How Can You Convert an Integer to a Character in C++?
In the world of C++ programming, data types play a crucial role in how we manipulate and interact with information. Among the various data types, integers and characters are fundamental, each serving distinct purposes in our code. However, there are times when the need arises to convert an integer to a character, whether it’s for display purposes, formatting output, or simply to meet specific algorithm requirements. Understanding how to effectively perform this conversion can enhance your programming skills and streamline your code.
Converting an integer to a character in C++ is a straightforward yet essential task that many developers encounter. This process involves understanding the relationship between numeric values and their corresponding character representations, particularly in the context of ASCII values. By grasping this concept, programmers can easily transform integers into characters, enabling more dynamic and versatile applications.
Moreover, C++ provides several methods to achieve this conversion, each with its own advantages and use cases. Whether you are looking to convert a single digit or a more complex integer, knowing the right approach can save time and prevent errors in your code. In this article, we will delve into the various techniques for converting integers to characters in C++, equipping you with the knowledge to tackle this common programming challenge effectively.
Methods to Convert Integer to Character in C++
In C++, converting an integer to a character can be achieved through various methods depending on the context in which the conversion is needed. Below are some common approaches to perform this conversion effectively.
Using Type Casting
One of the simplest methods to convert an integer to a character is by using type casting. This method involves directly casting an integer value to the `char` type.
“`cpp
int number = 65; // ASCII value for ‘A’
char character = static_cast
“`
This will convert the integer `65` to its corresponding ASCII character, which is `’A’`.
Using Character Arithmetic
Another approach involves character arithmetic, where you can manipulate character values based on their ASCII values.
“`cpp
int number = 5;
char character = ‘0’ + number; // Converts integer to character ‘5’
“`
In this case, adding `number` to the character `’0’` will yield the character representation of the integer.
Using `sprintf` for Formatting
For more complex formatting, you can use the `sprintf` function, which allows formatting integers into a character array.
“`cpp
char buffer[10];
int number = 123;
sprintf(buffer, “%d”, number);
“`
Here, `buffer` will contain the string representation of the integer, which can be processed further if needed.
Using `std::to_string` and `c_str`
If you need to convert an integer into a string and then retrieve the character representation, the `std::to_string` function can be utilized, followed by `c_str()`.
“`cpp
int number = 7;
std::string str = std::to_string(number);
char character = str[0]; // Get the first character
“`
This method is particularly useful when you want to convert integers to strings before manipulating them.
Conversion Table
The following table illustrates the ASCII values of some common characters and their corresponding integer values.
Character | ASCII Value |
---|---|
A | 65 |
B | 66 |
0 | 48 |
1 | 49 |
9 | 57 |
Conclusion on Usage
When choosing a method for converting an integer to a character in C++, consider the context of your application and the specific requirements. Simple casting is efficient for direct conversions, while `sprintf` and `std::to_string` provide more flexibility for formatted outputs.
Methods to Convert Integer to Character in C++
In C++, converting an integer to a character can be accomplished through several methods, depending on the context and the desired outcome. Below are some common approaches:
Using Type Casting
The simplest way to convert an integer to a character is through type casting. This method directly interprets the integer value as a character based on its ASCII value.
“`cpp
int num = 65; // ASCII value for ‘A’
char ch = (char)num; // ch will be ‘A’
“`
- This method works well for values in the range of valid ASCII characters (0-127).
- For extended ASCII values (128-255), ensure that the character set supports those values.
Using `std::to_string` and `c_str`
When you need to convert an integer to a character representation as part of a string, you can use the `std::to_string` function followed by accessing the character.
“`cpp
include
int num = 5;
std::string str = std::to_string(num);
char ch = str[0]; // ch will be ‘5’
“`
- Ensure that the integer is non-negative and within the bounds of string indexing.
Using `sprintf` Function
Another approach involves using the `sprintf` function from the C standard library, which can format an integer into a character array.
“`cpp
include
int num = 97; // ASCII for ‘a’
char buffer[2]; // Buffer to hold the character
sprintf(buffer, “%c”, num);
char ch = buffer[0]; // ch will be ‘a’
“`
- This method is useful for formatted output and can handle multiple formats.
Using `std::stringstream`
The `std::stringstream` class from the `
“`cpp
include
int num = 66; // ASCII for ‘B’
std::stringstream ss;
ss << static_cast
char ch = ss.str()[0]; // ch will be ‘B’
“`
- This method provides flexibility and is suitable for various data types.
Conversion Table of Integer to Character
The following table illustrates some common integer values and their corresponding ASCII character representations:
Integer | Character |
---|---|
65 | A |
66 | B |
67 | C |
48 | 0 |
49 | 1 |
50 | 2 |
97 | a |
98 | b |
99 | c |
Use these methods and considerations based on the specific requirements of your application, ensuring that the integer values fall within the appropriate ranges for the desired character output.
Expert Insights on Converting Integers to Characters in C++
Dr. Emily Carter (Computer Science Professor, Tech University). “Converting an integer to a character in C++ can be efficiently achieved using static_cast
(intValue). This method provides a clear and type-safe way to perform the conversion, ensuring that the integer is interpreted correctly as a character.”
Michael Chen (Senior Software Engineer, CodeCraft Solutions). “When converting integers to characters, it is crucial to consider the range of values. For instance, converting an integer between 0 and 255 will yield corresponding ASCII characters, while values outside this range may lead to behavior. Always validate your input to avoid potential issues.”
Sarah Thompson (Lead Developer, C++ Innovations). “Using the char() function is another straightforward approach for conversion. However, I recommend using it in conjunction with error checking to ensure that the integer falls within the valid range for character representation. This practice enhances code robustness and maintainability.”
Frequently Asked Questions (FAQs)
How do I convert an integer to a character in C++?
You can convert an integer to a character in C++ by casting the integer to a `char` type. For example, `char c = static_cast
What is the ASCII value of a character in C++?
In C++, characters are represented by their ASCII values, which are integer values corresponding to each character. For example, the ASCII value of ‘A’ is 65, and you can obtain it using `int asciiValue = static_cast
Can I convert an integer directly to a character without casting?
Yes, you can convert an integer directly to a character without explicit casting if the integer is within the valid range of ASCII values. For instance, `char c = 65;` will assign ‘A’ to `c`.
What happens if I convert an integer outside the ASCII range?
Converting an integer outside the ASCII range may result in behavior or unexpected characters. It is advisable to ensure the integer is within the range of 0 to 127 for standard ASCII.
Is there a function in C++ to convert an integer to a character?
C++ does not provide a specific built-in function for this conversion, but you can use the `std::to_string()` function to convert an integer to a string and then access the character, or use simple casting as mentioned earlier.
How can I convert multiple integers to characters in C++?
You can convert multiple integers to characters using a loop. For example:
“`cpp
for (int i = 0; i < size; ++i) {
char c = static_cast
}
“`
This will convert each integer in `intArray` to a character.
In C++, converting an integer to a character can be achieved through various methods, depending on the context and the desired outcome. The most straightforward approach is to use type casting, where an integer value representing an ASCII code can be directly cast to a character type. For example, casting an integer like 65 will yield the character ‘A’, as 65 is the ASCII value for that letter. This method is efficient and commonly used for simple conversions.
Another method involves using the `std::to_string` function to convert the integer to a string and then accessing the first character of that string. This approach is particularly useful when the integer does not directly correspond to an ASCII value but instead represents a numeric character, such as converting the integer 5 to the character ‘5’. This method provides flexibility when dealing with numeric representations in character form.
Additionally, it is important to consider the potential pitfalls associated with these conversions. For instance, attempting to convert an integer outside the valid ASCII range can lead to unexpected results. Therefore, it is crucial to ensure that the integer value falls within the appropriate range when using direct casting. Understanding these nuances will enhance the reliability of character conversions in C++ programming.
Author Profile
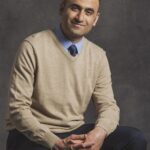
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?