How Can You Convert an Integer to a Double in Java?
In the world of programming, data types are fundamental building blocks that dictate how information is stored and manipulated. Among these types, integers and doubles play crucial roles, especially in languages like Java. While integers represent whole numbers, doubles allow for the representation of decimal values, enabling a broader range of mathematical operations and precision. However, there are times when developers need to convert an integer to a double, whether to perform calculations that require decimal precision or to ensure compatibility with methods that demand a floating-point argument. Understanding how to seamlessly execute this conversion is essential for any Java programmer looking to enhance their coding efficiency and accuracy.
Converting an integer to a double in Java is a straightforward process, thanks to the language’s robust type system and built-in functionalities. This conversion can be achieved through several methods, each with its own nuances and use cases. For instance, implicit type casting allows for a simple and automatic transition from an int to a double, while explicit casting provides more control over the conversion process. Knowing when to use each method can significantly impact the performance and readability of your code.
Moreover, understanding the implications of this conversion is vital. While converting an integer to a double is generally safe, it’s important to recognize potential pitfalls, such as loss of precision in certain scenarios or the
Methods to Convert int to double in Java
In Java, converting an `int` to a `double` is straightforward, as this conversion is done automatically due to Java’s type promotion rules. However, there are several explicit methods to perform this conversion, which can be useful for clarity and ensuring that the conversion is intentional.
Implicit Conversion
When an `int` is assigned to a `double` variable, Java automatically converts the `int` to `double`. This process is known as implicit conversion or type promotion. For example:
“`java
int integerValue = 5;
double doubleValue = integerValue; // Implicit conversion
“`
In this case, the value of `integerValue` is automatically converted to a `double`, resulting in `doubleValue` holding the value `5.0`.
Explicit Conversion Using Casting
While implicit conversion is convenient, you may choose to use explicit casting to enhance readability and indicate that a conversion is taking place:
“`java
int integerValue = 5;
double doubleValue = (double) integerValue; // Explicit conversion
“`
This approach makes it clear to anyone reading the code that a conversion is occurring, even though it is not strictly necessary in this case.
Using Wrapper Classes
Java’s wrapper class `Double` provides a method to convert an `int` to a `double`. Using `Double.valueOf(int)` is another way to achieve the same result:
“`java
int integerValue = 5;
double doubleValue = Double.valueOf(integerValue); // Using wrapper class
“`
This method returns a `Double` object, but it can be unboxed to a primitive `double` if necessary.
Comparison of Conversion Methods
The following table summarizes the different methods to convert an `int` to a `double`:
Method | Code Example | Returns |
---|---|---|
Implicit Conversion | doubleValue = integerValue; | double |
Explicit Casting | doubleValue = (double) integerValue; | double |
Using Wrapper Class | doubleValue = Double.valueOf(integerValue); | Double object (can be unboxed to double) |
Best Practices
When converting `int` to `double`, consider the following best practices:
- Clarity: Use explicit casting if it improves the readability of your code.
- Performance: Implicit conversion is generally faster and should be preferred unless clarity is a concern.
- Use of Wrapper Classes: Utilize wrapper classes when working with collections or APIs that require object types rather than primitives.
By understanding these methods and best practices, developers can efficiently manage data type conversions while maintaining code readability and performance.
Methods to Convert `int` to `double` in Java
In Java, converting an `int` to a `double` is straightforward due to Java’s automatic type promotion in arithmetic operations. Below are the primary methods to achieve this conversion.
Using Explicit Casting
Explicit casting allows you to convert an `int` to a `double` by simply using the cast operator. This method is clear and direct.
“`java
int intValue = 42;
double doubleValue = (double) intValue;
“`
Using Automatic Type Promotion
When an `int` is involved in an arithmetic operation with a `double`, Java automatically promotes the `int` to a `double`. This method does not require any explicit casting.
“`java
int intValue = 42;
double doubleValue = intValue + 0.0; // Automatic promotion
“`
Using Wrapper Class Method
Java’s `Double` wrapper class provides a method for conversion. The method `Double.valueOf()` can be used to convert an `int` to a `double`.
“`java
int intValue = 42;
double doubleValue = Double.valueOf(intValue); // Returns a Double object
“`
Performance Considerations
While all methods are effective, there are slight differences in performance:
Method | Performance | Use Case |
---|---|---|
Explicit Casting | Fast, no overhead | When clarity is needed in conversion |
Automatic Promotion | Fast, seamless | When performing arithmetic operations |
Wrapper Class Method | Slightly slower | When converting to `Double` object for collections |
Example Code Snippet
Here is a complete example demonstrating the conversion from `int` to `double` using the methods discussed:
“`java
public class IntToDoubleExample {
public static void main(String[] args) {
int intValue = 42;
// Method 1: Explicit Casting
double doubleValue1 = (double) intValue;
System.out.println(“Explicit Casting: ” + doubleValue1);
// Method 2: Automatic Type Promotion
double doubleValue2 = intValue + 0.0;
System.out.println(“Automatic Promotion: ” + doubleValue2);
// Method 3: Wrapper Class Method
double doubleValue3 = Double.valueOf(intValue);
System.out.println(“Wrapper Class Method: ” + doubleValue3);
}
}
“`
This code snippet illustrates all three methods in action, providing output that confirms successful conversion.
Expert Insights on Converting int to double in Java
Dr. Emily Carter (Senior Software Engineer, Java Development Group). “In Java, converting an integer to a double is a straightforward process, typically achieved through implicit type casting. This conversion allows developers to perform arithmetic operations that require floating-point precision without losing the integer’s value.”
Mark Thompson (Java Language Specialist, Tech Innovations Inc.). “When converting from int to double, it is crucial to understand the implications of precision and rounding. Java handles this conversion seamlessly, but developers should be cautious when performing calculations that could lead to loss of accuracy in larger datasets.”
Linda Garcia (Lead Java Architect, FutureTech Solutions). “Using the ‘double’ type in Java provides a wider range for numerical representation. Therefore, converting an int to double is not only syntactically simple but also essential for applications requiring high precision in mathematical computations.”
Frequently Asked Questions (FAQs)
How can I convert an int to a double in Java?
You can convert an int to a double in Java by simply assigning the int value to a double variable. Java performs automatic type promotion in this case. For example:
“`java
int intValue = 5;
double doubleValue = intValue;
“`
Is explicit casting necessary when converting int to double in Java?
No, explicit casting is not necessary when converting an int to a double in Java, as the conversion is implicit. However, if you were converting from double to int, explicit casting would be required.
What happens to the value when converting int to double?
The value remains the same when converting from int to double. The only difference is the data type; the double can represent fractional values, while int cannot.
Can I convert an array of ints to an array of doubles in Java?
Yes, you can convert an array of ints to an array of doubles by iterating through the int array and assigning each value to a corresponding position in the double array. For example:
“`java
int[] intArray = {1, 2, 3};
double[] doubleArray = new double[intArray.length];
for (int i = 0; i < intArray.length; i++) {
doubleArray[i] = intArray[i];
}
```
Are there any performance implications when converting int to double in Java?
While converting int to double is generally efficient, it may have performance implications in scenarios involving large datasets or high-frequency conversions due to the overhead of floating-point arithmetic. However, for typical use cases, the impact is negligible.
Can I use the Double.valueOf() method for converting int to double?
Yes, you can use the `Double.valueOf(int)` method to convert an int to a double. This method returns a Double object representing the specified int value. For example:
“`java
int intValue = 10;
Double doubleObject = Double.valueOf(intValue);
“`
In Java, converting an integer to a double is a straightforward process that can be accomplished in several ways. The most common method is through implicit type casting, where the integer value is automatically converted to a double when assigned to a double variable. This conversion is safe and does not result in data loss, as a double can represent a much wider range of values than an integer.
Another method for converting an integer to a double is by using the `Double.valueOf(int)` method or the `Double` constructor. Both approaches explicitly create a double object from an integer, which can be useful when working with collections or APIs that require object types. It is essential to choose the method that best fits the context of the application to ensure clarity and maintainability in the code.
Overall, understanding how to convert integers to doubles in Java is crucial for developers, especially when dealing with mathematical operations that require floating-point precision. By leveraging the built-in capabilities of the Java language, developers can efficiently manage type conversions without compromising performance or accuracy.
Author Profile
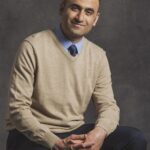
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?