several factors can contribute to a click event not firing as expected in JavaScript. Understanding these can help in diagnosing and resolving the issue effectively.
- Element Not in the DOM: If the element to which you have bound the click event has not been added to the DOM at the time of binding, the event will not fire.
- Event Delegation Misconfiguration: If you are using event delegation but have not set the listener on the appropriate ancestor element, the click event may not trigger.
- JavaScript Errors: Any JavaScript errors occurring before the event is fired can halt execution, preventing the click handler from running.
- CSS Issues: Sometimes, elements may be hidden or positioned in such a way that they are not clickable (e.g., `display: none;` or `pointer-events: none;`).
- Multiple Event Listeners: If multiple event listeners are set on the same element, they may conflict, preventing the expected behavior.
- Prevent Default Behavior: If there is a `preventDefault()` call on a parent element, it may stop the event from reaching your handler, especially in cases of form submissions or anchor tags.
Troubleshooting Steps
To diagnose and fix click event issues, follow these troubleshooting steps:
- **Check Console for Errors**: Open the browser console (F12 or right-click > Inspect) and look for any JavaScript errors that may be affecting event firing.
- Verify Element Existence: Ensure the element is present in the DOM when the click event is bound. Use `console.log()` to check its status.
- Use Event Delegation Properly: If using event delegation, ensure that the listener is applied to a parent element that exists in the DOM when the event is triggered.
- Inspect CSS Styles: Verify that the element is not styled in a way that prevents interaction. Check for properties like `visibility`, `display`, and `pointer-events`.
- Test Event Listeners: Temporarily remove other event listeners to see if they may be interfering with the click event.
- Debug with Breakpoints: Use breakpoints in the browser’s developer tools to step through your code and confirm that the event handler is reached.
Example Code Snippet
Here is an example demonstrating the correct binding of a click event:
“`javascript
document.addEventListener(‘DOMContentLoaded’, function () {
const button = document.getElementById(‘myButton’);
button.addEventListener(‘click’, function () {
console.log(‘Button clicked!’);
});
});
“`
In this snippet, the event listener is added after the DOM is fully loaded, ensuring that the button element exists when the listener is attached.
Using jQuery for Click Events
If you are using jQuery, the click event can be bound as follows:
“`javascript
$(document).ready(function () {
$(‘myButton’).click(function () {
console.log(‘Button clicked!’);
});
});
“`
jQuery simplifies event handling but also requires that the DOM is fully loaded before binding events.
Advanced Techniques
For more complex scenarios, consider the following techniques:
- Event Delegation: Use event delegation for dynamically added elements.
“`javascript
$(document).on(‘click’, ‘.dynamic-element’, function () {
console.log(‘Dynamic element clicked!’);
});
“`
- Custom Events: Create and trigger custom events if necessary, providing more control over event handling.
“`javascript
const event = new CustomEvent(‘myCustomEvent’);
document.dispatchEvent(event);
document.addEventListener(‘myCustomEvent’, function () {
console.log(‘Custom event triggered!’);
});
“`
By systematically identifying the cause of the issue and employing effective coding practices, you can ensure that click events function correctly in your JavaScript applications.
Understanding Why Click Events May Not Fire in JavaScript
Dr. Emily Carter (Senior JavaScript Developer, Tech Innovations Inc.). “One common reason for click events not firing is the improper binding of event listeners. If the listener is attached to an element that does not exist in the DOM at the time of binding, the event will not trigger. It is crucial to ensure that the event listener is added after the element is available.”
Mark Thompson (Front-End Engineer, CodeCraft Solutions). “Another frequent issue arises from event propagation. If a click event is being stopped by a parent element’s event handler, the intended child element’s click event may never fire. It is essential to check for any calls to event.stopPropagation() or event.preventDefault() that might be affecting the click event.”
Sarah Kim (UI/UX Specialist, Design Dynamics). “Sometimes, the issue can stem from CSS properties such as ‘pointer-events: none’, which can prevent click events from being registered on an element. Ensuring that the element is interactive and visible is key to troubleshooting click event issues.”
Frequently Asked Questions (FAQs)
What causes a click event not to fire in JavaScript?
A click event may not fire due to various reasons, including incorrect event binding, elements being covered by other elements, or the element being disabled. Additionally, if JavaScript is not properly loaded or there are syntax errors in the script, the event may fail to trigger.
How can I check if my click event is properly bound?
You can check if a click event is properly bound by using `console.log()` within the event handler function. This will allow you to see if the function is being executed when the element is clicked. Additionally, ensure that your event listener is attached after the DOM is fully loaded.
What should I do if the click event is not firing on dynamically created elements?
For dynamically created elements, ensure that you use event delegation by attaching the event listener to a parent element that exists in the DOM at the time of binding. Use the `addEventListener()` method on the parent and check for the target element in the event handler.
Are there any browser compatibility issues that could prevent a click event from firing?
While modern browsers generally support click events consistently, older browsers may have quirks. Ensure that you are using standard event handling methods and check for compatibility issues in older versions of browsers if necessary.
How can I troubleshoot a click event that is not firing?
To troubleshoot, check for JavaScript errors in the console, ensure the element is not hidden or disabled, verify that the event listener is correctly attached, and confirm that there are no CSS styles or scripts preventing the click action. Testing in different browsers can also help identify the issue.
What tools can I use to debug JavaScript click events?
You can use browser developer tools, such as Chrome DevTools or Firefox Developer Edition, to inspect elements, view console logs, and debug JavaScript. The “Elements” tab allows you to check event listeners, while the “Console” tab is useful for logging and error checking.
In summary, the issue of a click event not firing in JavaScript can arise from various factors, including incorrect event listener attachment, conflicts with other scripts, or issues related to the element’s visibility and interactivity. It is crucial to ensure that the event listener is properly bound to the intended element and that the element is present in the DOM at the time of binding. Additionally, developers should consider the possibility of event propagation issues or the use of `preventDefault()` in other event handlers that may interfere with the click event.
Key takeaways from the discussion include the importance of debugging techniques such as using console logs to verify whether the event listener is being triggered. Inspecting the DOM structure and ensuring that the element is not covered by other elements can also help identify the root cause of the problem. Furthermore, using modern JavaScript practices, such as event delegation, can enhance the reliability of click events, especially in dynamic applications where elements may be added or removed from the DOM.
Ultimately, a methodical approach to troubleshooting click events in JavaScript is essential for ensuring robust user interactions. By systematically checking for common pitfalls and employing effective debugging strategies, developers can resolve issues related to click events not firing and enhance the overall functionality of their web
Author Profile
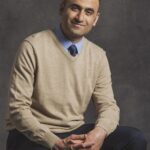
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?