How Can You Convert VARCHAR to DATE in SQL?
In the world of database management, handling data types efficiently is crucial for ensuring accuracy and performance. Among the various data types, `varchar` and `date` play significant roles, particularly when it comes to storing and manipulating date-related information. However, converting a `varchar` representation of a date into a proper `date` format can often be a source of confusion for many developers and database administrators. Whether you’re dealing with user input, legacy systems, or data imports, mastering this conversion process is essential for maintaining data integrity and facilitating effective queries.
The conversion of `varchar` to `date` in SQL is not just a technical necessity; it’s an integral part of data cleansing and validation. When dates are stored as strings, they can lead to inconsistencies and errors in calculations, comparisons, and reporting. Understanding the nuances of this conversion process allows developers to streamline their workflows and enhance the reliability of their applications. Different SQL dialects may offer various functions and methods for achieving this conversion, each with its own syntax and best practices.
As we delve deeper into this topic, we will explore the common challenges faced during the conversion process, the specific SQL functions available for different database systems, and practical examples to illustrate the best approaches. By the end of this article, you will be
Understanding Varchar and Date Types in SQL
In SQL, the `varchar` data type is used to store variable-length strings, which can include a wide range of characters. On the other hand, the `date` data type is specifically designed to store date values in a format that allows for various date-related operations. Converting `varchar` to `date` is a common requirement when dealing with data that has been incorrectly formatted or imported as strings.
When converting `varchar` to `date`, it is crucial to ensure that the string format matches the expected date format in your database system. Different SQL dialects may have different functions for performing this conversion, and the format of the date string can significantly affect the success of the operation.
Conversion Functions by SQL Dialect
Different SQL databases provide various functions for converting `varchar` to `date`. Below are some common examples:
- SQL Server: Use the `CAST` or `CONVERT` functions.
- MySQL: Use the `STR_TO_DATE` function.
- PostgreSQL: Use the `TO_DATE` function.
- Oracle: Use the `TO_DATE` function.
Examples of Conversion
Here are some examples of how to convert `varchar` to `date` in different SQL dialects:
SQL Dialect | Conversion Example |
---|---|
SQL Server |
|
MySQL |
|
PostgreSQL |
|
Oracle |
|
Handling Errors in Conversion
When converting `varchar` to `date`, it is essential to handle potential errors due to format mismatches. Here are some strategies to consider:
- Use TRY_CONVERT or TRY_CAST (SQL Server): These functions return `NULL` instead of throwing an error if the conversion fails.
- Check Format Before Conversion: Ensure that the `varchar` string is in the correct format before attempting to convert it.
- Use Regular Expressions: In databases that support it, you can use regular expressions to validate the format of the date string prior to conversion.
Adopting these practices will enhance the robustness of your SQL queries and minimize the occurrence of runtime errors.
Methods to Convert Varchar to Date in SQL
Converting a `VARCHAR` data type to a `DATE` type in SQL can vary based on the SQL database management system (DBMS) being used. The following sections outline common methods across popular systems such as SQL Server, MySQL, and PostgreSQL.
SQL Server
In SQL Server, you can use the `CONVERT` or `CAST` functions to change a `VARCHAR` to a `DATE`.
Using CONVERT:
“`sql
SELECT CONVERT(DATE, ‘2023-10-15’, 120) AS ConvertedDate;
“`
Using CAST:
“`sql
SELECT CAST(‘2023-10-15’ AS DATE) AS ConvertedDate;
“`
Important Note: The format of the `VARCHAR` string must be compatible with the expected date format. If the string is not in an acceptable format, SQL Server will return an error.
MySQL
In MySQL, the `STR_TO_DATE` function is commonly used for conversion.
Example:
“`sql
SELECT STR_TO_DATE(’15-10-2023′, ‘%d-%m-%Y’) AS ConvertedDate;
“`
Common Format Specifiers:
- `%Y` – Four-digit year
- `%m` – Two-digit month
- `%d` – Two-digit day
You can also use the `CAST` function similarly to SQL Server:
“`sql
SELECT CAST(‘2023-10-15’ AS DATE) AS ConvertedDate;
“`
PostgreSQL
In PostgreSQL, you can convert `VARCHAR` to `DATE` using the `TO_DATE` function.
Example:
“`sql
SELECT TO_DATE(’15-10-2023′, ‘DD-MM-YYYY’) AS ConvertedDate;
“`
Alternative Method:
You can also use the `CAST` function:
“`sql
SELECT ‘2023-10-15’::DATE AS ConvertedDate;
“`
Common Errors and Troubleshooting
When performing conversions, several common errors may arise:
- Invalid Format: The string may not conform to the expected date format.
- Out-of-range Dates: Dates that are out of the acceptable range for the database will result in errors.
- Locale Settings: Different databases might have different default locale settings affecting date formats.
Tips for Troubleshooting:
- Always ensure the format of the `VARCHAR` string matches the expected input format.
- Use error handling techniques provided by the database to capture and manage conversion errors.
- Test conversions with a variety of date formats to ensure robustness.
Performance Considerations
When converting large datasets, performance can be impacted. Here are some considerations:
- Index Usage: If the column being converted is indexed, performance may degrade as conversions on indexed columns do not utilize the index effectively.
- Batch Processing: Consider processing data in smaller batches to improve performance and reduce locking issues.
- Data Validation: Pre-validate the `VARCHAR` data to ensure it can be converted before executing conversion commands. This minimizes errors during large-scale operations.
By following the outlined methods and considering potential issues, you can effectively convert `VARCHAR` to `DATE` in SQL across various systems.
Expert Insights on Converting Varchar to Date in SQL
Dr. Emily Carter (Database Architect, Tech Solutions Inc.). “When converting varchar to date in SQL, it is crucial to ensure that the varchar format matches the expected date format of your SQL database. Utilizing the CONVERT function with the appropriate style code can prevent errors and ensure data integrity.”
James Liu (Senior SQL Developer, Data Innovations Group). “I recommend using the TRY_CONVERT function in SQL Server, as it provides a safer approach by returning NULL for invalid conversions instead of throwing an error. This can be particularly useful when dealing with inconsistent data formats.”
Maria Gonzalez (Data Analyst, Insight Analytics). “Always validate your data before conversion. Implementing checks for invalid date entries in varchar fields can save significant troubleshooting time later. A clean dataset leads to more reliable date conversions.”
Frequently Asked Questions (FAQs)
What is the basic syntax to convert varchar to date in SQL?
The basic syntax to convert a varchar to date in SQL is `CAST(your_column AS DATE)` or `CONVERT(DATE, your_column, style)`, where `style` is an optional parameter that defines the format of the date.
What are the common formats for the varchar date string?
Common formats include ‘YYYY-MM-DD’, ‘MM/DD/YYYY’, and ‘DD-MM-YYYY’. The format must match the expected input for successful conversion.
What SQL function can be used to handle different date formats during conversion?
The `CONVERT()` function allows you to specify a style code that corresponds to the format of the date string, enabling the handling of various date formats.
What happens if the varchar string cannot be converted to a date?
If the varchar string cannot be converted to a date, SQL will raise an error indicating that the conversion failed. It is advisable to validate the format before conversion.
How can I ensure successful conversion from varchar to date?
To ensure successful conversion, validate the varchar format against expected date formats, use appropriate style codes with `CONVERT()`, and handle potential errors using `TRY_CONVERT()` or similar error handling techniques.
Can you convert varchar to datetime in SQL as well?
Yes, you can convert varchar to datetime in SQL using the same functions: `CAST(your_column AS DATETIME)` or `CONVERT(DATETIME, your_column, style)`, allowing for more precise date and time representation.
Converting a varchar to a date in SQL is a common task that allows for the manipulation and analysis of date-related data stored as strings. Various SQL databases provide specific functions to perform this conversion, such as the `CAST()` and `CONVERT()` functions in SQL Server, or the `TO_DATE()` function in Oracle SQL. Understanding the syntax and format requirements of these functions is crucial for successful conversion, as improper formats can lead to errors or incorrect data interpretations.
One of the key takeaways is the importance of ensuring that the varchar data is in a recognizable date format before attempting conversion. This may involve using string manipulation functions to reformat the data into a standard format, such as ‘YYYY-MM-DD’. Additionally, awareness of the database’s default date format settings can help prevent conversion issues. It is also advisable to handle potential exceptions or errors that may arise from invalid date strings during the conversion process.
In summary, successfully converting varchar to date in SQL requires a solid understanding of the specific functions available in the SQL dialect being used, as well as the formats and potential pitfalls associated with date strings. By following best practices and ensuring data integrity, users can effectively manage date conversions within their SQL queries and applications.
Author Profile
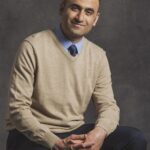
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?