Why Am I Seeing ‘Could Not Determine Recommended JDBCTYPE for Java Type?’ – Common Causes and Solutions
In the world of Java database connectivity, developers often encounter a myriad of challenges that can hinder their progress. One such issue that frequently arises is the perplexing error message: “could not determine recommended jdbctype for java type.” This seemingly cryptic notification can leave even seasoned programmers scratching their heads, as it disrupts the seamless interaction between Java applications and databases. Understanding the root causes of this error is crucial for any developer looking to maintain efficient data handling and ensure robust application performance.
At its core, this error message signifies a mismatch or ambiguity in the data types being utilized within a Java application when interfacing with a database. Java’s rich type system, while powerful, can sometimes lead to confusion, particularly when the database expects a specific JDBC type that isn’t clearly defined. This situation can arise in various scenarios, such as when using frameworks like Hibernate or JPA, where automatic type mapping is essential for smooth operation. As developers navigate through these complexities, it becomes imperative to grasp the underlying principles of JDBC types and how they correspond to Java’s data types.
In the following sections, we will delve deeper into the intricacies of JDBC type mapping, explore common pitfalls that lead to this error, and provide practical strategies for troubleshooting and resolution. Whether you are a novice developer or a
Understanding JDBC Type Mappings
When working with Java Database Connectivity (JDBC), it’s essential to understand how Java types map to SQL types. This mapping is crucial for ensuring that data is correctly transmitted between Java applications and relational databases. JDBC provides a set of data types that correspond to SQL types, but sometimes developers encounter issues where the system cannot determine the appropriate JDBC type for a given Java type.
The inability to determine a recommended JDBC type may arise from several scenarios:
- Custom Java Types: If a developer uses custom or user-defined data types, JDBC may not recognize these types automatically.
- Mismatched Data Types: Differences in data type definitions between Java and SQL can lead to ambiguity.
- Driver Limitations: Some JDBC drivers may not support certain Java types, causing the system to fail in identifying the correct mapping.
Common Java to JDBC Type Mappings
Understanding the standard mappings can help mitigate the issue of type determination. Below is a table that outlines common Java types and their corresponding JDBC types:
Java Type | JDBC Type |
---|---|
String | VARCHAR |
int | INTEGER |
boolean | BOOLEAN |
double | DOUBLE |
java.sql.Date | DATE |
java.sql.Timestamp | TIMESTAMP |
Troubleshooting Type Determination Issues
To address the issue of “could not determine recommended JDBC type for Java type,” consider the following troubleshooting steps:
- Check Driver Compatibility: Ensure that the JDBC driver being used supports all relevant data types.
- Explicit Type Declaration: When possible, explicitly define the JDBC type in your SQL statements or prepared statements. For example:
“`java
PreparedStatement pstmt = connection.prepareStatement(“INSERT INTO table_name (column_name) VALUES (?)”);
pstmt.setObject(1, myObject, java.sql.Types.VARCHAR);
“`
- Inspect Data Type Mappings: Review the data types being passed to the database to ensure they align with the expected JDBC types.
- Logging and Debugging: Implement logging to capture the types being processed. This can help identify where the mismatch occurs.
Best Practices for JDBC Type Management
To prevent type determination issues, adhere to the following best practices:
- Use Standard Types: Stick to standard Java and JDBC data types whenever possible.
- Document Custom Types: If custom types are necessary, document them thoroughly and consider creating a utility method for mapping to JDBC types.
- Validate Input Data: Always validate input data types before sending them to the database.
- Regularly Update Drivers: Keep JDBC drivers up to date to leverage improvements and bug fixes related to type handling.
By following these guidelines, developers can minimize the risk of encountering type determination issues in JDBC operations.
Understanding the Error Message
The error message “could not determine recommended jdbctype for java type” typically arises in Java applications when there is a mismatch between Java data types and their corresponding JDBC (Java Database Connectivity) types. This issue often occurs during operations involving database interactions, such as executing queries or mapping Java objects to database tables.
Several factors can contribute to this error:
- Incompatible Data Types: The Java data type used does not have a clear equivalent in JDBC.
- Unrecognized Custom Types: If a custom Java type is not registered with the JDBC driver, it may lead to this error.
- Driver Limitations: Some JDBC drivers may not support certain Java types or may have specific requirements for type mapping.
Common Causes and Solutions
To address the error, it is essential to identify its root cause. Below are common causes along with their respective solutions:
Cause | Solution |
---|---|
Incompatible Java Type | Verify the Java type being used and ensure it maps correctly to JDBC. Use standard types like `String`, `int`, `Date`, etc. |
Custom Java Type | Register the custom type with the JDBC driver, or implement a proper type converter. |
Driver Support Issues | Check the documentation of the JDBC driver to confirm it supports the Java types being used. Consider updating the driver if necessary. |
Incorrect Configuration | Review the database configuration settings in your application for any discrepancies. |
Best Practices for Type Mapping
To minimize the occurrence of this error, adhere to the following best practices:
- Use Standard Data Types: Stick to standard Java data types that have well-defined JDBC counterparts.
- Implement Type Converters: For custom types, create a mapping strategy using converters to translate between Java and JDBC types.
- Review JDBC Documentation: Familiarize yourself with the specific JDBC driver’s documentation to understand its type mappings and limitations.
- Error Handling: Implement robust error handling to gracefully manage situations where type mismatches occur.
Debugging Steps
When encountering this error, follow these debugging steps to identify the underlying issue:
- Check the Stack Trace: Review the stack trace for additional context about where the error originated.
- Log Data Types: Log the data types being used in your database operations for analysis.
- Simplify Queries: Simplify your SQL queries to isolate the problematic data type.
- Test with Standard Types: Temporarily replace custom types with standard types to determine if the error persists.
- Consult Documentation: Refer to both the Java and JDBC driver documentation for insights on type compatibility.
By systematically addressing these areas, developers can effectively resolve the “could not determine recommended jdbctype for java type” error and enhance their application’s reliability in database interactions.
Understanding JDBC Type Determination Challenges
Dr. Emily Carter (Senior Database Architect, Tech Innovations Inc.). “The error message ‘could not determine recommended jdbctype for java type’ often arises when there is a mismatch between the Java data types and the expected JDBC types in the database schema. It is crucial to ensure that the Java types used in your application are correctly mapped to the corresponding JDBC types to avoid such issues.”
Mark Thompson (Lead Java Developer, Enterprise Solutions Group). “This problem typically indicates that the JDBC driver is unable to infer the correct SQL type from the Java type provided. Developers should explicitly define the mappings in their code or configuration files to prevent ambiguity and ensure smooth data handling between Java applications and databases.”
Lisa Chen (Database Consultant, Data Dynamics). “When encountering the ‘could not determine recommended jdbctype for java type’ error, it is advisable to review the documentation of the JDBC driver being used. Different drivers may have varying levels of support for Java types, and understanding these nuances can help in resolving the issue effectively.”
Frequently Asked Questions (FAQs)
What does the error “could not determine recommended jdbctype for java type” mean?
This error indicates that the application is unable to map a specific Java type to a corresponding JDBC type. This can occur when the Java type is not recognized or supported by the JDBC driver.
What are common causes for this error?
Common causes include using unsupported Java data types, misconfiguration of the database connection, or incorrect type mappings in the ORM framework being used.
How can I resolve the “could not determine recommended jdbctype for java type” error?
To resolve this error, ensure that you are using supported Java types. Verify the configuration settings in your ORM or JDBC connection and consult the documentation for the specific database and driver being used.
Are there specific Java types that frequently cause this issue?
Yes, types such as custom objects, certain collections, or unsupported primitive types may frequently lead to this issue. Always check the compatibility of your Java types with the JDBC driver.
What should I do if I need to use a custom Java type?
If you need to use a custom Java type, consider implementing a custom type handler or converter that explicitly maps your Java type to a corresponding JDBC type recognized by the database.
Is there a way to get more detailed information about this error?
Yes, enabling detailed logging for your ORM or JDBC framework can provide more context about the error. This may include stack traces or additional error messages that can help diagnose the issue further.
The error message “could not determine recommended jdbctype for java type” typically arises in Java applications that interact with databases using JDBC (Java Database Connectivity). This issue often indicates that the Java type being used does not have a corresponding JDBC type that can be automatically inferred by the framework or library in use. This can occur due to custom types, unsupported data types, or misconfigurations in the database connection settings.
To resolve this issue, developers should ensure that the Java types being utilized are compatible with the JDBC types. This may involve explicitly specifying the JDBC type in the code or configuration files. Additionally, reviewing the database schema and ensuring that the data types defined there are supported by the JDBC driver being used can help mitigate this problem. It is also advisable to consult the documentation of the specific JDBC driver for guidance on supported data types.
In summary, understanding the relationship between Java types and JDBC types is crucial for effective database interactions. By maintaining compatibility and ensuring proper configurations, developers can avoid the pitfalls associated with this error message. Ultimately, thorough testing and validation of data types can lead to more robust and error-free database operations in Java applications.
Author Profile
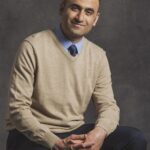
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?