How Can You Convert a String to JSON in Java?
In today’s data-driven world, the ability to seamlessly convert strings to JSON in Java is an essential skill for developers. As applications become increasingly reliant on structured data interchange formats, understanding how to manipulate JSON effectively can significantly enhance your programming toolkit. Whether you’re building web services, handling API responses, or simply managing data storage, mastering this conversion process will empower you to create more efficient and robust applications. In this article, we will explore the nuances of converting strings to JSON in Java, equipping you with the knowledge to tackle common challenges and optimize your code.
Java, with its rich ecosystem and extensive libraries, provides several methods for converting strings into JSON objects. This process typically involves parsing a string representation of JSON data and transforming it into a format that Java can easily manipulate. While the core concept is straightforward, the intricacies of handling various data types, managing exceptions, and ensuring compatibility with JSON standards can present challenges for developers.
As we delve into this topic, we will examine popular libraries like Jackson and Gson, which are widely used for JSON processing in Java. Each library offers unique features and benefits, making it crucial to understand their differences and how they can fit into your development workflow. By the end of this article, you’ll be well-equipped to convert strings to JSON in Java,
Using JSON Libraries in Java
To convert a string to JSON in Java, you typically utilize libraries that facilitate JSON parsing and serialization. Two popular libraries for handling JSON in Java are Jackson and Gson. Both libraries are robust and provide comprehensive features for converting between Java objects and JSON strings.
Converting String to JSON with Gson
Gson is a library developed by Google that can easily convert Java Objects into their JSON representation and vice versa. To convert a string to a JSON object, you can follow these steps:
- Include the Gson dependency in your project. If you’re using Maven, add the following to your `pom.xml`:
“`xml
“`
- Use the `Gson` class to convert your string to a JSON object. Here’s an example:
“`java
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
JsonObject jsonObject = JsonParser.parseString(jsonString).getAsJsonObject();
System.out.println(jsonObject);
}
}
“`
Converting String to JSON with Jackson
Jackson is another widely-used library for processing JSON in Java. It provides a more extensive set of features compared to Gson, including support for streaming and data binding.
- Include the Jackson dependency in your project. For Maven, add this to your `pom.xml`:
“`xml
“`
- Use the `ObjectMapper` class to convert a string to a JSON node. Here’s an example:
“`java
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public class JacksonExample {
public static void main(String[] args) throws Exception {
String jsonString = “{\”name\”:\”Jane\”, \”age\”:25}”;
ObjectMapper objectMapper = new ObjectMapper();
JsonNode jsonNode = objectMapper.readTree(jsonString);
System.out.println(jsonNode);
}
}
“`
Comparison of Gson and Jackson
Both libraries have their strengths and can be chosen based on the requirements of your project. Below is a comparison table highlighting key differences:
Feature | Gson | Jackson |
---|---|---|
Performance | Good for small to medium data | Excellent performance for large datasets |
Ease of Use | Simple and easy to use | More complex, more features |
Data Binding | Limited data binding capabilities | Advanced data binding features |
Streaming API | Not available | Supports streaming |
The choice between Gson and Jackson often depends on the specific needs of your application, including performance requirements and ease of integration.
Methods for Converting String to JSON in Java
Java provides several libraries for converting strings to JSON objects, with two of the most popular being Jackson and Gson. Each library has its own methods and advantages.
Using Jackson Library
Jackson is a widely used library for processing JSON data in Java. To convert a string to a JSON object using Jackson, follow these steps:
- Add Jackson Dependency: Include the following dependency in your `pom.xml` if you are using Maven:
“`xml
“`
- Convert String to JSON:
“`java
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
ObjectMapper objectMapper = new ObjectMapper();
try {
JsonNode jsonNode = objectMapper.readTree(jsonString);
System.out.println(jsonNode);
} catch (Exception e) {
e.printStackTrace();
}
}
}
“`
- Explanation:
- `ObjectMapper` is the main class used for conversions.
- `readTree` method parses the string and converts it to a `JsonNode` object.
Using Gson Library
Gson is another popular library for JSON processing in Java, developed by Google. To convert a string to a JSON object using Gson, follow these steps:
- Add Gson Dependency: Include the Gson library in your `pom.xml`:
“`xml
“`
- Convert String to JSON:
“`java
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
public class GsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
JsonObject jsonObject = JsonParser.parseString(jsonString).getAsJsonObject();
System.out.println(jsonObject);
}
}
“`
- Explanation:
- `JsonParser` is used to parse the string.
- The method `parseString` converts the string to a `JsonObject`.
Comparison of Jackson and Gson
Feature | Jackson | Gson |
---|---|---|
Performance | Generally faster | Slightly slower |
Data Binding | Comprehensive support | Limited to simple objects |
Annotations | Extensive support | Limited support |
Readability | JSON tree model | Direct model mapping |
Streaming Support | Yes | Yes |
Handling Exceptions
When converting strings to JSON, it is essential to handle exceptions properly:
- Jackson: Use `JsonProcessingException` to catch errors related to JSON parsing.
- Gson: Use `JsonSyntaxException` for catching syntax errors in JSON strings.
Example for Jackson:
“`java
try {
JsonNode jsonNode = objectMapper.readTree(jsonString);
} catch (JsonProcessingException e) {
System.out.println(“Invalid JSON format”);
}
“`
Example for Gson:
“`java
try {
JsonObject jsonObject = JsonParser.parseString(jsonString).getAsJsonObject();
} catch (JsonSyntaxException e) {
System.out.println(“Invalid JSON syntax”);
}
“`
These methods and practices enable efficient conversion of strings to JSON in Java, catering to different project requirements and preferences.
Expert Insights on Converting Strings to JSON in Java
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When converting strings to JSON in Java, it is crucial to utilize libraries such as Jackson or Gson, as they provide robust parsing capabilities and handle edge cases effectively, ensuring that the resulting JSON is both valid and usable.”
Michael Chen (Lead Java Developer, CodeCraft Solutions). “Understanding the structure of the string data is essential before conversion. Properly formatted strings can be seamlessly transformed into JSON objects, while malformed strings can lead to runtime exceptions that require careful debugging.”
Sarah Lopez (Java Architect, Enterprise Systems Group). “It is advisable to implement error handling when converting strings to JSON. Utilizing try-catch blocks can help manage exceptions that arise from invalid input, thereby enhancing the reliability of your application.”
Frequently Asked Questions (FAQs)
What is the process of converting a string to JSON in Java?
To convert a string to JSON in Java, you typically use a library such as Jackson or Gson. You can parse the string using the library’s methods to create a JSON object, allowing for easy manipulation and access to the data.
Which libraries are commonly used for JSON conversion in Java?
The most commonly used libraries for JSON conversion in Java are Jackson, Gson, and org.json. Each library offers different features and performance characteristics, so the choice depends on the specific requirements of your project.
How do I handle exceptions when converting a string to JSON?
When converting a string to JSON, it is essential to handle exceptions such as JsonParseException or JsonSyntaxException. You can use try-catch blocks to manage these exceptions and ensure that your application can gracefully handle invalid JSON strings.
Can I convert a JSON string into a Java object?
Yes, you can convert a JSON string into a Java object using libraries like Jackson or Gson. These libraries provide methods to deserialize JSON strings into Java objects, allowing you to work with the data in a structured format.
What are the common pitfalls when converting strings to JSON in Java?
Common pitfalls include improperly formatted JSON strings, which can lead to parsing errors, and failing to match the JSON structure with the Java object structure during deserialization. Always validate your JSON format and ensure that your Java classes are compatible with the JSON structure.
Is it possible to convert JSON back to a string in Java?
Yes, you can convert JSON back to a string in Java using libraries like Jackson or Gson. These libraries provide methods to serialize Java objects into JSON strings, allowing for easy data transmission and storage.
Converting a string to JSON in Java is a common task that developers encounter when working with data interchange formats. Java provides various libraries that facilitate this conversion, with popular choices including Jackson, Gson, and org.json. Each of these libraries offers methods to parse strings and convert them into JSON objects, allowing developers to manipulate and access data easily.
One of the primary considerations when converting strings to JSON is ensuring that the input string is properly formatted. A well-structured JSON string adheres to specific syntax rules, such as using double quotes for keys and values, and correctly nesting objects and arrays. Failure to comply with these rules can lead to parsing errors, which necessitates proper error handling in the code.
Additionally, choosing the right library can significantly impact performance and ease of use. For instance, Jackson is known for its speed and efficiency, making it suitable for large data sets, while Gson is appreciated for its simplicity and ease of integration. Understanding the strengths and weaknesses of each library can help developers make informed decisions based on their specific project requirements.
converting strings to JSON in Java is a straightforward process when utilizing the appropriate libraries and adhering to JSON formatting standards. By leveraging these tools effectively, developers can enhance
Author Profile
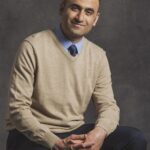
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?